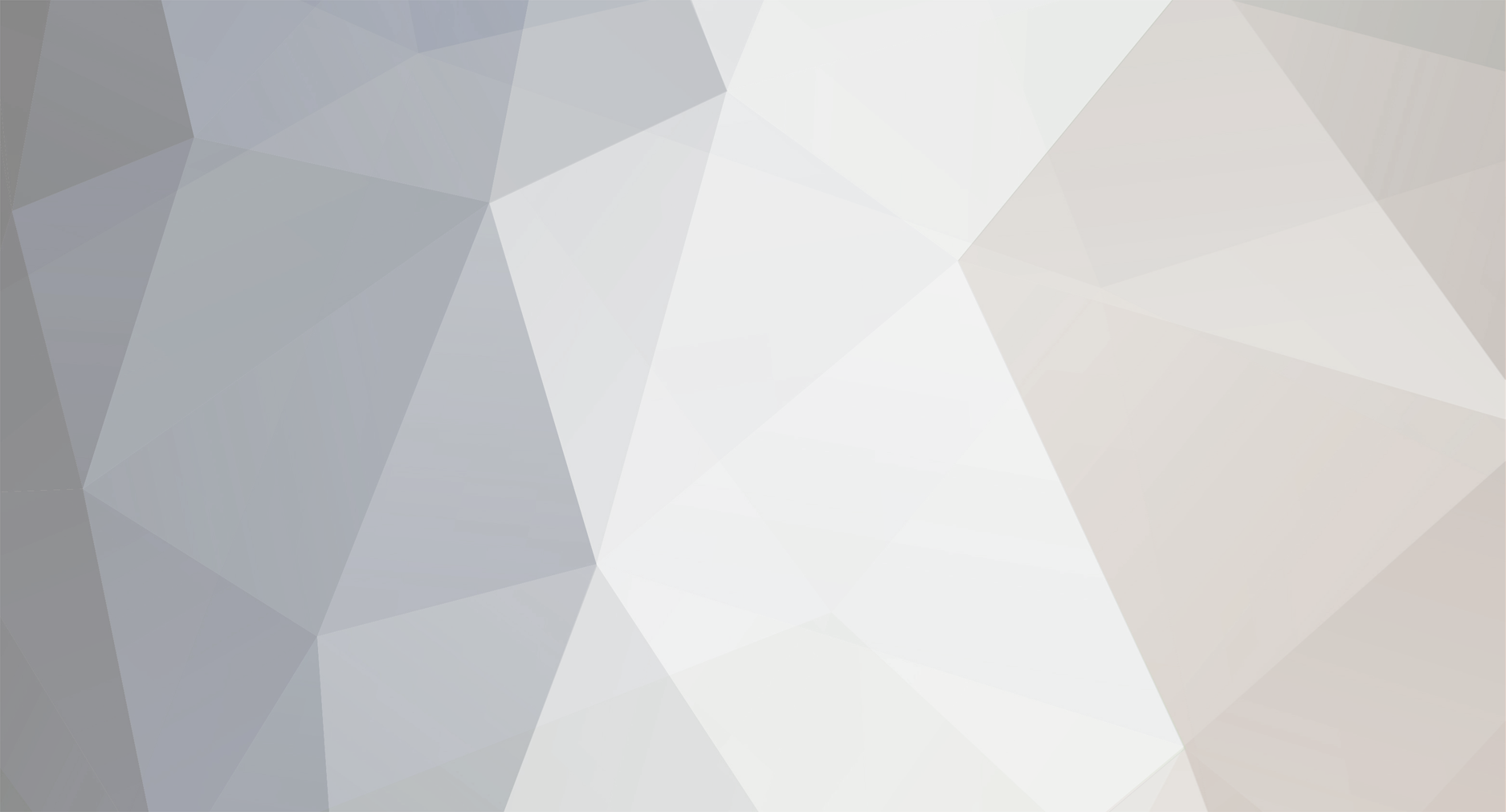
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
Depending if you post or get $_POST $_GET After you enter the info the text box you have to submit that page in order to do what you want. During the <form> tag declaration define method as get or post depending on which use the above to access what you chose.
-
Because $row is an array. <?php if (isset($_GET['q'])) { require('../vdb/includes/connection.php'); $q=$_GET["q"]; echo $q; $statement = "SELECT engine_family_name FROM eo_engine_family WHERE engine_family_name LIKE '".$q."%'"; $results = mysql_query($statement) or die(mysql_error()); while($row=mysql_fetch_assoc($results)) { $items[]=$row; } //print_r($items); foreach ($items as $value) { foreach ($value as $key => $newval) { echo "Value: $newval<br />\n"; } } } ?> Should give you what you are looking for.
-
Another way is call the file using the url. <?php $file = file_get_contents("http://www.yoursite.com/filetogetcontents.php"); echo $file; ?> Should print out "Some text" given that the above URL exists.
-
If $num is greater than one, then subtract 1 from $num then times that by 20 then subtract 2 from that. If $num is less than one set $num to one. So lets say $num is 10 10 - 1 is 9 times that by 20 is 180 then subtract 2 is 178 So the width would be set to 178 pixels. This I am assuming would make sure that the name can fit inside the div without being cut off. And if there was no name it would be set to 1 so the div wouldn't really be visible....at least that is how I interpret it.
-
mod_rewrite using .htaccess Do a google on mod_rewrite for examples and usage.
-
$file = basename($_SERVER['PHP_SELF']); basename Defaults returns only the page name.
-
How are you calling the file, are you using the fullpath? After you use the chomd check the file permissions with fileperms I also seem to remember that the directory has to be writeable for this to work too...I am unsure if that is true or not.
-
I know 0777 will give you full access, but after you are done writing to it I would reset it back to 644.
-
chmod You need to change the file permissions to allow writing. By default the permissions are I think 644, which allows reading but no writing.
-
[SOLVED] simple string func not working correctly
premiso replied to scottybwoy's topic in PHP Coding Help
<?php $products_description = "\" TESTING \""; $f_char = substr($products_description, 0, 1); $l_char = substr($products_description, -1); echo $f_char . " - f_char<br />" . $l_char . " - l_char<br />"; if (($f_char == '"') && ($l_char == '"')) { echo "cleaning description"; $len = strlen($products_description); $products_description = substr($products_description, 1, $len -2); } echo $products_description . "<Br /><Br />"; ?> Simple debugging would of found that you needed to do $len - 2 since the substr is 0 index based. -
After reading the comments it seems only the replace functions are your options...well other than using explode then implode which would be silly to do, but an example of that is: <?php $string = "123,423,234"; $int = (int) implode("", explode(",", $string)); echo $int; // should output 123423234 ?> =)
-
Looks like str_replace would be the way to go or ereg_replace (gathered this from reading the comments on int type-casting). Either will work. EDIT: Source for more information http://us.php.net/manual/en/function.intval.php#76803 Also removed number_format after reading it does not take strings for a param.
-
That is just a bad way of doing that. Not recommended at all. Here is an improved way. <?php $sql= mysql_query("INSERT INTO events(`pic`,`title`,`date`,`time`,`about`,`picip`,`publish`,`pdate`) VALUES('" . $_POST['pic'] . "','" . $_POST['title'] . "','" . $_POST['date'] . "','" . $_POST['time'] . "','" . $_POST['about'] . "','" . $picip . "','" . $_POST['publish'] . "','" . $now. "')") or die(mysql_error()); if (!mysql_query($sql,$connect)) { die('Error: ' . mysql_error()); } ?> I always like to concatenate strings just because it makes it easier to identify. And always use single quotes (') when access array elements via association, if you have any type of error reporting on it will throw and undefined constant error. Now to protect yourself from SQL injection I would recommend doing this: <?php // note you can easily do this by defining each value instead of looping // but for demonstration purposes I will loop instead of writing the 5 if statements. foreach ($_POST as $key => $val) { $_POST[$key] = mysql_real_escape_string($val); } $sql= mysql_query("INSERT INTO events(`pic`,`title`,`date`,`time`,`about`,`picip`,`publish`,`pdate`) VALUES('" . $_POST['pic'] . "','" . $_POST['title'] . "','" . $_POST['date'] . "','" . $_POST['time'] . "','" . $_POST['about'] . "','" . $picip . "','" . $_POST['publish'] . "','" . $now. "')") or die(mysql_error()); if (!mysql_query($sql,$connect)) { die('Error: ' . mysql_error()); } ?> Questions let me know. For demonstration purposes you could also do this instead of using concatenation: <?php $sql= mysql_query("INSERT INTO events(`pic`,`title`,`date`,`time`,`about`,`picip`,`publish`,`pdate`) VALUES('{$_POST['pic']}','{$_POST['title']}','{$_POST['date']}','{$_POST['time']}','{$_POST['about']}','$picip','{$_POST['publish']}','$now')") or die(mysql_error()); if (!mysql_query($sql,$connect)) { die('Error: ' . mysql_error()); } ?>
-
<?php include ('../string_link.php'); # Set error reporting and connect to database $query = 'SELECT ek, prform FROM dchords'; $result = mysql_query ($query) or die (mysql_error()); while($row = mysql_fetch_assoc ($result)) { # WORKS PERFECTLY WITH "if", BUT NOT "while" $prform=$row['prform']; $ek=$row['ek']; $v_beauty=$prform+1; print "$ek: $prform, $v_beauty. "; $query = "UPDATE dchords SET v_beauty='$v_beauty' WHERE ek='$ek' LIMIT 1 ;"; mysql_query ($query); } ?> In your update statement you need the single quotes around the data so it does not throw errors, especially if it is in a string format. Also you have a semicolon after the if statement. Above is an updated code and should work. As per the remark above, mysql_fetch_assoc will work fine, and if you are not planning on also using the index in the array is better because it does not return both assoc array and and index array which will double the amount of data coming into the script.
-
<?php include "../connectdb.php"; $query = "UPDATE staffsched SET "; $set = array(); foreach($_POST as $field => $value){ $field = mysql_real_escape_string($field); $value = mysql_real_escape_string($value); $set[] = "`{$field}` = '{$value}'"; echo "field: " . $field . "<br>"; echo "value: " . $value . "<br>"; echo "set: " . $set . "<br>"; } ?> Change the ' (single quotes) in the field name to backticks (`) for correct usage. That should correct it.
-
Echo out the $query and see what that is displaying. Is the script dieing with the mysql error? Are you initiaiting the mysql connection first with mysql_connection then selecting the database with mysql_select_database before you run mysql_query? I would echo out this line: $query .= implode(", ",$set) . " WHERE current_status = 'c'"; echo "Query is: " . $query . "<br />; mysql_query($query) or die(mysql_error()); Other than that I have no clue. Without it throwing any error messages or error messages being provided.
-
Your not using the timeset anywhere in the cookie declaration or the browser is set to clear out all cookies... setcookie suggest reading the usage there.
-
No my version is correct, yours would take implode literally and write it out. $query .= implode(", ",$set) . " WHERE blah='blah'"; mysql_query($query) or die(mysql_error());
-
need a semi-colon after this line $hour = time() + 60*60*24*1000;
-
A. You are not calling mysql_query to run the update query. B. You would put a where statement after the last update. So basically here: $query .= implode(", ",$set) . " WHERE blah=blah";
-
Can some one help me with spamming attempts?
premiso replied to nevesgodnroc's topic in PHP Coding Help
Would work, but spammers often send a fake IP or use several proxies. IPs are easy to spoof/get a new one it is absurd. Plus if you have multiple users in an office/school setting it will block them out if 3 different people from work used that form. Not a very viable option. -
mmm the most efficient is preg_replace I do believe. But if it is just as simple as replacing * with $string I think an easier/better way would be to use str_replace since it does not use REGEX it will be much easier and I would think quicker for something that easy.
-
If you do not have access to the target location I do not think this is possible. I believe it is up to that location to determine whether to display a cached version or not. I could be wrong, but I think that is how it works.
-
Most of us are not psychics. There could be thousands of reasons why it is not working. Check your code and be more specific. I would actually try and load the page that displays the captcha in my browser and see if there are any errors.
-
$LIST is an array. If you want to do an IN on it try this: $list[0] = 1; $list[1] = 7; $lists = implode("', '", $list); $result=mysql_query("select * from table where field IN('" . $lists . "')"); Should work.