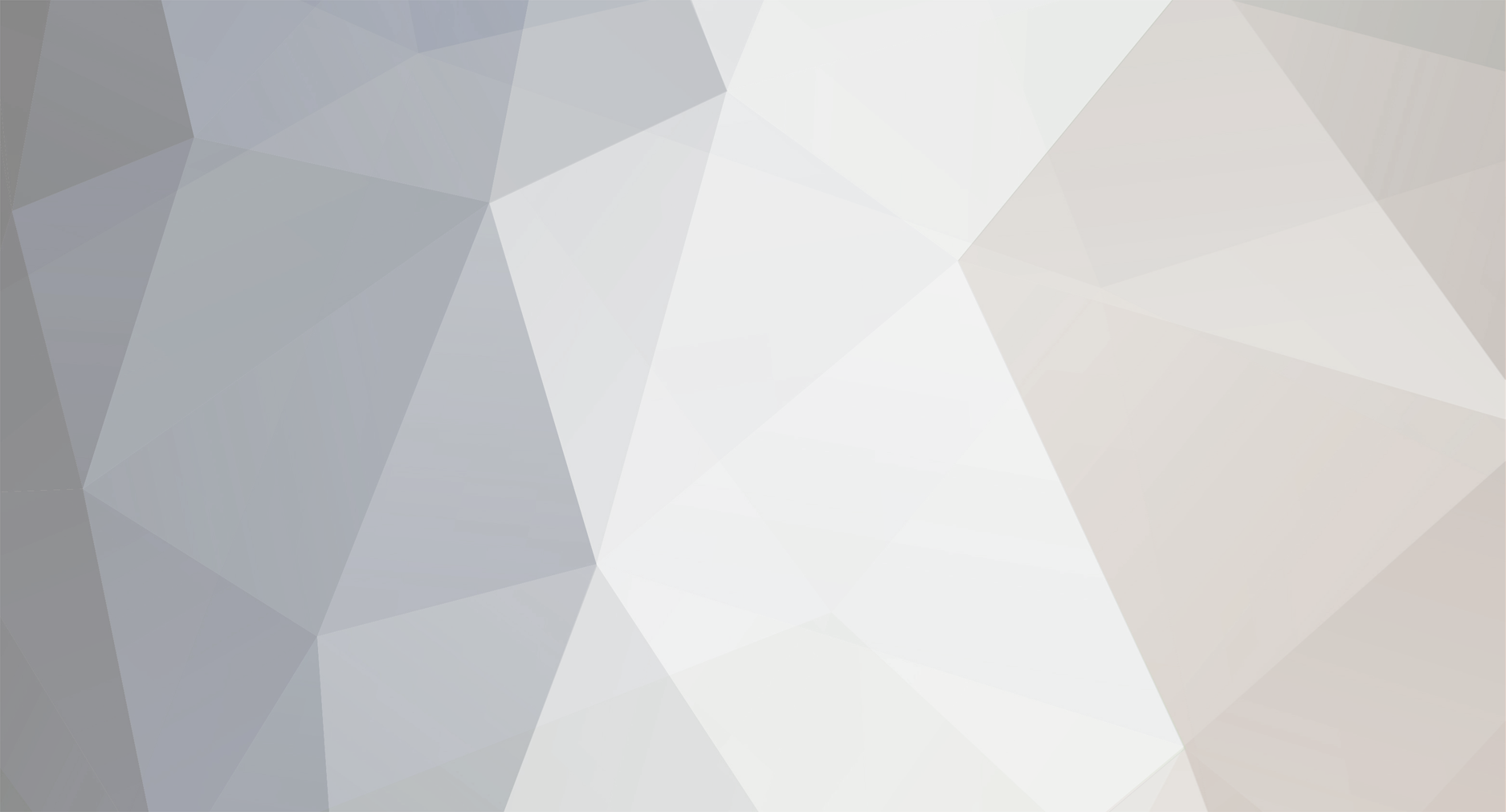
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
Honestly, I would do a clean install of WAMP on your machine. I would not mess with a dedicated production machine for a task like this. I would also use the CLI to run the program, meaning you do not even need apache, so I would not install apache. Simply PHP. You can get the latest version of PHP then modify the php.ini file to set the memory_limit to be 2048M. Then save the script in the same dir as php, change the file paths for the text file and run this command from the php directory: php -f yourscript.php Which should run through. The problem with doing this under Apache / Browser is simply that they can have limitations as well. Doing it with just PHP you can effectively eliminate one of them causing the issue.
-
*premiso vaguely wonders if that will ever get fixed anytime soon.
-
The memory_limit setting, upping that should allow. I am not sure what the correct "scaling" number would be, but try setting it to 1.5GB's instead of 1GB. or 2GB's even.
