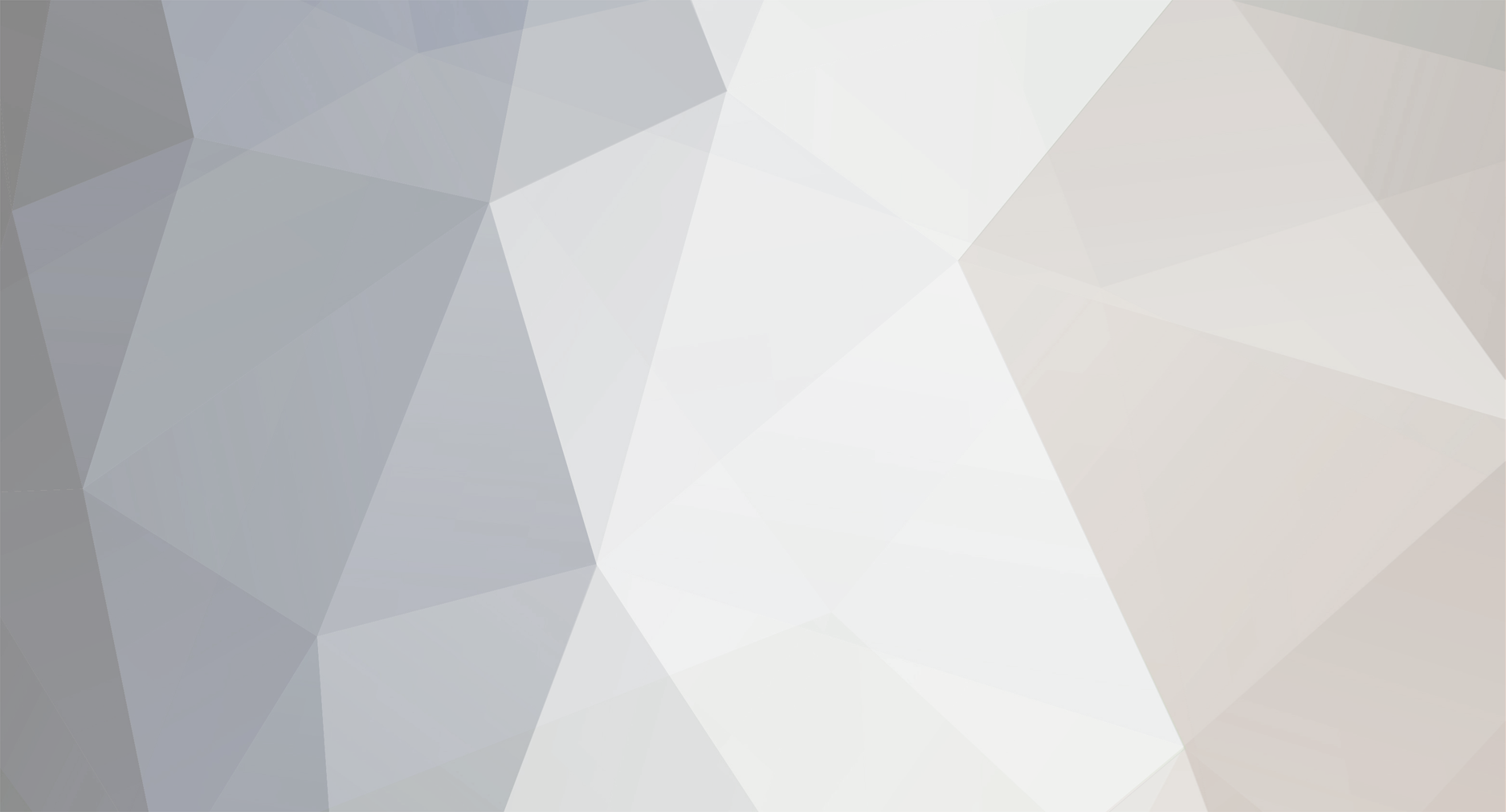
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
It probably will. It's a bit messy, if you're doing things the way I think you're doing them, but it'll save you time. Just be sure to die() after each error is reached. That way you won't have cascading errors on the screen (a failed query leads to another error, which leads to another, etc).
