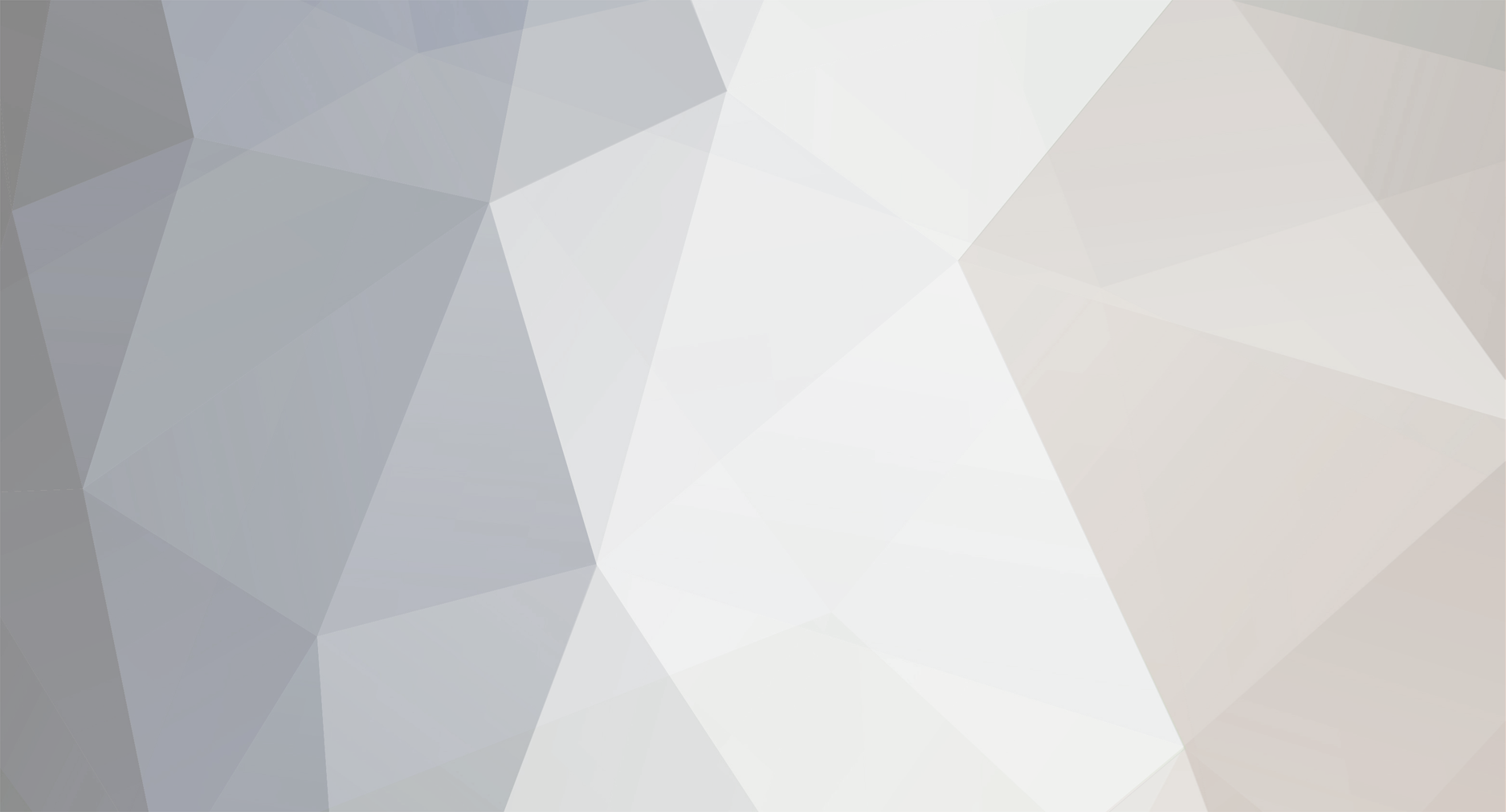
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
6 inches. And that's diameter. Oh, wait, this is the e-peen thread. My mistake.
