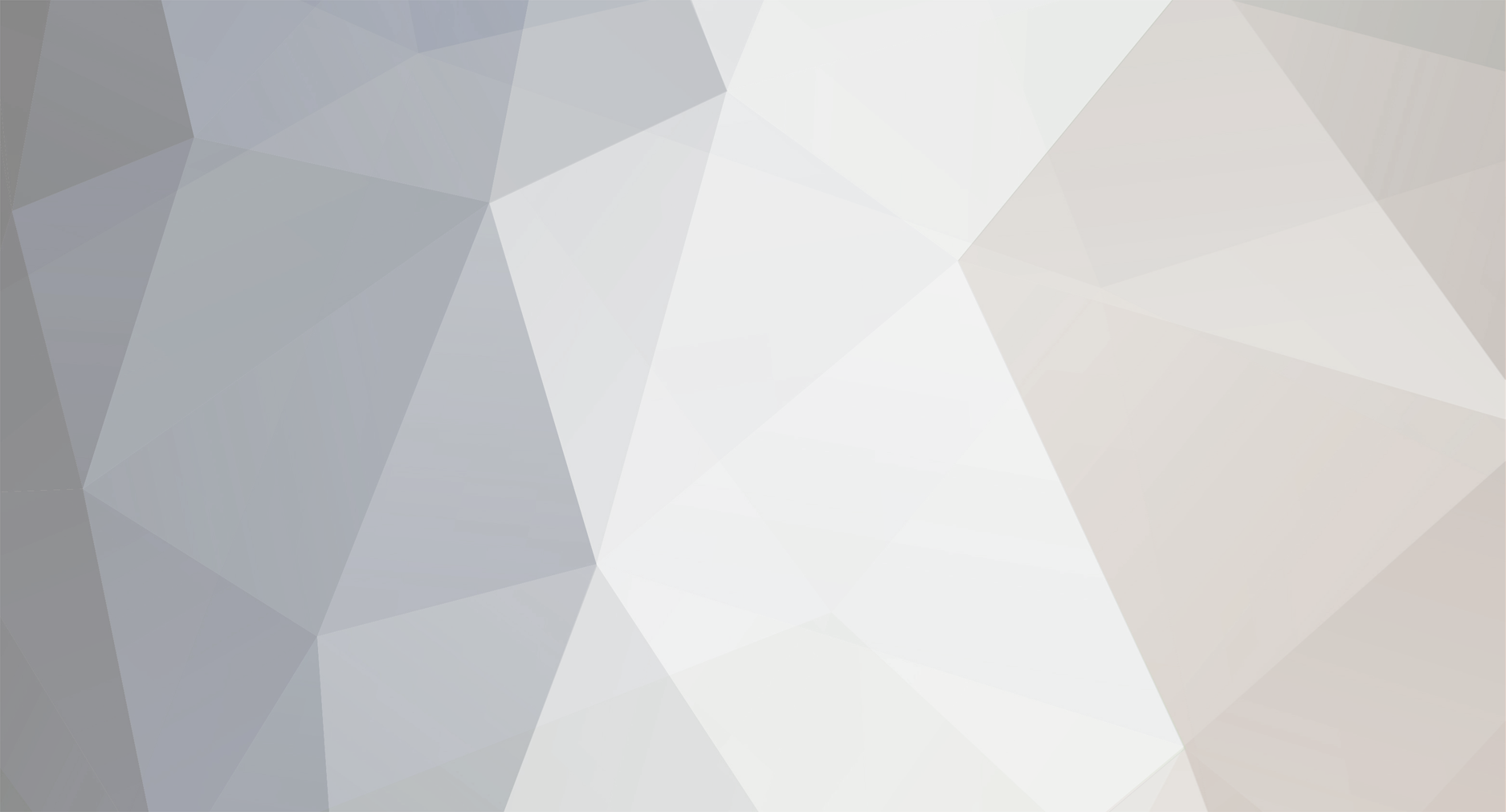
The Little Guy
-
Posts
6,675 -
Joined
-
Last visited
Posts posted by The Little Guy
-
-
you are probably missing a "}"
please don't copy and paste your whole code next time. No one wants to look through 300 lines of code to find line 178.
