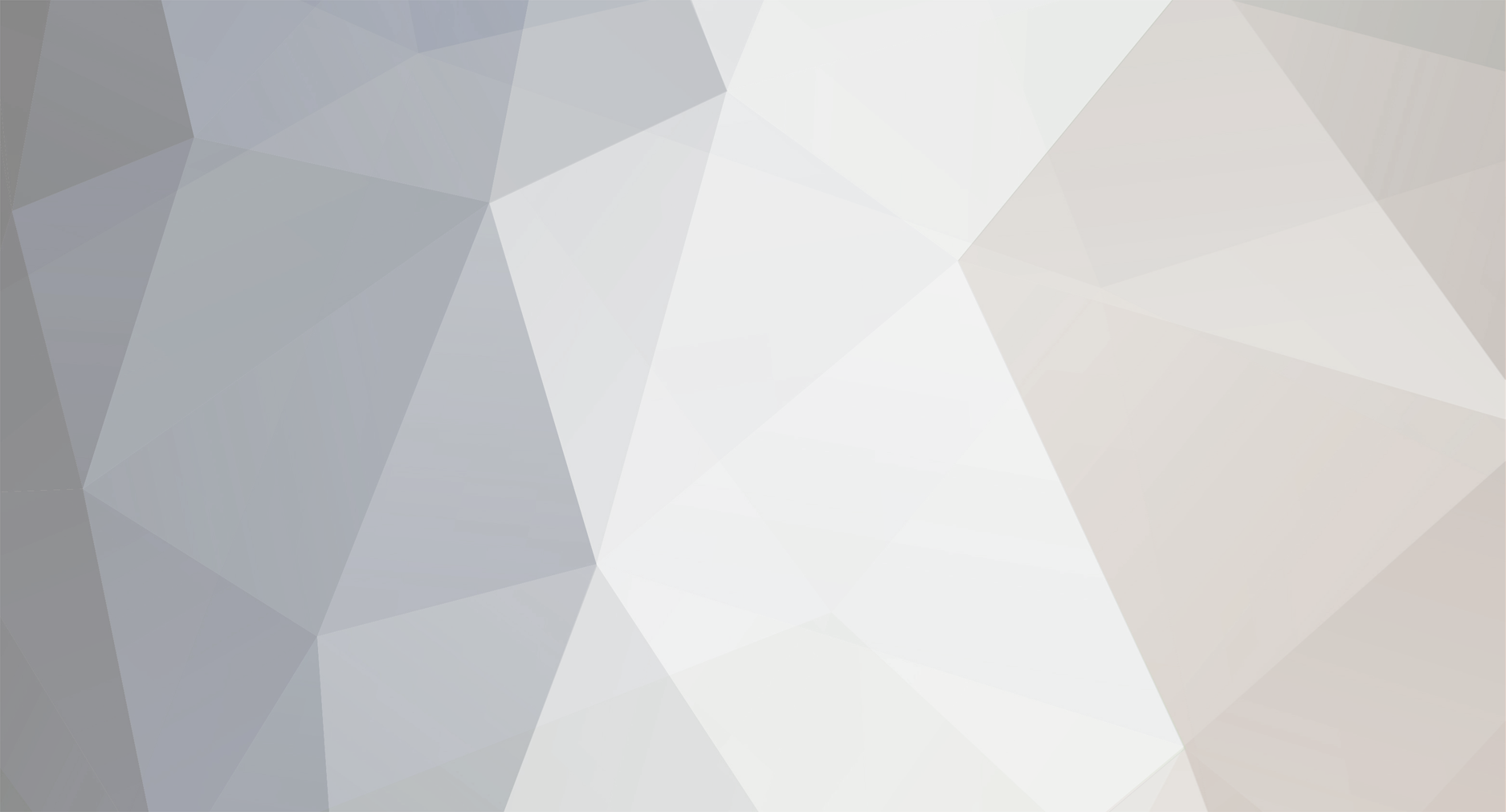
mbeals
Members-
Posts
247 -
Joined
-
Last visited
Never
Everything posted by mbeals
-
Thanks. strangely enough, just using a single file_get_contents() call worked perfectly. No clue why CURL was giving me so many problems.
