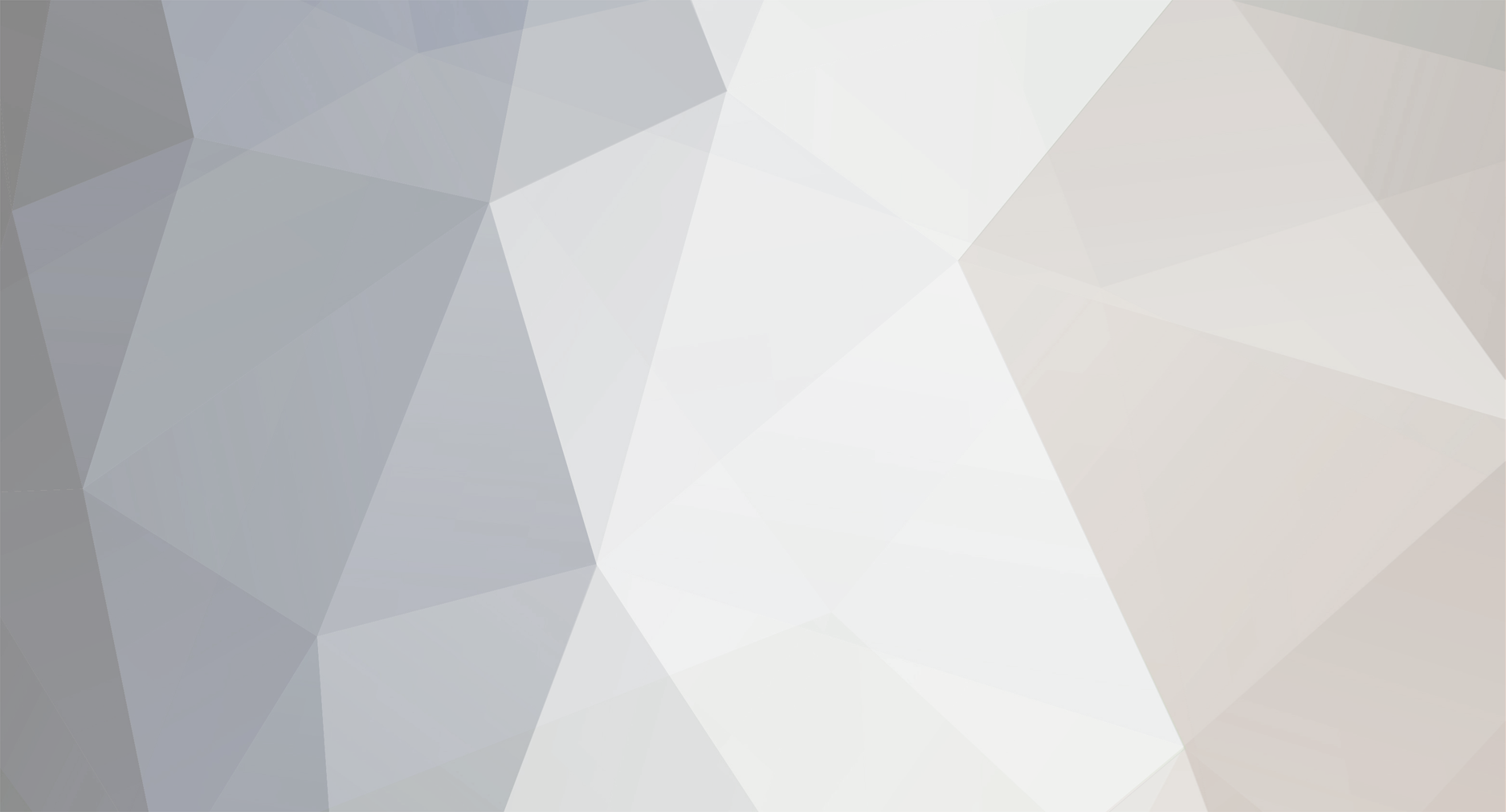
mbeals
Members-
Posts
247 -
Joined
-
Last visited
Never
Everything posted by mbeals
-
[SOLVED] csv file to array .. got myself stuck
mbeals replied to severndigital's topic in PHP Coding Help
You need to reference it like: $all[$row_num][$key]; so the first product ID would be $all[0]['productid'] the second: $all[1]['productid'] does that help? -
i learned through practice too. mostly w3 Your issue is $navvalue= $_GET['$secid->id']; php interprets single quotes as string literals, so it is physically looking for the $_GET key named $secid->id and not the dereferenced value. try: $id = secid->id; $navvalue= $_GET[$id]; or $navvalue= $_GET[$secid->id]; and see what happens. Might also be worth adding an echo secid->id; to make sure it is what you think it is.
