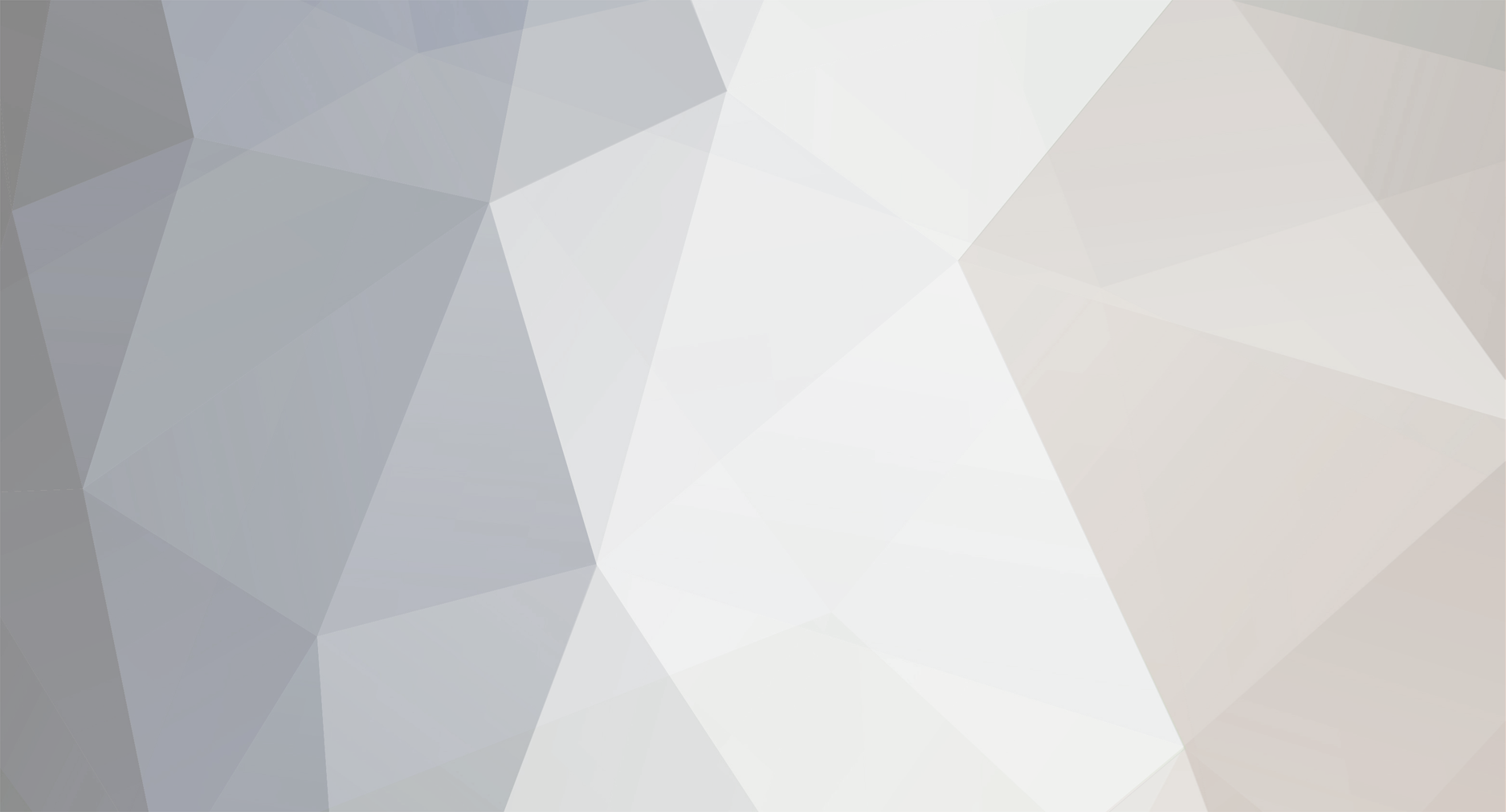
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Yeah, JavaScript has just enough minute differences in syntax from PHP to make a person want to rip their hair out at times. I haven't had the time to read through all of the code you wrote a few posts ago, but the best way to get a hold of a group of checkboxes that you're passing to PHP as an array in JS is to do the following: var myBoxes = document.forms["myForm"].elements["boxGroup1[]"]; That will assign all the checkboxes with the name boxGroup1 as an array in the myBoxes variable (see: http://www.javascripttoolbox.com/bestpractices/#squarebracket).