-
I've fought with this on more than a few occasions. The issue is always with the server's configuration. ****I'm assuming Linux server here....no idea how to work it on a windows machine********** open up a second terminal and 'tail -f /var/log/syslog' then run the script. You should get some errors that point you in the right direction. Are you attempting to use sendmail or postfix? have you modified your php.ini file? Are you behind a firewall that blocks stmp traffic? I've had the most luck using postfix to relay the mail out.
-
I have some equipment at remote sites that is managed by an appliance with an HTTP interface. I'm working on an automated script to log into the HTTP interface and fetch the status of the attached equipment. The appliance uses GET based, plain text authentication (not the best, but we have it IP locked over a secure link). I can successfully connect to the appliance and fetch the HTML containing the info I need, but the session is hanging, and causing the appliance to lock out any other sessions for a period of time. I know the session is hanging, because I can watch is stuck in ACK-FIN with lsof. I think the problem is the session is being abandoned after the initial login and data fetch, so when I issue the logout command, it initiates a new session, leaving the old one hanging (making the appliance think I'm still logged in). This is the code that I'm using: <?php $IP = 'xxx.xxx.xxx.xxx'; $user = 'admin'; $pass = 'xxx'; $login = "UserID=$user&Pwd=$pass"; $info = "HeadendStatus.htm?"; $logout = "LOGIN.htm?LOGOFF=LOG+OFF&"; //Fetch Status $ch = curl_init("http://$IP/".$info.$login); curl_setopt($ch, CURLOPT_HEADER, 0); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 20); $data = curl_exec($ch); //Log out curl_setopt($ch, CURLOPT_URL,"http://$IP/".$logout.$login); $result=curl_exec($ch); curl_close($ch); // Process data..... ?> So, any thoughts on how to simulate login->fetch data->log out with curl, or any other functions?
-
so I made a brand new page : <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Untitled Document</title> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1"> <script type='text/javascript'> function with_var(){ var img_tag = document.getElementById('image'); alert(typeof img_tag); alert(typeof img_tag.src); } function without_var(){ img_tag = document.getElementById('image'); alert(typeof img_tag); alert(typeof img_tag.src); } </script> </head> <body> <img src='images/lock.gif' id='image'> <a href='#' onClick='with_var()'>With Var</a> <a href='#' onClick='without_var()'>Without Var</a> </body> </html> and it works the same in both IE and firefox...no errors img_tag is a object and img_tag.src is a string. I put the same functions in my main script page and it dies. There has to be something else in there interfering. I guess I have some digging to do. Thanks for your help.
-
It's interesting though that it works in firefox and the the img tag is the only one that breaks. I have tried to declair them each time in the calling function, and the img tag still breaks: function test(){ img_tag = document.getElementById('img_1'); alert(img_tag.src); } This pukes in IE too (but not firefox). Change img_1 to an a tag, and all is good. edit.... apparently that dies, but function test(){ var img_tag = document.getElementById('img_1'); alert(img_tag.src); } does not. I was under the impression that declaring a variable without a 'var' statement inside a function made that variable a global. I think I'm going to do the returned array route though
-
in the actual code though that statement is part of a function. I also have other getElementById() calls for other objects (tr,a, etc) that work fine. Here's actual full code: function load_obs(){ wo_textbox = document.getElementById('wo_number'); wo_hlink = document.getElementById('wo_link'); wo_img = document.getElementById('wo_img'); } function change_button(style){ load_obs(); switch(style){ case 'edit': wo_hlink.setAttribute('onClick', 'wo_edit()'); wo_hlink.title = 'edit'; wo_img.src = 'images/edit.png'; break; case 'save': wo_hlink.setAttribute('onClick', 'commit_wo()'); wo_hlink.title = 'save'; wo_img.src = 'images/accept.jpg'; break; } } <a href="#" onClick='commit_wo()' id='wo_link' ><img src='images/accept.jpg' alt='accept' width='16' height='16' border='0' id='wo_img'/></a>
-
<script> var img_tag = document.getElementById('img_1'); alert(img_tag.src); </script> <img id='img_1' src='images/testimage.jpg' /> That's quick and dirty code that breaks. I could post everything, but I know it's a combo of that html img tag and that getElementById() call; [/code]
-
I have a script that changes the src attribute of an img tag. When I fetch the object reference through document.getElementById(), IE returns the error: "Object doesn't support this property or method" It works fine in firefox. Did MS discontinue the id property of img tags?
-
Sorry, I figured out a workaround, but I'm not completely happy with it. Basically I want to create an object that extends the p object such that when I append it as a child to another object (table cell), it treats it like a p object. here's some sample code: function button(type,id){ this.id = id; this.type = type; this.p_tag = document.createElement('p'); this.a_tag = document.createElement('a'); this.img_tag = document.createElement('img'); this.img_tag.width = 16; this.img_tag.height = 16; this.img_tag.border = 0; this.p_tag.appendChild(this.a_tag); this.a_tag.appendChild(this.img_tag); this.a_tag.setAttribute('href', '#'); switch(this.type){ case 'accept' : this.a_tag.setAttribute('onClick', 'accept('+this.id+')'); this.img_tag.src = 'images/accept.jpg'; this.img_tag.alt = 'accept'; this.img_tag.title = 'accept'; break; case 'cancel' : this.a_tag.setAttribute('onClick', 'cancel('+this.id+')'); this.img_tag.src = 'images/restart.jpg'; this.img_tag.alt = 'cancel'; this.img_tag.title = 'cancel'; break; case 'delete' : this.a_tag.setAttribute('onClick', 'del('+this.id+')'); this.img_tag.src = 'images/b_drop.png'; this.img_tag.alt = 'delete'; this.img_tag.title = 'delete'; break; case 'edit' : this.a_tag.setAttribute('onClick', 'edit('+this.id+')'); this.img_tag.src = 'images/b_edit.png'; this.img_tag.alt = 'edit'; this.img_tag.title = 'edit'; break; } } with this code I can do this: var cell = document.getElementById('cell'); var accept = new button('accept','button1'); var cancel = new button('cancel','button2'); cell.appendChild(accept.p_tag); cell.appendChild(cancel.p_tag); I would prefer this behavior: var cell = document.getElementById('cell'); var accept = new button('accept','button1'); var cancel = new button('cancel','button2'); cell.appendChild(accept); cell.appendChild(cancel); in which case the base 'button' class would really be an extension of the p class. Does that help?
-
I have a page that does dynamic database updates/queries via ajax. Part of this allows the user to click an edit icon in a table row, which switches that row into "edit mode". When I switch between modes, I change the layout of buttons (in the form of hyperlinked images) in the last cell. Each "button" consists of a p tag with a href child with an img child. I build each object individually, then use appendChild to nest them. Finally, I use appendChild again to assign the final "Button" to the cell in the table. To simplify this, I created an object that takes button type as an argument. I want to be able to do something like: var cell = getElementById('cell'); var accept = new button('accept'); var cancel = new button('cancel'); cell.appendChild(accept); cell.appendChild(cancel); I appologize for not having full code. My internet connection is currently down and I'm typing this up on by phone. I will post full code a little later if needed.
