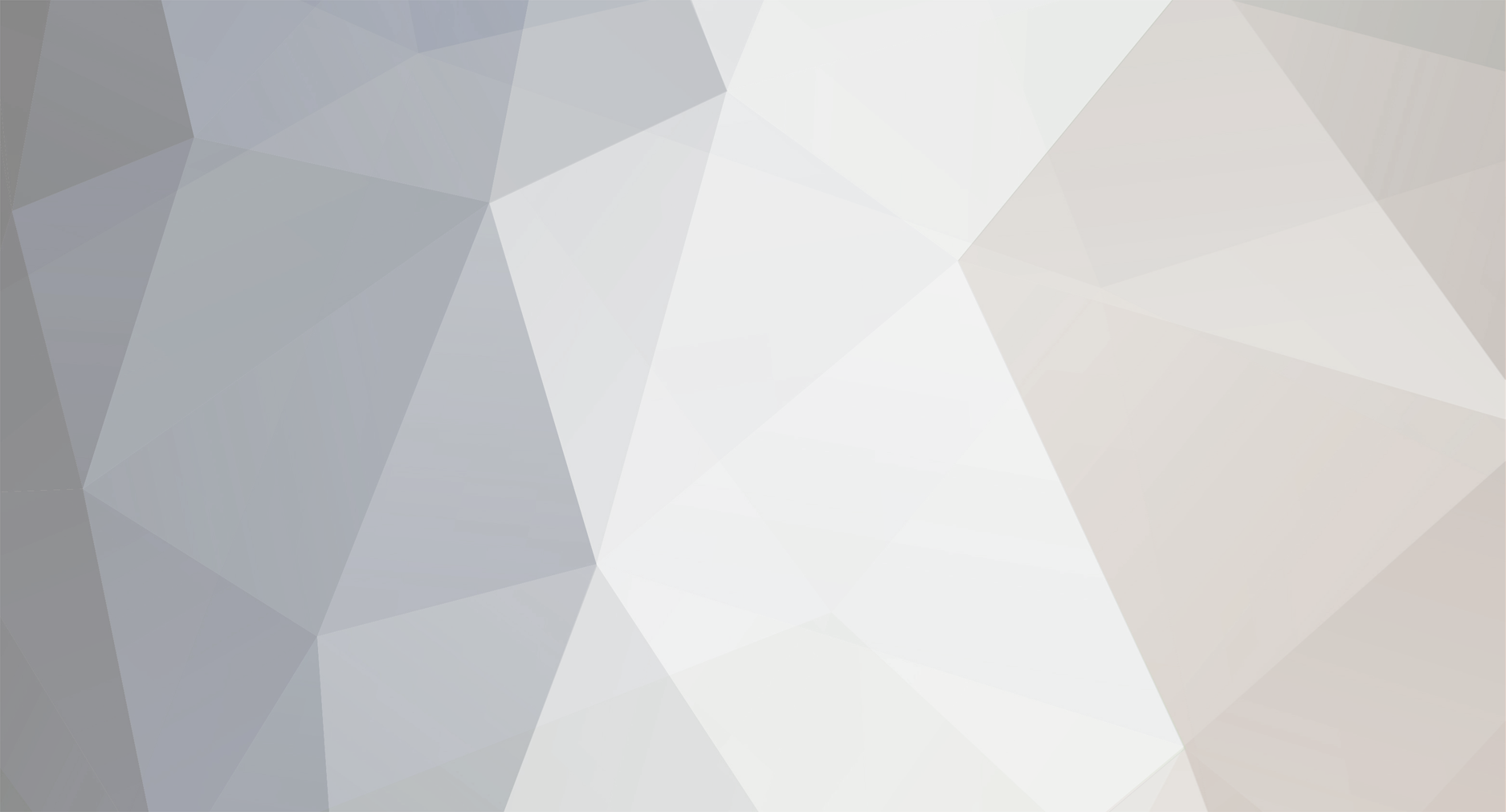
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
I forgot that you needed to escape the $ also. $stringData = "<?php // Change the 4 variables below $yourName = 'change this to your name'; $yourEmail = 'you@yourdomain.com'; $yourSubject = 'change to your subject line'; $referringPage = 'http://www.yourdomain.com/contact.php'; // No need to edit below unless you really want to. It's using a simple php mail() function. Use your own if you want function cleanPosUrl ($str) { return stripslashes($str); } if ( isset(\$_POST['sendContactEmail']) ) { $to = $yourEmail; $subject = $yourSubject.': '.\$_POST['posRegard']; $message = cleanPosUrl(\$_POST['posText']); $headers = 'From: '.cleanPosUrl(\$_POST['posName']).' <'.\$_POST['posEmail'].\">\r\n\"; $headers .= 'To: '.\$yourName.' <'.\$yourEmail.'>'.\"\r\n\"; $mailit = mail(\$to,\$subject,\$message,\$headers); if ( @\$mailit ) { header('Location: '.\$referringPage.'?success=true'); } else { header('Location: '.\$referringPage.'?error=true'); } } ?> "; Should work. Or you could just change the ' to double like so: $stringData = '<?php // Change the 4 variables below $yourName = "change this to your name"; $yourEmail = "you@yourdomain.com"; $yourSubject = "change to your subject line"; $referringPage = "http://www.yourdomain.com/contact.php"; // No need to edit below unless you really want to. It"s using a simple php mail() function. Use your own if you want function cleanPosUrl ($str) { return stripslashes($str); } if ( isset($_POST["sendContactEmail"]) ) { $to = $yourEmail; $subject = $yourSubject.": ".$_POST["posRegard"]; $message = cleanPosUrl($_POST["posText"]); $headers = "From: ".cleanPosUrl($_POST["posName"])." <".$_POST["posEmail"].\">\r\n\"; $headers .= "To: ".$yourName." <".$yourEmail.">".\"\r\n\"; $mailit = mail($to,$subject,$message,\$headers); if ( @$mailit ) { header("Location: ".$referringPage."?success=true"); } else { header("Location: ".$referringPage."?error=true"); } } ?> ';
-
<?php $writeToFile = "// Change the 4 variables below $yourName = 'change this to your name'; $yourEmail = 'you@yourdomain.com'; $yourSubject = 'change to your subject line'; $referringPage = 'http://www.yourdomain.com/contact.php'; // No need to edit below unless you really want to. It's using a simple php mail() function. Use your own if you want function cleanPosUrl ($str) { return stripslashes($str); } if ( isset($_POST['sendContactEmail']) ) { $to = $yourEmail; $subject = $yourSubject.': '.$_POST['posRegard']; $message = cleanPosUrl($_POST['posText']); $headers = 'From: '.cleanPosUrl($_POST['posName']).' <'.$_POST['posEmail'].\">\r\n\"; $headers .= 'To: '.$yourName.' <'.$yourEmail.'>'.\"\r\n\"; $mailit = mail($to,$subject,$message,$headers); if ( @$mailit ) { header('Location: '.$referringPage.'?success=true'); } else { header('Location: '.$referringPage.'?error=true'); } }"; ?> That should allow you to write to a file. You use \ to escape certain characters. So to escape the double quote its \" as seen above.
-