-
It looks like you're rushing your code without really thinking about what you're adding. So, let's take a step back and look at this objectively. You have two password fields. You need to send each of them to the underlying PHP script. The first step is to obtain the value from each field: var pass = document.getElementById('password'); var passCheck = document.getElementById('password1'); Okay, now, you need to send them along: if(pass.value.length > 0 && passCheck.value.length > 0) { var fullUrl = url + 'do=check_password_exists&password=' + encodeURIComponent(pass.value) + '&password1=' + encodeURIComponent(passCheck.value); /* continue like normal from here */ } That should be all you need to change. Just remember to think about each component individually. In your first attempt, you were only sending one password to the PHP script. In your second attempt, you were sending the value from the first password field twice.
-
jQuery is a JavaScript framework designed and written primarily by John Resig. It's primary purpose is to allow JavaScript coders to get quick results without having to reinvent the wheel for every project. If you don't have a clue about JavaScript, then you should probably try to get the basics down before attempting to incorporate animation into your site. Unfortunately, most online tutorials are lacking. Therefore I recommend the following book: The JavaScript and DHTML Cookbook by Danny Goodman (http://www.amazon.com/JavaScript-DHTML-Cookbook-Danny-Goodman/dp/0596514085/ref=sr_1_1?ie=UTF8&s=books&qid=1227044793&sr=1-1). It's a decent introduction to the language, and it's written in a results-oriented way, so you'll see code in action. It doesn't teach best practices, or professionally written JavaScript, but it's a good place to start.
-
In your constructor, it should be: $this->common = new buildCommonTrans(); Also, you should set your data members as private, just to ensure that they aren't directly accessed.
-
You could do it easily if you incorporated jQuery. Something like: <html> <head> <title>Scroll test</title> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.2.6/jquery.min.js"></script> <script type="text/javascript"> $(document).ready(function() { $("#tab").mouseover(function() { $("#myTable).show("slow"); }); $("#tab").mouseout(function() { $("#myTable).hide("slow"); }); }); </script> </head> <body> <table id="myTable"> <!-- fill out your table here --> </table> <div id="tab"> <!-- fill out your tab here --> </div> </body> </html>
-
Sorry, I wrote that in a rush. I believe it should be: var formElems = document.forms['categoryRegister'].elements; That should assign all of the categoryRegister's fields to the formElems variable as an array. Then you can just iterate through all of them with the for-loop I wrote earlier. Keep in mind, my solution doesn't keep track of which checkbox was checked (if any). It merely stores all checked values in an array for you to use elsewhere. If you need to keep track of which checkbox(es) were accessed, you'll need to create an associative array, something along the lines of: for(var i = 0; i < checkboxRange; i++) { if(formElems[i].type == "checkbox" && formElems[i].checked) { results[formElems[i].name] = formElems[i].value; } } Then you could output it like so: for(var key in results) { alert("Element " + key + " checked. Its value is: " + results[key]); }
-
Hmm...you don't name your forms or form elements? It's kind of strange reading a bunch of form references with empty strings as their names. In any event, since multiple checkboxes can be checked, you'll need to come up with an array to store the info. Something like: var checkboxRange = document.forms[''].elements[''].length; var formElems = document.forms[''].elements['']; //save typing and make code more efficient and readable var results = new Array(); for(var i = 0; i < checkboxRange; i++) { if(formElems[i].type == "checkbox" && formElems[i].checked) { results.push(formElems[i].value); } }
-
[SOLVED] Retrieving values from Associative arrays?
KevinM1 replied to idire's topic in PHP Coding Help
You can do something along these lines: for($i = 0; $i < count($result); i++) { echo $result[$i]['username']; echo $result[$i]['password']; } -
How to pass the form to a javascript function?
KevinM1 replied to ballhogjoni's topic in Javascript Help
Try: <script type="text/javascript"> window.onload = function() { var form1 = document.forms["form1"]; var splitLink = document.getElementById("splitLink"); splitLink.onclick = function() { splitDate(form1); } function splitDate(form) { var checkin_dates = form.checkin.value.split("/"); } } </script> . . . <form name="form 1" action=""> <input>... <a href="#" id="splitLink">test</a> </form> One thing I noticed, as well, is that you're not doing anything with your checkin_dates variable. You assign something to it, but that value is gone once the function is out of scope. You should declare that variable in the global space (where I declared my two variables) if you actually want to retain checkin_dates. -
PHP Code security exploit (PHP injuction) How do I fix this?