-
Email body empty in Gmail & body gets cut off in Mac exchange server
mbeals replied to freephoneid's topic in PHP Coding Help
did you take "Content-Transfer-Encoding: base64" out of the header too? poking around, I found two things that may be important here: 1. there is a limit on email line length (70 chars). So be sure to: $body = chunk_split( base64_encode( $body ) ); or $body = word_wrap($body,70); 2. I found issues with sending utf-8 encoded mail with mail(). Possibly remove that from the header. 3. I found numerous issues with gmail compatibility and lots of different 'tricks' browse through the comments in the man entry: http://us2.php.net/manual/en/function.mail.php that and googleing 'php mail() gmail' turned up a lot. good luck -
Email body empty in Gmail & body gets cut off in Mac exchange server
mbeals replied to freephoneid's topic in PHP Coding Help
my guess is something with the encoding. Try sending the mail with the body as plain text (ditch the base64_encode) and just see if it fixes the problem. -
this is cool technology and all and I think it definitely is where the future of portable devices is heading, but how easy would it be for someone to steal your power? Look at how easy it is to pick up stray wifi.... What's to stop my neighbor (in an apartment building) from connecting to my wireless grid that I happen to place too close to the shared wall?
-
I actually kind of figured it out: SELECT `orders`.*, progress.* from `orders` left join (Select `order`, COUNT(*) as attached from order_progress group by `order`) as progress on `orders`.id = progress.`order` WHERE attached < num_receivers"; Now the issue I have is if I have a record in `orders` and nothing that references that record in order_progress, the COUNT(*) returns NULL instead of 0, which breaks the logic in the WHERE statement.
-
I have an order tracking system. I have one table that has the details of the actual order, including the number or items being ordered. When we begin processing an item, an entry is created in a transaction table, linking the specific item to the order. IE: CREATE TABLE IF NOT EXISTS `orders` ( `id` int(11) NOT NULL AUTO_INCREMENT, `wo` int(11) NOT NULL, `customer` int(11) NOT NULL, `num_items` int(11) NOT NULL, `details` text NOT NULL, PRIMARY KEY (`id`); CREATE TABLE IF NOT EXISTS `order_progress` ( `id` int(11) NOT NULL AUTO_INCREMENT, `order` int(11) NOT NULL, `item` int(11) NOT NULL, `shipment` varchar(20) NOT NULL, `scanned` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP, `called` timestamp NOT NULL DEFAULT '0000-00-00 00:00:00', `boxed` timestamp NOT NULL DEFAULT '0000-00-00 00:00:00', PRIMARY KEY (`id`) I need a way to only pull the records from `orders` where the number of associated records in `order_progress` are less then the number in ordes.num_items.
-
What I'm trying to get to is a way to reference multiple devices with different attributes from a single place. I maintain a transaction log which logs activity, such as shipping a device out, or performing maintenance. When I query this log, I would like to be able to retrieve the information about the device referenced in the same query. So if I have table: CREATE TABLE `TransactionLog` ( `transactionID` INT NOT NULL AUTO_INCREMENT PRIMARY KEY , `deviceID` INT NOT NULL, `timestamp` INT NOT NULL , `comments` FULLTEXT, .......... ) ENGINE = InnoDB ; I need that deviceID to reference a unique device in such a way that I can write a join statement that would result in something like: transactionID deviceID timestamp type OS IP comments 1 1 March 3, 2008 Server BSD 192.168.1.5 Server Installed 2 2 March 5, 2008 Router [/td] 192.168.1.1 Configuration updated 2 3 March 6, 2008 Switch-unmanaged [td] Eaten by wild boars am I going to have to store the table name in the 'device_type' table, then write some crazy query using subqueries?
