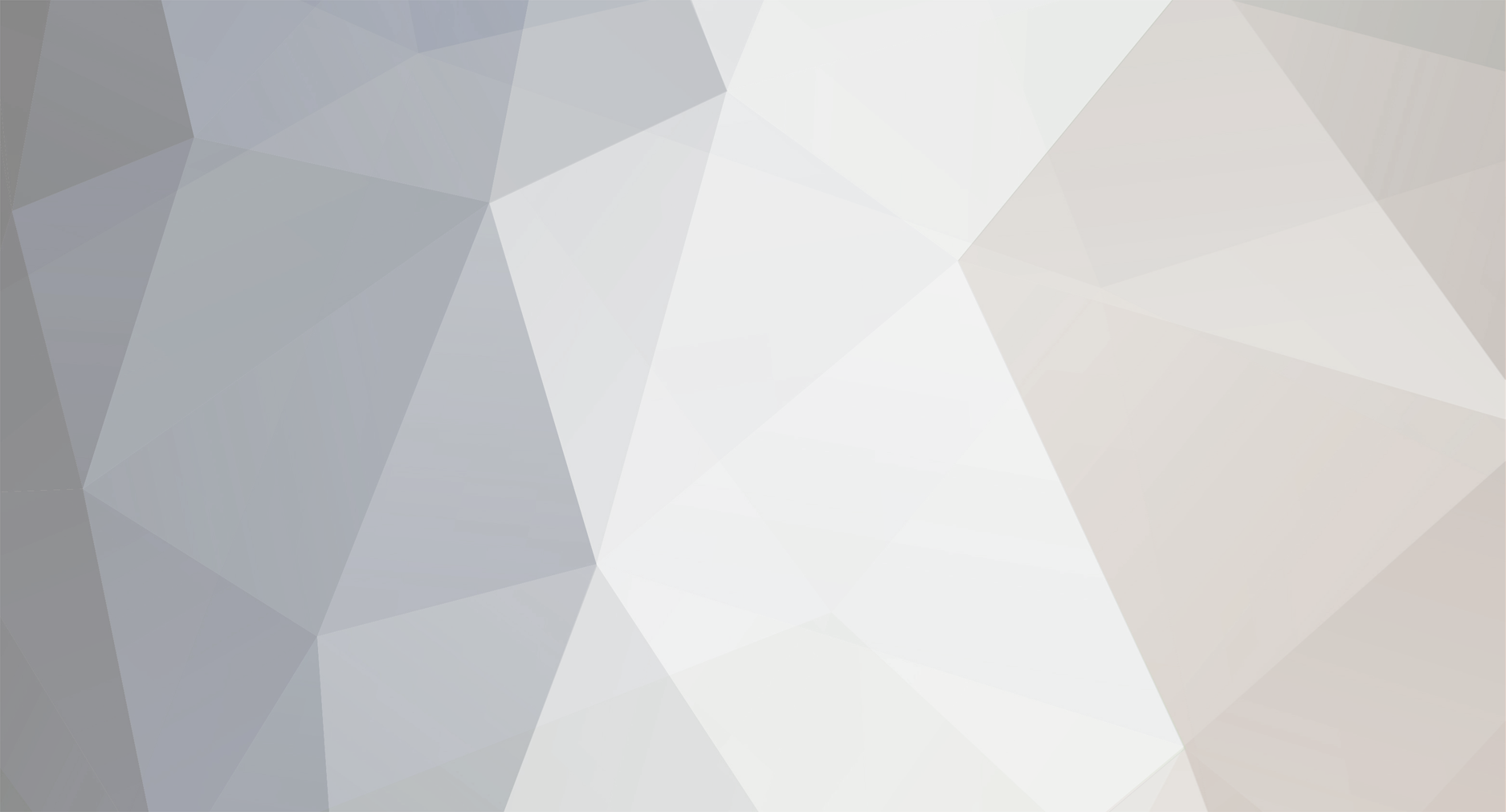
mbeals
Members-
Posts
247 -
Joined
-
Last visited
Never
Everything posted by mbeals
-
first off, do you realize that your formula works for all cases except for wins & losses = 0? <?php if(!$Wins && !$Losses){ $Perc = 0.5; }else{ $Perc = $Wins /(float)($Wins + $Losses); } echo $Perc; ?> but.... your original code should work. You might want to check $Wins and $Losses just before the IF and make sure they are what you think they are
-
if the script is in a loop, deleting the original file won't do anything, as it is running from memory. do: ps -aux |grep php or ps -aux |grep "nameofscript" and see if it brings anything up. Look at the emails you are getting too. Is the 'sent time' in the header the same for all of them? If it is, or it's very similar, then chances are the que is full. If the sent time keeps up with the receive time, then i'd guess the script is running. If this script was initiated through apache (IE a web interface), then chances are it is not still running, as it would have timed out by now
-
are you sure the user you are logging in with has permissions to log in remotely?