-
How would that even work?
-
only error i get is in Internet Exploror, lmao..
KevinM1 replied to Monkuar's topic in Javascript Help
Without being able to see more of your code, I have two suggestions: 1. Use brackets with your conditionals. 2. Break up the string you're trying to assign to the innerHTML into smaller strings. JavaScript occasionally has issues with long strings. You should also check to see if itm actually exists in IE. It could be that you're attempting to manipulate the innerHTML of a null object. -
OOP PHP: Where to put a "variable assigning" function
KevinM1 replied to mtorbin's topic in PHP Coding Help
OK, so I'm going through and making the suggested changes. In reference to your protected/private/public comment, we're talking about variables, right? In other words, all of my variables that are not in the abstract class should be declared private, yes? - MT Pretty much. Like I said before, those that are in parent class(es...whether or not they're abstract) should be protected. This will ensure that inheritance works and they're passed down to their children. The rest should be private. In all cases, public accessor functions (get/set functions) should be used to modify or retrieve their values. -
OOP PHP: Where to put a "variable assigning" function
KevinM1 replied to mtorbin's topic in PHP Coding Help
The following line: $tempOBJ = new Object(); Isn't doing what you think it is. PHP isn't like JavaScript - you can't create a generic object, then populate it. PHP interprets this line as you attempting to create a new object of type Object. It's throwing an error because no such data type exists, as there is no class named 'Object' in your system. Also, your system is becoming a bit circular with the way you have it now. You don't need to create a temporary object that's only going to be discarded after the function ends. It's simpler to stuff these results in an array. Finally, with abstract classes, you can have data members. So, you can have something like: abstract class TranslateXML { protected $eventResults; public function setEventVars($obj, $count) { $this->eventResults['myGameID'] = $obj[$count]->getGameID(); $this->eventResults['myHomeTeamName'] = $obj[$count]->getTeamName('home'); $this->eventResults['myHomeTeamCity'] = $obj[$count]->getTeamCity('home'); $this->eventResults['myVisitTeamName'] = $obj[$count]->getTeamName('visit'); $this->eventResults['myVisitTeamCity'] = $obj[$count]->getTeamCity('visit'); $this->eventResults['myGameDate'] = $obj[$count]->getGameDate(); $this->eventResults['myGameTime'] = $obj[$count]->getGameTime(); $this->eventResults['myVenueID'] = $obj[$count]->getVenueID(); $this->eventResults['myPerfID'] = $obj[$count]->getPerfID(); $this->eventResults['myGenre'] = $obj[$count]->getPcomGenre(); return $this->eventResults; } } You should be able to access the data just as easly. This brings me to encapsulation. I've noticed that you like to keep data members public. This is actually a bad idea, as this means they can be overwritten/modified/deleted at any time. This, in turn, pretty much negates the entire point of doing OOP, as objects are supposed to be used like black boxes...their internals shouldn't be exposed for them to be able to work. The general rule of thumb is to give data members only as much access to the public world that they need to function, and nothing more. This generally means that data members declared in the parent classes are protected, and everything else is private. Public get/set functions are then used to modify those protected/private values. It's a bit of a pain in the butt, but a necessary one, especially if you write code that could be used by other sources within your project. It's better to be safe than wonder why values are mysteriously changing. -
You've sorta got it right. When using a query string (the part after the '?' in the URL), the name/value pair that's passed in is used as a command to the site itself. In this case, the 'page' property that's being sent into the script has a value of 'About'. There are a few ways to implement it, but the idea is the same across the board - the main script (probably index.php) intercepts that name/value pair, parses it, and then fetches the correct data based on the value. It could be something as simple as: $page = $_GET['page']; switch($page) { case 'Home': require_once("home.php"); break; case 'Gallery': require_once("gallery.php"); break; case 'Contact': require_once("contact.php"); break; case 'About': require_once("about.php"); break; default: require_once("default.php"); } Or it could be a relatively complex OOP interaction, where the values passed into the script from the query string are encapsulated as objects, are parsed by other objects, and fetch a multitude of other objects back as the necessary page components. I suggest looking up 'front controller' and perhaps 'page controller'.
