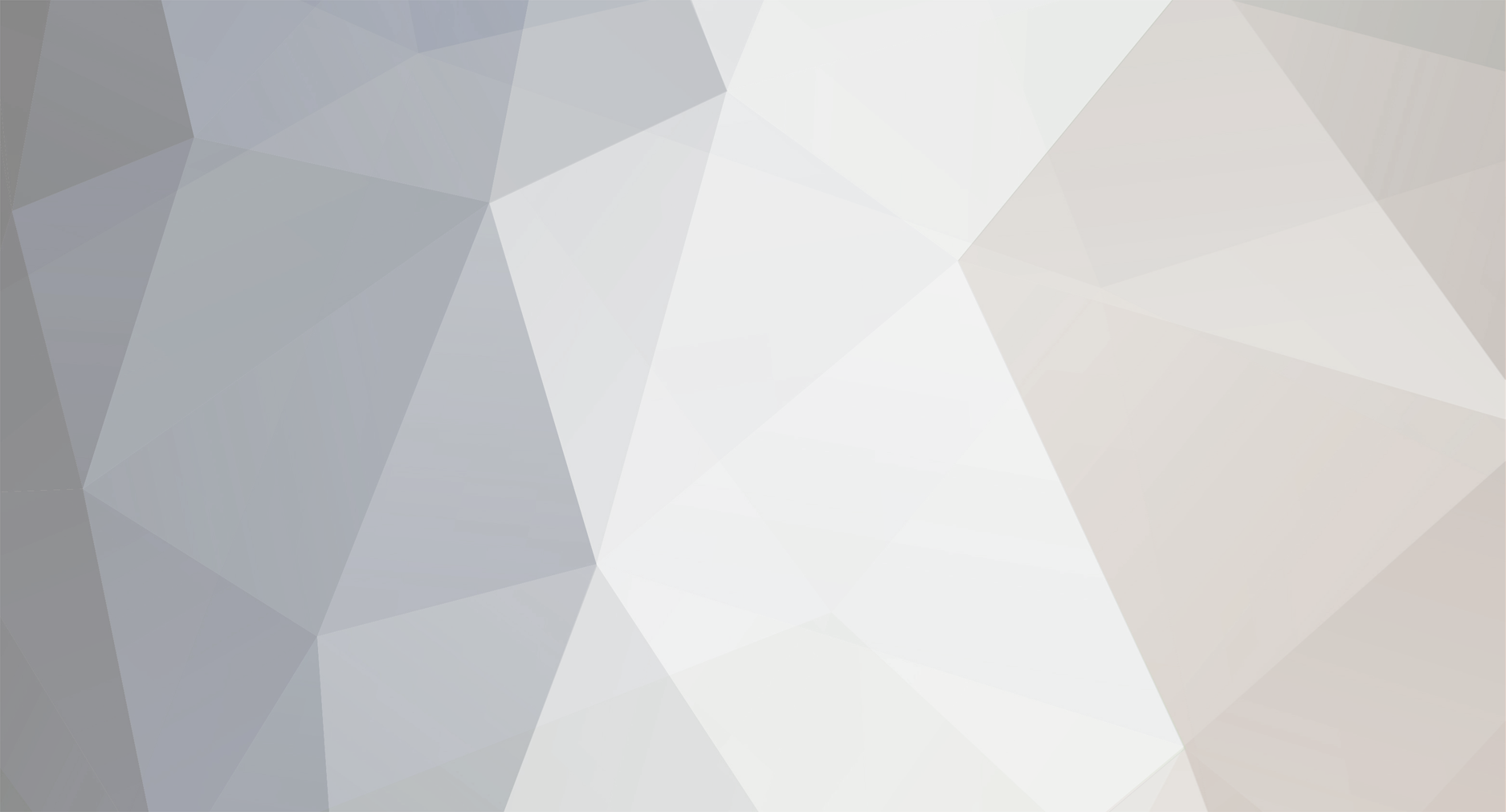
Jenk
Members-
Posts
778 -
Joined
-
Last visited
Never
Everything posted by Jenk
-
Then why are you posting in the "O.O.P. Help" sub-forum?
-
<?php $name = new test(); ?> Instantiates an object. If you are trying to call a function called test(), the above code will throw an error. <?php $name = &test(); ?> Returns a reference to whatever is being returned by the test function. These are two completely different things. Best, Patrick but what I said still applies. The assignment operators in PHP4 do not pass objects by reference unless you tell them to, with the reference operator.
