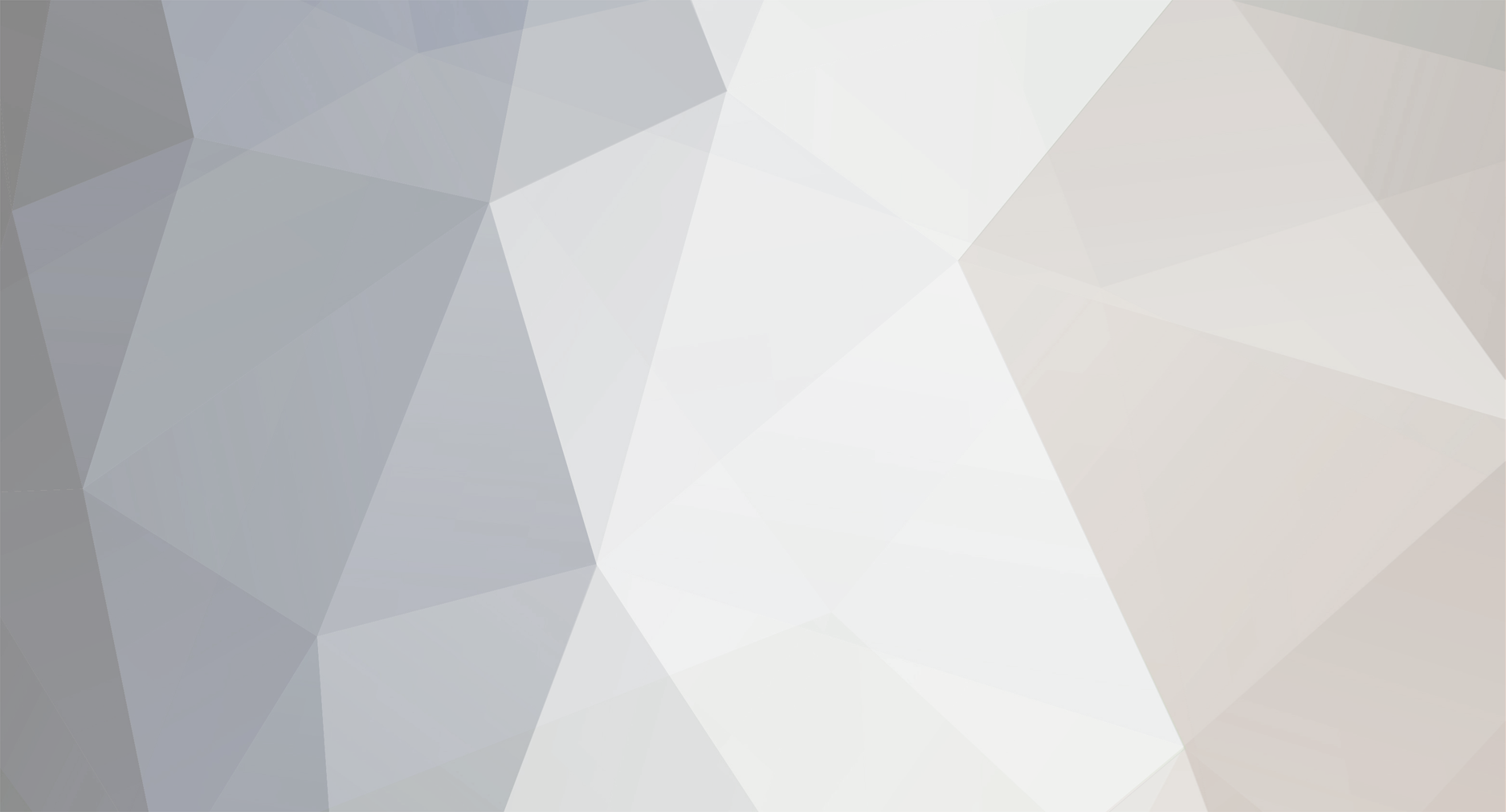
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Here's a hint, with all the subtlety of a sledgehammer to the forehead: PHP isn't telepathic. Just because you think there should be a function called getPage() in PHP doesn't mean one actually exists. In fact, checking the manual (http://www.php.net/quickref.php ...you did know that there exists an online manual, right?) verifies that there's no built-in getPage() function in the language. What does that mean? It means that you'll need to create the getPage() function yourself. And that means doing more than merely trying to tweak the syntax used to invoke the function. Start there.
-
You're not actually calling removeElement() anywhere.