-
It probably depends on where that 3rd arm sprouts. I mean, if it came out of your ass crack...well, you'd have one funky armpit.
-
OOP PHP: Where to put a "variable assigning" function
KevinM1 replied to mtorbin's topic in PHP Coding Help
You could have TranslateXML be the parent class. Nothing wrong with that. -
OOP PHP: Where to put a "variable assigning" function
KevinM1 replied to mtorbin's topic in PHP Coding Help
Hmm... I think the best way to tackle it is this: 1. Create an abstract base class that will contain all of the shared functionality (both data members and functions) that the rest of the classes need. Abstract classes are special classes that cannot be instantiated themselves, and are used just for this kind of thing. They're easy to create: abstract class BaseXML { } But, again, doing something like: $x = new BaseXML(); Won't work, and will generate an error. 2. Put all of that shared stuff I mentioned in step 1 in the class. This works just like creating a normal, non-abstract class. So, all the data members that are used in every other class should go there, and the setEventVars function should be there. 3. Create your child classes. You should have five of them - the generalized TranslateXML class (which we'll use in exactly the same way as before), and the specialized, sport-specific classes. What goes into these classes are all the things that aren't shared. So, a rough sketch should look like: abstract class BaseXML { /* data members that all other classes use - make them PROTECTED */ public function setEventVars(/* argument list */) { /* function body */ } } class TranslateXML extends BaseXML { private $myXML; public function __construct($type, $url) { switch($type) { /* same switch code as before */ } } } class XMLMlb extends BaseXML { public function __construct() { } } You ensure that all child classes have access to what they need by stuffing the shared functionality as high on the inheritance chain as it can go. That way, all the children automatically have those abilities, without the need for you to specify them in each class. It's only the things that vary (example: TranslateXML's $myXML data member) that need to be defined in the children. I hope this makes sense. OOP is a bit hard to get into, at least, if you want to do it right. If you can, do a google search on 'PHP OOP inheritance' - that should help explain what I'm trying to do. -
OOP PHP: Where to put a "variable assigning" function
KevinM1 replied to mtorbin's topic in PHP Coding Help
What class contains setEventVars? And, again, a function not returning a value != static function. A static function is a very specific thing...you'll know when you have one because the word 'static' will be visible before the word 'function' in its definition (see: http://us.php.net/manual/en/language.oop5.static.php). So, use $this->setEventVars(). You should also use $this->counter. It'd be greatly helpful if I could see all of your class code. It's a bit hard to follow what's going on without seeing all the properties and whatnot. But, yes, you seem to be on the right track. The key is a concept called 'polymorphism'. Your client code will be dealing with the TranslateXML object's interface (its public functions). But, under the hood, those function calls will be delegated to the specific XML object that's stored in the $myXML data member. So, you'd have something like: $xml = new TranslateXML('mlb', $url); echo $xml->getResults(); class TranslateXML { /* other data members and functions here */ public function getResults() { return $this->myXML->getResults(); } } Notice how the function call gets passed down to the more specialized object you're storing in $myXML. The client code doesn't care about the particulars of the operation, only that the right results are returned. -
OOP PHP: Where to put a "variable assigning" function
KevinM1 replied to mtorbin's topic in PHP Coding Help
That's an interesting suggestion. I'm still very much learning OOP and the proper way to write things (thanks, BTW, to the great suggestions made at this forum). I always like to write things to make my life easier in the long run, though it was my understanding that if you can boil functions/classes down to their most simplistic format, it's better to have a bunch of things that each do one thing rather then a few things that each do many things. Is this the right way of looking at this? - MT Yup, more or less. While an object can be complex in the way it performs its job, it shouldn't be overloaded with many jobs. It should do one or two things well, and that's it. Regarding aggriv8d's suggestion, your constructor has what's sometimes called a 'bad