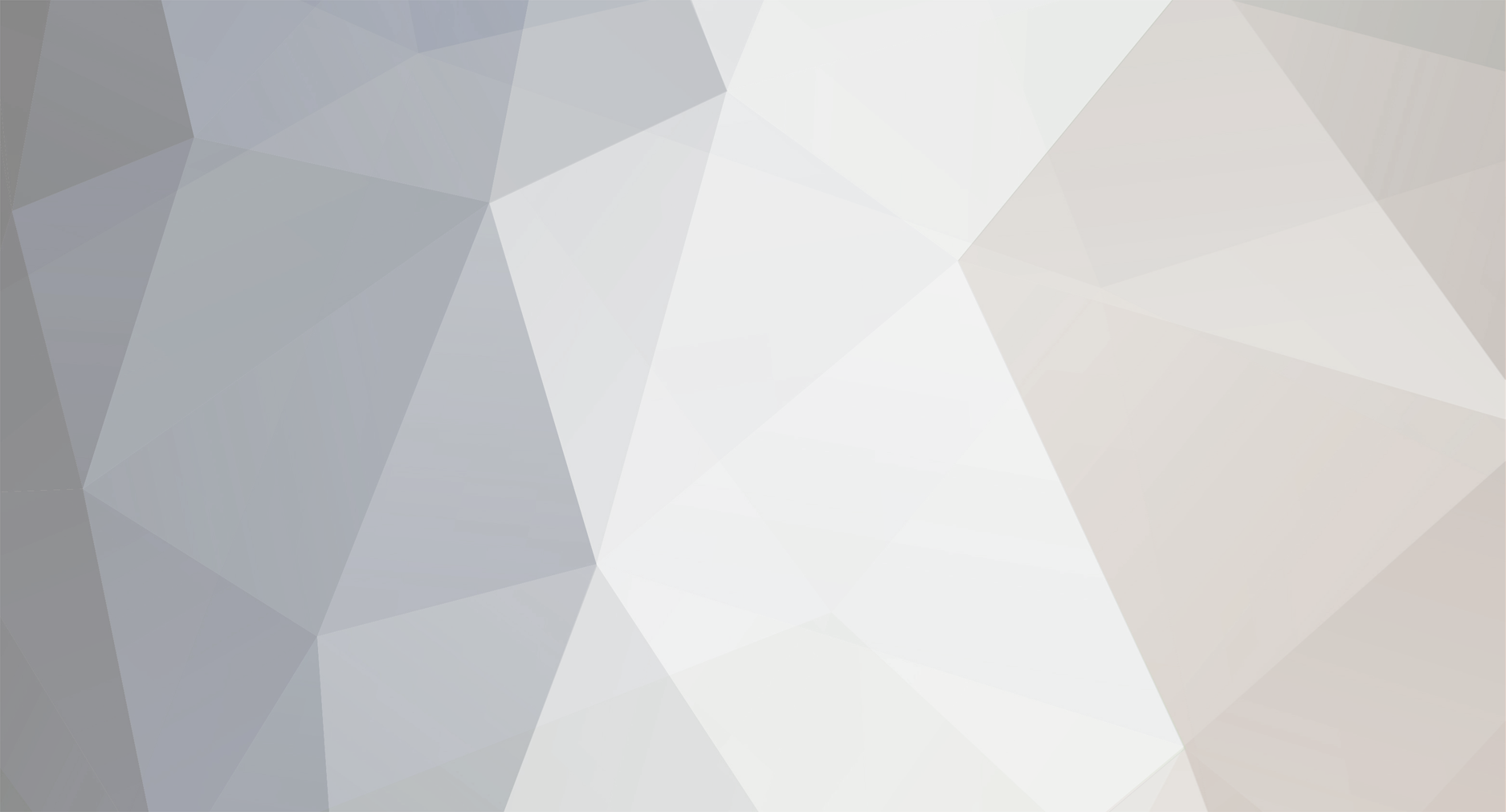
mbeals
Members-
Posts
247 -
Joined
-
Last visited
Never
Everything posted by mbeals
-
what do you get when you type in: crontab -e and what flavor of nix is this?
