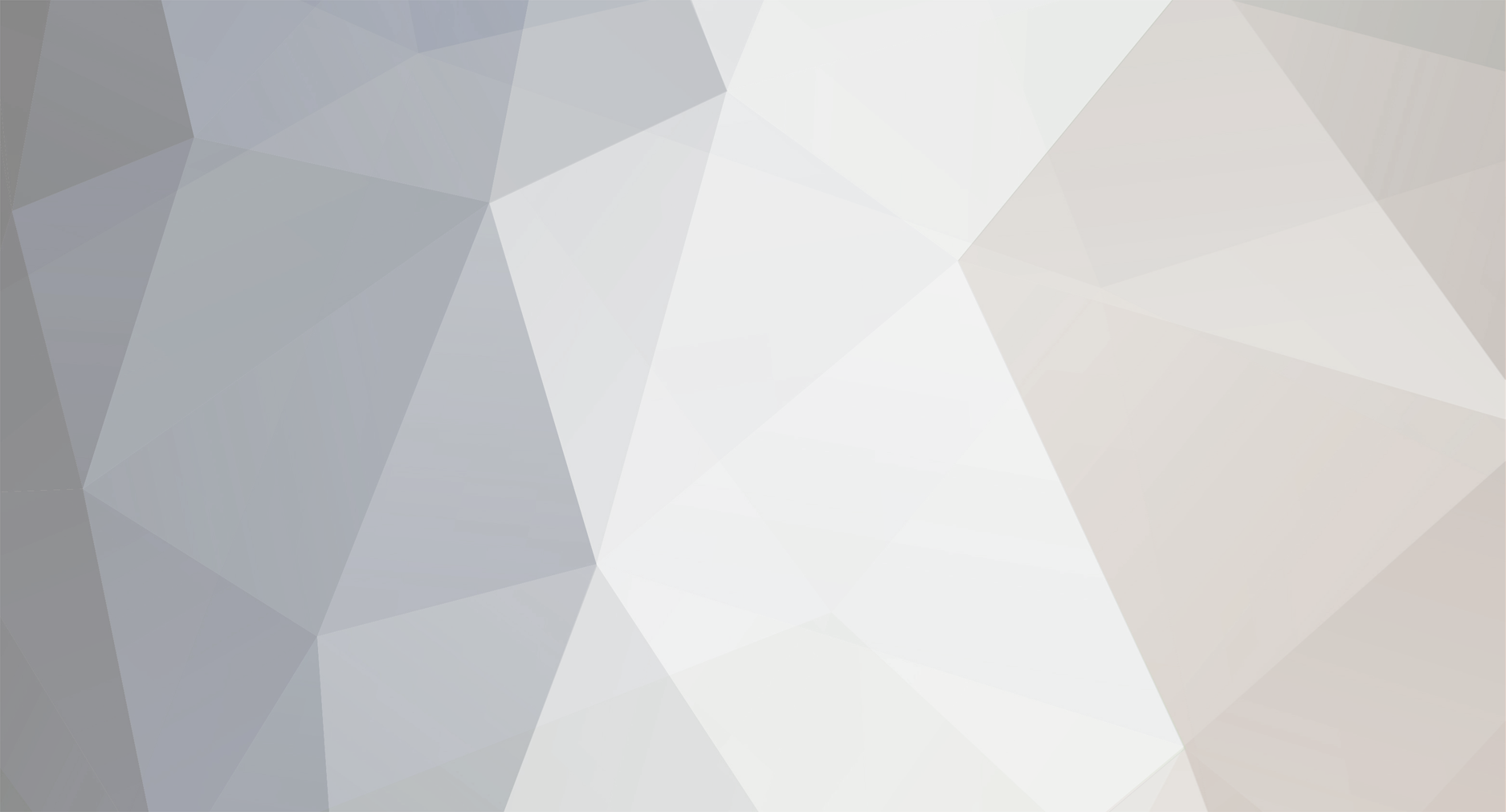
mbeals
Members-
Posts
247 -
Joined
-
Last visited
Never
Everything posted by mbeals
-
let me guess, php5 on a windows machine? the mysql libraries are not included with php5. You need to manually install them. I was actually fighting this last night and couldn't find a resolution (although I didn't try that long) so hopefully someone will give a solution.
-
damianjames is sort of right. fopen will work with the html wrapper if allow_url_fopen is turned on. see here: http://us.php.net/manual/en/filesystem.configuration.php#ini.allow-url-fopen Although this isn't usually a good thing to turn on unless you are sure you really want to. The first error could be caused by lots of stuff. The file doesn't exist...the file lacks proper permissions... Looking at some actual code would help a lot.
