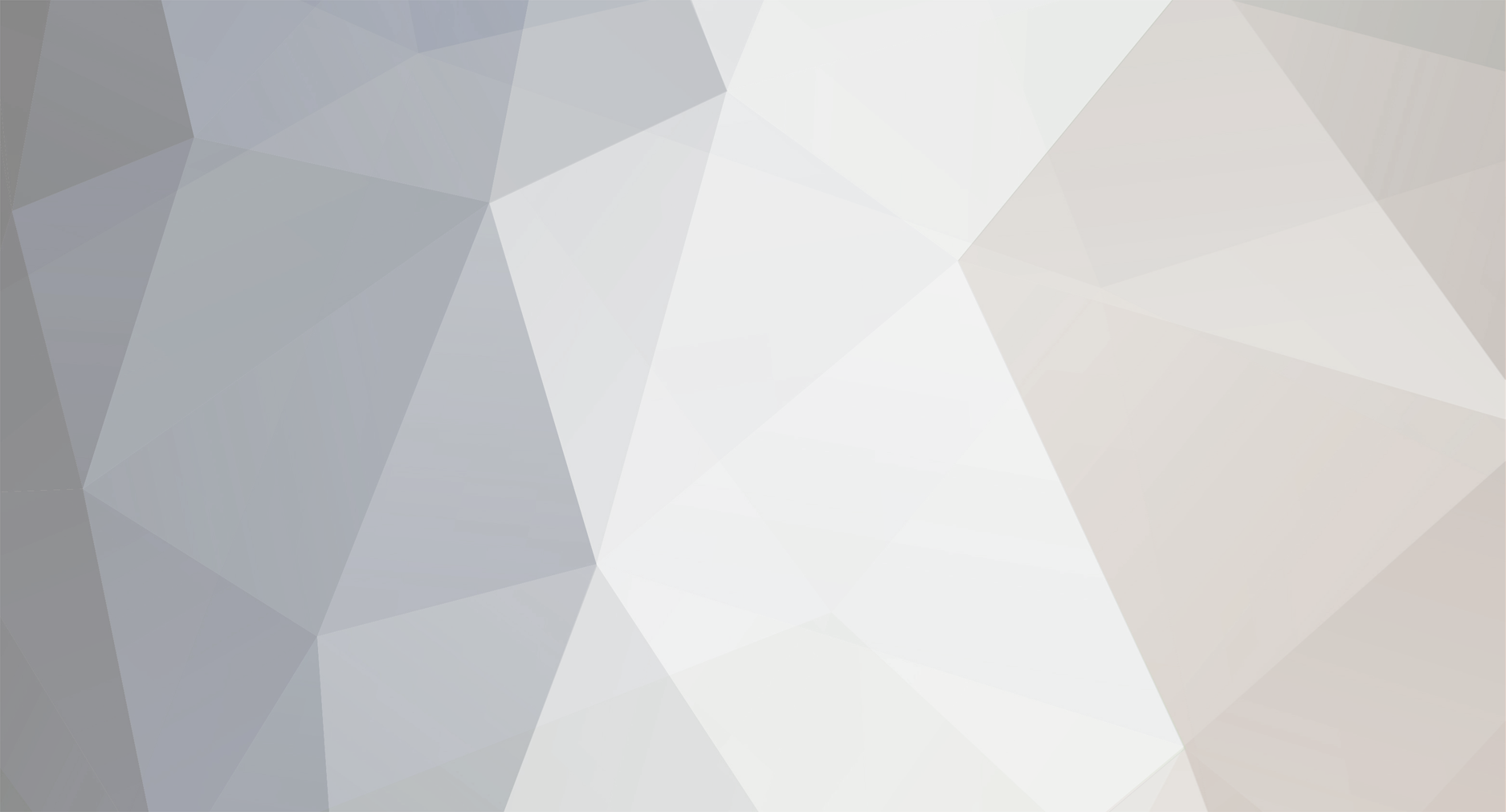
The Little Guy
-
Posts
6,675 -
Joined
-
Last visited
Posts posted by The Little Guy
-
-
I like using Putty. its small, but it is command line which is faster than a gui.
-
You could try this:
select * from table order by Column3 desc limit 2
-
you need to have quotes around the date
-
I was using no-ip like 5+ years ago which I hear is a similar service. I am currently not on my computer to test this, but doesn't that still mask the URL doing it like that?
found this:
- Go to your DNS manager and create a DNS CNAME entry for your domain (abc.com) and make it go to your free no-ip subdomain (xyz.redirectme.net)
-
I have a dynamic IP address, and port 80 blocked (So I use port 3333) and I was wondering if anyone had any solutions to use a domain with that. I would like to register a domain under godaddy. I have see some sites were it masks the urls, so if I go to:
Then click on a link that takes me to:
http://mysite.com/somepage.php
the URL then still displays:
I would like to have a way around this, does anyone know of any free solution?