-
cron is the exact same as you typing the string in the control panel. Cron takes whatever you have there and runs it. go to a terminal and type in: cat /var/log/syslog | grep CRON and hit enter. That will bring up all logged entries for cron. Look for something like: Jul 11 17:20:01 user /USR/SBIN/CRON[24228]: (user) CMD (/usr/bin/php -q /home/public_html/ranking1.php | addts which indicates the script is actually running. better yet, change your crontab to be: /usr/bin/php -q /home/public_html/ranking1.php >> /var/log/ranking1.log let it run for a while and see if log entries are popping up in there.
-
so when you run it from the command line it works fine but no in cron? Remember when you run a script, it is running in your local shell, with your local env variables set. When cron runs a script, it's running under cron's shell and those variables may be different. So make sure that you have all paths explicitly defined. bad: 0 0 * * * php myscript.php better: 0 0 * * * /usr/bin/php -q /home/phpFiles/myscript.php best: 0 0 * * */usr/bin/php -q /home/phpFiles/myscript.php >> /var/log/myscript.log
-
sorry, went to edit the last post to fix the short tags and must of hit quote instead of modify note: you could also use explode instead of preg_split in the last example. Would be faster
-
this is all server side, so unless your webserver is opening the file from a network share, no network traffic will be involved in reading the file. since the file is so large I would go with fgets() instead of file() or fread(). fgets() only reads one line at a time, saving memory. I don't see mysql being an option here as it appears he is parsing server logs of some sort...and there is nothing wrong with playing with flat-files. People were doing this with C and Fortran long before most of you were born. <?php function find_dir($needle, $file = 'test.txt'){ $matches = array(); $needle = '/'.trim($needle); $fh = @fopen($file, "r"); if (!$fh) return 0; while (!feof($fh)) { $line = fgets($handle); if (strpos($line, $needle)){ $out = str_replace($needle, '',$line); $out = str_replace('//', '',$out); $out = explode('/', $out); $matches[] = '<a href="file://'.implode('\\',$out).'">'.$out[count($out)-1].'</a>'; } } fclose($fh); return $matches; } ?> I just cannibalized sasa's code, replacing the array line by line operations with file line by line operations, so don't get mad at me if it doesn't work. It should be pretty close though. with that being said..... if this is linux it may be faster just to backtick grep: <? $file = '/some/file'; $search = "some-term"; $matched_lines = preg_split("/\n/",`cat $file |grep $search`); ?>
-
I'm using php as part of a network provisioning system for cable modems. Without dragging out the details, I control whether a modem can connect (and provide service) with the DHCP host tables... I have been controlling this through hacked together code making system() calls to omshell, but would rather be able to access the objects directly. Unfortunately there isn't much documentation or development in OMAPI, so I've decided to start putting together my own extension to take care of it. So first of all, is anyone aware of an existing project along these lines? I'm fine working with C, and found some decent documentation on the omapi libraries for C (http://linuxreviews.org/man/omapi/), but am not sure where to start in terms of creating the actual extetnsion.
-
funny, my system had no problem with it
-
Fill Text Boxes With Data From mySql Table HeLp pLeAsE...?