-
what is the server environment? easiest thing to do would be to write a script to sync the files that you trigger after an upload with either system(), exec(), or backticks, depending on what level of interaction verification you need. if both hosts are linux, look into rsync. Otherwise ftp is your best bet. If it's not critical the servers are in real time sync, I'd just rsync each dir with a cron job. even if you need it real time and do the transfer on a per-transaction basis, i would still have a cron job that runs every night or so to make sure the dirs are in sync. if the directory you are using to hold the images is locked down, and you take sufficient precautions, I don't see this being a security risk at all.
-
[SOLVED] HTML tables don't show up in e-mail message
mbeals replied to miinet's topic in PHP Coding Help
try changing rn to \r\n. IE: $headers = "MIME-Version: 1.0\r\n"; $headers .= "Content-type: text/html\r\n"; $headers .= "charset=iso-8859-1\r\n"; //probably should add a 'from' header too $headers = "From: $from\r\n"; [\code] -
that's kind of ugly and convoluted though... I also like to try and avoid that (except for handling simple form requests), for visibility while debugging reasons. i could also do: if(mysql_error()) return 0; but I was hoping for an elegantly simple one-liner oh well
-
what are the syntax and limitations for the 'or' function? I can't find it in the manual (try searching for "or" and see how many random sites you get). I'm familiar with using it in mysql queries, like: mysql_query or die(mysql_error()); but would like to use it more like you can in perl.... <?php function test($foo = NULL){ $var = $foo or return 0; return $var; } if($val = test(4)) echo $val; ?> or more usefull: <?php function query($query){ $result = mysql_query($query) or return 0; while($row = mysql_fetch_assoc($result)){ $data[] = $row; } return $data; } ?> but alas, php pukes. I could use standard conditionals with mysql_errno but why use 3 lines of code when you can do it in 1? So... can or be manipulated to function like that or is that just something php won't do? Any other perl-like shortcuts in php?
-
Please help me to create simple if and else php script
mbeals replied to linux1880's topic in PHP Coding Help
that block of code as to be the VERY first thing executed. So before anything else on the page (even whitespace), put that block -
#1 in a class statement you have to initialize attributes with visibility, otherwise php doesn't know what is allowed to access it. Once you get the hang of it, you will start to see the importance. #2 Variable scope in a function is the same whether you are writing a function i OOP or in procedural. In a function block, all variables are local to that function. IE: <?php $foo = 1; function printFoo(){ echo $foo; } ?> will not output anything in any form of php, as $foo is not defined in the function's scope. This also means you can declare variables in method definitions without worrying about overriding : <?php class newClass{ private $foo = 'test'; function printFoo(){ $foo = 1; $bar = 2; echo $foo."\n"; echo $this->foo."\n"; echo $bar."\n"; } } ?> So calling the printFoo() method would print: 1 test 2 The reference to $foo in the first echo statement was inside the scope of the method. You have to use the $this variable to access the scope of the class. Sort of like (but not really) using global in a procedural function.
-
make each tag type it's own class. so for an input tag you could use this: <?php class input { private $options = array(); function __construct($name = NULL, $type = NULL){ if($type) $name = array('name' => $name, 'type' => $type); if(is_array($name)) $this->fill($name); } function fill($stuff){ foreach($stuff as $option=>$value) $this->options[$option] = $value; } function setOption($option,$value){ $this->options[$option] = $value; } ////insert more add/modify methods here function getHTML(){ $html = '<input'; foreach($this->options as $option=>$value) $html .= " $option='$value'"; $html .= ">"; return $html; } function printHTML(){ echo $this->getHTML(); } } ?> This class keeps all options (everything in the form of something="value") in one array. This is just a prototype... I wrote it off the top of my head and rather quickly, so it could stand for some cleaning up. When you create the object, you can either just define the name and type of the input like: $foo = new input('name', 'type'); or pass an array of options to it: $options = array('name' => 'bar', 'type' =>'text', 'class' => 'someclass'); $foo = new input($options); Passing no arguments will just create an empty object. Additional options are set and unset with fill() in array form, or by setOption() like: $foo->setOption('options', 'value'); getHTML() returns the html string wile printHTML() echos it out I have a select class similar to this that is exceedingly useful. I event went so far as to write a tag class that will build any html tag given type, options (within the opening tag) and the contents (between the tags). If you go that route, the above code would simplify even more.
