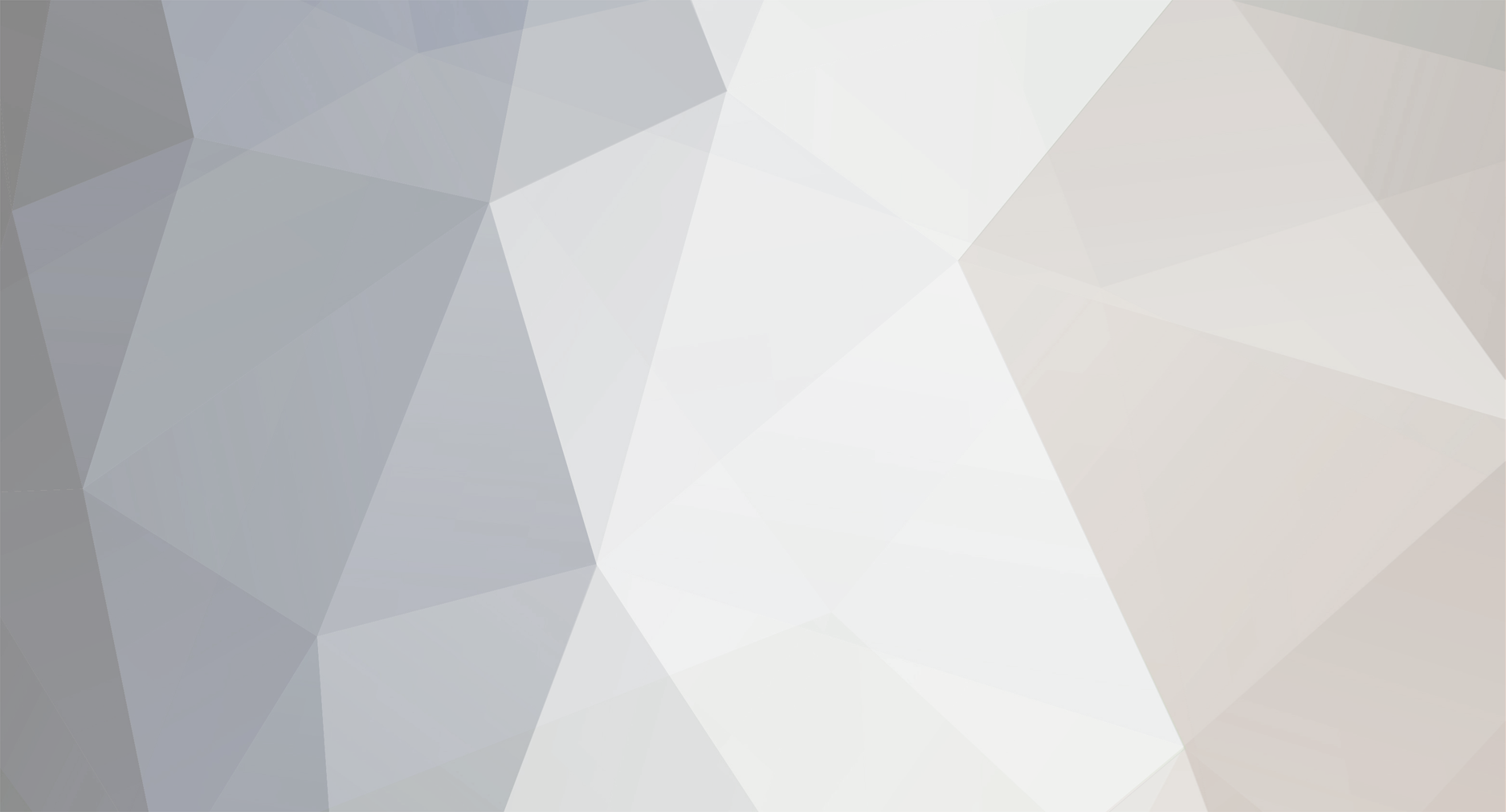
hansford
Members-
Posts
562 -
Joined
-
Last visited
-
Days Won
4
Everything posted by hansford
-
I don't know what the event arguments look like, but try this: $alertValue = array('Tornado Warning', 'Tornado Watch', 'Severe Thunderstorm Warning', 'Severe Thunderstorm Watch', 'Flash Flood Warning', 'Flash Flood Watch', 'Flood Warning', 'Flood Watch', 'Flood Advisory', 'Winter Storm Warning', 'Winter Storm Watch', 'Winter Weather Advisory', 'Special Weather Statement'); $event = array('Tornado Warning', 'Tornado Watch', 'Severe Thunderstorm Warning', 'Severe Thunderstorm Watch', 'Flash Flood Warning', 'Flash Flood Watch', 'Flood Warning', 'Flood Watch', 'Flood Advisory', 'Winter Storm Warning', 'Winter Storm Watch', 'Winter Weather Advisory', 'Special Weather Statement'); $events = array_intersect($alertValue, $event); $alertValue = array_keys(array_flip($events)); foreach( $alertValue as $alert ) { echo $alert . '<br />'; }
-
Personally, since this is data related, and that's what tables are for, I would use a table. You want everything to be flush and presentable. Tables are not "bad" like everyone is lead to believe with CSS. Otherwise, they would have dropped them from the specs. Use what available resources you have is my "opinion". As far as the dups go - store the events in an array and then compare them as a string to see if they match.