-
okay, your loop issue can probably be solved with unix time stamps. Look up mktime and date. It might require some trickery, but you should be able to make it work. I would figure out the timestamp for the starting date and for the ending date (today), then run a for loop using 86400 as your interval step. Time stamps are increasing integers, so it won't be phased by spanning months. Since you aren't worried about H:M:S, just day, you might have to do some regex matching to only match the first so many digits of the time stamp, but it shouldn't be difficult. For the ftp server, I think you are going to have to pull in a list of files into an array, figure out which ones you want, then run a loop to fetch and process. You could use ftp_nlist to get a list of files, then in your loop test the file with ftp_mdtm() to decide whether it needs to be downloaded and processes, or you could use ftp_rawlist to pull down a list of file name and info (including mod time) that you would have to process with regex to get a mod time you could test against. I could see doing it either way.
-
i think your main issue is type conversion. $rate starts as a string, but you want to process it like a float. To convert it to a float, you started off correctly with the ltrim to ditch the '$'. Once that is gone, we need to convert $rate into a number. This is called type casting and in php it is done with (type): So the first part of the code is : $rate_num = (float)ltrim($rate,'$'); There I stripped the $ off the string, converted it to a float. Those steps were common to both if blocks, so I pulled them out. Now all we need to do is check the value of load_dock and add 75 and then multiply by 1.13. if ($load_dock=='Load_Dock_Yes') $rate_num += 75; $rate_num *= 1.13; ; So if $load_dock == 'Load_Dock_Yes' we add 75. If it doesn't, nothing happens. Then we finally multiply the number by 1.13. The += is short hand for $rate_num = $rate_num + 75. If you want to be technical, it is also a faster (computationally) way of doing it as well. Same for the *=. so the overall code is: <? $rate_num = (float)ltrim($rate,'$'); if ($load_dock=='Load_Dock_Yes') $rate_num += 75; $rate_num *= 1.13; ?>
-
<tr> <td><?=$row['prod_id'] ?></td> <td><?=$row['prod_name'] ?></td> <td><?=$row['prod_desc'] ?></td> <td><?=$row['prod_price'] ?></td> <td><input type="checkbox" name="<?=$row['prod_id'] ?>" value="1" /></td> </tr> then wrap the whole table in a form tag. If prod_id isn't unique to each product, then just replace the 'name' attribute with something that is. This will give you a $_POST array with all prod_id's as keys and '1' as values for the ones that were checked. Just iterate through the $_POST array to get all 'checked' values. To see what I mean, direct the form to a php page with print_r($_POST); at the top and you will see how that table is being broken down.
-
my only good example is written perl, but take a look at http://www.w3schools.com/PHP/php_ref_ftp.asp It should give you enough info to put together a script.
-
how is it behaving now and how do you want it to behave? You are also doing some funky type conversion, which could be causing issues until we know what exactly is going on here, i'm not really sure where to begin
-
if it's a low load server like you say, just take the same function you'd use in the cron job and stick it in an exec() block on either page. If it's deleting file older then 5 days, you should have no issue interfering with the other app. you can use "find" on a linux box to get the list of files older (or newer) then x days
-
this may not be a popular answer on a php forum, BUT.... do it in Perl. Perl has both mysql and ftp modules that will allow for direct interaction with an FTP server... so you won't have to make system calls and hack apart the serialized return. read: http://search.cpan.org/dist/libnet/Net/FTP.pm I would use the ls() method to get a list of files into an array. Then I'd iterate over that array, getting the last modified time using the mdtm( ) method.... then test that time and perform whatever action it is you need. I've done ftp in php, perl and BASH and by far, perl is the simplest and most elegant way of handling it. I can post one of my ftp scripts that uses mysql queries if you'd like to give you a head start
-
maybe it's just not syntax I'm used to, but I've never seen queries handled like that. Personally, I would do like: <? //database connection stuff if($db){ $query = "select * from product"; $result = mysql_query($query); ?> <table border bgcolor ='green'> <tr> <th>prod_id</th> <th>prod_name</th> <th>prod_desc</th> <th>prod_price</th> </tr> <? while($row = mysql_fetch_array($result) ){ ?> <tr> <td><?=$row['prod_id'] ?></td> <td><?=$row['prod_name'] ?></td> <td><?=$row['prod_desc'] ?></td> <td><?=$row['prod_price'] ?></td> </tr> <?} //end while }else die("sorry could not retreive product listings"); ?> basically, I perform the search, build the table structure and headings (static data), then fill in the dynamic stuff with a while loop. Does that make sense? You are also missing your / on the closing <th> blocks in the original code.