-
This should break it at 1000 records per extended insert. If you want to change that simply modify the if ($i % 1000) and change 1000 to be whatever you want it to be. <?php ini_set("memory_limit", "1024M"); // sets to 1 GB set_time_limit(0); $items = file_get_contents('test_text.txt'); // If we need to take into account not having line breaks, let's do so. if (strstr($items, "\n") !== false) { preg_match_all('~[0-9]+\|[a-z0-9]+\|[A-Z]{2}\|.*\|[0-9]+\|~Ui', $items, $matches); $matches = $matches[0]; }else { $items = str_replace("\r", "", $items); // just incase. $matches = explode("\n", $items); } $sql = array(); $cnt = count($matches); $x=0; for ($i=0; $i<$cnt; $i++) { $item = explode("|", $matches[$i]); // break at 1000 rows if (($i % 1000) == 0) { $x++; $sql[$x] = array(); } $sql[$x][] = "('" . escapeSq($item[0]) . "', '" . escapeSq($item[1]) . "', '" . escapeSq($item[2]) . "', '" . escapeSq($item[3]) . "', '" . escapeSq($item[4]) . "')"; } $fh = fopen('sql_file.sql', 'w'); foreach ($sql as $stmt) { fwrite($fh, "INSERT INTO table_name (col1, col2, col3, col4, col5) VALUES " . implode(",\n", $stmt) . ";\n"); } fclose($fh); echo "Completed.\n"; function escapeSq($string) { return str_replace("'", "\\'", $string); } ?>
-
if (preg_match_all('~[(]{0,1}[0-9]{3}[)- _]{0,1}[0-9]{7}~', $string, $matches)) { Should resolve that.
-
<?php $string = "asdfasdf asdf asdf asdf asdf asdf 085-7466081 087-9911143 asdfasdf asdf asdf asdf asdf asdf 086-6814743 asdfasdf asdf asdf asdf asdf asdf (087)-2084528 asdfasdf asdf asdf asdf asdf asdf 086 8281633"; if (preg_match_all('~[(]{0,1}[0-9]{3}[- _]{0,1}[0-9]{7}~', $string, $matches)) { $number = array(); foreach ($matches as $match) { $number[] = preg_replace('~[^0-9]~', '', $match); } print_r($number); } ?> Should output: Array ( [0] => Array ( [0] => 0857466081 [1] => 0879911143 [2] => 0866814743 [3] => 0868281633 ) ) The regex can probably be done better / more efficient but there was my attempt at it EDIT: Posted after Ken's as mine removes the - ( and spaces as well.
-
Here is a basic mock up and how I would handle it. First up, you will need to change your allowed memory for php, this can be changed with ini_set or in the php.ini file, I would suggest the ini_set so this script only gets the extra memory. You will also need to set_time_limit to 0 (for unlimited). Finally, you will need to have some type of access to your MySQL command line so you can import the file we are going to create. As you will not be able to do this over the internet due to the shear size of the file and the amount of inserts it is going to do. Just will not happen. This is untested, as I do not have large enough files but the theory should work. I should also mention, linebreaks are not <br /> in text files they are a "hidden" character \n. This will only show up in command line / editing the file type of environment. So your file may in deed have line breaks in it, however, the code I am giving you, that will not matter. The following script takes into account if there are line breaks or not, so it should work either way. I tested it on a 200MB file, it took about 15-20 seconds to complete. Questions let me know. <?php ini_set("memory_limit", "1024M"); // sets to 1 GB set_time_limit(0); $items = file_get_contents('test_text.txt'); // If we need to take into account not having line breaks, let's do so. if (strstr($items, "\n") !== false) { preg_match_all('~[0-9]+\|[a-z0-9]+\|[A-Z]{2}\|.*\|[0-9]+\|~Ui', $items, $matches); $matches = $matches[0]; }else { $items = str_replace("\r", "", $items); // just incase. $matches = explode("\n", $items); } $sql = array(); foreach ($matches as $match) { $item = explode("|", $match); $sql[] = "('" . escapeSq($item[0]) . "', '" . escapeSq($item[1]) . "', '" . escapeSq($item[2]) . "', '" . escapeSq($item[3]) . "', '" . escapeSq($item[4]) . "')"; } $fh = fopen('sql_file.sql', 'w'); fwrite($fh, "INSERT INTO table_name (col1, col2, col3, col4, col5) VALUES " . implode(",\n", $sql) . ";"); fclose($fh); echo "Completed.\n"; // escapes single quotes to not break the sql. function escapeSq($string) { return str_replace("'", "\\'", $string); } ?> Now I am not sure if MySQL limits how many extended inserts can be done, so if that causes guff we just need to add in logic to do another insert after x amount of records.