-
I have a database for tracking assets. Because different assets have different attributes that need to be logged, I can't have a single table that handles every asset. I need a way to reference these individual tables back to a common table so that I can perform joins. So in essence, I have a `devices` table: CREATE TABLE `Devices` ( `deviceID` INT NOT NULL AUTO_INCREMENT PRIMARY KEY , `type` INT NOT NULL , `location` INT NOT NULL ) ENGINE = InnoDB ; and tables for types of devices: CREATE TABLE `Routers` ( `deviceID` INT NOT NULL AUTO_INCREMENT PRIMARY KEY , `Model` INT NOT NULL , `Serial #` INT NOT NULL, `IP` VARCHAR(20) NOT NULL ) ENGINE = InnoDB ; CREATE TABLE `Servers` ( `deviceID` INT NOT NULL AUTO_INCREMENT PRIMARY KEY , `Model` INT NOT NULL , `Serial #` INT NOT NULL, `OS` VARCHAR(20) NOT NULL ) ENGINE = InnoDB ; I know I can apply a foreign key across deviceID, so that keys across the different device tables don't clash. So here are my questions: 1. How can I reference the appropriate table in the Devices table, so that I can query data from the child tables without knowing what they are? IE: Select Devices.*, Devices.type.* from `Devices` join Devices.type using deviceID. I know that doesn't work, but I hope it gets the point across. 2. When I insert to this database, how do I make the auto increment key span the tables. Do I just need to drop the auto_increment from the Devices table and use something like mysql_insert_id() to grab the auto-inc value from the subtable insert, then update the Devices table? I would love to be able to write a single insert statement that would update Devices and the sub table at the same time as if it were a single entity. The problem with this, however, is that I may need to be able to reference a simple device that doesn't have any attributes outside of what are already in the Devices table. 3. Can any of this be translated over to MSSQL? Unfortunately I'm developing on mysql, because that is what I have to work with, but the company wants to eventually migrate it all onto their MSSQL server. So far everything I've done translates, but I want to make sure the two servers handle foreign keys and the like similarly. Any help would be greatly appreciated.
-
I have an email formatting script that reads in data from environmental variables, generates an email body and then sends it. I format the body of the email in heredoc with my editor set to unix style newlines. I have a variable that contains text (including new line chars) that I need to insert into the heredoc. When I do so, I notice that my '\n' chars are being treated as literal text when viewed in outlook. So I used str_replace to change them. I'll just show the results of my trials as it will be easier then explaining it. first, base code: <?php $subject = "Alert: $SERVICEDESC on $HOSTNAME is $SERVICESTATE"; $priority = "X-Priority: 1\r\nPriority: Urgent\r\nimportance: high"; $body = <<<EOT The $SERVICEDESC service on $HOSTNAME has entered the $SERVICESTATE state on $CURRENTTIME. The plugin performing the check returned the following output: $SERVICEOUTPUT $LONGSERVICEOUTPUT *************************************** Host: $HOSTNAME Service: $SERVICEDESC Current State: $SERVICESTATE Previous State: $LASTSERVICESTATE Valid Time: $CURRENTTIME EOT; ?> In this config, the $LONGSERVICEOUTPUT portion of the email is formatted as: OK - 8 services checked, 8 ok [ 1] Registered_Modems Registered_Modems OK - 0 \n[ 2] Active_Modems Active_Modems OK - 0 \n[ 3] bitrate bitrate OK - 0 bps \n[ 4] snr SNR: 24 dB OK \n[ 5] fec_Unerroreds Unerroreds OK - 504421 c \n[ 6] fec_Correcteds Correcteds OK \n[ 7] fec_Uncorrectables Uncorrectables OK \n[ 8] Microreflections Microreflections OK - 0 c The new line chars are printed as text. Encasing the variable with {} does not help. $LONGSERVICEOUTPUT = mysql_escape_string($LONGSERVICEOUTPUT); results in: OK - 8 services checked, 8 ok [ 1] Registered_Modems Registered_Modems OK - 0 \\n[ 2] Active_Modems Active_Modems OK - 0 \\n[ 3] bitrate bitrate OK - 0 bps \\n[ 4] snr SNR: 24 dB OK \\n[ 5] fec_Unerroreds