-
Okay, if you use the first one, you might also want to check the mime type, which can be found from curl_getinfo as well as the http_code from above
-
you can also do it like this:
$size = getimagesize($url); if($size !== false){ // Image exists }else{ // Image doesn't exist }
-
I would use cURL
Something like this:
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_BINARYTRANSFER, true); $opt = curl_exec(); $info = (object)curl_getinfo($ch); if($info->http_code == 200){ // Image exists }else{ // Image doesn't exist }
-
This is how you can check:
<?php phpinfo(); ?>
You will probably need to restart Apache after you change the value to off.
-
I see your inserting into the database without escaping your strings. I have a feeling the rows that are failing have a quote in them and the ones that work don't have a quote in them.
This could be the problem...
-
to help you build a better table here is my suggestion:
1. Make a points table
2. have 3 fields: member_id, create_date, points
3. every time you give out points insert a value into the table
4. every time you remove points insert a value into the table (as a negative point value)
5. to get a total sum:
select sum(points) as amount from myTable where member_id = 123;
6. to get a total sum between 2 dates:
select sum(points) as amount from myTable where create_date between '2011-01-01' and '2011-02-01 23:59:59' where member_id = 123;
There is a basic way of what you could do.
-
be careful when using floats, you should round them because some floats are off by just a tiny bit.
Read (short): http://edn.embarcadero.com/article/22507
You should round your floating point values to 2 decimal points using round especially when comparing two values.
-
I would recommend using a database, but this is a non-database way (NOT TESTED!):
function checkFolder(){ $.ajax({ type: "GET", url: "/process/checkfolder.php", success: function(data){ $("#myDiv").append(data); } }); } setInterval("checkFolder()", 10000); // Run checkFolder every 10 seconds
session_start(); if(!isset($_SESSION['last_check'])) $_SESSION['last_check'] = 0; $last_check = $_SESSION['last_check']; $_SESSION['last_check'] = time(); $newest_files = array(); foreach(glob("folder/*.*") as $file){ if(filemtime($file) >= $last_check){ $newest_files[] = $file; } } echo "<div>".implode("</div><div>", $newest_files)."</div>";
-
I did the same, they both finished in about 0.0074920654296875 seconds. That was for a basic page, what about a more complex page?
-
supposedly from what I hear the second way is slower, because it has to open/close or start/stop the php parser. Also the second way looks really messy IMO.
I also think HEREDOC is nice, not sure if it has anything to do with your question but...
<?php $variable = 100; echo <<<HTMLPHP <p>"This has single and double quote's that are not escaped."</p> <p>$variable</p> HTMLPHP; ?>
-
when you look in the database do you see \n or a newline?
if you see \n, that than is your problem. don't save it in the database as \n, save it as a newline.
-
I believe your looking for this:
<input type="text" name="artist" value="<?php echo $a; ?>">
-
what it sounds like is happening is that you are over writing session data.
I would do something like this:
$_SESSION[0] = array( "ID" => 123123, "something" => "some value" ); $_SESSION[1] = array( "ID" => 123321, "something" => "some value again" );
-
Change this:
$selected_radio = $_POST['method'];
To this:
$selected_radio = isset($_POST['method'])?$_POST['method']:"";
The reason you have that error is because you have php strict mode on, and since you have not posted the form yet, "method" doesn't exist.
-
why not use str_replace?
Because that makes for bad database data.
I don't know how your inserting your values, php? phpMyAdmin? Other?
-
because it is \n not /n
also \n is a string, so that wont be converted.
-
parameters can not do anything except hold values, yours would then try to run the constructor of the class Foo, and a parameter is not allowed to do that.
-
Okay, this should handle sub-domains.
<?php $url = $_POST['url']; if(!preg_match("/^(http:\/\/|https:\/\/)/", $url)){ $url = "http://$url"; } $url_info = (object)parse_url($url); $domain = preg_replace("/^www\./","",$url_info->host); $BlockedDomains = array('nepwk.com', 'teleworm.com', 'yopmail.com', 'adf.ly'); $mesaj = "<div class=\"msg\">Your URL has been submitted!</div>"; foreach($BlockedDomains as $dom){ $dom = preg_quote($dom); if(preg_match("/$dom/", $domain)){ $mesaj = "<div class=\"error\">Your URL has been blocked by our system!</div>"; break; } } echo $mesaj; ?>
-
You can't have $foo = new Foo()
Try this:
public function __construct( $string = 'abc', $foo = null ) { if($foo == null) $foo = new Foo(); $this->string = $string; $this->foo = $foo; }
how to get 3 letters php function
in PHP Coding Help
Posted
I would use str_repeat for this: