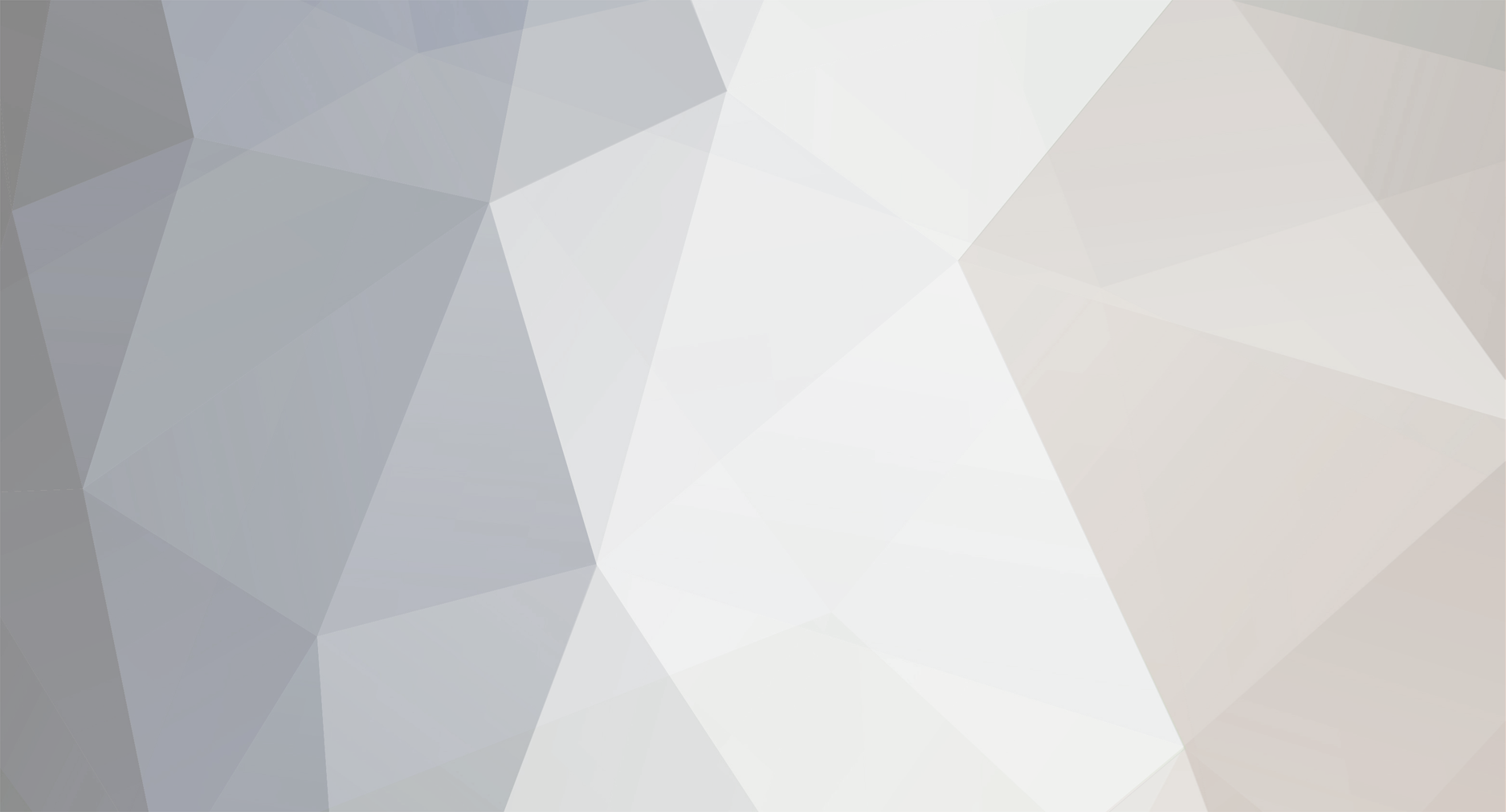
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
You're processing the radio buttons correctly. However, the overall design of your form handler needs to be addressed. There are two steps you need to take, in order: 1. Check to see if the form has been submitted 2. Check each form input manually So, the form handler should have the structure of: if(isset($_POST['submit'])) { if(isset($_POST['name']) && isset($_POST['email'])) { //run regex checks } else { //non-existent data error } //... } Essentially, you need to check for the existence of an input value before you can use it. Also, you shouldn't use the $_REQUEST array. Use the one you specify in your form's method, in this case $_POST. Right now, your e-mail script is open to processing data sent via query string (i.e., in the url), which you probably don't want.
-
Can you show an example of your table code? I have the feeling it could be failing because of multiple elements with the same id, but would like to be sure.