-
Well, the simplest way is to do something like: $errors = ""; if(/* some condition */) { //something good } else { //error $errors += "Error message 1<br />"; } /* ... */ if(!is_empty($errors)) //we have errors { echo $errors; } else { echo $goodOutput; } In essence, you want to build two output variables as your script runs. One for the good output - the site itself - and one for the errors. If any errors exist, then those should be output instead of the site. You don't have to store the outputs as string variables. You can use associative arrays, or objects, to keep track of everything. Output buffering could work, too, but it may get a bit messy considering there's two output streams that need to be accounted for.
-
I think you'd be better off looking at the design of your site as a whole. Well-designed PHP sites tend to save rendering output to the end, so any errors can be caught and printed to the screen if necessary, in place of the actual site output. In other words: 1. Process your data (this includes obtaining and handling form data) 2. Is there an error? Yes - Print error message No - Render site No JavaScript is needed in that kind of setup, and you won't have half a site created and output to the screen before you run into an error.
-
I'm looking for a good ASP.NET forum. So far, I haven't really found any that are decent across the board. They're either filled with people who don't know what they're talking about, or have very little activity. It's gotten to the point where I've received more quality help from the Penny Arcade forums than from the .NET group on Google. So, if anyone could recommend a good .NET forum - ideally something like this place - it'd be greatly appreciated.
-
Does your <li> have a set width? Because unless it's set, it'll just be the default width, which is slightly smaller than the width of the list's (<ul>) containing element. I doubt this attribute would have a concrete number in terms of pixels, due to the way it's generated.
-
I just don't understand why the ads are embedded within the threads themselves. From a design standpoint, it makes more sense for the ads to be at the top of the page so they're always visible during navigation. Given the amount of free space in the header, I don't see why they're not put in there, next to the PHP Freaks logo. Most of the other sites I visit have their ads there. It's a more natural/logical location, and it doesn't clutter up the content space. Like I said in the locked thread I started a couple days ago, my beef isn't with the ads themselves, but their location.
-
I understand that this place needs money in order to stay afloat, but putting ads as posts in the middle of threads is a horrible idea. I'd rather see a banner ad at the top of every page than have them clutter up the threads.
-
What is logoutCheck supposed to do?
-
Hmm...I'm not sure why it'd choke in IE. Nothing jumps out at me, although I must confess that when I have to use AJAX, I tend to rely on jQuery to do most of the heavy lifting for me. It saves on a ton of code, and just plain works.
-
First, whoever gave you that JavaScript code should be banned from coding. Using pseudo-protocols is horrible. Second, and more importantly, you're not asking for the right thing. You don't want a pop-up window. A pop-up window is literally a separate browser window created by JavaScript. No, what you want is a tooltip to appear next to the mouse. Unfortunately, creating a tooltip is harder than just writing one line of code. Generally speaking, it consists of the following steps: 1. You create a <div> with all the info/graphics/whatever you want as the tooltip itself. 2. You set that element's display attribute to "none". 3. You set the onmouseover event for every link that's supposed to show the tooltip to show the right one. The tricky part here is ensuring that the tooltip is displayed on the right place on the screen. 4. You set those links' onmouseout event to hide the tooltip, so they're not displayed when the mouse is not hovering over them.