KevinM1 replied to drfate's topic in PHP Coding Help
1. This has nothing to do with object oriented programming (OOP). 2. Code tags are your friends. 3. Your problem stems from not securing your incoming query strings. At all. A simple way to fix your problem is to create a whitelist of possible page values that actually represent the pages of your site, assuming your site is small (say fewer than 20 pages). Then you can compare the incoming value with your list of legit pages. $allowedPages = array("home", "news", "contact", "faqs"); //example values...replace with your actual page names $page = strtolower($_GET['page']); //make sure the incoming value is all lowercase if(!in_array($page, $allowedPages)) { echo "I don't think so...."; } else { include $page . '.' . $type; } -
Strip_tags is good, but why aren't you validating incoming data? I mean, an age shouldn't have the possible value of "My cat's breath smells like cat food." It should simply be an integer.
-
Hey, it's all good. jQuery and Prototype are a bit different from one another. I haven't played with Prototype myself, but a quick scan of its API makes it seem like both a decent framework and a way to integrate inheritance-driven OOP into JavaScript. jQuery is primarily focused on being a simple (but powerful) lightweight framework. It's not much more than that. That said, it's still immensely powerful. A quick example of jQuery in action. Let's say you have an element that, when clicked, you want to toggle between two colors, and the original state. So, the element would go from blue, to red, to normal, and back again, depending on the click. In 'normal' JavaScript, you'd have to write something like: <script type="text/javascript"> window.onload = function() { var toggledElement = document.getElementById('toggle'); toggledElement.onclick = function() { if(this.style.color == "black") { this.style.color = "blue"; } else if(this.style.color == "blue") { this.style.color = "red"; } else { this.style.color = "black"; } } } </script> In jQuery, it'd be: <script type="text/javascript"> $(document).ready(function() { $("#toggle").toggle( function(){ $(this).css({"color : blue"}); }, function(){ $(this).css({"color : red"}); }, function(){ $(this).css({"color : "}); } ); //end toggle function }); //end ready function </script> jQuery really comes into its own when you start playing with AJAX. It's really nice to simply write something like: $.post("backendScript.php", {name : "Bubba", age : "23"}); Without having to worry about creating xmlhttp objects, or keeping tabs of the ready state.
-
I can't help but think that this is directed at me. ;-) I learn in a step-by-step manner myself. I've found, when looking back on the years of struggle I've had in trying to truly grasp JavaScript, that the vast majority of tutorials were severely lacking. That's why I never recommend w3schools - they teach only enough to fulfil certain simple problems, and never anything about how to be a better coder. While there may not be a right and a wrong way to code, there is a professional way and an unprofessional way. I firmly believe that teaching best practices, even to new coders, is the way to go. Keep in mind that these best practices don't exist in a vacuum. There's a lot of logic behind them. Unobtrusive JavaScript is easier to debug, easier to update, and can be placed in external files. This, in turn allows an entire site's behavior to be stored in a central location, much like CSS files. The markup is then separated from the script, making it easier to maintain as well. A change in one does not necessitate a change in the other. Also, placing referenced elements in variables improves readablity and execution speed. It's cheaper for the script to call a variable repeatedly than to continually traverse the DOM with document.forms["myForm"].elements... statements. In addition, I daresay that my solution to the problem is a hell of a lot easier to read than the tutorial's code. And, honestly, your AJAX comment is a non sequitur. When the technology behind AJAX was developed, we were living in the dark ages. It was the age of frequent script errors, user ignorance, and embedded MIDI files. Many users were distrustful of JavaScript, and turned it off. Those that weren't as savvy tried to avoid JS enhanced sites where they could. AJAX, rightfully, wasn't viewed as a practical solution at that time. To the OP, I recommend two books: The first is The JavaScript and DHTML Cookbook by Danny Goodman. It'll teach you the basics of the language, but not best practices. Some of his examples are lacking, but it's still a decent introduction to the language. The second is Pro JavaScript Techniques by John Resig, the guy who created the jQuery framework. This book, quite simply, changed my JavaScript life. I consider it the most important JavaScript resource ever written. It's a pretty easy read if you understand the basics of the language, and I guarantee it'll change the way you see JavaScript as a whole. Once you're comfortable with the language, you should check out jQuery itself. I truly believe that if you want to become a talented, professional JavaScript developer this is the way to go. My $0.02.