code smell.' Your gigantic switch statement is a sign that you need to refactor your class. What you could do is subclass based on the sport you want. Something like: class TranslateXML { private $specificXML; public function __construct($type, $url) { switch ($type) { case 'mlb': $this->specificXML = new TranslateMLB($url); break; case 'nba': $this->specificXML = new TranslateNBA($url); break; case 'nfl': $this->specificXML = new TranslateNFL($url); break; case 'nhl': $this->specificXML = new TranslateNHL($url); break; } } } class TranslateMLB { public __constructor($url) { /* build your XML */ } } No...a static function has the keyword 'static' in front of it. A function without a return value is still just a function. -
OOP PHP: Where to put a "variable assigning" function
KevinM1 replied to mtorbin's topic in PHP Coding Help
So, in reference to my script this line: $this->setEventVars($sportSchedules,$counter); should really be this line: self::setEventVars($sportSchedules,$counter); yes? - MT Only if setEventVars is a static function. -
OOP PHP: Where to put a "variable assigning" function
KevinM1 replied to mtorbin's topic in PHP Coding Help
'This' is used when dealing with individual objects. Example: class Person { private $name; private $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function getName() { return $this->name; } } $bob = new Person('Bob', 23); $sally = new Person('Sally', 45); echo "Names: " . $bob->getName . ", " . $sally->getName(); $this->name's actual value varies from object to object. Static members are class-wide members. They don't belong to individual objects, but to the entire class. Example: class StaticExample { private static $num = 0; public function increment() { self::$num++; } public function getNum() { return self::$num; } } $example = new StaticExample(); $example->increment(); $example->increment(); echo $example->getNum(); // 2 More info: http://us.php.net/manual/en/language.oop5.static.php -
You should know what page id's are legit. Simply test the incoming id against the allowable ones. If they don't match, deny access.
-
Will someone ready to an amount for getting support ?
KevinM1 replied to starphp's topic in Miscellaneous
I seriously doubt that anyone would pay you in advance to be a webmaster. You could look at your local non-profit organizations. They tend to want to have some sort of web presence, and are willing to pay something, even if it's not the going rate for more professional gigs. I had a cushy gig for a while being the webmaster for a local historical/cultural center. I rebuilt their site (it was just a handful of static HTML pages), and would update it from time to time. They paid surprisingly well, given how little work I actually did. Then again, the women who ran the place were mostly psychotic, so I figured the extra money was for my pain and suffering in dealing with them. -
OOP PHP: Where to put a "variable assigning" function
KevinM1 replied to mtorbin's topic in PHP Coding Help
When accessing static functions that are part of the class itself, you should use the 'self' keyword. As in: self::functionName($arg1, $arg2) -
You're echoing out four distinct values...are they all screwed up, or just one of them?
-
Only with AJAX.
-
How do you want them to work?
-
Are you sure? I see a preload function but I do not see the function being called. Have you done a test clearing the cache of your browser while testing? Also, this (among other things): for(i=0; i<=3; i++) { imageObj.src=images[i]; } Isn't doing what you think it's doing.
-
Your fetch function likely only fetches one row at a time. You'll need to call that function 10 times to get 10 different rows of data.
-
Ah, thanks.
-
I've always wondered about the cost of running ASP.NET driven sites. Microsoft has a free IDE/database server combo - Visual Web Developer 2008 Express - which has everything a developer needs to actually write the code. So, I'm assuming the costs lie on the server. Anyone know how much IIS servers cost to run, especially compared to Apache?
-
It could be done that way. I think, though, it'd be better to structure your validation based on the function of what the incoming data is supposed to be, rather than merely it's datatype. Example: an e-mail address is a string, but it must conform to other rules as well (such as requiring an '@' symbol somewhere). Here's an example of one solution to OOP validation: http://www.phpfreaks.com/forums/index.php/topic,188111.msg845281.html#msg845281
-
Scaling methodologies for a high traffic app?
KevinM1 replied to extrovertive's topic in Application Design
To quote the recently departed Ed McMahon, you are correct, sir.