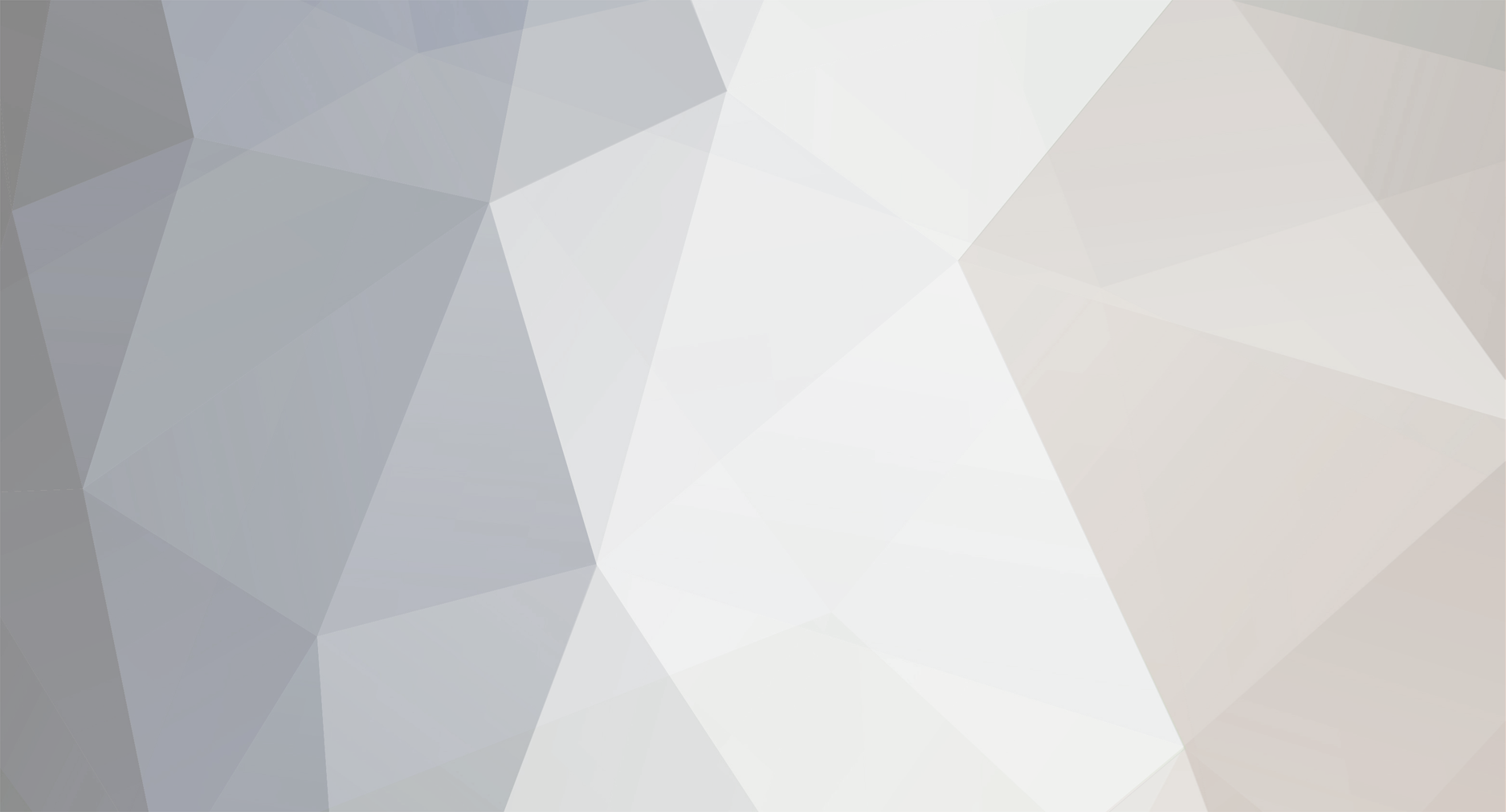
mbeals
Members-
Posts
247 -
Joined
-
Last visited
Never
Everything posted by mbeals
-
sorry, didn't think about this being console output, so ditch the br. The line break isn't working because you are using /n instead of \n. copy and paste this: echo "$file already exists\n" ;
-
the original syntax is fine. The query is inside double quotes so the variable should be parsed. i would still put echo "SELECT * FROM links WHERE `id` = '$id'"; on the line just under the $result = line to make sure the query is being populated correctly. This was working fine before the upgrade?