-
but the Ids should be unique as they are set as arrays with [] ?? They're not set, though, as your markup has all the elements having an id of editsection[]. Again, you're confusing names with ids. When you do something like this: var myCheckboxes = document.forms["myForm"].elements["editsection[]"]; You're accessing the checkboxes through their names and not their id's. And, in this example, all checkboxes with the name editsection[] are stored in the variable myCheckboxes as an array. Indeed, the same thing happens with PHP, which obtains $_GET and $_POST values by their names, and not their id's (which is why giving a group of checkboxes a name as an array works). Similarly, something like: var myCheckboxes = document.getElementById('editsection[]'); Obtains only one checkbox element - the first one. Why? Because all of the boxes have the same id, so the function stops at the first match. Try something like the following to see what I'm talking about in action: <html> <head> <title>Blah</title> <script type="text/javascript"> window.onload = function() { var myCheckboxes = document.forms["myForm"].elements["editsection[]"]; for(var i = 0; i < myCheckboxes.length; i++) { alert("Checkbox id: " + myCheckboxes[i].id); alert("Current checkbox: myCheckboxes[" + i + "]"); } } </script> </head> <body> <form name="myForm" action="#" method="post"> <input type="checkbox" id="editsection[]" name="editsection[]" /> 1 <input type="checkbox" id="editsection[]" name="editsection[]" /> 2 <input type="checkbox" id="editsection[]" name="editsection[]" /> 3 <input type="checkbox" id="editsection[]" name="editsection[]" /> 4 <input type="checkbox" id="editsection[]" name="editsection[]" /> 5 </form> </body> </html>
-
The first version of the link uses what is called a pseudo-protocol to invoke the JavaScript. http:// and ftp:// are real protocols. javascript: is meant to be used within the href attribute of a link, much like those protocols. Like Dj Kat said above, if JavaScript is turned off, then any link using the pseudo-protocol will be broken. The second version uses an inline event handler. Again, like Dj Kat said, if JavaScript is disabled, the URL in the href attribute will be followed. I don't know if one is faster than the other. The second option is the best of those two choices. I prefer to not use inline event handlers, though, because it couples site structure (the HTML) to site behavior (the JavaScript). Instead, I code unobtrusively, like so: <html> <head> <title>Blah</title> <script type="text/javascript"> window.onload = function() { var myLink = document.getElementById('myLink'); myLink.onclick = someFunction; function someFunction() { alert("My id is: " + this.id); return false; //make sure the link IS NOT FOLLOWED } } </script> </head> <body> <a href="http://www.google.com" id="myLink">Clicky</a> </body> </html> Same functionality, but editing, maintaining, and debugging the JavaScript just got a whole lot easier as it's all in one centralized location, and not sprinkled throughout the HTML. The code, with very little modification (remove window.onload = function() { }), could even be placed in an external file, usable by the entire site, much like external CSS files.
-
The problem is that you're trying to use an id like a name. Remember: id's are UNIQUE. Each element with an id should have a unique id. So, try something like: HTML: <input type="checkbox" id="editsection[1]" name="editsection[]" onclick="show_amendment(1);" />1 JavaScript: function show_amendment(id) { var ele = document.getElementById('editsection[' + id + ']'); /* continue function */ }
-
This line: $user->addUser($this->username, $this->hash_password, $this->nombre, $this->apellido, $this->email, $this->userlevel); Is the source of your problem. Like the error says, you can't use '$this' outside of an object. What you should be doing is the following: class User { public function addUser($userName, $hashPassword, $nombre, $apellido, $email, $userLevel) { $this->username = mysql_real_escape_string($userName); $this->hash_password = sha1($hashPassword); $this->nombre = $nombre; $this->apellido = $apellido; $this->email = $email; $this->userlevel = $userLevel; $this->query = "INSERT INTO users VALUES ({$this->username}, {$this->hash_password}, {$this->nombre}, {$this->apellido}, {$this->email}, {$this->userlevel});"; return mysql_query($this->query, $this->connection); } } $user = new User(); $user->addUser($_POST['username'], $_POST['hash_password'], $_POST['nombre'], $_POST['apellido'], $_POST['email'], $_POST['userlevel']);
-
With ASP.NET, you do have to compile it yourself. Don't worry, though, because you can do it right through Visual Studio or Visual Web Developer 2008 Express. They also come with built-in debuggers, too.
-
Yes, you can dynamically allocate ids like that. Quick pseudocode: var contentDiv = document.getElementById('content'); for(var i = 0; i < availableRecords.length; i++) { (function() //anonymous function to make sure scope is left intact { var myDiv = document.createElement("div"); myDiv.id = "rcPhoto" + (i + 1); //fill out more stuff with myDiv contentDiv.appendChild(myDiv); })(); //close and invoke function } //end for-loop Where availableRecords is an array of records retrieved from the XML file.
