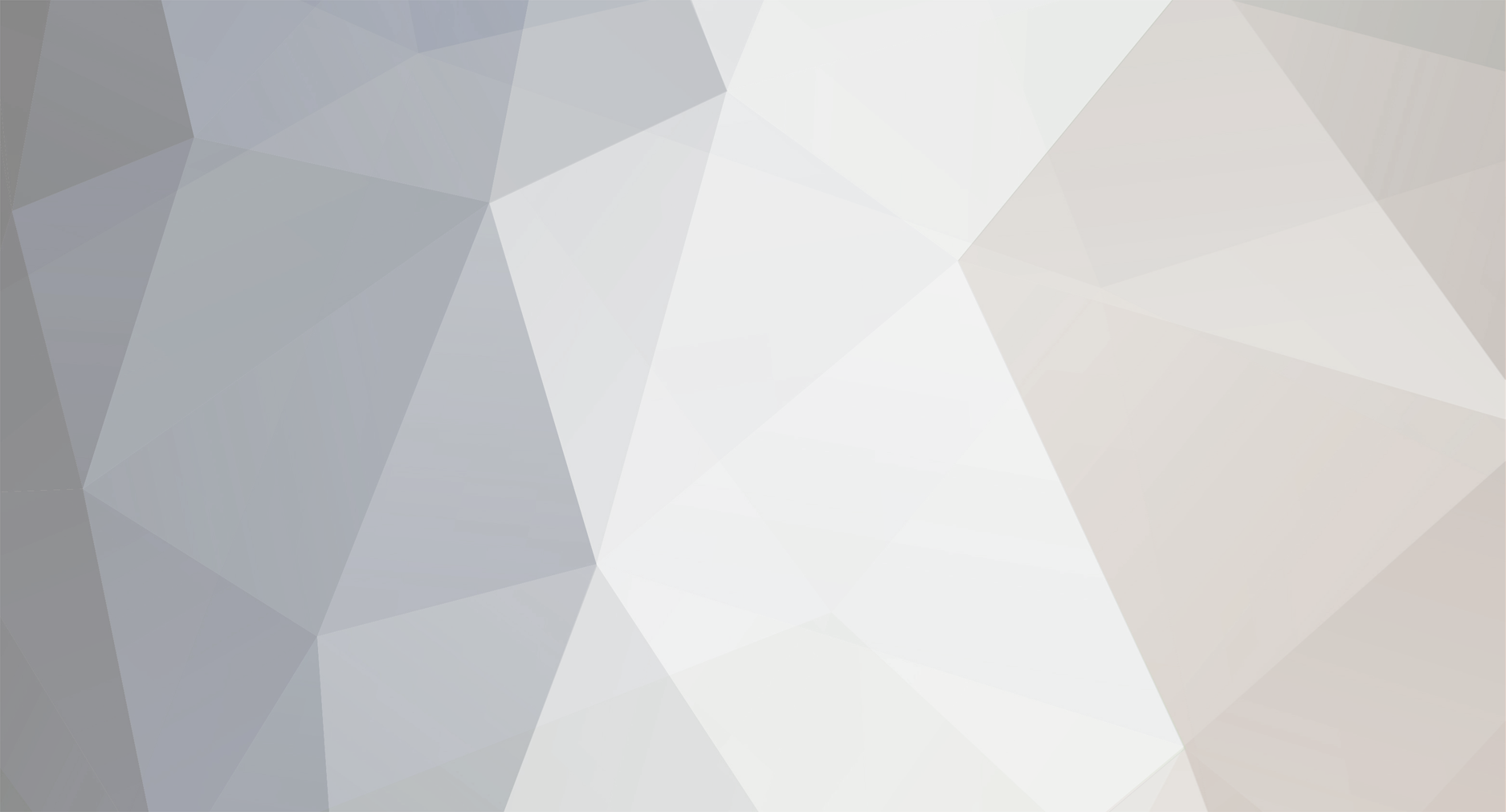
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
[SOLVED] Is somebody trying to hack my shopping cart?
premiso replied to trampolinejoe's topic in PHP Coding Help
It sounds like the cart uses JScript to validate fields and not the PHP. If someone creates their own form with the right data they can easily post an item to the database and bypass the validation. I would suggest coding the validation inside php and doing the checks there. You can use the same function with AJAX if you want dynamic non-page reload checking. Are they trying to hack, probably not. They are probably just testing a way to spam you and if it works. -
[SOLVED] How to include local page with '?' in url ??
premiso replied to nicob's topic in PHP Coding Help
<?php $_GET['keyword'] = "garden"; $_GET['submit'] = "Search"; include "/home/account/domains/domain.com/public_html/search.php"; ?> -
$type_data = $type . "1"; mysql_query("UPDATE tablename set `$type`= '$type_data' where id = '$playerid'"); or mysql_query("UPDATE tablename set `$type`= '" . $type . "1' where id = '$playerid'"); Should do the trick.