mbeals replied to TheStalker's topic in PHP Coding Help
you've got some crazy stuff going on in your html. There is at least one unclosed <td> tag and putting a <form> tag inside a table block is bad juju. <center> <form action="<?php echo $_SERVER['PHP_SELF']?>" method="POST" name="names"> <table border='0' width='300'> <tr> <td><p>CustomerID:</p></td> <td> <SELECT NAME='results' onchange="this.form.submit();"> <?php // Search alternativeDishes $query = "SELECT CustomerID FROM customers"; $result = mysql_query($query); while ($line = mysql_fetch_array($result)) { echo "<OPTION value='$line[0]'>$line[0]</OPTION>"; }?> </SELECT> </td> </tr> <?php if($value=$_POST['results']){ $result = mysql_query("SELECT * FROM customers WHERE CustomerID = '$value'"); $surname = mysql_result($result,0,'surname'); $forename = mysql_result($result,0,'forename'); } ?> <tr> <td>Surname:</td> <td><input type="text" name="surname" value="<?php echo $surname ?>" /></td> </tr> <tr> <td>Forename:</td> <td><input type="text" name="forename" value="<?php echo $forename?>"/></td> </tr> </table> </form> </center> There, I cleaned it up and fixed some stuff. Besides the html and php being funky, the reason it wasn't working was because you weren't processing the form. The flow of this type of thing is: 1. enter data into form 2. submit form 3. page calls itself and passes data from form 4. page checks for form data and if it is there, uses it. The original script wasn't passing the form data to anything and it wasn't listening for any form data -
rand(1000000000,9999999999)
-
not exactly sure what you are asking. Is your script currently just sending a blank email and you want it to actually send some info? the syntax for mail() is mail($to, $subject, $message, $headers); so in your script you just need to create a string containing the info you want to email and then pass it to mail() as the 3rd argument. everything echo'd (to stdout) in a cron run script will be written to syslog unless you redirect it. So echoing output in a cron script is only really good for debugging and logging.
-
may or may not help, but you know you can mix php and html right? This is valid code: <?php $test = 1; $test2 = 2; $test3 = array("one" => 1, "two"=> 2, "three"=> 3); ?> <table> <tr> <td><?=$test?></td> <td><?=$test2?></td> </tr> <? foreach($test3 as $key => $value){ ?> <tr> <td><?=$key?></td> <td><?=$value?></td> </tr> <? }?> </table> Not a single echo statement and my html is basically how it would be without the php at all.
-
but you aren't submitting back to login.php. You are submitting to index.php that has login.php included. don't think about includes as loading different pages. An include essentially copy/pastes code from the included file to the location in the parent script where the include is located. So you need to put your checks for the login credentials somewhere on index.php that they will be parsed. without dissecting your code, this is how I handle user managment: I have an 'accesscontrol' page similar to your login.php It is included at the top of every content page. This page does the following: <?php session_start(); ##################### Logout ########################### if($_GET['logout']){ $logout = 1; $_SESSION = array(); session_destroy(); } ######## Check to see if previous session exists ######################### #### if it's a login event, $uid and $pwd take the passed values #### otherwise they take the session value. If both are empty, the variables are left empty $uid = isset($_POST['uid']) ? $_POST['uid'] : $_SESSION['uid']; $pwd = isset($_POST['pwd']) ? md5($_POST['pwd']) : $_SESSION['pwd']; ############## If previous session does not exist, prompt for login ################ if(!isset($uid)) { //display log in prompt exit; //prevent rest of page from loading } ################# If uid is set, then refresh session variables ############################### #### Since I made it past the $uid check without the script dieing, it has to be set $_SESSION['uid'] = $uid; $_SESSION['pwd'] = $pwd; $query = "Select `group` from users where `name` like '$uid' AND `pass` like '$pwd'"; $result = mysql_query($query); ######################## IF uid or password not found in the database, deny access ################## if (mysql_num_rows($result) == 0) { unset($_SESSION['uid']); unset($_SESSION['pwd']); display "Access denied page" exit; } ################ If all checks are passed, continue in to page ##################################### ?> Since this is loaded before everything else, it verifies users before loading anything else and catches login events. In the login section, the form just reloads whatever page it was trying to load the first time, but passes on the $_POST login variables which are caught and used to verify and create the session.
-
if you have to ask 'is it safe' it probably isn't and in this case that is true. escape ALL form generated input going into a database. Doesn't matter if it comes from a text box or a select box or even a hidden field. In fact, you have to escape it to allow single quotes, otherwise the query will break, regardless if it's intentional injection or not.