-
We're not telepathic. 'Not working' is hardly descriptive of the problem, and you have multiple if-conditionals. What precisely is the problem, and which 'if' is the cause?
-
If you use Firefox, get the SQL Inject Me addon. It'll scan whatever site you're currently visiting for the possibility of an injection attack. It runs over 300 tests, so chances are if it passes, you're pretty safe. There's also an XSS Me addon that does the same thing for XSS attacks.
-
Where is the test to find out what % you are in regex? I wonder what my overall PHP coding skill % is at... My skill in PHP is over 9000....
-
Well, the problem stems from a lack of clarity of what an object like this should do, and how it should be invoked. First, if you have PHP 5+, use its OOP syntax. Second, one of the main points behind OOP is to decouple the nitty-gritty code from the main system. Unfortunately, your class does the opposite. The class shouldn't be concerning itself with whether or not the submit button has been pressed, for example. That's for the system code to worry about. Without getting too far into OOP design (which is a topic too broad for this post), to get fast results with relatively good code, do something like: class SimpleValidator { private $regEx = '/[A-Za-z]+/'; public function validate($data) { if (!empty($data) && preg_match($regEx, $data)) { return "Name successfully entered."; } else { return false; } } //end of the class code if (isset($_POST['submit'])) { $validator = new SimpleValidator(); $result = $validator->validate($_POST['name']); if ($result) { echo $result } else { echo "Something went wrong"; } }
-
Ah, yes, implode. I always forget about that one.
-
You can't echo it directly as $_POST['fname'] is an array. So, you'll need to loop through it: foreach ($_POST['fname'] as $value) { echo "$value "; }
-
Ah, okay, sorry for the misunderstanding. There have been a lot of people who swear by relying on global, so I misread your intent.
-
Good grief, some of you are young.
-
Unless they're returned from the function and assigned to a variable outside of the function's scope. Two different variables, yes, but both contain the same value. Sure it would. Again, that's what argument lists are for. function displayAmount($shopConfig, $amount) { return $shopConfig['currency'] . number_format($amount); } Same result, but better code.
-
Untrue. getShopConfig() returns $shopConfig. Simply pass that returned value into displayAmount() through the function's argument list. Using global is a sign of bad design, and isn't necessary to use.
-
A quick idea would be: User arrives at your site. Since they're there for the first time, there's no action to process, so the controller displays the default page, which includes your login form. Once the user enters their info, it's sent to the controller (most likely through a postback). The controller then decides what to do (was the user attempting to log in? write a comment? etc), and funnels it to the mechanism that will validate the data. The data is either accepted, and the user is successfully logged in, or rejected, and the user is denied. Either way, the logic updates the page (view) with the status of the login attempt. So, essentially, you'll have a controller sitting 'on top' of your application, retrieving request data and sending it to the right sub-system to be processed. The sub-system will then update the view, either directly, or through the controller.
-
Heard about this in the spring, but apparently today is D-day. As much as I love the web today, I miss the kind of small-scale, home brew atmosphere the internet had in spades in the late 90's/early 2000's. It really felt like a digital frontier back then, where small clusters of enterprising nerds would create fan sites for their favorite game, anime, or manga. Not that it doesn't happen today, but the rough edges made everything feel more quaint. Nothing quite like being on one of my campus' linux boxes surfing to find a good Phantasy Star fan site.
-
You may not want your site to look 'typical', but adding extra bits like bright red question marks as hyperlinks is not the way to go. Red is the color of attention, but also of failure. Also, the question marks themselves don't give a hint that they're links. Overall, they're distracting, and should go. Why do you have a contact form if anyone can call or e-mail you given the info in your header? You should rewrite your introduction. You may not like writing a sales pitch, but they're necessary. Statistics don't demand anything. And telling potential clients that you only have 15 seconds to make a good impression, then essentially say "This is what I can do. I'm not going to go out of my way to convince you to hire me," is a very bad decision. Let me put it this way: if the choice is between hiring you based on your site, and another person/company based on a site that appears to actually want my business, I'm going to pick the second choice every time. Especially if the former has nothing more than a few links to incomplete sites as their portfolio. And, yes, the footer looks chunky. At the very least, use a smaller font. Footers like yours are a site's fine print. Emphasis on fine.
-
I don't think anyone is going to rewrite the whole thing for you and include form validation on a whim. You'll most likely need to work on it yourself (and we'll help you along the way with specific answers to specific problems), or you'll need to pay someone for that kind of work. That said, here's where you should look: $message is never set. I'm guessing that it's supposed to contain the values of the other variables, but you never actually do it. Thus, no message is in the e-mail.
-
[SOLVED] Get the ID of cells in a table inside a div ..