-
The code works for me, not well-formed and formatted html and php code, but it works. Try setting some error reporting at the beginning of your php file - in case you have error reporting turned off. my.php <filename> <?php // used when debugging code only error_reporting(E_ALL); ini_set('display_errors', 1); if(isset ($_POST["submit"])){ // Do something; // Do something; echo 'isset'; } else { // Do something; // Do something; echo 'not isset'; }
-
This code isn't tested and off the cuff, so I don't want to hear complaints lol This is the basic idea. if (file_exists($_FILES['pic']['tmp_name']) || is_uploaded_file($_FILES['pic']['tmp_name'])) { // grab temp file name $cur_file = $_FILES['pic']['tmp_name']; // check if it's even an image file if ((getimagesize($cur_file)) === FALSE) { // error - file is not an image file } // specify what directory to upload the file to $user_dir = "/uploaded_files_dir/"; // create the path and new file name $newfile = $user_dir . preg_replace( '/[^_A-Za-z0-9.]/', '', basename($_FILES['pic']['name'])); // upload file if ( ! move_uploaded_file($cur_file, $newfile)) { // error - failed to upload file } }
-
Ok, so my example wasn't perfect. You can choose whether to allow spaces in your passwords or not when users register. Some users like to copy and paste their passwords which sometimes adds a space. Users shouldn't be copy/pasting, but they do. <?php // use error reporting, but only while debugging error_reporting(E_ALL); ini_set('display_errors', 1); if ( isset($_POST['submit']) ) { if ( isset($_POST['password']) && isset($_POST['user']) ) { $password = $_POST['password']; // get password as it is. $user = trim($_POST['user']); $con = new mysqli($host, $dbuser, $dbpass, $dbname); if ($con->connect_errno) { die('Connection failed: ' . $con->connect_error); } // do your database stuff here } else { // redirect back to login screen } } else // redirect or whatever { exit('Direct access to this page is not allowed'); }
-
You can use anything we post on this forum. The important thing is to understand why you are writing the code you write. Regurgitating code without knowing it's purpose will never make you a better programmer. A deeper understanding of what your coding is the key. When you understand something, then you can create something. Copy/paste programming can't help you when you get stuck and actually have to come up with a solution on your own. Never post a question to a forum when you can easily obtain the answer from a simple search. (not referring to your post, but generalizing)
-
I would like to see some attempts at error reporting and some printed feedback as to just what exactly is getting returned from things such as forms, functions/methods. After your opening <?php tag you should have full error reporting turned on when in debug mode. <?php error_reporting(E_ALL); ini_set('display_errors', 1); Before you attempt to execute an query - echo it out to the screen or log the query to a file for viewing. $query = "SELECT Student_Id, Lname, Fname FROM Students WHERE Student_Id = '$id'"; echo $query; exit; When your form is submitted to the php page for processing - print the values of what the $_POST array contains. if (isset($_POST['some_value'])) { echo '<pre>'; print_r($_POST); echo '</pre>'; }
-
$_user (an underscore at the beginning of a variable name is usually used to indicate the variable is private - like in class visibility) This will come up time and time again, so I might as well say it now - meaning you might as well spend a little time learning it now rather than later - when you have no choice. Best practices for database interactions are to use prepared statements. The code you presented in this regard is deprecated and slated for removal. This means it won't run on newer versions of PHP and it will look like you don't know what your doing if you present code in this manner expecting a paycheck someday. http://php.net/manual/en/mysqli.quickstart.prepared-statements.php <?php // don't let php hide any errors every how humbling it makes you feel error_reporting(E_ALL); ini_set('display_errors', 1); if ( isset($_POST['submit']) ) { if ( isset($_POST['password']) && isset($_POST['user']) ) { $password = trim($_POST['password']); $user = trim($_POST['user']); $con = new mysqli($host, $user, $password, $dbname); if ($con->connect_errno) { die('Connection failed: ' . $con->connect_error); } // do your database stuff here } }