-
To be honest, I think that you should start over. Online tutorials, especially JavaScript tutorials, tend to be crap. They teach you just enough to code incorrectly, causing most new JavaScript coders to hate the language. I was one of those people myself before I was shown the right way to do things. The key to good JavaScript design is to write the code to be inobtrusive. There's no need for any JavaScript to be written directly in the HTML. The whole point of JavaScript is to add dynamic behavior to sites without impacting the markup. So, in your test script, you're trying to do some simple e-mail validation, correct? Let's start with the form first: <form name="myForm" action="#" method="post"> Sender Name: <input name="sender_name" type="text" /><br /> Sender E-mail: <input name="sender_email" type="text" /><br /><br /> Recipient Name: <input name="recipient_name" type="text" /><br /> Recipient E-mail: <input name="recipient_email" type="text" /><br /><br /> Subject: <input name="subject" type="text" /><br /> Message: <textarea name="message"></textarea><br /> <input name="submit" type="submit" value="Submit" /> </form> Nothing fancy, but this more or less is the same as what you originally had. Now, it's time for the JavaScript. The first, and perhaps most important, thing to do is to ensure that the JavaScript code doesn't initialize until the entire HTML document is loaded. One of the most common things that a rookie JS coder doesn't know/realize is that JavaScript tends to be loaded before the actual document is loaded. This causes a host of errors, as the script attempts to gain access to elements that don't exist. So, in order to combat that, you must wait for the document to load. Another thing to keep in mind is that you don't need to hide your JS code within HTML comments or CDATA blocks. All modern browsers recognize and can handle JavaScript. If you see code within HTML comments or CDATA blocks, run away, the tutorial is crap. So, with that said, a properly coded unobtrusive bit of JavaScript starts out as: <script type="text/javascript"> window.onload = function() { //script goes here } </script> Putting everything in an anonymous function tied to the window's onload event ensures that the code won't initialize until the document is completely loaded. Now, we go to the validation iself: <script type="text/javascript"> window.onload = function() { var myForm = document.forms["myForm"]; var senderEmail = document.forms["myForm"].elements["sender_email"]; var recipientEmail = document.forms["myForm"].elements["recipient_email"]; var subject = document.forms["myForm"].elements["subject"]; myForm.onsubmit = validateForm; function validateForm() { if(subject.value.length >= 40) { alert("E-mail subject must be shorter than 40 characters!"); return false; } else if(senderEmail.value == "" || recipientEmail.value == "" || subject.value == "") { alert("You did not fill out one or more of the required fields: Sender E-mail, Recipient E-mail, Subject"); return false; } else { return true; } } } </script> I've tested this code in both IE and Firefox, and it works. It may not be 100% what you're striving for, but as a learning exercise, it should do. Notice how the script doesn't interfere with the actual HTML document. All of the code is within the script tags. There's no function calls within the form elements themselves. Also note how assigning the form element info to variables saves on typing and makes for more readable code. This is the professional way of writing JavaScript, and is something that most tutorials don't address.
-
Ah but that's the thing: you can do that. If there's 100 people that show up to vote whether gay marriages are allowed or not, and the majority vote no, then guess what, the law will say no, gay marriages are not allowed. It is possible that every single person who voted no could have voted no because they flipped a coin, but it's also possible that they all voted no because they feel it's morally wrong, because the bible says so. Until, you know, the state Supreme Court gets involved.... I'm hoping that the California Court does overturn the law. I've yet to hear a logical reason as to why homosexual marriage should not be allowed.
-
Well, each function addresses a different need. To guard against SQL injection, you need to escape potential dangerous characters. Unfortunately, PHP adds a wrinkle to this with its Magic Quotes capabilities. Magic Quotes, when turned on, automatically escapes some characters. Unfortunately, it doesn't escape all of the dangerous ones, and it also causes headaches when you want to output strings to the screen. Thankfully, Magic Quotes is turned off by default in PHP 5, and won't even be available in PHP 6, but there are still many scripts that rely on it, and many PHP installations that have it turned on. So, typically the first order of business when scrubbing input is to strip the slashes that Magic Quotes automatically adds to all input: $myInput = get_magic_quotes_gpc($_REQUEST['input']) ? stripslashes($_REQUEST['input']) : $_REQUEST['input']; If you're not used to the ternary operator, that's the same as writing: if(get_magic_quotes_gpc($_REQUEST['input']) { $myInput = stripslashes($_REQUEST['input']); } else { $myInput = $_REQUEST['input']; } So, now that the damage caused by Magic Quotes is taken care of, you now need to properly escape the incoming data, which is where mysql_real_escape_string comes in. $myInput = get_magic_quotes_gpc($_REQUEST['input']) ? mysql_real_escape_string(stripslashes($_REQUEST['input'])) : mysql_real_escape_string($_REQUEST['input']); You'll still need to validate the incoming data, but most string-based attacks are no longer a grave concern. The other functions deal with exactly what you want to store. You probably don't want a user to submit pure HTML or, even worse, JavaScript to your system, so, to be safe, you can either remove all tags from incoming input (the striptags function) or transform the tags to their HTML entity counterparts (htmlentities function, which I like more than htmlspecialchars). So, like I originally said, all of these functions have a distinct use.