-
I'm assuming you are looking for a way to run cron on windows? I haven't used this personally, but can't see why it wouldn't work http://cronw.sourceforge.net/ your other option is to learn to use windows task scheduler, which will get the job done but (IMHO) isn't as simple or flexible as cron
-
So you want a table with a button on each row such that when you press the button it submits just that one row? Build your table and populate the rows with a button at the end of every row. Give the button whatever value you want, but dynamically give each button a unique name corresponding to the 'row id'. When you submit this form, your $_POST array will contain the info from the button pressed to submit the form. Since it has a unique name, you know with button was pressed even though you have many on the same form. Here's an example: <?php if(isset($_POST)) print_r($_POST); $query = "" // put query here $result = mysql($query); ?> <form action="<?=$_SERVER['PHP_SELF']?>" method="POST" name="tableData"> <table> <tr> <td>Col 1</td> <td>Col 2</td> <td>Col 3</td> <td> </td> </tr> <?php while($row = mysql_fetch_array($result)){ ?> <tr> <td><?=$row['col1']?></td> <td><?=$row['col2']?></td> <td><?=$row['col3']?></td> <td><input name="<?=$row['index']?>" type="submit" value="Submit"/></td> </tr> <? } ?> </table> </form> if you insert some database code and change the values in the while block to match, that script will build a table of the rows returned by the query with a submit button at the end. Click on different buttons and see what happens.
-
I use this and it works in both FF and IE7 (don't know about 6) <?php if (ob_get_level() == 0) ob_start(); echo str_pad('',4096)."\n"; ?> <div id='loading'> Loading. Please wait </div> <? ob_flush(); flush(); //Do stuff here ob_end_flush(); ?> The echo str_pad('',4096)."\n"; is kind of important as some browsers won't flush the buffer (even if you tell it to) unless there are a minimum number of characters in it.
-
I hate using now() and all mysql date functions because of the way it stores the date. Maybe I'm just old school, but I prefer to work with normal old unix time stamps. I know my code will explode and cause a rift in the space time fabric if I attempt to insert a date before 1970, but for this type of app, that doesn't seem to be an issue.
-
where is the 18 / 50 coming from? because 18 / 50 is .36, but 365 / 1000 is .365
-
there are several ways to time stamp. MYSQL has some timestamping functions, but I would just use time() to get the current time stamp and then add it to the insert statement.
-
good question. Also what is the structure of the 'customers' table? When someone completes the transaction are you storing their personal data? Do they create an account holding their address info? I would probably make that field store a reference to the customer table if it's a logged in or returning customer. If it's not, use the session ID, then store that ID in a cookie if you want the customer to be able to retrieve the cart after closing and reopening the browser. It might also be worth timestamping the entries so you can have an automated script run every night and clean up entries that are xxx hours/days/weeks old.