-
I think you are better off using a regular expression using preg_match_all I am not the best at Regex and I am sure Salathe will have criticism to this, but it works, so yea. $items = "item-name|description for item|12345|5432|5|item name|description for item|12345|5432|5|item name|description for item|12345|5432|5|item name|description for item|12345|5432|5|"; // item name|description for item|item number|item stock reference|item quantity| preg_match_all('~([a-z0-9.\-_\'" ]+)\|(.*)\|([0-9]+)\|([0-9]+)\|([0-9]+)\|~U', $items, $matches); echo "<pre>", print_r($matches, true), "</pre>"; Should give you an idea of how the multi-dimensional array, which is returned, is setup.
-
Try changing it to this: $to = $row["email"] . ', email@hopethisworks.com' ; The main issue is that you need to put the comma inside of the quotes (since it needs to be apart of the string) and you need to concatenate the $row['email'] (which is done with the period character) on to the string as well. Let me know if this is confusing.
-
No, it is $field, but you may want your ternary if statements setup like this: $fields[] = (isset($_POST['proname']) && !empty($_POST['proname']))?"`proname` = '{$_POST['proname']}'":'';
-
Neither. $fields[] = isset($_POST['interestedin'])?"`interestedin` = '" . strtolower($_POST['interestedin']) . "'":''; Would be the correct way to do that.
-
Modifying the pointer for a multidimensional array
premiso replied to cyberRobot's topic in PHP Coding Help
I think the functionality you are looking for is: array_slice. -
Eh hit quote instead of modify. Fixed in previous post.
-
Given that you want to use fields: // grab the form fields. $fields = array(); $fields[] = isset($_POST['proname'])?"`proname` = '{$_POST['proname']}'":''; $fields[] = isset($_POST'interestedin'])?"`interestedin` = '{$_POST['interestedin']}'":''; $fields[] = isset($_POST['lookingfor'])?"`lookingfor` = '{$_POST['lookingfor']}'":''; foreach ($fields as $key => $field) { if (empty($field)) { unset($fields[$key]); } } mysql_query("UPDATE profile SET " . implode(", ", $fields) . " WHERE where `user_id`='$user->id'") or trigger_error("Update Failed: " . mysql_error()); Should give you a better idea what is going on. And for reference the ? and : make up the ternary operator which is just a shortened if / else statement. (if some condition) ? do this : else this; Given that you do not care what gets lowerecased, I added another statement in the foreach to remove empties to escape the data (As it should be escaped to prevent SQL injection) and lowercase the variable. If magic_quotes are on, it will stripslashes before escaping it to avoid dupping the slashes. Removed that part because, yea I was just being an idiot. You should look into mysql_real_escape_string and use it with get_magic_quotes_gpc to verify if you need to stripslashes before you escape to prevent SQL Injection Hope that helps ya.
-
Dynamically build the update query and leave out any empty fields. For an example of how to dynamically build a SQL Query from GET data you can take a look at this tutorial: http://www.phpfreaks.com/tutorial/simple-sql-search
-
Contact form Mail lands-up only in spam or junk folder Help
premiso replied to dingi's topic in PHP Coding Help
http://old.openspf.org/dns.html -
Thanks for the link Neil, maybe it will help me "Freshen" up on my OOP
-
http://www.w3.org/TR/xhtml1/ You may do well to read up on the standards for the doctoype. If that is not of much help, well provide us with the first 10 lines of your html file so we can see that your are indeed not doing any funky stuff.
-
Time-Sensitive...but I thought time was just a figment of our imaginations. So therefore this topic really does not exist. And poof, it has been ignored!
-
Pseudocode is the only code I program in!
-
I really want to punch Justin Bieber in the face!
premiso replied to Orionsbelter's topic in Miscellaneous
Who? -
I really want to punch Justin Bieber in the face!
premiso replied to Orionsbelter's topic in Miscellaneous
-
You can find a tutorial on how to do this here: http://www.slunked.com/blog/php-mysql/2010/01/simple-sql-search-using-php-mysql
-
page_title I would do a varchar(50) As it is not a set width, like a password hash would be. IE: If you know how long the string has to be no more no less, use Char. If it can var, use VARCHAR. page_content it depends on your need, it can be clob / text / bigtext etc. Read up on them and determine which is right for you. Page_keywords, save as title, varchar(100) since it can be anything from 0-100.
-
Arg!!! I am going crazy with header function !!
premiso replied to jtorral's topic in PHP Coding Help
If the above suggestions do not work, chances are your script is erroring out before it hits the header call. Make sure error reporting is set to E_ALL and display errors is turned on for development so you can actively see the error and debug properly.