-
Their code is using a framework, most likely Prototype or jQuery. Look at their script tag(s)...do they include any other JavaScript files?
-
You need to use JavaScript for this. Why? Because that form's action will never be set.
-
Even English women? Would that make them English English?
-
Clients, in a word, suck. They're stubborn, have bad taste, and expect loads of hard work to be cheap. Around here, clients come in two basic flavors: The lazy. These are the clients that think a website will magically increase their sales, but don't want to collaborate with the developer on how the site should look and what the content should be. Here's an example: I was 'hired' by someone whose sister works with my mother. This man sells pub game (darts, poker, billiards) equipment. He bought pre-packaged online store software through storesonline.com. It's basically an online templating system with a database attached to it. In order to access it, he needs to pay a yearly subscription. He bought this store software because he thought it would be easy for himself to setup. He hadn't touched the software in over a year before I got involved. I asked him what he'd rather do - continue paying storesonline.com, or have me build a site from scratch. He decided to stick with storesonline.com because it meant less work for me in the long run, and he felt that the continued subscription price would be worth it if the site finally was launched. So, naturally, I asked him for certain things, the most important being his inventory information. I was having problems with Photoshop at the time, so I figured I might as well get started on the most tedious, and important, part of the project. I received nothing. A few weeks later, as I was compiling images of the items he sold, I asked him for the inventory info again, because, at the very least, I wanted to make sure I had the right image for the right product. Still nothing. This went on for months. I still haven't received any inventory info from him, and at this point, it looks like the project is dead. Thankfully, I didn't waste too much of my time on the project as, well, he couldn't give me any other info or ideas on other aspects of the site, either. He was one of those clients who thinks web developers are telepathic and can just wave a magic wand to create an incredible site. The other category I've encountered is the over-ambitious. When I was working for the local computing solutions place (which crashed and burned, but that's another story), one of our potential clients was another computing solutions place on the other side of the state. It was a small, family-owned ISP and computer repair company. It was very mom-and-pop. If I remember correctly, the company had three people working for it - the father and his two sons. So, it was small time. The oldest son, who thought he was some slick, hip business man wanted to redesign their website (which the younger son designed), so he came to us. During the meeting, he and his father agreed that we should keep the site as warm as possible. It was a small town New Hampshire company, and they didn't want to lose that local feel. Naturally, the oldest son changed his mind and wanted me to go behind his father's back. He wanted to change this: http://www.iamnow.net/ into something akin to Dell, Gateway, or PlayStation. So, I sent him a few rough prototypes, mostly to show color combinations and navigation options. Apparently his father saw these designs and was not happy. Looking at it now, the site has changed a little, but it's still largely the same as it was early last year. There are, of course, other stories, but I think my point is clear. The one thing that links these two categories of clients is that neither wants to pay for quality work. The vast majority of the potential clients I've met have bailed after telling them my going rate (which is only $30/hr). A lot of these people fall into the "My 14 year old nephew/niece/cousin can do it through Frontpage, so why should I pay you?" column. *sigh* Clients are frustrating.
-
What about the rest of the "evils"? There were 7 presidential candidates on my ballot. Holy crap! What state do you live in? From what I remember when I voted (it was a few weeks ago with an absentee ballot), there were four choices - Obama, McCain, Nader, and Barr - on the New Hampshire ballot.
-
PHP has several superglobal (read: accessible everywhere in a script) variables: $_GET, which contains data passed into the script via query string and the get method of an HTML form. $_POST, which contains data passed into the script via the post method of an HTML form. $_COOKIE, which contains data passed into the script via a cookie. There's also $_REQUEST, which is a catch-all superglobal. It quite literally holds all data passed to the script by those other methods of data input. So $_POST['someValue'] and $_REQUEST['someValue'] point to the same thing.
-
VB?? *barf* If you're going to do .NET programming, at least have the decency to use C#.
-
That's actually the biggest myth the other side tried to rally around (apart from the "Oh noes, Obama is brown, has a funny last name, and knows a guy who was a radical when he was 8 years old!" crap). The Obama tax increase only kicks in if a business (or person) makes $250,000 in profit. So, unless you make that much after all of the business expenses are calculated, there's nothing to worry about.
-
Your first line should be: var x = document.forms['formName'].elements['fieldName'].value; Notice the 's' at the end of 'forms' and 'elements'.