-
can someone make me a script for user registration
premiso replied to berry05's topic in PHP Coding Help
Google it, http://www.google.com/search?hl=en&q=registration+php+script&btnG=Google+Search&aq=f&oq= If you want it custom, I suggest posting in the freelance section. -
Need help - using php to check if file exists and then get it
premiso replied to DreamHack's topic in PHP Coding Help
I think you just want the file_exists function to check if it exists. To get it, depending on how you want it, file for an array or file_get_contents I would play around with that and check back if you have any problems. If you want this done for you, post in the freelance section. -
You need to use backticks (`) around column names and single quotes(') around data. mysql_query("UPDATE tablename set `$type`= ($type+1) where id = '$playerid'"); Also does $type hold a column name? (oh I see thats already been pointed out oh well.)
-
It is obvious we need to see more code. It could be in the 2 functions you call on the lines etc. post more code and your question may be solved. In that code there are no errors.
-
PHP - MySQL - Selecting multiple rows from DB
premiso replied to AlwaysDead's topic in PHP Coding Help
<?php $query = "SELECT id FROM table WHERE name = 'some name'"; // and if you wanted no more than 3 rows: // $query = "SELECT id FROM table WHERE name = 'some name' LIMIT 3"; // Perform Query $result = mysql_query($query); // Check result // This shows the actual query sent to MySQL, and the error. Useful for debugging. if (!$result) { $message = 'Invalid query: ' . mysql_error() . "\n"; $message .= 'Whole query: ' . $query; die($message); } // Use result // Attempting to print $result won't allow access to information in the resource // One of the mysql result functions must be used // See also mysql_result(), mysql_fetch_array(), mysql_fetch_row(), etc. while ($row = mysql_fetch_assoc($result)) { $ids[] = $row['id']; } //accesed by foreach ($ids as $id) { echo $id . "<br />"; } // or echo $ids[0]; // to echo the id in position 0. // Free the resources associated with the result set // This is done automatically at the end of the script mysql_free_result($result); ?> If you want a better example be more specific on what you would like to do with the id. -
It is instant, what can happen is the browser can cache a page, hold ctrl and press F5 to refresh the page to the new copy. This is generally a problem in IE, but that ctrl+F5 refresh generally does the trick.
-
Yea I figured. Let's try this route instead. $getLinks = file($this->feed_file); echo ' <form method="post" action="index.php?page=edit">'; foreach ($getLinks as $key => $value) { echo ' <input type="checkbox" name="links['.$key.']" />'.$value.'<br />'; // note change here } echo ' <input type="submit" name="sub_delete" value="Delete Links" /> </form>'; $file = file($this->feed_file); $links = ""; if (isset($_POST['links'])) { foreach ($_POST['links'] as $key => $val) { if ($val == "on") { unset($file[$key]); } } $links = implode("\n", $file); } $handle = fopen($this->feed_file, "w"); if ($handle) { fwrite($handle, $links); } fclose($handle); That should be a bit better...and work lol. EDIT: Removed the extra index part because it was unecessary. Should yield the same results.
-
Thanks for the help! $file = file($this->feed_file); $links = ''; for ($i = 0; $i < count($file); $i++) { if (isset($_POST['links'][$i]) && $_POST['links'][$i] != "on") { $links .= $file[$i] . "\n"; } } $handle = fopen($this->feed_file, "w"); if ($handle) { fwrite($handle, $links); } fclose($handle); That should work, not 100% sure. Try that and let me know if not I do know the solution.
-
Updated version: $file = file($this->feed_file); $links = ''; for ($i = 0; $i < count($file); $i++) { if ($_POST['links'][$i] != "on") { $links .= $file[$i] . "\n"; } } $handle = fopen($this->feed_file, "w"); if ($handle) { fwrite($handle, $links); } fclose($handle); added a \n to the links so it writes the new links on seperate lines like expected.
-
Part of your problem here: foreach ($getLinks as $key => $value) { echo ' <input type="checkbox" name="links[',$key,']" />',$value,'<br />'; } Is you are using commas instead of periods for concatenation. EDIT: Ok figured it out. This should work. $file = file($this->feed_file); $links = ''; for ($i = 0; $i < count($file); $i++) { if ($_POST['links'][$i] != "on") { $links .= $file[$i]; } } $handle = fopen($this->feed_file, "w"); if ($handle) { fwrite($handle, $links); } fclose($handle); Basically just re-worked the logic since a checkbox is returned as "on" the above should do the right checks and omit the right links.
