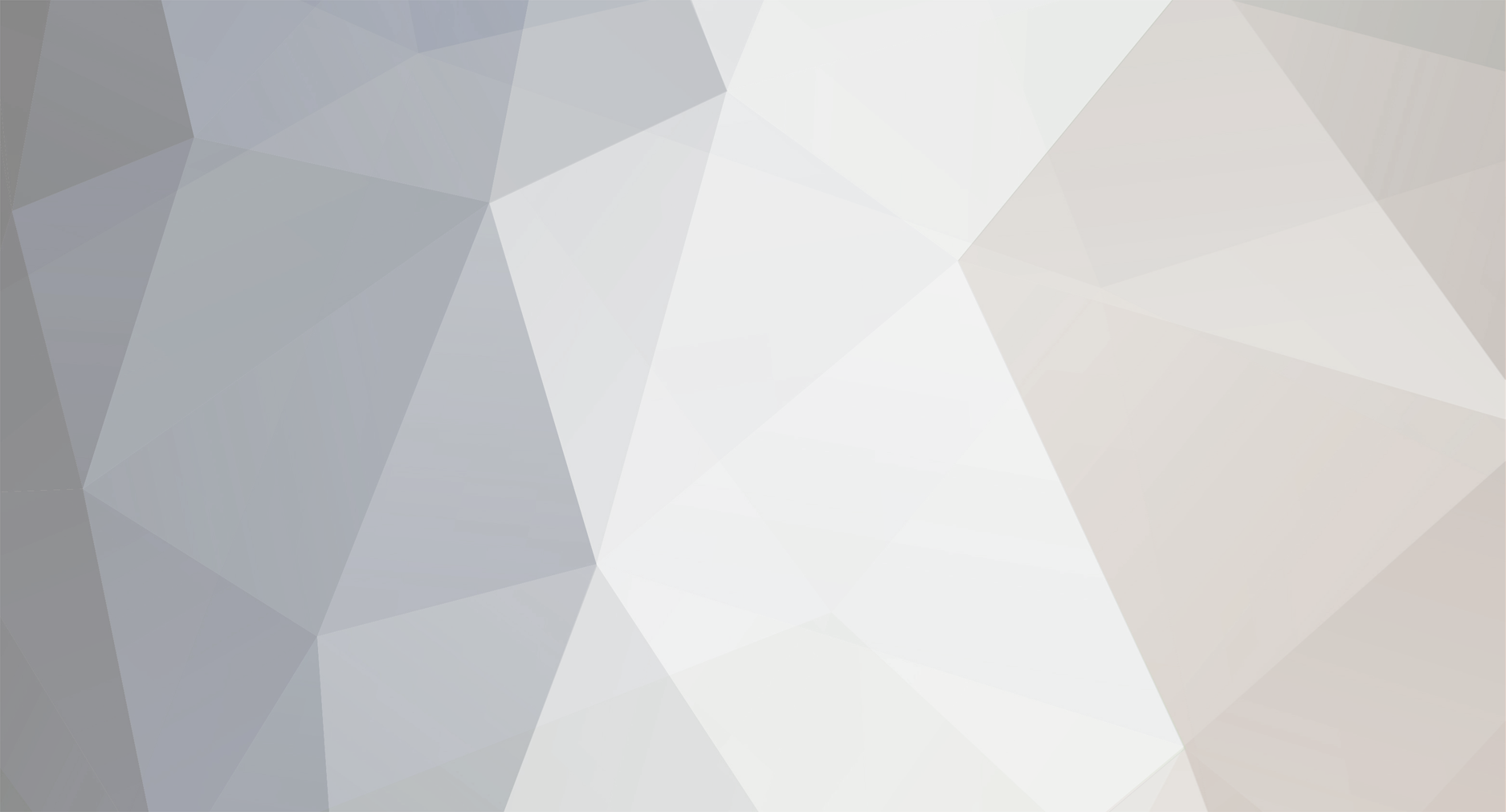
hansford
Members-
Posts
562 -
Joined
-
Last visited
-
Days Won
4
Everything posted by hansford
-
value gets inserted after refeshing the page? need help
hansford replied to shan's topic in PHP Coding Help
<form action="1.2.php" method="post"> This form goes to the page set in the action. 1.2.php needs to do its work and then redirect back to this form - no refresh on a form page as it just resubmits the form and thus the blank db insert. -
value gets inserted after refeshing the page? need help
hansford replied to shan's topic in PHP Coding Help
comment out the actual code that inserts anything into your db - echo the query instead so you can see what's happening. You must be hitting the db somewhere. Get rid of the location header until you can find the problem. Use an include for that page instead. -
value gets inserted after refeshing the page? need help
hansford replied to shan's topic in PHP Coding Help
Every time you refresh the page, it resubmits the form. Have the php page go back to your html page. -
Do yourself a favor to save frustration - echo out your query before hitting the database. echo $query; exit(); $squery = mysql_query($query); $numrows = mysql_num_rows($squery);
-
Very confusing Notion as we only see parts of the code. PHPMailerAutoload() must be getting called somewhere - code is not "magic" - to us anyways.