KevinM1 replied to mwl707's topic in Javascript Help
To obtain the ids, you'll need to do something similar to what I had you do in the last thread. var oTable = document.GetElementById('visi'); var cells = oTable.getElementsByTagName('div'); for(var i = 0; i < cells.length; ++i) { alert("Cell id: " + cells[i].id); } -
I don't mind WoW's look. It's been a conscious decision by Blizzard to keep the system requirements to play the game low, hence the lack of noticeable upgrade in the last five years. The thing can be played on computers built in the late 90's. Yeah, WoW has a variety of skills a character can learn. There are three secondary professions anyone can learn - cooking, first aid, and fishing. Everyone also has two primary professions they can learn. The choices are available to all, but you're limited to two. Some are 'gather' professions that allow you to obtain the materials necessary for the 'crafting' professions. Examples include herbalism and mining. The 'crafting' professions allow you to make stuff. Examples include blacksmithing and alchemy. My main is an herbalist/enchanter. Herbs can be sold for decent money, and enchanting materials can be expensive.
-
[SOLVED] Get the ID of cells in a table inside a div ..
KevinM1 replied to mwl707's topic in Javascript Help
Can you show the resulting HTML of the table? -
Not true! XR is a prime place to hear chuck norris jokes! I think Horde are getting kinda short-sticked on the new race though. Goblin vs. Worgen? WTF??? That's not a fair tradeoff. Should have given ally furblogs or else given horde something cooler to match the worgen. Just about anything short of another Dranaei retcon would've been better than goblins.
-
Hey, nothing wrong with fighting for the whores For me, Cataclysm has three things going for it: 1. Reshaped Azeroth. I miss the days when The Crossroads actually felt like a crossroads. I would love to recapture some of the old magic when I was leveling my Orc Hunter back in the day. Now, even Outland is barren, used primarily as a transportation hub or place to solo until one reaches Northrend. 2. New races and starting areas. The Goblins don't interest me much. I'm not a fan of the game's jungle areas, and the Goblins themselves don't seem very compelling. Worgen, on the other hand, sound pretty cool in that over-the-top way. From what I've seen, Gilneas - their starting area - is this sort of dreary, Victorian place, slowly rotting away due to the curse. The story progression will be sort of like that for Death Knights - taking place over several years, with some set pieces thrown in. 3. New race/class combinations. I always wanted a Human Hunter, so when the expansion hits, I'll be able to roll one up. I have three characters waiting for me: Level ~62 Blood Elf Paladin. Currently ret spec. Level 58 Blood Elf Death Knight. Haven't done much with them. Blood spec, I believe. Level 20-something Human Paladin. My Alliance guy. That's it. I like playing the easy classes due to the way my hands work. I can't use a setup where both movement and action are done on the keyboard, so I pick classes that allow me to click without much penalty. Can't get much simpler than a Paladin.
-
I play WoW occassionally. I sign up when I'm bored, play for a month or so, then suspend my account. I've played from launch with various lowbie characters, and my main, whom I've had since TBC came out, has only recently got to Outland (he's ~level 62). I'm a very casual player. I just don't feel the need to rush to the level cap. The upcoming Cataclysm expansion pack looks interesting. It's nice that they're breathing new life into old Azeroth, and the worgen are right in my wheelhouse for a race. I'm mostly a Horde player (BE Paladin) because none of the Alliance races interest me. The Dranaei didn't do it for me. I'll probably sign back up in a week or so in order to get the vanity pet for transferring my account to a Battle.net account, and the mini-Onyxia vanity pet for logging on during their anniversary. I'm very interested in Bioware's Star Wars: The Old Republic. The initial preview vids look promising. Sith? Yes, please.
-
An even better solution would be to simply crate an associative array of capitols to country: $capsToCountries = array("London" => "England", "Paris" => "France", /* etc */); foreach($capsToCountries as $cap => $country) { echo "The capitol of $country is $cap"; }
-
Your submit button needs a name. In this case, it should be 'posted' (without the single quotes) because that's what you're checking to see is set before echoing out the result. You also need to ensure that all non-variable array keys are in quotes. Also, when echoing arrays in a string, I find it useful to use curly brackets, like so: echo "The capital of {$_POST['country']} is <b>{$country_capital[$counter]}</b>"; When you get into more advanced code, like retrieving values from a database, you'll save yourself some headaches doing it that way.
-
ASP.NET - Form Not Inserting into Database - C#
KevinM1 replied to twilitegxa's topic in Other Programming Languages
Which error message is it displaying? One from failing validation, or the one from failing the insert itself? EDIT: Why have you hard wired the EmployeeID value? If you're using it as the primary key of the HelpDesk table, the insert will only work once.