-
[SOLVED] initialize class attribute as an array;
mbeals replied to mbeals's topic in PHP Coding Help
your right. I had this: foreach($this as $key => $value) $this->$key = $row[$key]; in my constructor to populate the object variable from a database. It was resetting every attribute that didn't have an entry in the database to NULL. Changed it to: foreach($row as $key => $value) $this->$key = $value; and all is well. Thanks -
In my classes I tend to define an attribute as an array to hold children objects created by the parent object. I figured declaring the attribute as: protected $attribute = array(); would be enough but it's not. When I initialize the attribute as an array, it doesn't become an array until I put stuff in it. I checked this by echoing gettype() of the attribute before and after filling it. Just after initialization, the attribute has type NULL. I'm guessing this is to save memory, but it's really inconvenient. If I run a foreach on this attribute, I need it to see it as an array with 0 members, so it will just bypass it instead of popping a warning. is there a way around this besides if($this->attribute) foreach() ?
-
first off change: $valid = @fsockopen("$url", 80, $errno, $errstr, 10); to $valid = fsockopen("$url", 80, $errno, $errstr, 10); and put: echo "Error $errno: $errstr"; at the end.
-
thanks for the shortcut, but I was mostly asking about the performance aspects. Given the two options (persisting the object or repopulating), which is 'faster'. In the absence of database queries, is it more costly to rebuild an object or just pull the object out of the session variable?
-
I've figured out that you can persist an object with sessions by serializing the object to a session variable on page close and then checking for and unserializing it on page load. Very cool stuff, but I'm wondering about the benifits. Take this example: I have an object that builds an html form. It has two text boxes and a select box to change the type of input box (password, hidden or text). When you change the type, it reloads the page to reflect the change. Here's the class structure. Ignore the select class. It's not really part of the example, I'm just too lazy to write a dynamic select now that I wrote that class. <?php class Form{ protected $box1; protected $box2; protected $type; protected $formAction; function __construct($stuff = NULL){ if($stuff) $this->fill($stuff); } function fill($stuff){ foreach($stuff as $key => $value){ if(array_key_exists($key,get_object_vars($this))) $this->$key = $value; } } function updateFromPost(){ $this->fill($_POST); if($_POST['Submit']) echo "Box1 = {$this->box1} Box2 = {$this->box2}<br>"; } function getHTML(){ $sel = new select; $options = array('password' => 'password', 'text' => 'text', 'hidden' => 'hidden'); $sel->addOption($options); $sel->name('type'); $sel->setSelected($this->type); $sel->setArgs(array("onchange" => "this.form.submit();")); $html = "<form action='{$this->formAction}' method='POST'>\n"; $html .= $sel->getHTML(); $html .= "<input name='box1' type='{$this->type}' value='{$this->box1}'/>\n"; $html .= "<input name='box2' type='{$this->type}' value='{$this->box2}'/>\n"; $html .= "<input name='Submit' type='submit' value='submit'>"; $html .= '</form>'; return $html; } function printHTML(){ echo $this->getHTML(); } } ############################ class select { protected $options; protected $selected; protected $name; protected $args = array(); function __construct($stuff = NULL){ if($stuff){ foreach($stuff as $option){ $this->addOption($option); } } } function addOption($option, $value = NULL){ if(is_array($option)){ $this->options = $option; }else{ $this->options[$option] = $value; } } function setArgs($option, $value = NULL){ if(is_array($option)){ $this->args = $option; }else{ $this->args[$option] = $value; } } function setSelected($val){ $this->selected = $val; } function name($val){ $this->name = $val; } function getHTML(){ $html = "<select name={$this->name}"; foreach($this->args as $option=>$value) $html .= " $option='$value'"; $html .= ">\n"; foreach($this->options as $option=>$value){ $html .= "\t<option value='$value' "; if($this->selected == $value) $html .= 'selected="selected"'; $html .= " >$option</option>\n"; } $html .= "</select>\n"; return $html; } function printHTML(){ echo $this->getHTML(); } } ?> Now the two ways to do it. Option one is to serialize the object into a session variable: <?php session_start(); if($_SESSION['object']){ $test = unserialize($_SESSION['object']); $test->updateFromPost(); $_SESSION['object'] = serialize($test); }else{ $test = new Form; $data = array('box1' => 'test1', 'box2' => 'test2', 'type' => 'text'); $_SESSION['object'] = serialize($test); $test->fill($data); } $test->printHTML(); ?> If the object is cached in the session variable, it is reinstated and update with new form information, then recached. If this is the first time creating the object (no cache), a new instance is created and cached. Option 2: just create a new object every time <?php if($_POST){ $test = new Form; $test->updateFromPost(); }else{ $test = new Form; $data = array('box1' => 'test1', 'box2' => 'test2', 'type' => 'text'); $_SESSION['object'] = serialize($test); $test->fill($data); } $test->printHTML(); ?> This does the same thing as option 1, but relies on POST to contain all the attributes of the old object, which in this case works. Now it seems to me that option 1 would be a lot more flexible, as I'm maintaining the structure of my data from page to page and don't have to worry about reconstructing stuff from the post variable. It's also very clean and scalable. Since the only thing that is really going back to the server is the POST variable, bandwidth usage should be about the same. However, is there a performance hit for caching an object in a session variable? I can't see it happening on an object this small, but what about for larger ones? Is there any reason not to do this that I'm not seeing, because I really like the potential.