-
That's some inefficient code for marking fields as required. I'm not even sure how it would work, as it doesn't check to see if the inputs have a value within them.... :-\ The biggest thing that jumps out at me is the second line in the validForm() function. It should be: var allTags = document.getElementsByTagName("*"); That is, getElementsByTagName("*"). You're also missing an ending curly brace for all of your functions. In JavaScript, functions look like: function functionName([argument 1, argument 2, ...]) { // function body } Try it like that and see if it works.
-
You're right, what do you recommend though? Should I just place the data outside the class then use the "require_once" function when it's needed? Well, it depends on the data and how you're going to use it. For a lot of basic config data, storing it in a separate location (like, say an XML file, or a file you can directly require_once()) and reading it into the system is the way to go. This promotes flexibility and modularity as you don't have to change your class code when migrating environments, only the environmental data. Just be sure to store it in a secure place (outside the web root) and thoroughly scrub the data before attempting to access the db or any other critical component of your system.
-
Correct. Typically constructors are marked as private (and, in some cases, both private and final) when objects aren't supposed to be directly instantiated. The Singleton (anti?) pattern relies on this. Factories are also a common place to see this, as they're most often interacted with via static methods that return the correct child object, and are often Singletons themselves.
-
A couple things: 1. You don't need to exit, then re-enter PHP between statements. You can also get rid of one of your conditionals, simplifying the code. The following is better style: <?php writeLog(); if (!$context['user']['is_logged'] || $context['user']['is_guest']) { redirectexit('action=login'); } ?> 2. This kind of thing is better done with sessions, as they naturally persist from page to page.
-
If you're hard wiring your data within a class (not a good idea in most cases), then you don't need an explicitly defined constructor at all. Simply assign the data to the data members directly: class config { private $_username = 'somelogin'; private $_password = 'thepassword'; private $_listid = '202'; //etc. }
-
Moreover, like your error states, there is no such function as validate_numeric() in PHP. For simple things like this, looking at the manual (http://www.php.net/quickref.php) is the best place to start.
-
Well, it's not a closing tag that I had to fix, but no end to the opening tag... <textarea name="enquiry" cols="39" rows="4" class="<?=isset($class)? $class : ''?> /* <-- right here */ Whats Up?</textarea>
-
To explain further, the ++ operator is the increment operator. It increases the value stored in the variable by 1. The += operator is shorthand. Example: $total = $total + $value; // is the same as $total += $value;
-
You're kind of shooting yourself in the foot with the way you're trying to go about it. For one, your function has some issues (a counter defined within a function won't persist, add_input is not a variable, etc), and you're trying to do too much inside of it. Let's make it simple: <html> <head> <title>Adding a form input</title> </head> <body> <table id="add_episodes" class="editor" border="0" cellspacing="0" cellpadding="5"> <tr> <td><input size="3" type="text" name="number" /></td> <td><input type="text" name="title" /></td> <td><input type="button" value="Add another text input"></td> </tr> <tr> <td></td> <td></td> <td> <input name="entry_id" type="hidden" value="<?php echo $id; ?>"> <input class="input submit" type="submit" id="addInput" name="submit" value="Add <?php echo $type; ?>"> </td> </tr> </table> </body> <script type="text/javascript"> var addInput = document.getElementById('addInput'); var count = 1; var limit = 50; addInput.onclick = function(){ if (count == limit){ alert("You have reached the limit of " + limit + " inputs"); return false; } else{ addToForm('add_episodes', 'number'); addToForm('add_episodes', 'title'); ++count; return true; } } function addToForm(divName, type){ // function body goes here } </script> </html> I left the addToForm function body blank because you'll need to rework it a bit to get it to work (you need to figure out exactly what element to append to, as I can't see an add_input element anywhere). This should give you the basic idea, though. Notice that I've added an id of "addInput" to the submit button, and that there's no onclick call within the element itself. I prefer an unobtrusive style of coding, and I find it makes it easier to read and maintain code when it's written like this. YMMV.