Unerroreds OK - 504421 c \\n[ 6] fec_Correcteds Correcteds OK \\n[ 7] fec_Uncorrectables Uncorrectables OK \\n[ 8] Microreflections Microreflections OK - 0 c So it appears as if php is parsing the \n as a special char. if I do: $LONGSERVICEOUTPUT = str_replace('\n',"\r\n",$LONGSERVICEOUTPUT); $LONGSERVICEOUTPUT = mysql_escape_string($LONGSERVICEOUTPUT); I get [ 1] Registered_Modems Registered_Modems OK - 0 \r\n[ 2] Active_Modems Active_Modems OK - 0 \r\n[ 3] bitrate bitrate OK - 0 bps \r\n[ 4] snr SNR: 23 dB WARNING \r\n[ 5] fec_Unerroreds Unerroreds OK - 504485 c \r\n[ 6] fec_Correcteds Correcteds OK \r\n[ 7] fec_Uncorrectables Uncorrectables OK \r\n[ 8] Microreflections Microreflections OK - 0 c however, $LONGSERVICEOUTPUT = str_replace("\n","\r\n",$LONGSERVICEOUTPUT); $LONGSERVICEOUTPUT = mysql_escape_string($LONGSERVICEOUTPUT); returns [ 1] Registered_Modems Registered_Modems OK - 0 \n[ 2] Active_Modems Active_Modems OK - 0 \n[ 3] bitrate bitrate OK - 0 bps \n[ 4] snr SNR: 23 dB WARNING \n[ 5] fec_Unerroreds Unerroreds OK - 504501 c \n[ 6] fec_Correcteds Correcteds OK \n[ 7] fec_Uncorrectables Uncorrectables OK \n[ 8] Microreflections Microreflections OK - 0 c indicating that it can match the string literal \n but not the new line character. if I compromise and do $LONGSERVICEOUTPUT = str_replace('\n',"\r\n",$LONGSERVICEOUTPUT); I get extra printed newlines [ 1] Registered_Modems Registered_Modems OK - 0 [ 2] Active_Modems Active_Modems OK - 0 [ 3] bitrate bitrate OK - 0 bps [ 4] snr SNR: 23 dB WARNING [ 5] fec_Unerroreds Unerroreds OK - 504501 c [ 6] fec_Correcteds Correcteds OK [ 7] fec_Uncorrectables Uncorrectables OK [ 8] Microreflections Microreflections OK - 0 c Is this an issue with heredoc, with windows line feeds or what? All I want is a single spaced list.
-
well the only other server going in (for now) is going to be named Zuul. It's in charge of provisioning cable modems and serving as the 'gatekeeper'.
-
i think i found a winner. A friend suggested Uatu, which is very geeky, but not so obscure that it's lost on everyone.
-
I was thinking along the lines of a norse god, but wans't familiar enough with the mythology to know of specific one. Heimdall actually fits really nicely. It's also funny that my other thoughts were transformer and LoTR names as well
-
I'm building a new server here at work to centralize my network monitoring. It just sits there and watches the network and attached devices, and sends out alerts if something isn't working right. I have the software and the configuration all worked out....but it lacks a cool hostname. I'm looking for a character from either popular fiction (read movies) or history (some greek/eqyption/norse god) that was all seeing/knowing but not necessarily powerful (omniscient but not omnipotent) The geekier the reference the better, and this place can't get much geekier, so give me your best stuff. What are your ideas?
-
<?php $text = <<<EOT host=mail.gmail.com username=abc@gmail.com password=uuuuuuuuuuu peer_host=mail.peer.com EOT; echo $text; preg_match("/^host=(.*)/",$text,$matches); print_r($matches); $host = $matches[1]; ?>
-
so the UK team has no issues running the same scripts, but the Indian team does? Have the indian team run a traceroute on the server, or better yet, use MTR. Have the UK team do the same and compare results. My first instinct in a matter like this is a slow hop or networking issue. Is this server multi-homed or part of a load balanced cluster?
-
if the script was run through apache (opened in a web browser) it would have timed out already...meaning it's not in an infinite loop. srv07:/ # ps aux|grep index.php root 2924 0.0 0.2 1828 616 pts/0 S+ 16:54 0:00 grep index.php is showing you that process 2924 is "grep index.php" not "index.php". It's the PID of the grep command you just issued, not the script actually running. Also, as far as I know, when a script is executed via the apache module, it doesn't show up as a separate process (unless it forks, and even then I'm not sure it would). So in short... the chances of the script still running are slim to none.