-
so you have a stats class which has the data you wish to graph, and you want to be able to make many graphs from the data contained within stats? So you want to make the graph class an child of the stats class? extends lets you share common methods and attributes for the same instance. I would create a stats class (sounds like you have it already) and define a public $graphs = array(); attribute. Then create a graph Rendering class. If this class needs to be unique to the datatype, use the extends here. This might also be a good place for an abstract class. Now in the stats class, add methods to create new graph objects and assign their reference to the $graphs array. If you need different methods to handle the processing of the different stat types, then extend stats.
-
sorry, I was attempting to tab over while writing code (which doesn't work in this box) and submitted the topic before it was complete. I was going to ask if you have an object which is a child of another (or even of an abstract), is it possible to change the child objects class, retaining the abstracted attributes. Effectively taking an object of one class, destroying it, then creating a new instance of another class (with the same parent) and persisting the common attributes. ie: <?php class parent{ protected $id; protected $info; function changeClass($newClass){ } } class child1 extends parent{ function __construct(){ } } class child2 extends parent{ } $test = new child1; $test->changeClass('child2'); ?> where any attributes defined through child one that are common with child2 would persisit. Could I just do something like: <?php function ChangeClass($newClass){ unset($this); return new($newClass); } ?>
-
Is it possible to change the class of an object if it retains the same parent? IE <?php class device {
-
Using firefox, the web developer extension and my server I was able to verify this is a BAD idea. anyone can set their session variable to a relative path (../../../) and attempt to make a dir and upload a file anywhere on the file system. Hopefully you have your www-data user well restricted. What are the permissions on your website files as well? Think about what would happen with the previously posted code if www-data had write privileges and someone set their session variable to "." and uploaded 'index.html'.
-
word of caution... Instead of checking on the fly to see if the folder exists, I would create the folder when the user first signs up. I would also pull the path to the folder from the db or sanitize and check it really well. Session variables can be changed and if you just check and create a new folder based on a session variable, you are asking for problems.
-
could also just do <?php $rand = (range(1, 200); shuffle($rand); ?>
-
This is my first attempt at OOP programming. It's 3 classes that build and HTML table. My goal was to create something that would let me take an array of data and quickly build a table, yet retain enough granularity to format individual rows and cells. Like I said, it's my first attempt at OOP, so I'm sure there are things that aren't Kosher, and I would appreciate some feedback on those ways to improve and streamline the code. Thought about putting this in the beta testing forum, but it seemed more fitting here. here's the class file: <?php class Cell { /**** Class Cell **************** This class defines the cell of an html table. The constructor and fill methods accept either a single string value, which will become the contents of the cell or an associative array, setting: alignment : alignment background color : color width : width contents : contents formatting tags : format if it's a header cell : headerCell */ private $alignment; private $color; private $width; private $contents; private $format; private $headerCell; private $colspan; function __construct($stuff = NULL) { $this->fill($stuff); } function fill($stuff){ if(is_array($stuff)){ $this->contents = $stuff['contents']; $this->color = $stuff['color']; $this->alignment = $stuff['alignment']; $this->width = $stuff['width']; $this->format = $stuff['format']; }else{ $this->contents = $stuff; } } function setFormat($var){ $this->format = $var; } function isHeader($bool){ $this->headerCell = $bool; } function bgColor($bgcolor) { $this->color = $bgcolor; } function textAlign($align) { $this->alignment = $align; } function setWidth($val){ $this->width = $val; } function setColSpan($val){ $this->colspan = $val; } function appendCell($stuff) { $this->contents .