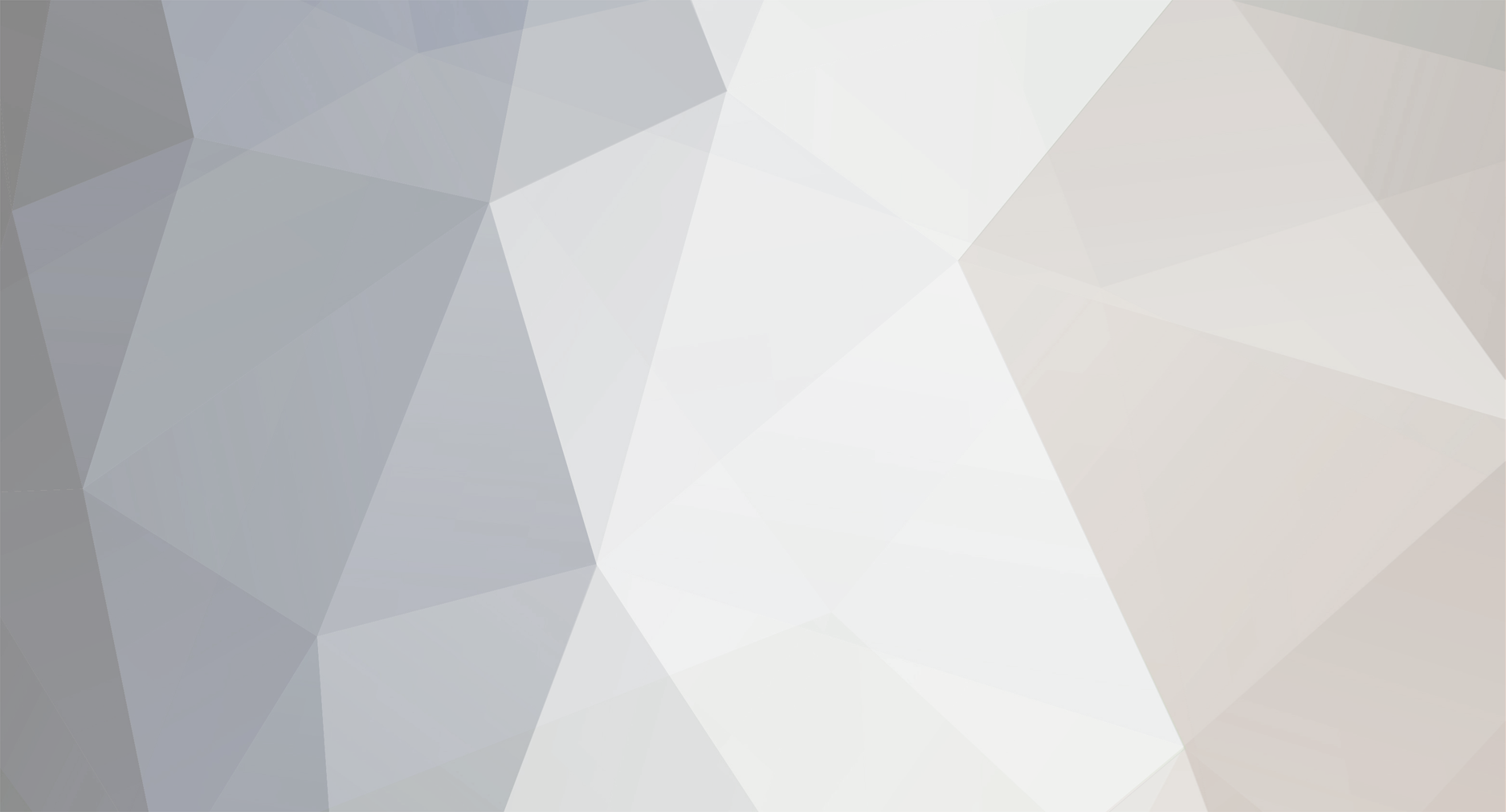
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
I firmly believe that the reason why people hate JavaScript is that they've never learned the proper way to write it. The vast majority of tutorials I've seen are, in a word, putrid. A lot of them rely on tricks from the dark ages of Netscape Navigator and IE 4. Most fail to caution against simple mistakes (problems with scope, runtime errors, etc). I cringe when I see code that has the JavaScript portion hidden in HTML comments, as it's a sure sign that the coder's headaches stem from reading a dated tutorial. And, to be fair, I used to be in the same boat until I read Resig's book on the subject. Now that I know how to structure my scripts, things run a hell of a lot more smoothly. JavaScript is actually pretty nice if you stick to the good parts, use it only to enhance existing functionality, and use a framework like jQuery to smooth over any cross-browser issues.
-
Arrays are arrays are arrays. They behave pretty much the same across languages. All that's different is the syntax. What you're looking for isn't a multidimensional array, but an associative array/hash. Something like: var ids = new Array(); function setId(key, value){ ids[key] = value; } function getId(key){ if (ids[key]){ return ids[key]; } else{ alert("There is no ID available for " + key); } } var name = "Test"; var id = 1; setId(name, id); var result = getId(name);
-
I'm not really a fan of an object having dynamic data members. It can be hard to write a public interface for, and can lead to confusion over what an object should contain. Read this message for an idea on how to implement validation via OOP: http://www.phpfreaks.com/forums/index.php/topic,188111.msg845281.html#msg845281 I also suggest reading through that entire thread, as the overall discussion is pretty informative.