-
This can be accomplished, but why would you need to - once the user is out of the picture - using "automatically", then you can just process the form info on one page - what are you really trying to do - hide a URL or something. Tell us the task you really want to accomplish.
-
You're trying to follow someone else's code which is daunting for even a seasoned developer. If you need help finding explanations on PHP functionality - it's everywhere. The best documented programming language in the world. Google any php method, language construct etc.. and you will get a link to the php manual. It will be your best friend.
-
WordPress has been pieced together through the years and with all of the plugins - it just makes it that much more difficult to follow the code. You need tools to properly work. You need an IDE with search capabilities. I use Eclipse, as I'm a throwback from Java, but I don't recommend it for PHP. I've read PhpStorm is good, but no first hand knowledge.
-
Here is a link to the particular WordPress plugin you are using. http://auctionplugin.net/ They claim to have world class support.
-
echo preg_replace('/two.*three/s','xxx',$str); But I "believe" this is more efficient. echo preg_replace('/two\s+three/','xxx',$str);
-
"bgcolor", haven't seen that one used in awhile. I would use CSS for this - I'm no expert on rgb, but the browser has the last say in how anything is rendered. Why do you need to use rgb btw - can't just use a class
-
For the jQuery, simply change live() to on() For the Mysql - Every line you write today will have to be changed sooner or later because it simply won't parse one day.
-
In jQuery, the live() method is deprecated. The on() method is the current preferred method. The mysql code you're using is deprecated. The preferred way is Mysqli or PDO.
-
Here is an ugly, down and dirty way example. Not downloadable (copy and paste), zero formatting etc.. <?php if (isset($_POST['submit'])) { // grab your post values, do DB interactions etc. echo 'Form submitted successfuly.'; exit(); // or redirect to another page } elseif (isset($_POST['xml'])) { $name = $_POST['name']; $birthday = $_POST['birthday']; echo '<pre>'; echo htmlspecialchars('<?xml version="1.0"?>'); echo htmlspecialchars('<person>'); echo htmlspecialchars("<name>$name</name>"); echo htmlspecialchars("<birthday>$birthday</birthday>"); echo htmlspecialchars('</person>'); echo '</pre>'; exit(); } ?> <html> <head> </head> <body> <form name="form" method="post" action="index.php"> Name:<input type="text" name="name"><br /> Birthday:<input type="text" name="birthday"><br /> <input type="submit" name="submit" value="submit"> <input type="submit" name="xml" value="export to xml"> </form> </body> </html>
-
ginerjm - posted the preferred way to code this - smooth, elegant - with error handling. Just test that rowCount() works on the particular DB you're using. I use rowCount(), but because of the manual "warning", I am in the habit of using fetchColumnt() - however, it is an extra query and slows things down and usually unnecessary.
-
I would reword the problem - exactly - and repost - let other's have a nibble at it.