Your main issue is going to be the use of both single and double quotes. Stick to one. That way you can put the whole thing into a string properly without having to escape the characters. Chances are the errors are being thrown cause of " or ' single quotes. I would escape the double quotes around the \r\n since those need the double quotes. Let me know if that fixes your issue.
-
[SOLVED] How do you replace character " in array?
premiso replied to nicob's topic in PHP Coding Help
Or another variation for fyi purposes: $from = array("+",'"'); -
Than you are overriding $ext somewhere else. I would search your code for $ext and see where it is being set and where it might possibly be overwritten. I bet if you put a echo $ext; right after the declaration it will echo out the correct value.
-
Nulling variables if they don't exist - why bother?
premiso replied to psyickphuk's topic in PHP Coding Help
In most other languages you have to define the variable for you to use them. It is good practice and ensures that the variable will contain what you expect it to. Security reasons is probably the main reason, but it also helps the server out since it will not search all variables and find out that one is not there, it sees it and returns it. -
As long as you have a mysql db setup, you just have to make a connection to that DB and do not necessarily have to use that db. But if you do some googling you can find functions that "simulate" the mysql_real_escape_string in fact you may be able to find it in the comments on the page. However it simulates it, it is not it. So yea.
-
Another working example.. function writeShoppingCart() { ... return '<p>You have <a href="cart.php?fname=' . $fname . '">'.count($items).' item'.$s.' in your shopping cart</a></p>'; ... } Or even function writeShoppingCart() { ... return '<p>You have <a href="cart.php?fname={$fname}">'.count($items).' item'.$s.' in your shopping cart</a></p>'; ... } It is just a matter of preference. Me I like to go in and out so I know where my variables are when I look at my code.
-
Sounds like an ftp flaw/human error. You cannot really account for that unless you had some script that could tell when a file was manipulated to that directory and when it is it alerts the database that x has been deleted or b has been uploaded. The only way to do that, which I know of, would be to constantly parse the ftp logs, which would be a huge resource hog. I think you are stuck between a rock and a hard spot here.
-
how can I have SELECT menu populate hidden field thru javascript/php...?
premiso replied to mac007's topic in PHP Coding Help
Either you need to code the sizes into the javascript or you want to look into using AJAX. -
My 2 cents, the file ("sound/$sid.mp3") does not exist so it is not entering into that if statement. Does $file1 echo out anything? The statement that you can manually set it to "mp3" and not get a value is what made me come to that conclusion.
-
Only if $_POST['players'] is an array. The updated code works as expecte you only give it one userid. You would need an array of players id's somehow for it to work like you want it to.
-
Could be because of a syntax error: $pages = array('home', 'news', 'media', ''); // comma needed before the '' But not sure why you would have '', it would allow for a .php included which would throw an error. I believe, but yea. See what that does for ya.