-
php4 does not pass objects by reference, so it is mandatory to use =&, unless you want copies of your objects everywhere.
-
yes, or not overload the method. <?php class A { public function __call($name, $args) { // etc. } } class B extends A { } $b = new B; $b->SomeMethod(); ?>
-
Create stubs to tally class/method calls.
-
It'll also save a heck of a lot of time.. <?php $reflect = new ReflectionClass('SomeClass'); echo $reflect->getFileName(); echo $reflect->getParentClass()->getName(); echo $reflect->getParentClass()->getConstructor()->getName(); //etc. ?>
-
Use reflection, don't parse files.
-
Hint: <?php switch (true) { case ($var < 10): doSomething(); break; } ?>
-
Using an oject reference directly return from a function call
Jenk replied to johnwarde's topic in PHP Coding Help
It's only possible in PHP5+. The above people are only correct if they are referring to php4. -
[SOLVED] Predefined Variable not working as expected
Jenk replied to scottybwoy's topic in PHP Coding Help
-
You could call the function at the point where you would store the page in a collection; ergo just skipping the collection all together. so for a brief example: <?php $array = array(); for ($i = 0; $i < 10; $i++) { $array[$i] = $i; } foreach ($array as $var) { doSomething($var); } // change to.. for($i = 0; $i < 10; $i++) { doSomething($i); } ?> That may seem quite a weak example, but the logic is still the same.
-
Just use what is suitable to your needs.
-
I meant do you have a problem that requires the visitor pattern to solve it It's very difficult to just "implement" a pattern without a problem to solve. Visitor pattern can be metamorph'd as "A guest comes to your house, for every guest you offer a cup of tea. The guest is then free to do what it likes with that cup of tea."
-
Do you have a reason to use the visitor pattern, or do you simply want to learn about it?
-
If you want to use a different DB class (but it must have the same interface) it would not be a problem at all, you just provide a different object.. <?php $user1 = new User(new DB()); $user2 = new User(new OtherDB()); ?> add extra arguments, or extra methods for setting the objects, as shown above by Patrick.
-
Option 3: <?php class User { private $_db; public function __construct (DB $db) { $this->_db = $db; } public function doSomething() { //do something with $this->_db } } $user = new User(new DB()); ?>
-
Learning the purpose is needed before learning the mechanics. You can't teach someone how to construct an engine if they don't know it will be used to generate motion. Same goes for programming. You can't just tell someone to create a class/object until they understand the requirements of said object, and the purpose for it.
-
MVC has been around for 30+ years, it is tried and tested, not only popular. A lot of people will also find they have been doing what is known as MVC and not even know it.
-
Note that using the scope resolution operator superflously is a code smell; you should be passing your objects (example, you user object) around as a parameter instead of using the pseudo global that is the scope resolution operator. There are a few circumstances where the SRO is warranted, but this is certainly not one of them.
-
interface DoesSomething { public function doSomething (); } class Foo { public function bar (DoesSomething $obj) { $obj->doSomething(); } } It assures that $obj conforms to the interface of DoesSomething; if it didn't - it may not have the method doSomething() which could have catastophic results compared to the light-weight error received for not implementing the interface.
-
Calling a class from another file in a function?
Jenk replied to rockinaway's topic in PHP Coding Help
It's still a code smell. Negates the entire reason for having a modular application, but it breaks the capsulation by having a direct and explicit dependency on not only a global value, but also the name of the variable containing that value. It is much better practice to present that value as a parameter to your object. -
Calling a class from another file in a function?
Jenk replied to rockinaway's topic in PHP Coding Help
"global" is a code smell. Pass your objects and variables around, as they should be handled. -
Fatal error: Class 'Test' not found in..... - Creating a class instance
Jenk replied to webbiz's topic in PHP Coding Help
Start by noting down the directory you are currently in, then adjust includes accordingly.. echo realpath('.'); to see current working directory, then you can make a relative path accordingly. -
keep php sessions going until web browser closed
Jenk replied to arianhojat's topic in PHP Coding Help
All sessions remain active until timeout, or a call to session_destroy(). It is impossible to reliably have the users browser trigger a session_destroy() as the browser is closed.