-
create the php script so that it will run from the command line (test it first). go to start -> control panel -> scheduled tasks. add a new task. In the the task properties is a 'run' box. Enter the full path to the php executable and to the script with any needed arguments. in the batch file option, you would just write a batch file to do the search and delete and ignore php completely. Not as easy as the linux shell script, but still doable. In fact, if you google around a bit, you should be able to find some compiled scripts that will do this. I've been in the linux environment so long now that no names come to mind.
-
his syntax should work. First step in debugging mysql is to echo the query back to STDOUT to make sure it is what you think it is. For this reason, I consider it good practice to structure queries in the form: $query = "My mysql query"; $result = mysql_query($query); So add this to the second file's while loop and verify the final string is correct. echo "UPDATE `thinkweb_advanced`.`section` SET `nav` = '$navvalue' WHERE `section`.`id` =$secid LIMIT 1<br>";
-
is this a linux or a windows server and do you own it? This type of task is MUCH better suited for a system daemon running in the background. What happens if you get 10,000 visitors to the page in 2 minutes? that's a lot of unnecessary calls to the function. If it's a linux system, just google cron and file clean up. It's literally a 1 line command. If it's windows, write a batch file and schedule it. as was mentioned before, it can be done with php server calls too (look up exec() and system() ), but it's really not the best way to go about it. It sounds as if you are using a flat file database system for temporary storage. There might also be a more elegant means to accomplish the task without the use of temp files. What exactly is the script doing?
-
insert echo $output_data; before the preg_match and post the contents. I just tested: $string = '<a href="http://www.mysite.com/index.php?a=in&u=username&sid=rjhgf2hWm9WDFTkgx9JJd290udtkIgfM" class="buttongo">Enter</a>'; $regex = '/href="(.*?)" class="buttongo"/'; preg_match($regex, $string, $stuff); print_r($stuff); //Nothing comes out and it spit out: Array ( [0] => href="http://www.mysite.com/index.php?a=in&u=username&sid=rjhgf2hWm9WDFTkgx9JJd290udtkIgfM" class="buttongo" [1] => http://www.mysite.com/index.php?a=in&u=username&sid=rjhgf2hWm9WDFTkgx9JJd290udtkIgfM ) so make sure that your $output_data variable is defined and it contains what you think it does just before being parsed by the regex
-
well I think i figured out a workaround, but it's complicated. Select customers.name, customers.phone, modems.mac, modems.qos, modems.subdate, addresses.num, addresses.street, addresses.unit, addresses.building, Walkdata.MAC, Walkdata.SNR, Walkdata.RX, Walkdata.STATUS FROM addresses join customers on customers.addID = addresses.index join modems on modems.custID = customers.index left join Walkdata on Walkdata.MAC = modems.mac union Select '','','','','','','','','',`MAC`, `SNR`, `RX`, `STATUS` FROM Walkdata where `MAC` NOT IN (select `mac` from modems)" It does work, but can it be optimized?
-
doing some googling, I think I need a full outer join, but mysql doesn't support that yet. Any thoughts on a workaround? I saw one where you perform a union to a right join, but with the additional joins, that seems really clunky. Can I turn the first set of joins into a sub query and just perform the two sets of joins on that?
-
that will truncate the non matching rows from the right table though. I need to preserve those rows. Say I have these two tables: subscribers Name MAC John1 Jane2 active modems MAC status 1online 3online I need to create: Name MAC status John1online Jane2[/td] 3online the difference is that the first table is the result of two joins
-
I have 4 tables that I need to join together in a not so simple way and my brain is hurting. Table one is a table of addresses..nothing fancy. Table two contains a list of customers, where one of the attributes is a reference back to an index in the address table. Table three is a table of cable modems which has an attribute which is an index in the customer table. So joining all three tables on these ID's produces a list of customer name, address, and modem info. I have that part of the query working and it's structured like this: Select customers.*, modems.*, addresses.*, Walkdata.* FROM addresses join customers on customers.addID = addresses.index join modems on modems.custID = customers.index This produces name, address, MAC address (and some other stuff) for all modems that are registered as subscribers. Now, I have another table, which is the list of modems (and stats) for all modems that are connected to the cable plant. I need to take this info and match it against the table we just created based on mac address (a common attribute), but I don't want to throw out non matching rows from either table (so I can see who isn't connected as well as unregistered modems attempting to connect). I'm sure the answer is stupidly simple, but my brain is shutting down.