-
Appears you already wrote the code. All you have to do now is implement it. <?php if (isset($_GET['my_chamber'])) { $user_chamber = intval($_GET['my_chamber']); $bullet_pos = rand(1,6); if ($bullet_pos == $user_chamber) { echo "BOOM! (bullet was in chamber " . $bullet_pos . ")"; } else { echo "You made it bro :-) (bullet was in chamber " . $bullet_pos . ")"; } } else { 'DANG! out of bullets. Time to reload'; }
-
I ran the code myself. It works - all menu items and sub-menu items display. It will not update as designed. You would need to make each one of those menu items with the "update" button, its own separate form. Otherwise, it's just defaulting to the last form element - which is the contact menu item. You can change that one, but the others get ignored because they all have the same form element names. $con = mysql_connect($server, $user_name, $password); $db_found = mysql_select_db($database, $con); if(isset($_POST['update'])) { $sql = "UPDATE `menus` SET Menu_bar = '".$_POST['menu_bar']."' WHERE ID = ".$_POST['hidden']; if(!mysql_query( $sql, $con )) { echo 'update error: ' . mysql_error(); } } $resultMainMenu = mysql_query("SELECT * FROM menus WHERE Parent_id=0 ") or die(mysql_error()); echo '<form action="page.php" method="post">'; echo '<ul>'; while($row = mysql_fetch_assoc($resultMainMenu)) { echo '<li><input type="submit" name="update" value="update"><input type="hidden" name="hidden" value="'.$row['ID'].'"><input type="text" name="menu_bar" value="'.$row['Menu_bar'].'"><br/>'; // echo main menu $resultSubmenu = mysql_query("SELECT * FROM menus WHERE Parent_id=" . $row['ID']) or die(mysql_error()); if( ! mysql_num_rows($resultSubmenu)) { echo '</li>'; } else { echo '<ul>'; while($rowSub = mysql_fetch_assoc($resultSubmenu)) { echo '<li><input type="submit" name="update" value="update"><input type="hidden" name="hidden" value="'. $rowSub['ID'].'"><input type="text" name="menu_bar" value="'.$rowSub['Menu_bar'].'"><br/></li>'; // echo sub menu } // end sub-menu loop echo '</ul>'; // end sub-menu ul echo '</li>'; // end main-menu li } } // end main-menu loop echo '</ul>'; // end main-menu ul echo '</form>'; ?> And I know you're just getting your feet wet, but it would be better to get off on the right one. You should be using PDO or mysqli to make your database connections/interactions as the way you're doing it has been deprecated because of serious security related issues and slated for removal.
-
Let's clear this up - I'm sure you know what you meant, but a beginner might not. The object of the class can't even access a private variable - only within the class itself.
-
Try this - if it doesn't work, then I'll have to create a dummy db structure on my local and run the code myself to see what's going on. $con = mysql_connect($server, $user_name, $password); $db_found = mysql_select_db($database, $con); if(isset($_POST['update'])) { $sql = "UPDATE `menus` SET Menu_bar = '".$_POST['menu_bar']."' WHERE ID = ".$_POST['hidden']; mysql_query( $sql, $con ); } $resultMainMenu = mysql_query("SELECT * FROM menus WHERE Parent_id=0 ") or die(mysql_error()); echo '<form action=\"page.php\" method=\"post\">'; echo '<ul>'; while($row = mysql_fetch_assoc($resultMainMenu)) { echo "<li><input type=\"submit\" name=\"update\" value=\"update\"><input type=\"hidden\" name=\"hidden" value=\"".$row['ID']."\"><input type=\"text\" name=\"menu_bar\" value=\"".$row['Menu_bar']."\"><br/>"; // echo main menu $resultSubmenu = mysql_query("SELECT * FROM menus WHERE Parent_id = " . $row['ID']) or die(mysql_error()); if( ! mysql_num_rows($resultSubmenu)) { echo '</li>'; } else { echo '<ul>'; while($rowSub = mysql_fetch_assoc($resultSubmenu)) { echo "<li><input type=\"submit\" name=\"update\" value=\"update\"><input type=\"hidden\" name=\"hidden\" value=\"". $rowSub['ID']."\"><input type=\"text\" name=\"menu_bar\" value="\".$rowSub['Menu_bar']."\"><br/></li>"; // echo sub menu } // end sub-menu loop echo '</ul>'; // end sub-menu ul echo '</li>'; // end main-menu li } } // end main-menu loop echo '</ul>'; // end main-menu ul echo '</form>'; ?>
-
I am unable to reproduce your error on strings containing " or '. Let us look at the form code and see if there is something there. You wouldn't happen to have magic_quotes on would you?