-
Chances are the opportunity and where are combined which is throwing an error: $query = "select * from Opportunity" . " where oppId = 10"; // added space here $results = mysql_query( $query, $con ); To have gotten the error this would have thrown it: $results = mysql_query( $query, $con ) or die(mysql_error());
-
You are missing the close bracket to the foreach: foreach($_POST['players'] as $pz){ $sql = "SELECT usrID, usrFirst, usrLast"; $sql .= " FROM tblUsers"; $sql .= " WHERE usrID = $pz"; } require("../connection.php"); $rs=mysql_db_query($DBname,$sql,$link); if ($rs) { while ($row=mysql_fetch_array($rs)){ $first = $row['usrFirst']; $last = $row['usrLast']; $full = $first . " " . $last; print ("<div>"); print $full; print ("</div>"); } //end while } // end if } // end foreach ?> There should have been a syntax error thrown on that. but yea. Hopefully that is the issue.
-
edit: removed below and re-thinking it. <?php $arrA = array(1, 5, 10); $firstRun = true; foreachLoop($arrA, 5); function foreachLoop($array, $endAt=false) { foreach ($array as $value) { echo $value.' '; if (!$endAt) continue; // if endat is false just continue and skip below if (($value == $endAt) ) { foreachLoop($array, false); break; } } } ?> That would work, that way you give it a value and once it hits that it breaks out etc. As for which is better, this or a for loop really it is your call. But foreach doesn't have a built in function to do that as far as I know.
-
With proxies, networks etc it is nearly impossible. Like right now, my pc and my wife's pc show the same IP even though our internal IP is seperate. I can also use a proxy to hide my IP. So yea, IP just isn't reliable. I am unsure if they can set it to be anything, but adding the real_escape_string around it will protect you from SQL injection.
-
I do not think it is possible, but you could make your own function that is recursive... <?php $arrA = array(1, 5, 10); $firstRun = true; foreachTwice($arrA, true); function foreachTwice($array, $continue) { foreach ($array as $value) { echo $value.' '; if ( ($continue) && ($value == 10) ) { foreachTwice($array, false); } } } ?> That can also be manipulated to go so much further to, as it is it will only run twice.
-
Simple as this, browsers should kill sessions when closed. One possible scenario (which you can test with firefox and inspect cookies) is that your session is by chance being stored in a session and when you go back to the page it re-initializes that session. That would be a server deal, so if you have a .htaccess file look there. Every site I have worked on, the sessions reset on a browser close, so the scenario above (if possible) I am sure is rare. But yea. That is all I can think of.
-
<?php $AAA = 'a'; $BBB = 'b'; $ezPhoneRun = 0; $ezPhoneCount = 100; // this value varies. while ($ezPhoneRun < $ezPhoneCount) { $ezAPCSCCounter = 0; $ezAPCSCDRCount = 1; //I have just added this here for your sake, the real value is a varied. while ($ezAPCSCCounter < $ezAPCSCDRCount) { if ($AAA == $BBB){ // do some code stuffs, loop $ezAPCSCCounter++; echo 'here1'; continue 0; }else { $AAA = 'b'; $string = ""; } $ezAPCSCCounter++; } // END while ($ezAPCSCCounter < $ezAPCSCDRCount) { echo 'end'; $ezPhoneRun++; } // end loop for isp's phone table loop ?> I did that for test reasons, but that returned the right results. Maybe your code is flawed in some way...mind posting the actual code?
-
It happens to everyone. Just as you get more advanced the stupider the answer is...seriously. It is always the small little stupid thing that makes a script go bad. I've spent countless hours on a problem and the solution ended up being something that was soo simple I should have saw it first. But yea, it still happens to me.
-
It uses templates to form the pages I imagine. But if you really want to know phpbb, check the forms and documentation. vbulletin and phpbb use styles to "skin" and they have users code in different templates so you change the template. Each template would require the design so you would have to do a design per template. I would like to help more, but the questions are really vague and the explanations are rather in-depth. You would need to talk to a phpbb guru to learn how it works etc.
-
Not really a php issue. You are missing the end > on the <body tag. Add that and it should work.
-
Well for starters your for is weird, it starts at 1 and adds 1 to it before anything happens, thus you start at 2. //I have to start at 1. its been tested already for($i = 1; $i <= $nb_dispos; $i++) { $valide = $valide && !in_array($_POST['date' . $i], $date); $date[] = $_POST['date' . $i]; } That would be better, if you do not have a date0 and only have a date1...etc. Also this part: $valide = $valide && !in_array($_POST['date' . $i], $date); Is weird as $valide will only amount to the last date. So if the last date is in the array, than it will always return false. And if $valide, is false than it will always return false, cause $valide can never be set to true. That statement in itself is flawed. Hope that helps to get you on the right track. I would be more than happy to help more, I just need to know what your goal is for this function. What does it ultimately need to do?
-
for($i = 1; $i <= $nb_dispos; ++$i) { $valide = $valide && !in_array($_POST['date' . $i], $date); $date .= $_POST['date' . $i]; } You are setting $date to a string when it should be an array. Thus the error. $strDate = ""; for($i = 1; $i <= $nb_dispos; ++$i) { $valide = $valide && !in_array($_POST['date' . $i], $date); $strDate .= $_POST['date' . $i]; } Try that. This assumes you want it in a string, if you want it in an array, use flyhoney's version.