-
then after the while loop ends put the same thing that is in the if statement <?php while($row = mysql_fetch_array(){ do a bunch of stuff } if($col != 1){ echo str_repeat('<td> </td>',4-$col).'</tr>'; $col = 1; } ?> </table>
-
but wget will do it over ftp too for the echo question, did you try echo "$file already exists\n<br />" ;
-
try playing around with str_repeat in the first if statement. <?php if ($strCurLetter!=$strLastLetter) { if($col != 1){ echo str_repeat('<td> </td>',4-$col).'</tr>'; $col = 1; } echo '<tr><td style="height:25px;" colspan="'.$colMax.'">'."\n"; echo '<strong>'.$strCurLetter.'</strong>'."\n"; echo '<a name="'.strtoupper($strCurLetter).'"></a> <a href="#top"><small>Back To Top</small></a><hr />'."\n"; echo '</td></tr>'."\n"; } ?> so if the next letter is reached (triggering the if statement), the previous row would still be have an unclosed <tr> tag. So we figure out how many more cells we need to add to make it 3 (4-$col), echo them out and the closing <tr> tag.
-
since you are using cron, you must be on linux. why not use wget with the --mirror option instead? or better yet, if you have ssh access just use rsync. Both would literally turn all of that code into a single line in your crontab
-
try moving the $col++ instead of if ($col == 1) {echo " <tr>\n"; } echo '<td><a class="smallG" href="record.php?ID='.$row["CompanyID"].'">'.$row["CompanyName"].'</a></td>'."\n"; $col++; if ($col == $colMax) { echo " </tr>\n"; $col = 1; } do if ($col == 1) {echo " <tr>\n"; } echo '<td><a class="smallG" href="record.php?ID='.$row["CompanyID"].'">'.$row["CompanyName"].'</a></td>'."\n"; if ($col == $colMax) { echo " </tr>\n"; $col = 1; }else { $col++; } when you hit column 2, you are applying ++ (making it == 3), then testing to see if it is equal to colMax... which it is, so it closes the <tr>. You need to test the column number, then increment it. You need to encase it in the else statement so that when $col is reset to 1, $col++ doesn't bump it up to 2.
-
give us some sample rows from the database and the desired final output
-
look into preg_match_all(); it will let you run regular expression searches. you can get as crazy as you want. for example preg_match_all("/<(\w).*?>.*<\/\w>/",$text,$matches); will search the text and return an array of 2 arrays. The first will be everything enclosed in < > tags and the second corresponds to the 'type' of tag it is (p,ul,a, etc). depending on how you formulate the regex, you can pull out whatever you want.
-
so you use preg_match to get the parts, build a real hyperlink from them, then use a normal str_replace to replace the entire string with the new one.
-
i would use preg_match to match the entire thing and pick it apart. Something like $url = '[url=http://www.phpfreaks.com/forums/index.php?action=post;topic=205723.0;num_replies=0]Link Title[url]'; preg_match("/\[url=(.*?)](.*?)\[url\]/",$url,$matches); print_r($matches); gives Array ( [0] => [url=http://www.phpfreaks.com/forums/index.php?action=post;topic=205723.0;num_replies=0]Link Title[url] [1] => http://www.phpfreaks.com/forums/index.php?action=post;topic=205723.0;num_replies=0 [2] => Link Title )
-
okay, lets just keep it simple for now. <?php #### Set up email ############## $to = "*myemail*"; $subject = "Custom Order Request"; $email = $_REQUEST['email'] ; $headers = "From: $email"; ### Get results from form ############ ## change these to reflect the names of the entries in ## your form. $name = $_REQUEST['name']; $phone= $_REQUEST['phone']; $product = $_REQUEST['product']; $wood = $_REQUEST['wood']; $date = $_REQUEST['date']; ##### Build the body of the email #### $body = "Name: $name\n Phone: $phone\n Product: $product\n Wood: $wood\n Date: $date"; $sent = mail($to,$subject,$body,$headers); if($sent) {print "Your mail was sent successfully"; } else {print "We encountered an error sending your mail"; } ?> make sure you change $name = $_REQUEST['name']; $phone= $_REQUEST['phone']; $product = $_REQUEST['product']; $wood = $_REQUEST['wood']; $date = $_REQUEST['date']; to match the names of the fields in your form. For that example, my form would have 5 fields, named name, phone, product, wood and date. If yours are named differently, then change the name in the $_REQUEST[' '] part.