-
$number = 100; if($me == 0) $result = 0; else $result = $number / $me; echo $result;
-
This would work:
require_once "incl/db_connections.php"; $rows = 1000; function genCode($len = 5){ return substr(md5(rand(0,1000).time().microtime().rand(0,1000)), 0, $len); } for($i=0;$i<$rows;$i++){ $randCode = genCode(); mysql_query("insert into my_table (code) values ('$randCode')"); }
-
I am messing around with HTML5's canvas tag, I am currently building a graph, and the problem that I am having is this:
This code is within a function:
points = new Array(); points.push(10); points.push(50); points.push(10); plotGraph(points); function plotGraph(values){ ctx.strokeStyle = "rgb(0,0,0)"; ctx.lineWidth = 2; ctx.beginPath(); ctx.moveTo(0, 0); var max = values.max(); var ratio = max / ctx.canvas.height; var x = 0; for(var i in values){ var val = values[i]; var y = (ratio * val) / ctx.canvas.height; ctx.lineTo(x * 20, y); x++; } ctx.stroke(); }
What I need is for y to get calculated but in reverse (I assume). If you look at my array, 10 is a small value it should be at the bottom of the graph but it is near the top, where 50 is closer to the bottom of the graph. What would be a good way to reverse it?
-
I think that the "PHP Math Help" Board is a stupid idea, there should be a board instead that is called "Programming Math Help", because php isn't the only language that some people need math help with, there is: JavaScript, Java, ActionScript, PHP, Python, C, C#, VB, ASP.NET, Python, etc.
Making a General Programming Math Board would be really helpful, instead of just one for php.
-
Why do you have 3 tables? You only need 3 tables for a "Many" to "Many" relationship. From what I am understanding, is you have a "One" to "Many" relationship (1 post with many comments).
-
First, you want a column that is made for timestamps, so you would use a data type of "datetime".
Second, you don't want to store the value like like this "20/10/2012 23:30" in the database, you would store it like this "2012-10-20 23:30:00" (YYYY-MM-DD HH:MM:SS)
Now MySQL can easily do date/datetime functions on your dates. What you have is a valid date format, but NOT a valid MySQL date format.
-
You need an index on numericid.
And don't use DISTINCT.
Wouldn't a index on "d.tarrif " be better... from what I see he already has an index on "h.tarrif_numericid".
-
It looks/sounds like your spider/scraping script is attacking your database with either too many queries too fast or too many un-optimized queries.
Sphinx has a MySQL storage engine that you can add to MySQL this will allow you to do MySQL queries using sphinx. Just FYI.
I had a search engine that could search 150,000 rows in about 0.02 seconds, and this was using Sphinx. I know 150k rows isn't that much, but compared to MySQL Full Text, which did that in about 2-4 seconds, all using the same hardware and MySQL configuration.
If you plan on using Full Text to make a search engine, your search engine will crash and burn, don't think you will become the new Google using Full Text Searches.
-
I went here:
and it saw that the port is opened. I also tried both passive/active (just on the FTP client not the server). I don't think that the firewall is blocking it, because I can connect just fine, it is that its just not getting the directory listing.
-
If your trying to build a search engine, I would recommend looking into Sphinx:
Full Text searches on one server are extremely slow when you start to get a large database. Splitting the database up on multiple servers may speed it up though.
-
I am using Filezilla for my FTP server, and I can connect, but I get not get the directory listing.
Status: Connecting to 24.179.144.72:3334... Status: Connection established, waiting for welcome message... Response: 220-FileZilla Server version 0.9.40 beta Response: 220-written by Tim Kosse (Tim.Kosse@gmx.de) Response: 220 Please visit http://sourceforge.net/projects/filezilla/ Command: USER root Response: 331 Password required for root Command: PASS ****** Response: 230 Logged on Status: Connected Status: Retrieving directory listing... Command: PWD Response: 257 "/" is current directory. Command: TYPE I Response: 200 Type set to I Command: PORT 10,0,1,195,212,33 Response: 200 Port command successful Command: MLSD Response: 150 Opening data channel for directory list. Response: 425 Can't open data connection. Error: Failed to retrieve directory listing
I have look at many solutions, but none have yet worked for me, so does anyone know of anything I can do to get this working?
Any help is appreciated, Thanks!
-
Solved!
drop temporary table if exists ap_purch; drop temporary table if exists need_ap; create temporary table ap_purch (member_id int, product_id int); create temporary table need_ap (member_id int, product_id int, is_enabled boolean, processor_id int, pid int); insert into ap_purch (member_id, product_id) select member_id, product_id from purchases where product_id in(1,25,87,88,89,90,91,92) group by member_id, product_id; insert into need_ap (member_id, product_id, is_enabled, processor_id, pid) select a.member_id, a.product_id, 1, 9, r.product_id pid from ap_purch a left join recurring r on(a.member_id = r.member_id and a.product_id = r.product_id) having pid is null; insert into recurring (member_id, product_id, is_enabled, processor_id) select member_id, product_id, is_enabled, processor_id from need_ap;
-
I need to use this code, to insert values that don't exist in a table. The issue that I am having, is my last query where I need to do an "insert into select from" using a "having". I need all fields where pid is null, but I don't want to insert pid into the "recurring" table. I need to select the column otherwise the having won't work. The error I am getting is:
ERROR 1136 (21S01): Column count doesn't match value count at row 1
I can't think of a solution to this problem, anyone have any suggestions?
drop temporary table if exists ap_purch; create temporary table ap_purch (member_id int, product_id int); insert into ap_purch (member_id, product_id) select member_id, product_id from purchases where product_id in(1,25,87,88,89,90,91,92) group by member_id, product_id; insert into recurring (member_id, product_id, is_enabled, processor_id) select a.member_id, a.product_id, 1, 9, r.product_id pid from ap_purch a left join recurring r on(a.member_id = r.member_id and a.product_id = r.product_id) having pid is null;
The select return the results I want, it is just that the insert doesn't work.