-
It looks like the following for me in FF 3.5 and IE8: Firefox - http://www.nightslyr.com/Firefox%20textarea.jpg IE8 - http://www.nightslyr.com/IE8%20textarea.jpg The test code: <html> <head> <title>Blah</title> <script type="text/javascript" src="js/jquery-1.3.2.min.js"> <script type="text/javascript"> $(document).ready(function(){ $('#contact textarea).each(function(){ $(this).addClass('js'); }); }); </script> <style> #contact textarea { display: block; width: 324px; border: 1px solid #c3c3c3; background-color: #c3c3c3; height: 87px; color: #f8f8f8; font-size: 1.2em; padding: 8px 11px 8px !important; } #contact textarea.js { font-size: 2.2em; } </style> </head> <body> <div id="contact"> <textarea name="enquiry" cols="39" rows="4">Whats Up?</textarea> </div> </body> </html> I didn't notice any text jumping around. I did see that your textarea was missing a closing '>' on the opening tag, however. EDIT: removed H-scroll
-
First, Java and JavaScript are two completely different things. Other than their names, they are not related at all. In fact, they were created by two separate companies. Second: http://www.w3schools.com
-
1. What exactly are you trying to do? 2. What isn't working? 3. Why aren't you using CSS for image rollovers?
-
Something like this (tested and working): <?php abstract class Output { protected $words; public function Display() { if (isset($this->words) && is_array($this->words)) { foreach($this->words as $item) { echo "$item, "; } } } } class Greetings extends Output { public function __construct() { $this->words = array("Hi", "Hello", "Hola", "Bonjour"); } } class Goodbyes extends Output { public function __construct() { $this->words = array("C'ya", "Bye", "Until next time", "Au revoir"); } } $greetings = new Greetings(); $goodbyes = new Goodbyes(); $greetings->Display(); echo "<br /><br />"; $goodbyes->Display(); ?>
-
Ah, good point.
-
Could you try something like: abstract class Master { protected $fileTypes; public function setFile($file) { if (isset($fileTypes)) { // compare the file against the array } } } class Child extends Master { public function __construct() { $this->fileTypes = array ( /* allowed file types for this class */ ); } } The parent class declares the $fileType member, but what it contains is dependent on the child classes, and set in their constructors.
-
I, for one, welcome our new beetle overlords.
-
Data members cannot be abstract. Only methods and classes themselves. Why are you trying so hard to keep this method in the parent? It seems like it's child-specific, from what you've shown so far.
-
Yeah, classes don't quite work like that. You need to keep in mind scope - a child has all of a parent's public and/or protected attributes, but a parent has none of the child's attributes. Therefore, the parent class can't access what's defined in the child class (in your case, the array). The simplest solution is to put that method definition in the child class.
-
Well, the OP only wants to know if the cursor left the fixed div and entered a dynamic one, right? Why not simply have a top-level 'counter' that contains some sort of flag that can be checked to see if the last element visited was the fixed div? So long as the dynamic divs aren't residing inside the fixed div, I would think it would work. Something like (this is a really rough sketch): var fixed = document.getElementById('fixed'); var lastVisited = ""; fixed.onmouseover = function(){ //other stuff lastVisited = "fixed"; //or: this.id } //creating new div var newDiv = createElement('div'); //yadda yadda newDiv.onmouseover = function(){ if (lastVisited == "fixed"){ //do special stuff } else{ //do generic stuff } lastVisited = "dynamic"; //or: this.id, if you give the dynamic divs an id } I could be missing something, but that seems to be the simplest way to do it, assuming, again, that the dynamic divs are not going to reside in the fixed div.
-
I'm pretty sure the other data members aren't being set properly. Look at this test code: abstract class TestParent { private $first = "hi"; private $second = "hello"; private $third = "hola"; private $fourth = "bonjour"; protected function setFirst($first) { $this->first = $first; } } class TestChild extends TestParent { public function testData() { $this->setFirst("yo, wassup"); echo "{$this->first}, {$this->second}, {$this->third}, and {$this->fourth}"; } } $test = new TestChild(); $test->testData(); Its output is ", , , and " It cannot get a hold of the parent's data members because they're private. Even the protected method doesn't work because the data member it modifies is private. Changing them all to protected generates the correct output of "yo, wassup, hello, hola, and bonjour" Try it yourself.
-
Your data members need to be protected as well....
-
Show more code. It could be a scope issue.
-
It depends on what you mean by 'completely separate.' In many cases, it will make sense for PHP to write the HTML document as output. This often includes incorporating JavaScript library code and writing JavaScript code pertaining to that particular page in script tags. Your best bet would be to use a MVC design pattern where you can separate request processing and all of the business a site is required to do from displaying the views of the site itself (the visible stuff that the end user interacts with). The views would most likely be HTML templates in a PHP file so they could grab and display the results from the request processing. So, look up MVC. And, if you're comfortable with OOP, front controller.