-
An id is supposed to be a unique identifier. That means one and only one per page. So, it's invalid to have multiple elements with the same id. Your best bet would be to generate divs with similar id's, like rcPhoto1, rcPhoto2, etc. You should be able to count how many of these divs you need from the incoming XML data.
-
I repeat to make sure I understand Ok I picked up two books on ASP.Net for beginners, I think one of them is by Sams Should I also get a C# book? ASP.NET can do everything PHP can do. It's just another form of server-side technology. So, yes, you can connect to databases, write login scripts, create content management systems, and everything else. I would recommend getting a C# book. If you don't want to get one, then you can read the Microsoft Developer's Networks (MSDN) C# resource: http://msdn.microsoft.com/en-us/library/67ef8sbd.aspx . When reading the online resource pages, be sure that you're reading the .NET 3.5 entries. There's a little box at the top-right of each page that will tell you what version of .NET it pertains to, with links to other versions. EDIT: Just to be clear, PHP, ASP.NET, and other server-side languages (like, say, Ruby) can all do the same things. The differences lie in the way the programmer goes about creating their project. Sites written in PHP and ASP.NET can look identical, but the methods used in creating them are vastly different.
-
To expand on this, you (the OP) would want to do something like: var regex = new RegExp("/^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/", "gi"); if(!regex.test(myform.email.value)) { alert("Invalid e-mail address!"); myform.email.focus(); return false; } else { return true; }
-
Compiling it essentially turns it into a program that IIS can serve to web browsers. ASP.NET has two components: 1. ASP.NET server controls. These essentially create the markup for your site. You can use plain HTML server controls, which look and act almost identical to regular HTML, or you can use more powerful server controls that automate tasks (like creating a calendar, or populating a table with DB data) and automatically generate the correct HTML markup for you. 2. Processing code. This is either written in VB (yuck) or C#. This is essentially the PHP side of things, where you process whatever happens in your ASP.NET page and handle the results. This code can be written in-line, just like PHP (think about how you can switch between HTML and PHP within a .php file), or in a code-behind style, where all of the processing code is separated from the ASP file and put in its own VB or C# file. To combine the two components, they need to be compiled. ASP.NET is a very different animal than PHP. The server controls are nice, although using CSS with them can be painful. The processing side of things isn't too bad, but data binding can be a pain, depending on what you want to do.
-
Hmm...why are you using innerHTML to retrieve certain values?
-
Congrats and good luck!
-
If you want to learn ASP.NET, do the following things: 1. Download Visual Web Developer 2008 Express from Microsoft for free. It comes with a built-in server and the Express version of their SQL Server, so you'll be able to compile your projects out of the box. 2. Use C# as your code-behind language and NOT VB. 3. Get yourself a decent book on ASP.NET and, more importantly, C#. You can use the MSDN sites as a reference, but they're not that great IMO.
-
Yes, there is a better way to do it. Try: <html> <head> <title>blah</title> <script type="text/javascript"> window.onload = function() { var toggle = document.getElementById('toggle'); var myImage = document.getElementById('blockTR'); toggle.onmouseover = function() { myImage.src = 'img/category_music.jpg'; } toggle.onmouseout = function() { myImage.src = 'img/category_music_bw.jpg'; } } </script> </head> <body> <div class="block"> <h2><a id="toggle" href="#">Music</a></h2> <img id="blockTR" src="img/category_music_bw.jpg" class="blockHd" alt="music" /> </div> </body> </html>
-
I don't know of any tutorials that combine all of the behaviors you want, but I'm pretty sure it's possible. Your best bet may be to combine jQuery's effects (easiest are show and hide: http://docs.jquery.com/Effects) with JavaScript's own setTimeout function. Something like (this is pseudo-code): On page load, show the box: $(document).ready(function() { $("#myBoxId").show(); Set the time out: var timeoutHandle = setTimeout("$('#myBoxId').hide()", 5000); //hide after 5 seconds });