-
if you just want to get rid of the html tags, use strip_tags() it will strip out all tags. If you want to allow some, just pass a string of allowed tags as the second arg. ie <?php $string = "<h1>Hello <strong>World</strong></h1>"; echo strip_tags($string); // echos "Hello World"; echo strip_tags($string, '<strong>') // echos "Hello <strong>World</strong>" ?>
-
that is true, but your code isn't sending any email... it's not even trying. I'm assuming you are pulling in the values in your $fields array from the previous form? try something like this: <?php #### Set up email ############## $to = "*myemail*"; $subject = "Custom Order Request"; $email = $_REQUEST['email'] ; $headers = "From: $email"; ### Get results from form ############ ## change these to reflect the names of the entries in ## your form. $fields['name'] = $_REQUEST['name']; $fields['phone'] = $_REQUEST['phone']; $fields['product'] = $_REQUEST['product']; $fields['wood'] = $_REQUEST['wood']; $fields['date'] = $_REQUEST['date']; ##### Build the body of the email #### foreach($fields as $key => $age){ $body .= "$key : $age\n"; } $sent = mail($to,$subject,$body,$headers); if($sent) {print "Your mail was sent successfully"; } else {print "We encountered an error sending your mail"; } ?> Before anyone jumps on me....yes there is much that can be optimized, but I didn't for the sake of clarity. So lets look at what this is doing.... Your first part is okay and you build the headers fine. Next you have to get the data from the form. This is in the form of an array which you are accessing with $_REQUEST. You could also access this array with $_POST or $_GET depending on what method you set in the form tag....anyway. The array from the form is an associative array, meaning that the key for an entry is the name of the form object and the value is the value. IE a text box named 'name' that is submitted with the value "Ted" will give $_REQUEST['name'] = 'Ted'. Make sense? So we pull those values out and put them in the new $fields array. Then to build the body, we loop through the array with foreach and create a string containing each key->value pair. We need to save the data to a string instead of echoing it. Echoing it just displays it on the screen. The .= operator means take the current value and add this new stuff to it. So every time the loop loops, the new value is just tacked on to the end. We use the \n so that the new value is started on a new line. The final part is actually sending the mail with the mail() command.
-
so you have a table with a single attribute containing the html? or is the html distributed through several attributes of the same row? If it is from one attribute: Select * from table where `HTML` like '%$tag%'; or Select * from table where `HTML` like REGEXP '$regex; %'s are wild, so option 1 will return all rows with $tag in the attribute HTML. The second option does the same thing as the first but searches the HTML attribute with the regular expression $regex. The other option is to create a full text index. If you are searching across several attributes, then full text is the way to go anyway. Google mysql fulltext and you will find a boatload of info on it.
-
you're right, it is ternary
-
that would do it. You may consider learning tertiary notation. It would clean up those statements and possibly make stuff like that easier to spot. if (!(isset($_POST['Cat'.$x]))){$Cat[$x]='';}else{$Cat[$x]=$_POST['Cat'.$x];} becomes $Cat[$x] = isset($_POST['Cat'.$x]) ? $_POST['Cat'.$x] : ''; syntax is: Variable = test ? val_if_true : val_if_false
-
just before you start to echo the table, do a print_r() on those arrays (outside the for loop). I'm curious to see if they are set and what they are set to
-
the line breaks are put into the database. That's not the issue. What happens is that HTML does parse line breaks (otherwise source formatting would be a pain). What you have to do is convert the line breaks into <br /> tags when you go to display the text. So insert the data like you have been, but on the page that you plan on displaying the text, just use nl2br(). ie: <? $text = mysql_result($result,0,'my_enty'); ?> <div class='my_class'> <?=nl2br($text)?> </div> Make sense?
-
to the processing script, GET and POST are basically the same thing. They are just arrays (with different names). The difference is in the page leading up to the processing script. With a form, you can choose between using post or get in the form 'method' attribute <form action="" method="get"></form> // uses GET array <form action="" method="post"></form> // uses POST array The advantage to GET is that you can set the variables in the URL. This lets you pass dynamic data to the script without using forms at all. The forum is a good example of this. It has a list of topics that simple hyperlinks. When you click on a link, it passes some data to a script that retrieves the messages from the database and prints them to the screen. This is performed without any forms.
-
http://us2.php.net/date lists all the format codes "G:i" or "H:i" instead of "g:i a" will give you 24 hour time
-
that would be the way to go. It will be the most flexible of the options, especially in terms of error handling
-
basically, you can't store a complex data structure, like an array in a database or pass it across a socket, so you have to transform it into something 'flat'. serialize() takes that complex data structure and encodes it into a string that can be decoded by unserialize. To see what it is doing just echo some serialized arrays $array1 = array("1","2","3"); $array2 = array("1"=>"one", "2"=>"two", "3"=>"three"); echo serialize($array1); echo serialize($array2);
-
never mind
-
i've done it both ways. Javascript is the toughest especially if you are pulling data from a database, but AJAX does make it 'easier'. Easiest way is to just add a onchage=this.form.submit() to the first select box, have the form action call the parent page and have code that catches the selected value and populates the second box. Having done it both ways, on a fast connection, I have yet to see a difference in performance between the two methods.