= $stuff; } function clear(){ $this->contents = ''; $this->color = ''; } function getColor() { return $this->color; } function getAlign() { return $this->alignment; } function getWidth(){ return $this->width; } function getContents(){ return $this->contents; } function getHeader(){ return $this->headerCell; } function getAll(){ $info['color'] = $this->getColor(); $info['alignment'] = $this->getAlign(); $info['width'] = $this->getWidth(); $info['contents'] = $this->getContents(); $info['header'] = $this->getHeader(); return $info; } function getHTML(){ if($this->headerCell){ $html = "\t<th "; }else{ $html = "\t<td ";} if($this->width) $html .= 'width="'.$this->width.'" '; if($this->color) $html .= 'bgcolor="'.$this->color.'" '; if($this->colspan) $html .= 'colspan="'.$this->colspan.'" '; if($this->alignment) $html .= 'align="'.$this->alignment.'" '; $html .= '>'; if($this->format) $html .= '<'.$this->format.'> '; $html .= $this->contents; if($this->format) $html .= '</'.$this->format.'> '; if($this->headerCell){ $html .= "</th>\n";}else{ $html .= "</td>\n";} return $html; } function printHTML(){ echo $this->getHTML(); } } ########################################################################################## class Row { /***** Class Row ************************* The Row Class builds html rows and contains methods for interacting with the Cell class. The constructor and fill methods create an array of cell objects which represent the row. These methods will accept an array of cells, where each item is either a previously declaired cell object or the standard input to the cell constructor (see class cell). This class also contains methods for updating cells (contained in $this->cells en-mass, allowing cell level formatting to be applied to an entire row's worth of cells at once. Common css tags, such as id and class can also be assigned to the row by setting the appropriate attributes. */ public $cells; private $alignment; private $valign; private $id; private $class; private $cellSpace; private $headerRow; private $color; function __construct($stuff = NULL) { if($stuff) $this->fill($stuff); } function fill($stuff) { $this->cells = array(); $this->addCells($stuff); return $this->cells; } function addCells($stuff) { foreach($stuff as $cell){ if(is_object($cell)){ $this->cells[] = $cell; }else{ $this->cells[] = new Cell($cell); } } return $this->cells; } function emptyRow() { foreach($this->cells as $cell) $cell->clear(); } function setValign($align){ $this->valign = $align; } function setId($val){ $this->id = $val; } function setClass($val){ $this->class = $val; } function setHeader($bool){ $this->headerRow = $bool; $this->setHeaderDO(); } function setHeaderDO(){ foreach($this->cells as $cell) $cell->isHeader($this->headerRow); } function setSpacing($space){ $this->cellSpace = $space; $this->setSpacingDO(); } function setSpacingDO(){ if(count($this->cells) == count($this->cellSpace)){ for($i=0;$i<count($this->cells);$i++) $this->cells[$i]->setWidth($this->cellSpace[$i]); } } function setColor($color){ $this->color = $color; $this->setColorDO(); } function setColorDO(){ foreach($this->cells as $cell) $cell->bgColor($this->color); } function setAlignment($align){ $this->alignment = $align; $this->setAlignmentDO(); } function setAlignmentDO(){ foreach($this->cells as $cell) $cell->textAlign($this->alignment); } function getHTML(){ $html = '<tr '; if($this->valign) $html .= 'valign="'.$this->valign.'" '; if($this->id) $html .= 'id="'.$this->id.'" '; if($this->class) $html .= 'class="'.$this->class.'" '; $html .= "> \n"; foreach($this->cells as $cell) $html .= $cell->getHTML(); $html .= "</tr>\n"; return $html; } function printHTML(){ echo $this->getHTML(); } } ########################################################################################################### class Table { /****** Class Table *********** This class builds an HTML table by forming an array of rows (defined by the row class) Rows can be added individually or in bulk through the insertRow and insertMRows methods respectively. Both methods will accept either an array (as defined in class Row) or a predefined row object, with the insertMRows method accepting an array of said items. Table attributes are not strict and can be assigned through the setAttribute($attribute, $value) and setAllAttributes($attribute) methods. The setAttribute method accepts two arguments: the attribute (tag) to set and it's value, while the setAllAttributes method accepts an associative array where the key is the attribute (tag) and the value is the value Zebra Striping can also be performed with the stripe() method which takes the color of the stripe as an argument. */ public $rows; private $cellSpacing; private $Attributes; private $zebra; function __construct($stuff = NULL) { if($stuff)insertMRows($stuff); } function insertMRows($stuff) { foreach($stuff as $row){ if(is_object($row)) $this->rows[] = $row; if(is_array($row)) $this->rows[] = new Row($row); } return $this->rows; } function insertRow($row) { if(is_array($row)){ $newRow = new Row($row); $this->rows[] = $newRow; return $newRow; } if(is_object($row)) $this->rows[] = $row; return $row; } function stripe($color){ $this->zebra = $color; $this->stripeDo(); } function stripeDo(){ $i = 0; if($this->rows){ foreach($this->rows as $row){ if($i%2) $row->setColor($this->zebra); $i++; } } } function setAllAttributes($stuff) { $this->Attributes = array(); foreach($stuff as $att => $value) $this->Attributes[$att] = $value; } function setAttribute($att, $value) { $this->Attributes[$att] = $value; } function setSpacing($spaceArr){ $this->cellSpacing = $spaceArr; $this->setSpacingDo(); } function setSpacingDo(){ foreach($this->rows as $row) $row->setSpacing(''); $this->rows[0]->setSpacing($this->cellSpacing); } function getHTML(){ $html = '<table '; if($this->Attributes){ foreach($this->Attributes as $attribute => $value) $html .= $attribute.'="'.$value.'" '; } $html .= ">\n"; if($this->rows) foreach($this->rows as $row) $html .= "\n".$row->getHTML(); $html .="</table>"; return $html; } function printHTML(){ echo $this->getHTML(); } } ################################################################################################################# ?> And here is a demo of how to use it <?php include 'TableClass.php'; $Table = new Table; ### build table all at once ###### $stuff = array( array(0,1,2,3), array(1,2,3,4), array(2,2,3,4), array(3,2,3,4), array(4,2,3,4), array(5,2,3,4)); $Table->insertMRows($stuff); #### Set a bunch of attributes ############ $attributes = array( 'width' => '80%', 'align' => 'center'); $Table->setAllAttributes( $attributes); #### Set a Single Attribute ############# $Table->setAttribute('border', 1); #### Zebra Stripe the Table ########## $Table->stripe('yellow'); ###Set the Table spacing (old school) ##### $Table->setSpacing(array(10,10,10,60)); #### Add a header Row ############# $Table->rows[0]->setHeader(1); ########## Row functions ########################## ### Add another row $cellA = new Cell('This is a test'); $CellB = new Cell( array('contents' => 'This is also a Test', 'color' => 'blue', 'format' => 'h1', 'alignment' => 'center')); $newRow = new Row(array('blank', $cellA, 'blank', $CellB)); $myRow = $Table->insertRow($newRow); $myRow->setValign('Top'); ### Cell functions $myRow->cells[0]->textAlign('center'); $newCell = new Cell; $newCell-> fill('This is a Spanned Cell'); $newCell-> setColSpan('4'); $Table->insertRow(array($newCell)); $Table->rows[7]->cells[0]->textAlign('center'); $Table->printHTML(); ?>
-
take a look at full text searching. it will do what you want
-
try: UPDATE mail SET msg = '<p>asdfasdfasdf</p>', title = 'frustrated' WHERE title = '3';
-
My database is very hierarchical in nature, which lends itself well to OOP classes. I have a ton of code originally written in functional logic that I'm porting over and it hit me that I'm deconstructing a lot of my more complicated queries into several smaller ones. Is this bad form? Is this going to impact server performance? For example, I have a site that tracks IP assignments. I have a database table that consists of public IP, local IP and a handfull of other info about the address. Then I have one table for each kind of device that can be associated with an address. In functional world, I write this nice long query using Unions and joins to build one array containing IP to device relationships. In OOP world, I have an IP class and different classes for each device. So instead of a single query, I have a method to find all ip addresses and create an IP object for each one. Then the IP class contains methods to search for each kind of device and create an object for each one of those. Each one of those searches is a simple query. So now i have one simple query for every device type (3) per every ip (a whole lot). It doesn't take long before I'm executing a very large number of individual queries as opposed to my single one in functional programming.
-
easiest way to to do it is to install phpmyadmin. It has the option to import a CSV file. So just format your excel doc so that the columns match the database, save as a CSV, then import into phpmyadmin. without phpmyadmin, I'd just write a script to read in the CSV file line by line and generate an Insert statement for each.