-
http://www.amazon.com/PHP-Objects-Patterns-Practice-Second/dp/1590599098/ref=sr_1_1?ie=UTF8&s=books&qid=1251579261&sr=8-1
-
function setErrorHandler($oldErrorHandler = null) { if($oldErrorHandler) { //use the old info //set with create_function $customErrorHandler = create_function("$errno, $errstr, $errfile, $errline, $errcontext", /* function code */); } else { //do the same thing, only without utilizing the old handler (because it doesn't exist) } $newErrorHandler = set_error_handler($customErrorHandler); return $newErrorHandler; } $errorHandler = setErrorHandler($oldErrorHandler); You use create_function to create two separate error handlers - one that relies on the old error handler, one that doesn't. You then set whichever one is used as the system's new error handler and return it.
-
I've never seen a good use for globals. Functions have argument lists for a reason.
-
array_search() searches an array's values. The first instance of the value '1' is the 5th element of the array, whose value is "1PM Finish watering the garden."
-
Reading the documentation on array_search() may come in handy. It explains why you're getting your results.
-
[SOLVED] Object help please - foreach not working.
KevinM1 replied to spires's topic in PHP Coding Help
get_object_vars will return null values if the attribute of the object is declared but not defined. Since you're creating a new version of the container object, but not passing any values to its constructor, those attributes of $object will be null, assuming you didn't assign default values to them in the class definition. -
[SOLVED] need advice on learning php please , php is my dream goal
KevinM1 replied to silverglade's topic in Miscellaneous
Also, keep in mind that failure is a part of being a programmer. Even experienced programmers make mistakes. That's why the debugging process is so important - things fall through the cracks. The key is to not get discouraged (easier said than done, I know), and realize that failing is merely an indication that you need to learn more, or need to remember to address certain things while coding. Even the pros don't always get it right the first time. If you can afford a book on the subject, I recommend PHP For The World Wide Web. -
I recommend not attempting to learn by trying to port procedural code. It won't teach you anything other than syntax. There's a lot more to OOP than stuffing functions within a class and moving on. Get yourself a good book or two. Matt Zandstra's PHP 5: Objects, Patterns, and Practice is the best book on the subject as it pertains to PHP. Then there's the Gang of Four's Design Patterns book, which Zandstra's book borrows heavily from. Be prepared to reread those books many times. OOP is not typically something a person can grasp in one sitting. An Amazon.com search should yield you those titles. In addition, practice what you've learned once you get your feet wet. There's nothing quite like hands-on experience with creating your own objects and playing with their possible combinations.
-
Have you tried simply: $num = 8; echo "0" . $num; ?
-
Okay, let's start slow with the code. Try the following code, just to get started: class InputValidator { private $errors = array(); public function __construct(){} public function getErrors(){ return $this->errors; } public function setError($error){ $this->errors[] = $error; } public function printErrors() { foreach($this->errors as $error) { echo $error . "<br />"; } } } $validator = new InputValidator(); $validator->setError("test"); $validator->printErrors(); Let me know if the word 'test' is output.
-
Show your new code.
-
Your basic OOP code is off. If you're using PHP 5 (which, really, you should if you want to use PHP) then there are a couple of things that immediately jump out. 1. The use of 'var' when declaring data members. You should use one of the access keywords - public, protected, or private - instead. In most cases, you'll want to use private to force client code to use your accessor methods (like get/setError()) in order to manipulate object data. 2. Your constructor is wrong. It's supposed to be: public function __construct(/* argument list */) { // constructor code } So, that's two underscores, and the function name 'construct', not 'constructor'. Start with those fixes, and try again.
-
Oh, I see the problem. Your assumption is correct - the 'this' in addDate is referencing the link, not your custom object. Why cant you just pass a reference of the object to the function? Something like: dayObject.prototype.addDate = function(objRef){ alert(objRef.counter); } //in init(): link.onclick = addDate(this);
-
Can you show your event handler code?
-
Rough sketch of what MrAdam described: <script type="text/javascript"> window.onload = function(){ var oTable = document.getElementById('purchaseOrders'); oTable.style.display = 'none'; var oToggle = document.getElementById('toggle'); oToggle.onclick = function(){ oTable.style.display = (oTable.style.display = 'none') ? 'block' : 'none'; } } </script> <!-- elsewhere in your HTML --> <table id="purchaseOrders"> <--! data --> </table> <button id="toggle">Click to show/hide purchase orders</button> That's more or less the skeleton of what you need to do.
-
At the very least, hopefully you learned to never do work without an agreement in writing.
-
In OOP, you don't typically access a data member directly. Publicly accessible members break encapsulation, which is one of the main points of OOP. You need an accessor method (function). Does this calender have a getTitle function, or something similar?
-
There's no closing bracket on your else.
-
Huh, that IS strange. Can I see the code for your function?
-
Make sure that your libraries aren't conflicting with one another. Check their documentation for no-conflict functions.
-
Is it stopping at 2002? Or something else?
-
Are you employing any validation here? Another component in stopping injection attacks is to ensure that the fields contain valid data. A name shouldn't contain an integer, for example.