-
your code could use some optimizing but I believe your issue is inconsistent units. Your velocity is being entered in as miles per hour, but you are using time in seconds. Here are my suggestions: 1. Convert time into seconds as soon as you read it in. 2. Convert velocity into miles/second 3. Cast all three values as floats What is happening... When you divide distance by velocity, your velocity is too high by a factor of 3600 (60^2) giving you a relatively small number for time (in seconds). When you convert to minutes, this number is almost always going to be less then a minute, so it is rounded to zero. So the simple solution is to just divide your incoming velocity by 3600, but I would simplify your code too. Something like this: <?php if(isset($_GET['time']) | isset($_GET['velocity']) | isset($_GET['distance'])) { $time = ((float)$_GET['time'])*60; //time in seconds $velocity = ((float)$_GET['velocity'])/3600; //velocity in mi/s $distance = (float)$_GET['distance']; //distance in mi if( $time != '' && $velocity != '') { $distance = $time * $velocity; } if( $time != '' && $distance != '') { $velocity = $distance/$time; } if( $distance!= '' && $velocity != '') { $time = $distance/$velocity; } $deg = $_GET['degrees']; //Convert results $distance_mi = round($distance); $velocity_knotts = round($velocity*3600*0.868976242); //convert to mph then to knotts $time_minutes = round($time/60); //convert to minutes ?> Hello, <br><br> Given the information and selections you provided, the calculations have been made and the generated information is below. <br><br> Traveling at <b><?=$velocity_knotts; ?> kts</b><br> Turning <b><?=$deg; ?></b>° degrees<br><br> Will take you <b><?=$time_minutes; ?></b> minutes and <b><?=$distance_miles; ?></b> miles <?php }else{ ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> </head> <body><FORM action="test.php" method="GET" enctype="multipart/form-data"> To calulate a number, enter the two you are not looking for (ie searching: time, provide: speed, distance) <br> <br> Speed: <INPUT maxlength="3" name="velocity" type="text"> mph<br> Time: <INPUT name="time" type="text"> minutes<br> Distance: <INPUT name="distance" type="text"> miles<br><br><INPUT type="submit" name="submit" value="Calculate!"> </FORM> </body> </html> <?php } ?> casting might not be necessary, but I don't trust do math problems on variables with implied type. I've had results turn out orders of magnitude wrong because one variable 3 equations deep was automatically cast as an int instead of a float (rounding off the decimal). Those are not fun to debug.
-
i haven't worked out the regex yet, but it might be easier to write it from the inside out. build a regex that matches to roughly [quote]anything but quote tags[/quote] so that it only matches the innermost tags, replaces them, then runs again.
-
something like this should work: <?php function transQuote($text){ $QuoteLayout = '<table width="90%" border="0" align="center" cellpadding="0" cellspacing="0"> <tr> <td class="quotecodeheader"> Quote:</td> </tr> <tr> <td class="quotebody">$1</td> </tr> </table>'; $pattern = "/\[quote\](.*)\[\/quote\]/is"; if(preg_match($pattern,$text)){ $text = transQuote(preg_replace($pattern, "$QuoteLayout", $text)); } return $text; } ?> $Text = '[quote][quote]This is a quote[/quote][/quote]'; echo transQuote($Text); gives <table width="90%" border="0" align="center" cellpadding="0" cellspacing="0"> <tr> <td class="quotecodeheader"> Quote:</td> </tr> <tr> <td class="quotebody"> <table width="90%" border="0" align="center" cellpadding="0" cellspacing="0"> <tr> <td class="quotecodeheader"> Quote:</td> </tr> <tr> <td class="quotebody">This is a quote</td> </tr> </table> </td> </tr> </table> Your regex was actually okay....you just needed to make it greedy NOTE: this will only handle a single set of nested tags. It doesn't work on " ", but the approach is valid. You would just need to use preg_match_all to pull out the subexpressions and act on them individually.
-
don't know for sure, but have an idea. I think you are going to want to formulate your regex using lookbehinds such that it catches the 'outside' tags. Right now with nested tags, the regex picks up the first opening tag and the first closing tag. This leaves the inner qoute with an opening tag and not closing tag and and extra closing tag at the end. (use preg_match and see what I mean). You need to have the regex look for the opening quote tag from the front and the closing quote tag from the rear of the string. Once you can do that, I would write a self recursive function. It would search the text string for the quote tags and if it finds them replace the block with the table (like you have) THEN call itself on the new string. It should continue to dig deeper and deeper until it runs out of tags, then exits. That is if there isn't a fancy way of having regex do it for you (which there very well might be)
-
or $value = mysql_result($resource,$row_num,'col_name'); mysql_fetch_assoc and mysql_fetch_array return an [associative] array of the entire row. If you just want a single value, mysql_result gives it to you. just replace $row_num with 0 (zero) if only a single row is going to be returned. If the query only returns a single attribute, then you can leave the 'col_name' off as well. so something like <?php $query = "SELECT COUNT(`names`) FROM table"; $result = mysql_query($query); $num_names = mysql_result($result,0); ?> would set $num_names to the COUNT(`names`);