-
i'm assuming you are using a loop to iterate through the pages. if you are worried about DOSing the site, then insert a sleep() command within the loop. This will run the loop once, then make the script sleep for however many seconds you tell it to. You could also do something like: <?php for($i=0;$i<$records;$i++){ //download page #$i if($i % 25) sleep(60); } ?> That would make the script sleep for 1 minute every 25th record. In this configuration (specifying the server as a command line arg) one running process will access one server. With a little trickery you could get the script to alternate servers, so that it never hits the same server twice in a row. You might want to take a look at some articles on daemon programming as a lot of the concepts apply to this sort of thing.
-
you may not even need cron. Cron is used if you want to run a script on a set interval (like every 5 minutes). it sounds like you just want to iterate through one server, then repeat on the next. I suspect you cannot do more then 25 pages because the php page is timing out. When you run a script from the command line, there is no time out. You can have a script that loops forever (essentially how daemons .... services in windows speak... work). so take your db_build.php script and add this to the top, removing the $name = $_POST stuff. <?php $name = $_SERVER["argv"][1]; if(!$name) die("No server defined!\n"); ?> Then modify the rest of it to loop through the entire server, instead of just the first 25. Then open a terminal on the server, chmod the file then execute it: chmod a+x db_build.php php -q db_build.php 122 & if you need to run this on a schedule (say you want to backup the website every day at noon), then you would insert that command into the crontab.
-
<?php switch($positoin){ case 1: $style = 'GK'; break; case 2: $style = 'DEF'; break; case 4: $style = 'MID'; break; case 6: $style = 'STR'; break; } ?>
-
in your Time_3.php <?php echo $_GET['name']; ?> for debugging form issues, place this at the top of your code (on the processing page): <? echo "<hr><pre>"; echo "GET:\n"; print_r($_GET); echo "\nPOST:\n"; print_r($_POST); echo "</pre><hr>"; ?> to see all form data being passed
-
the 0 * * * * is the cron schedule.... run on minute 0, every hour, every day of the month, every month of the year, every day of the week. man crontab for more details. I'm guessing you aren't installing the cronjob correctly. from shell, type in: crontab -e <enter> This opens the crontab. Paste that text in there and exit (with save). /usr/bin/find is the path to the find binary which is searching the folder for the files and removing them. If cron still isn't working, just paste this directly to a command line: /usr/bin/find /home/halo2freeek/infectionist.com/misc/bungie_webcam/images -mtime +24 -exec rm {} if you still get an error, post it.
-
I do this with one of my pages (with success). Just some things to consider: 1. if you use self recursive pages (pages that call themselves to process form data) use sessions to store the page index. On your index.php page, include something like this: <?php session_start(); function pageTranslate($id){ switch($id){ default: return 'default.php'; case 'users': return 'users.php'; case 'stats': return 'stats.php'; } } $site = isset($_GET['site']) ? pageTranslate($_GET['site']) : (isset($_SESSION['site']) ? $_SESSION['site'] : 'default.php'); $_SESSION['site'] = $site; include('$site'); ?> This first checks the get variable 'site' to see if a different page is being requested. If so, it translates the page reference into the proper php file name and stores it in the session variable. If a different page isn't being requested, it just uses whatever is stored in the session variable. If there is nothing, it show's the default page. now you only need to specify 'site' in the url when switching to a new page. You can call index.php all you want and it will continue to call the same page. It REALLY makes life easier. 2. Transform that pageTranslate function into one that queries a mysql database. You can add and reassign pages by moving stuff around in a database instead of editing the source. Use the same database table to generate your links. This makes it easier to implement user access levels too, as you can limit what links are shown to certain users and validate the link against their access rights before loading the page. 3. Learn to love modrewrite for apache and use it for common pages. Say you access the forums.php page with $site = forums and tutorials.php with $site = tutorials.php. Write modrewrite rules to that www.PhonePwners.com/forums is translated to www.PhonePwners.com?site=forums and www.PhonePwners.com/tutorials is translated to www.PhonePwners.com?site=tutorials. You don't have to do it to all of your sites, but it is handy for direct access to certain parts.
-
[SOLVED] Submit button calling two php scripts???
mbeals replied to budimir's topic in PHP Coding Help
no, you have the html page with the form on it. Point to actions to another php script. We'll call it dataViewer.php Now, if your two other pages are designed to process form data pulled directly from POST or GET, dataViewer.php would just look like: <?php ## display the data include('data_display.php'); ### display chart include('chart_display.php'); ?> otherwise, you will have to put the code in that formats the form data to the form needed by the scripts before the includes. in this case cURL would be sort of a waste. when you include a file, you basically take everything that is in it and dump in place of the include. So that script, as it is written will execute (in order) data_display.php then chart_display.php as if you had copied the code out of both files and pasted it into one. -
[SOLVED] Submit button calling two php scripts???
mbeals replied to budimir's topic in PHP Coding Help
Just write a page to get and pre-process (if needed) the form data, then include the two other pages with include(); -
echo your queries
-
assuming that structure (which I think is correct looking back at your original code, this should do it: <?php foreach($_SESSION['order'] as $key=>$value){ foreach($itemsRows as $row){ if($row['itemId'] != $key){ echo "<input type=\"hidden\" name=\"$key\" value=\"$value\" /><br>"; break; } } } ?>
-
is $itemRows an array of associative arrays? Like: array ( [0] = array( 'itemID' => ' ' , 'itemName' => ' ', .....) [1] = array( 'itemID' => ' ' , 'itemName' => ' ', .....) [2] = array( 'itemID' => ' ' , 'itemName' => ' ', .....) )
-
Sorry, Can you give me an example of the $_SESSION and $itemRows arrays? I just want to see their structure.
-
the file should be uploaded with correct permissions. The issue is probably the folder itself. Go check the destination folder and make sure it is owned by the web server's user and group and make sure it has user read/write privileges.
-
actually, a valid query that returns 0 rows will cause mysql_fetch_assoc to return 0, so you can just do: <?php $club_id = array('CLASS1' => 1, 'CLASS2' => 2, 'CLASS3' => 3); $sql = "SELECT activity FROM Activities WHERE booking_id = " . $id . " AND activity IN ('class1', 'class2', 'class3')"; $result = mysql_query($sql) or die(mysql_error()); while ($row = mysql_fetch_assoc($result)) { $sql = "INSERT INTO Activity_Review (booking_id, club_id) VALUES (" . $id . ", " . $club_id[$row['activity']] . ")"; mysql_query($sql) or die(mysql_error()); } $sql = "INSERT INTO Activity_Response (booking_id) VALUES (" . $id . ")"; mysql_query($sql) or die(mysql_error()); ?> You also had some extra end braces that I cleaned up. Not sure if they were part of a bigger code block or if you meant them to be there...but for this specific block, they shouldn't be. That code will generate and execute an sql statement for each row returned by the top query. If you are still having issues, double check the first sql statement is producing the results you are expecting and then throw an 'echo "$sql<br>"; into the while loop to take a look at what sql statements are being executed. 1. verify the results and number of results returned by the first query 2. verify you don't have any primary key conflicts (although that should pop an error)
-
awesome, thanks
-
Thanks, that will work, but ideally I need it as: Array ( [0] => Array ( [0] => 12345 [1] => R0012345678 [2] => S0012345678 [3] => 1Z52x3a1234567 ) [1] => Array ( [0] => 3456 [1] => R0012345622 [2] => S0012334522 ) ) or even just ( [0] => Array ( [0] => 12345 R0012345678 S0012345678 1Z52x3a1234567 ) [1] => Array ( [0] => 3456 R0012345622 S0012334522 ) ) as I need to recursively process each chunk, but I could make the preg split option work.