-
What are some common standards to writing a php class?
One thing I think is a standard is using "Camel Case" (example):
public function myFunctionName
instead of using:
public function my_function_name
Is that true, and are there any others that you know about? I would like to hear what they are!
Thanks!
-
-
Looks like I got it with this code:
select m.member_id from members m join purchases p on(m.member_id = p.member_id) where level_id = 3 and is_active and m.reg_date >= unix_timestamp('2010-01-01') and ( m.member_id not in(select member_id from purchases where product_id = 14) and m.member_id not in(select member_id from purchases where product_id = 42) and m.member_id not in(select member_id from purchases where product_id = 29) and m.member_id not in(select member_id from purchases where product_id = 86) and m.member_id not in(select member_id from purchases where product_id = 43) and m.member_id not in(select member_id from purchases where product_id = 9) and m.member_id not in(select member_id from purchases where product_id = 44) and m.member_id not in(select member_id from purchases where product_id = 12) and m.member_id not in(select member_id from purchases where product_id = 45) ) group by member_id;
-
I need to get a list of all the members in a table that have not purchased these products: 14,42,29,86,43,9,44,12,45
I thought that this would work but doesn't:
select m.member_id from members m join purchases p on(m.member_id = p.member_id) where and level_id = 3 and is_active and p.product_id not in(14,42,29,86,43,9,44,12,45) group by member_id;
I can not figure this out, thanks for any help!
-
preg_replace("/\<(script).*\>.*\<\/(script)\>/isU", " ", $data);
-
Now, this class can easily be reused! Basically it is an example social network news feed. Writing the class takes the longest, but then when you want to get some members news feed, you just need to use 1-2 lines of code and POOF! a news feed!
require_once "db.php"; class Member{ private $id = null; private $name = null; private $email = null; private $db = null; private $friends = array(); public function __construct($member_id){ $member_id = (int)$member_id; $db = new dbConnect(); $db->query("select * from members where member_id = $member_id"); $this->id = $db->row->member_id; $this->name = $db->row->name; $this->email = $db->row->email; } public function getNewsFeed(){ $this->getfriends(); $friends = implode(",", array_keys($this->friends)); $db->query("select * from news_feed where poster in($friends)"); while($db->row()){ return "<p>$db->row->message</p>"; } } public function getfriends(){ if(count($this->friends) > 0) return true; $db->query("select f.member_id, concat(m.first_name, ' ', m.last_name) full_name from friends f left join members m on (f.member_id = m.member_id) where owner = $this->member_id"); while($db->row()){ $this->friends[$db->row->member_id] = $db->row->full_name; } return true; } }
then, its usage would look like this:
require_once 'classes/Member.php'; $member = new Member(12312312); echo $member->getNewsFeed();
-
I need a query that gets a list of member id who purchased products 1 and 87. I can not figure out the best way to do this. How can I do this?
Here is my table structure:
+----------------+------------------+------+-----+-------------------+-----------------------------+ | Field | Type | Null | Key | Default | Extra | +----------------+------------------+------+-----+-------------------+-----------------------------+ | recurring_id | int(11) | NO | PRI | NULL | auto_increment | | member_id | int(11) | NO | MUL | 0 | | | product_id | int(10) unsigned | YES | MUL | NULL | | | special | enum('ap') | YES | | NULL | | | is_enabled | tinyint(1) | NO | MUL | 0 | | | processor_id | int(11) | NO | MUL | 0 | | | member_card_id | int(11) | NO | | 0 | | | date_updated | timestamp | NO | | CURRENT_TIMESTAMP | on update CURRENT_TIMESTAMP | +----------------+------------------+------+-----+-------------------+-----------------------------+
I thought that this query would work, but it doesn't:
select * from recurring where product_id in (87,1) group by member_id order by member_id limit 10;
Thanks for any help!
-
I am using php's zip class, and I am able to zip files up individually, but can I zip an entire directory with its file and sub-folders?
-
tinymce can edit, but you would then have to build the CMS for the editor, so might as well just download the CMS that has its own built in editor that works with the editor.
-
if you can't do that, can you use netbeans where each function has documentation with the auto complete?
blocking certain urls in a php form
in PHP Coding Help
Posted
Try this: