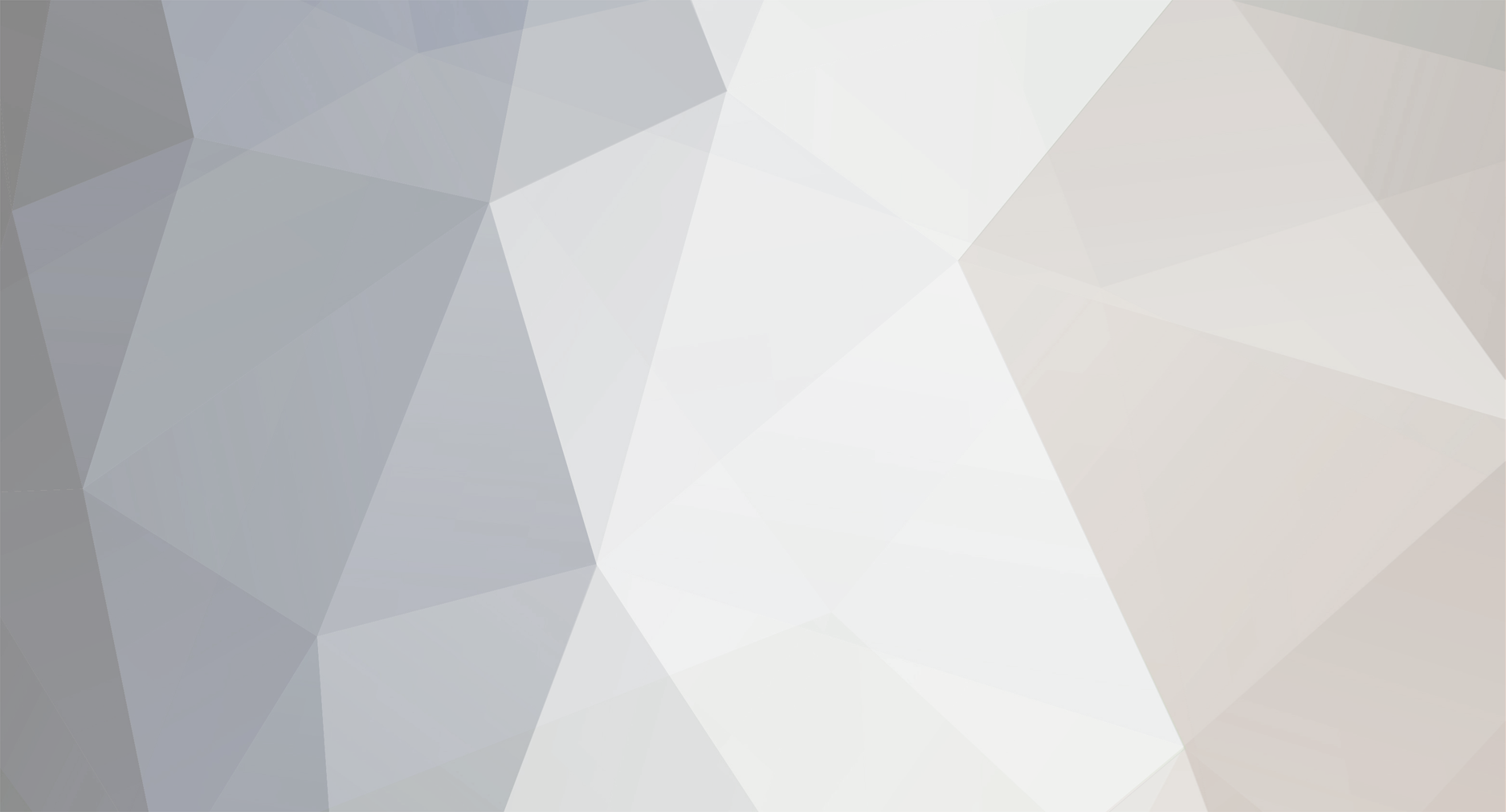
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
[SOLVED] it's not redirecting if !$_POST, any suggestions?
premiso replied to samoi's topic in PHP Coding Help
1. Yes it is the name you put in there. 2. The only error I would see is calling the header function after you have echo'd text to the screen. As I mentioned above that is not kosher. Also check that you are using "POST" variables and not "GET" in your form. As for the errors, if display_errors is set in the php.ini to on, yes that means it is on but this can be overwritten by .htaccess so it is good practice when debugging to add that to the top just in case. -
You need to define the variables outside of that switch then possible have a function and given what d is define them in that function. The function should probably be called within the constructor. But what you are trying to do is make the class have dynamic variables. I think you just need to write 2 separate classes, as I do not think that is "kosher"
-
[SOLVED] it's not redirecting if !$_POST, any suggestions?
premiso replied to samoi's topic in PHP Coding Help
If anything is echo'd to the screen the header function cannot work. I would suggest replacing this: header('Location: insert.php') or die(); with echo '<script>location.href = "insert.php"</script>'; Will give you the same result without having to re-do your current code. Although I would suggest outputting everything at the end by storing the output into a string, instead of just echo'ing it out. But yea. -
Post the code and we can help you alot faster and more efficient. It seems like the program was expecting a function, not a switch. Chances are you are missing a } somewhere.
-
select t.topic_id, t.topic_title, p.post_id from forum_posts p, forum_topics t where p.topic_id = t.topic_id order by p.post_create_time desc That should pick up the topics. You were limiting yourself by not including the post_id and using the distinct. That would return x rows depending on how many posts there are for a topic so you can show all the posts by the id. Hope that helps.
-
I would store the password in the DB as an MD5 hash. That will validate against case sensitivity and secure peoples passwords. You would have to change to when the password is inserted in the db it get's hashed using the md5 function to allow this. <?php require "config.php"; $username=$_REQUEST["uname"]; $password=md5($_REQUEST["password"]); $sql="SELECT * FROM users WHERE username='$username' and password='$password'"; $result=mysql_query($sql); ?> If you do not want to hash the password, then this would work: [code] <?php require "config.php"; $username=mysql_real_escape_string($_REQUEST["uname"]); $password=$_REQUEST["password"]; $sql="SELECT password FROM users WHERE username='$username' LIMIT 1"; $result=mysql_fetch_assoc(mysql_query($sql)); if (is_array($result)) { if ($result['password'] != $password) die("Invalid username or password"); }else { die("Invalid username or password"); } ?> I almost always store my passwords as an MD5 hash in the DB, securer and makes it easier to check against case sensitivity.
-
<?php $host = "localhost"; $user = "username"; $pwd = "password"; $db = "database"; ?> You are missing the end ?> which in return causes the $end error.
-
PHP 5 just allows alot more functionality. If you are starting to design a new site, I would recommend upgrading. But if you have many sites that run off the PHP 4, it may break them from working. So yea. The rough part with multiple sites is you have to code them all for php 5 before upgrading or you can break them all. As for me, I have been working on coding my sites for PHP 5, since I use OOP alot and PHP 5 is much better at that, and yea it is a pain. I just want PHP 5 for the more functionality and security it provides. Chances are in 5 years, PHP 4 will be like PHP 3, null and void and everyone will be using PHP 5.x while converting over to PHP6. The life cycle of PHP =)
-
I think I understand what you mean, but I'm a bit lost as to the how I would do that...Would I hve to "store" said values in a database table ? No just session: <?php session_start(); if (isset($_POST) && is_array($_POST)) { foreach ($_POST as $key => $val) $_SESSION[$key] = $val; } ?> Then just add this where you want the value displayed: <?php $name = (isset($_SESSION['name']) ? $_SESSION['name'] : ''); echo '<input type="text" name="name" value="' . $name . '" />'; ?> That should get you rolling. Just remember to unset the session data when done or else the next page load it will display the old data.
-
Replace parse_str("$QUERY_STRING"); with if (isset($QUERY_STRING)) { parse_str($QUERY_STRING); } But I think $QUERY_STRING assumes register_globals is on, it is better to code it this way: if (isset($_SERVER['QUERY_STRING'])) { parse_str($_SERVER['QUERY_STRING']); }
-
Your error is in the config.php file. Post that or look through it to see possible errors. Chances are it is missing a } or ; from the sounds of it.
-
Just link back to phpfreaks, never hurts to help promote the site you got your answer from.
-
In the constructor of the class, add a parameter for either each value needed or for an array of all values. Then after you are done using those values set the parameters to null, just in case.
-
Could be many reasons, the most likely one was you were viewing a cached version of the page instead of the new page. When I am testing, especially in IE, I always use ctrl+f5 to refresh to page to make sure I got the latest copy. In firefox, you generally do not have to do that, but I still do it because it is annoying refreshing a page, then changing code etc and then find out it was never getting changed.
-
$ukupno += $svi[$s]; Thats what's throwing the error. if (!in_array($svi[$s],$ukupno)) { $ukupno[] = $svi[$s]; } Hope that helps.
-
<?php while ($row = mysql_fetch_array($result)) { $ename = stripslashes($row['name']); $eemail = stripslashes($row['email']); $epost = nl2br(stripslashes($row['post'])); $grav_url = "http://www.gravatar.com/avatar.php?gravatar_id=".md5(strtolower($eemail))."&default=".urlencode($default)."&size=70"; echo('<li><div class="meta"><img src="'.$grav_url.'" alt="Gravatar" /><p>'.$ename.'</p></div><div class="shout"><p>'.$epost.'</p></div></li>'); } ?> While I look at the code, a rule of thumb is you should never stripslashes on data coming out of database. You should also escape data with mysql_real_escape_string that is going into the DB. Anyhow that should work.