-
good catch. I knew that the sprintf function was casting it to an unsigned integer, but I wasn't thinking of it like an integer. Changed that to a float and it seems to be working. thanks
-
I have function that given a network address in slash notation will return an array of host ip's. It works for 90% of the IP's I hand to it, but for one particular one, it breaks and I can't figure out why. Here is the code: function listIPs($ip,$mask){ echo $ip.'<br>'; $ip = sprintf("%u",ip2long($ip)); //convert ip string to unsigned decimal echo $ip.'<br>'; $freebits = 32 - $mask; //calculate host bits from mask $decfree = pow(2,$freebits); //calculate the number of hosts $ips = array(); ##### Create the subnet mask from the number of host bits ##### for($i=0;$i<32;$i++){ if($i<$freebits) continue; $submask += pow(2,$i); } echo "$ip<br>"; #####Find the Subnet ID ######### $subnet = ($ip & $submask); echo decbin($ip); #### Generate a list of hosts, ignoring the first (subnet ID) and last (broadcast) ip of the range### for($i=1;$i<$decfree-1;$i++){ $ips[] = long2ip($subnet + $i); } return $ips; } The echos are there for debugging. When I run this code on the ip 199.227.155.164 / 30 I get the following output: 199.227.155.164 3353582500 3353582500 1111111111111111111111111111111 So the ip in string for (line 1) enters the function fine, it is converted to a long fine (line 2), it is still fine before entering the bitwise calc (line 3), but for some reason php's bitwise operations don't work on it (evident by decbin returning all 1's...line 4). If you put 3353582500 into google's binary converter, it converts it to 11000111111000111001101110100100, which is the binary number that my subnet calculator is reporting the IP to be. So there is something funky with the bitwise operation. Any ideas on what is happening? Like I said, it works fine for other addresses...this is the only one breaking it.
-
thanks, Wasn't aware I could do that.
-
I have a table that stores information about IP address assignments for multiple sites. Each tuple contains the site's name, the device type, it's public (management) IP address and a few other attributes. The sites are all multihomed, meaning that a single device can be (but doesn't have to be) listed twice in the table, as it would have 2 public IP's. I'm attempting to write a query to list each unique device per site (Ignoring IP). For instance, take the following set of tuples: SiteDeviceIP Office 1gateway router123.456.798.1 Office 1gateway router123.456.700.1 Office 1printer123.456.798.2 Labprinter987.654.987.5 I need to query to return: SiteDevice Office 1gateway router Office 1printer Labprinter Simple distinct statements won't work. I'm thinking Its going to have to be a sub query or an alias
-
try: $preset_table = mysql_query("INSERT INTO stageone (`web_name`, `folder`, `image_fol`, `css_file`, `js_file`, `div`) VALUES ('$web_namex', '$folderx', '$image_folx', '$css_filex', '$js_filex', '$divx')"); If that fails, pull the query out of the mysql_query block and echo it out to see exactly what the query is (after the variables are parsed). Post this result. It could an unsanitary sql string (stray ; or ' in one of the variables. $query = "INSERT INTO stageone (`web_name`, `folder`, `image_fol`, `css_file`, `js_file`, `div`) VALUES ('$web_namex', '$folderx', '$image_folx', '$css_filex', '$js_filex', '$divx')"; echo $query; $preset_table = mysql_query($query); Since you are using phpmyadmin, try to generate the query like in the second block, then copy paste it into the "sql" tab in phpmyadmin. It usually does a good job of pointing out the syntax errors.
-
depends. I tend to keep functions in their own file for the sake of readability, but I will also avoid having one huge library file. I have different files based on different needs... aka one file for database functions, one for table manipulation, one for user authentication, ect.... I'll also usually have a separate one for the random ad hoc functions on a per site basis that holds the functions that are unique to that site (that I probably won't use else where). Just make sure you document your code well, so that whomever has to debug it in the future doesn't have to hunt through hundreds of lines of libraries to find a single function.
-
I think the switch might be overkill. You could get away with a single line of tertiary notation $path = $_SERVER['DOCUMENT_ROOT'] . '/guides/php/'; $page = isset($_GET['guide']) ? strtolower($_GET['guide']) : 'intro'; include "$path/$page.php"; For those that don't get the notation it is variable = (condition) ? if_condition_true : if_condition_false so if $_GET['guide'] is set, $page takes the name of php file (which gets the .php in the include). if not, then $page is set to intro. however it looks like your bigger issue was how you were referencing the $_GET and that seems to be in order now.