-
My apologies, but CroNiX posted the solution for you - here is the code he posted: <?php include("AddStats_admin_connect.php"); //connect to database doDB(); //Function to build select options based on passed array function buildSelectOptions($options) { $optionsHTML = ''; foreach($options as $id => $label) { $optionsHTML .= "<option value='{$id}'>{$label}</option>\n"; } return $optionsHTML; } //Run query to get the ID and Name from the table //Then populate into an array $clist_sql = "SELECT CID, Country FROM Countries"; $clist_res= mysqli_query($mysqli, $clist_sql) or die(mysqli_error($mysqli)); if (mysqli_num_rows($clist_res) < 1) { //this Country not exist $display_block = "<p><em>You have selected an invalid Country.<br/> Please try again.</em></p>"; } $countries = array(); while($Ctry_info = mysqli_fetch_array($clist_res)) { $countries[$Ctry_info['CID']] = $Ctry_info['Country']; } $countryOptions = buildSelectOptions($countries); ?> <!DOCTYPE html> <html lang="en"> <head> <title>Stats</title> <link rel="stylesheet" href="stylesheets/style.css" /> <!--[if IE]> <script src="http://html5shim.googlecode.com/svn/trunk/html5.js"></script> <![endif]--> </head> <body> <form action="CountryOptions.php" method="post"> <!-- You need to set up the select element to house the options. This will be set in $_POST as $_POST['country'] --> <select name="country"> <?php echo $countryOptions; ?> </select> <input type="submit" value="Submit Choice"> </form> </body> </html>
-
Sorry, I can't test the code for errors and I don't know from your file which line the error occurred. Looking at it - I see: if ($result->fetchcolumn() > 0) fetchcolumn - should be fetchColumn with a capital 'C'.
-
Try: $username = $_POST['username']; $pdo = new PDO('mysql:host=xxxxx;dbname=xxxx', 'xxxx', 'xxxx'); $sql = "SELECT COUNT(*) from TABLENAME where username='".$username."'"; if ($result = $pdo->query($sql) { if ($result->fetchcolumn() > 0) { $sql = "SELECT * from TABLENAME where username='".$username."'"; foreach ($pdo->query($sql) as $row) { echo $row['Player_Name'] . ' ' . $row['Time_Online']; } } } else { echo "Sorry, that username does not exist in our database."; }
-
There is a problem in your logic. If you enter any value in the form that exists in the database - it's going to return that row's value because you asked it to with this select statement. SELECT * from TABLENAME where username='".$username."'"
-
$countries[] = array($Ctry_info['CID'], $Ctry_info['Country']); buildSelectOptions( $countries ); function buildSelectOptions($options) { $optionsHTML = "<select name=\"countries\">\r\n"; foreach($options as $c) { $optionsHTML .= "<option value=\"{$c[0]}\">$c[1]</option>\r\n"; } $optionsHTML .= "</select>\r\n"; return $optionsHTML; } This code is meant to demonstrate how things could be done in your code. Wanted you to think, not just paste other's code as that does nothing for your knowledge. Let me demonstrate how this code works and how it could be used - and there is several ways to do the same thing. This might confuse you further or help you understand what's going on. <?php // lets assume this is your DB result set $row = array( array('CID'=>100,'Country'=>'France'), array('CID'=>200,'Country'=>'Germany'), array('CID'=>200,'Country'=>'Germany') ); // define the array to pass to buildSelectOptions() function $countries = array(); // loop through the DB result set and extract information // with a db result set most of this work is done for you behind the scenes // but we will have to do the work, since we don't have a result set for demo purposes foreach ($row as $r) { $c = array(); foreach ($r as $key => $value) { $c[] = $value; } // add the CID and Country of each row in the result set // to a row in the $countries array // in your result set this is all you would need // array($Ctry_info['CID'], $Ctry_info['Country']); // here we need to use the temp array $c $countries[] = array($c[0], $c[1]); } // in your html code, you can simply do this // call function and pass array as argument // echo buildSelectOptions( $countries ); // for demo - use this code to see how it works echo htmlspecialchars(buildSelectOptions( $countries )); Here is the buildSelectOptions() function again. function buildSelectOptions($options) { $optionsHTML = "<select name=\"countries\">\r\n"; foreach($options as $c) { $optionsHTML .= "<option value=\"{$c[0]}\">$c[1]</option>\r\n"; } $optionsHTML .= "</select>\r\n"; return $optionsHTML; }
-
I believe someone already mentioned that will need to use Ajax for this. However, when you created the user select element - you must have gotten the id for that item in the db - you won't need Ajax if you just create the select with the id as the value. <select name="whatever"> <option value="db_id">whatever name</option> </select> Use php to dynamically create the select element in the form - that's the whole purpose of the language.
-
It does, but not everybody might read it - it's grayed out and not the center of focus.
-
I was going to mention cutting out the middle man, but thought - maybe he uses it for other things, besides building a select array. But I was referring to this anomaly in the code: $countries = array(); $Ctry_info = array(); $Ctry_info['CID'] = 107; $Ctry_info['Country'] = 'Spain'; print_r( $Ctry_info ); // key value array $countries[$Ctry_info['CID'] = $Ctry_info['Country']]; // error print_r( $countries ); exit;