-
I have a block of text from a post variable that I need to break apart. Each 'block' will always start with a string that is nothing but numbers and it will be followed by any number of other strings adhering to 1 of 3 types. Examples work better so here's some sample data: 12345 R0012345678 S0012345678 1Z52x3a1234567 3456 R0012345622 S0012334522 Needs to broken into 12345 R0012345678 S0012345678 1Z52x3a1234567 ------------------------ 3456 R0012345622 S0012334522 I have regex that will identify each 'type' independently but I can't get a master string to work. this is what I have: <?php $wo = '\b\d+\b'; $rnum = '\b[Rr]\d+\b'; $snum = '\b[ss]\d+\b'; $track = '\b1[zZ]52[xX][\w\d]+\b'; $regex = "/($wo)[$rnum|$snum|$track]+/"; preg_match_all($regex,$text,$matches); print_r($matches); ?> It just needs to match a $wo, then any number of things matching $rnum, $snum, or $track.
-
you could seriously simplify your code with simple array functions which will get rid of some of the nesting and make the code easier to read: ie: <?php foreach ($_SESSION['order'] as $key => $value) { $found = false; foreach ($itemsRows as $itemsRow) { if ($itemsRow['itemId'] == $key) { $found = true; break; } } if (!$found) { echo "<input type=\"hidden\" name=\"$key\" value=\"$value\" />"; } } ?> becomes <?php foreach ($itemsRows as $itemsRow) { if (array_key_exists ($itemsRow['itemId'], $_SESSION['order'])) { echo "<input type=\"hidden\" name=\"$key\" value=\"$value\" />"; } } ?> peruse this list http://us2.php.net/array other then that, it's hard to help without more info on exactly what is breaking
-
It works on my end too. PHP5, ubuntu 7.05 mbeals@LAMPserver:~$ telnet 192.168.3.198 1337 Trying 192.168.3.198... Connected to 192.168.3.198. Escape character is '^]'. test null Connection closed by foreign host. mbeals@LAMPserver:/home/webroot/www$ sudo php -q socketTest.php Checking options... OK Creating Socket... OK Binding socket... OK; Bound to port 1337@192.168.3.198 Listening for connections... OK New connection accepted! Reading sent data... OK Sending data to client... OK you might also want to look into forking the process when a new connection is made.
-
you are missing the double $$. Also do only one or the other. <?php while ($row = mysql_fetch_array ($result, MYSQL_NUM)) { $varname = "vars$i"; //will create a variable name: var1, var2, var3.....varn $i++; $$varname = $row[0]; } ?> echo "$var1 $var2 $var3"; When this code is run the first time, $varname takes the value 'var1'. So when you call $$varname, php translates that to '$var1'. That way you create a new variable every time the loop repeats. The other option, which is more tidy and easier to work with is to use an array: <?php while ($row = mysql_fetch_array ($result, MYSQL_NUM)) { $var[] = $row[0]; } ?> print_r($var); This will produce a single array containing all the values for that particular column. This is a better option, depending on how you pass the variables into flash.
-
what is your naming scheme? I would dynamically create the name for the variable within the while loop. <?php $i=1; while ($row = mysql_fetch_array ($result, MYSQL_NUM)) { $varname = "var$i"; //will create a variable name: var1, var2, var3.....varn $i++; $$varname = $row[0]; ####### ##or use an array if you can $vars[] = $row[0]; } ?>
-
where are you applying the hash? perform your validation on the submitted password before hashing: <?php $pass = $_POST['pass']; if($pass) $hash = md5($pass); ?> Now...I wouldn't do it exactly like that. I'd write a function that does more validation first: <?php function validate($pass){ if(!$pass) return 0; if(!preg_match("some regex", $pass) return 0; ## more conditions return md5($pass); } if($hash = validate($_POST['pass'])){ #do something with the valid hashed password } ?>