-
The only way I can think of is doing a foreach loop <?php foreach ($_GET as $key => $val) { echo $key . " = " . $val . "<Br />"; } ?> You may also be able to use $_SERVER['QUERY_STRING'] echo $_SERVER['QUERY_STRING']; Than using explode you should be able to pull out the name. Or instead of explode you can use parse_str .
-
file_put_contents $file = "config.php"; file_put_contents($file, '<? $file = ' . var_export($config,true) . '; ?>'); Should fix you up.
-
[SOLVED] Escape String VS Trim VS Strip Slashes.
premiso replied to Stryves's topic in PHP Coding Help
Not true. If done right, you should never have to use stripslashes on data coming out of a database. This is why addslashes is bad, because you never really know how many times you must strip_slashes. That is why mysql_real_escape_string is the preferred method, you do not have to strip the slashes because they are removed as the content is entered into the DB. A prime example of this is when you display content on a page like so: <?php $file = "I am displaying \" double quote that has been escaped"; echo $file; ?> As you can see a properly escaped character has the escape character removed when displayed. This principle applies to a database in this same manner. It removes the escaping slashes so the data is displayed as it literally should be. The reason some people use strip_slashes is because they add extra escapes to their content, especially if get_magic_quotes_gpc is turned on and when the post data comes in they call add_slashes on that data, it will double up the slashes requireing them to be removed because an escaped slash displays that slash if that makes any sense. When you use the mysql_real_escape_String it is smart enough not to do this to escaping characters. -
[SOLVED] Escape String VS Trim VS Strip Slashes.
premiso replied to Stryves's topic in PHP Coding Help
mysql_real_escape_string is not really equivelent to strip_slashes as removing slashes could potentionally allow people to harm the database, I think you were thinking of add_slashes The rule of thumb is you should never have to use strip_slashes on data coming out of a database. Trim works well with everything and should not screw up data. As for mysql escape string being outdated that is just bologna, it still works great against SQL Injection to this day. -
Oh I thought that is how you wanted it...given the code. You would want to store each image in an array like the results, infact attached onto the results array would be ideal, then you could just use that in your smarty to display the image. How you have it currently coded, only the last image is returned because $stats is not an array and gets overwritten with each loop. Hope that helps you get moving.
-
[SOLVED] Problem extracting images from a database
premiso replied to Athgar's topic in PHP Coding Help
Have you tried turning on error_reporting and setting display_errors to 1 to make sure that any errors are being displayed? error_reporting(E_ALL); init_set("display_errors", 1); Other than that, are you sure the image type was input to the database properly? Sorry if this is all repetitive, but from the looks of the code, and your statements, everything looks good from this end. -
If you are afraid of the database being overloaded than xml should be fine, easy to manipulate/add to if necessary and easy to read. But I think that once the user has a connection to the database it will not matter running an extra 1 or 2 queries. Thats my opinion anyway. I think the database is the way to go.
-
Are you sure that 10.gif is in the right directory? Maybe try adding the site url to the beginning of each image. Like so: $img_url = "http://www.yoursite.com/path/to/image/"; $smarty->assign('results', $results); $smarty->assign('stats', $img_url . $stats); If the picture is not in the directory where the file is being called from that will not work. Unless the template has already taken account for that. Since there is no template I am throwing this up as an option.
-
<?php session_start(); // Check/set variables. if (isset($_SESSION['alert'])) { $alert = $_SESSION['alert']; }else { $alert = 0; $_SESSION['alert'] = 0; } $x=5; while ($alert < $x){ $alert++; } // should set $alert equaled to after the while loop // since we put isset up top remove it from here as the session variable should be set now. if (($alert > $_SESSION['alert']) ){ $_SESSION['alert'] = ($alert + 1); // add 1 to the alert echo "<script>alert('" . $_SESSION['alert'] . "');</script>"; } ?> Give that a try and see what comes of it.
-
The basename should do that for you. But another example would be: <?php $filename = "./files/pdf/something.pdf"; $filename = explode("/", $filename); $filename = $filename[count($filename)-1]; echo $filename; ?> As said above basename should do that without the fuss.
-
[SOLVED] Quick question regarding deletion of certain dates
premiso replied to waynew's topic in PHP Coding Help
WHERE orderDate < '2008-11-20 09:00:00' Less than the specific date for older greater than specific date for newer. I would also setup a test DB to test this out if you are afraid of wiping the wrong data, that way you know it works. -
I am not sure bud. The only other thing I can think of is you may have to give the file that is executing the chmod command the 0777 permissions via FTP for it to be able to assign the same permissions to another file. Other than that I am out of experience/ideas on and hopefully someone who really knows can help.