-
Here is the html structure you're after. <ul> <li>Home</li> <li>News <ul> <li>Events</li> <li>Places</li> </ul> </li> <li>Contact</li> </ul> Reworking your code. echo '<ul>'; echo "<li><input type=\"submit\" name=\"update\" value=\"update\"><input type=\"hidden\" name=\"hidden" value=\"".$row['ID']."\"><input type=\"text\" name=\"menu_bar\" value=\"".$row['Menu_bar']."\"><br/>"; // echo main menu $resultSubmenu = mysql_query("SELECT * FROM menus WHERE Parent_id = " . $row['ID']) or die(mysql_error()); if( ! mysql_num_rows($resultSubmenu)) { echo '</li>'; } else { echo '<ul>'; while($rowSub = mysql_fetch_assoc($resultSubmenu)) { echo "<li><input type=\"submit\" name=\"update\" value=\"update\"><input type=\"hidden\" name=\"hidden\" value="\". $rowSub['ID']."\"><input type=\"text\" name=\"menu_bar\" value="\".$rowSub['Menu_bar']."\"><br/></li>"; // ec ho sub menu } echo '</ul>'; echo '</li>'; } I believe I got that right - you'll have to test it - might have missed an escape or some other syntax error.
-
Show us your database table structures. All of the parent menus in the menus table have a Parent_id = 0; You're getting the sub-menu of each parent menu by looking for the Parent_id = $row['ID']. So we can assume from this that the $row['ID'] of each parent matches the Parent_id of their sub-menu.
-
array_push() not adding anything to array
hansford replied to pcristian43's topic in PHP Coding Help
@Psycho Actually, you goofed twice lol. You trim the $_POST variable and assign it to $var. But then check $foo for the empty string. Anyways, it happens - we get what you are saying. And QuickOldCar posted something similar, so it seems to be the the acceptable way of handling it. -
Welcome - You're attitude will take you far. Barand is in his sixties and I'm glad he and others stick around to share their knowledge with the rest of us. You are in good hands - if one of us post a reply and Barand, QuickOldCar or another senior member believe it's not the best solution - they will let us know and respond with something more robust.
-
The code seems a bit jumbled. <?php // CONNECT TO THE SERVER AND SELECT DATABASE // not needed $server = ' < // not needed $link = mysqli_connect( <?php ' // mysqli_connect($host, $user, $password, $database); // change $link = mysqli_connect('jimmybryantnet.ipagemysqli.com', 'jimmyb29', '*******'); if (!$link) { die('Could not connect: ' . mysqli_error()); } echo 'Connected successfully'; // not needed mysqli_select_db(guestbook); // not needed ?> ); // not needed if (!$link) { die('Could not connect: ' . mysqli_error()); // not needed echo 'Connected successfully'; // not needed mysqli_select_db(guestbook); // not needed ?> '; // appears to be making a redundant connection to the same database, so // not needed here - should be defined at top of page $user = 'jimmyb29'; // not needed here - should be defined at top of page $password = 'alfiep69'; // not needed here - should be defined at top of page$dataBase = 'guestbook'; // not needed $conx = mysqli_connect($server,$user,$password); // not needed $db_selected = mysqli_select_db($dataBase,$conx);
-
array_push() not adding anything to array
hansford replied to pcristian43's topic in PHP Coding Help
@Psycho Running a test: $arg = array(); $v = ""; if(isset($v)) { echo 'empty string allowed to pass <br />'; } // result: 'empty string allowed to pass' if(isset($v) && !empty($v)) { echo 'empty string slipped through <br />'; } else { echo 'empty string not allowed to pass <br />'; } // result: 'empty string not allowed to pass'; I had a problem with this many years ago and when I began doing this - I haven't had a problem since. Let me know your opinion. Trim - I trim all user input. " People do this "; With array_push, I used the return value improperly - thanks for the heads up. I personally wouldn't use it for this because it's slow and unnecessary. $your_array = array(); $your_array[] = 'some value'; $your_array[] = 'another value'; -
array_push() not adding anything to array
hansford replied to pcristian43's topic in PHP Coding Help
<form name="form_name" method="post" action=""> <?php $your_array = array(); if(isset($_POST['add']) && !empty($_POST['add']) { if(array_push($your_array, trim($_POST['add']) { print_r($your_array); } else { "failed to push item on array." } -
Good question Texan. I didn't know the answer, but went digging. Found an old post on stackoverflow answered by a senior member who states it is not possible using in-line styles. http://stackoverflow.com/questions/5293280/css-pseudo-classes-with-inline-styles
-
ul.alertLegend li Is an example of a class selector. An Unordered List with a class of "alertLegend" Semantics I know, but some might be viewing and looking things up by what we call them. pseudo classes have the double colon. a:link a:hover p:first-child p:first-line
-
Before you execute the query, echo the query string to see what it actually looks like in string form. We don't see any code showing the actual insert execution, so we can't see if there's potentially a problem there.