-
Looking through directories for cetain subdirectories
premiso replied to danscreations's topic in PHP Coding Help
scandir opendir Without any attempt at code you probably will not get much help. The following was taken from a comment on the scandir page, I am sure you can tweak it to your needs. <?php /** * returns the folder content names * * @param string $rootDir path to the root folder * @param array $allowext allowed extensions * @param array $notallownames not allowed names * @param array $allData array by reference which collects the root content */ static function ScanDirectories($rootDir, $allowext, $notallownames, &$allData) { $dirContent = scandir($rootDir); static $i=0; foreach($dirContent as $key => $content) { if ($content == '.' || $content == '..') continue; $path = $rootDir . SEPARATOR . $content; $ext = substr($content, strrpos($content, '.') + 1); if(in_array($ext, $allowext) && is_file($path) && is_readable($path) && !in_array($content, $notallownames)) { $allData[$i][name] = $content; $allData[$i][path] = $rootDir; $allData[$i][sub] = ''; } else if(is_dir($path) && is_readable($path) && !in_array($content, $notallownames)) { $allData[$i][name] = $content; $allData[$i][path] = $rootDir; $allData[$i][sub] = array(); self::scanDirectories($path, $allowext, $notallownames, &$allData[$i][sub]); } $i++; } } ?> -
nl2br Should solve your problem.
-
For the error report, put this at the top: error_reporting(E_ALL); ini_set('display_errors', 1); It will tell of any errors. As for why it is not writing it, I would make sure that $station is not over-written anywhere else before it is suppose to be printed...
-
Create the sql file on the server with each insert on a new line. Read the file in with file Do a foreach loop and run mysql_query on each element of the file array. <?php $createTableSQL[] = "CREATE TABLE IF NOT EXISTS `adsense` ( `id` int(3) NOT NULL auto_increment, `code` longtext NOT NULL COMMENT 'adsense code', `comment` mediumtext NOT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=3 ;"; $createTableSQL[] = "CREATE TABLE IF NOT EXISTS `headerimages` ( `id` int(2) NOT NULL auto_increment COMMENT 'image id', `imagename` varchar(255) NOT NULL default '' COMMENT 'user named image', `filename` varchar(255) NOT NULL default '' COMMENT 'name of file', `active` int(1) NOT NULL default '0', PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 COMMENT='images used for the home page header' AUTO_INCREMENT=16 ;"; foreach ($createTableSQL as $sql) { mysql_query($sql); } $sqlFile = file('createSQL.sql'); echo "Populating Tables...Please Wait"; $i=0; foreach ($sqlFile as $sql) { mysql_query($sql); if ($i % 100 == 0) { echo "."; } $i++; } echo "Tables populated!<br />"; ?> I would put the table creators in side the script as an array of strings and loop through them first since they do not have the " and '. You should be fine doing that. You can even add a "Please Wait" deal like in the sqlFile example above. Hopw that helps get you rolling.
-
Try error reporting before trecking through the conditions: <?PHP error_reporting(E_ALL); ini_set('display_errors', 1); include('Connect.php'); include('top.php'); include('pokeinfo.php'); if (!isset($_COOKIE['UserID'])) { $ID = $_COOKIE['UserID']; $ID = mysql_real_escape_string($ID); echo "Sorry, you are not logged in. <a href='Login.php'>Login now</a>"; include('bottom.php'); exit; } // etcc ?> If that pulls up nothing, good luck going through each condition. I had to do that many times. That is why I generally check code at stages and make sure it works instead of coding 300 conditions at once and having to find a needle in a haystack. You live you learn. Just hope you don't repeat the same mistakes in the future.
-
Creating Something that Rotates ad banners?
premiso replied to Gutspiller's topic in PHP Coding Help
PHP is just fine for it. You can easier use sessions or a database to determine what it is. Your line of thinking, while userA sees banner1 userB sees banner2 is hard to track and I would not suggest thinking like that. If you want this you would need a DB and chances are userA can see banner1 while userB sees banner2 but if userC comes on what is he going to see? If you have 50 users and only 10 banners, you have an issue if all users come on. Instead if userA has seen banner1 show banner2 if seen banner 1 and 2 show banner3 etc. Then just reset the hasseen once they have seen the last banner. Or better yet just do a random rotation works great too. -
Whats a "huge ass" amount of data. If it is really significant, I would import it via the mysql console, or via phpMyAdmin, if the file is less than 2mbs. Since browsers tend to timeout after 90seconds if no data is sent to the screen. There are ways around this, but that is just too unpredictable.
-
Force download of remote file saving my bandwidth
premiso replied to aseaofflames's topic in PHP Coding Help
Not a crime if the person does not put in the restrictions to prevent it. But I am with you it should be a crime. You leech my bandwidth and I slap you with a ban for your IP to my site. -
Nope, I hate messing with Mail servers. They just suck. Good luck! Maybe someone else can shine some light. In the mean time I would do some research via google if I were you.