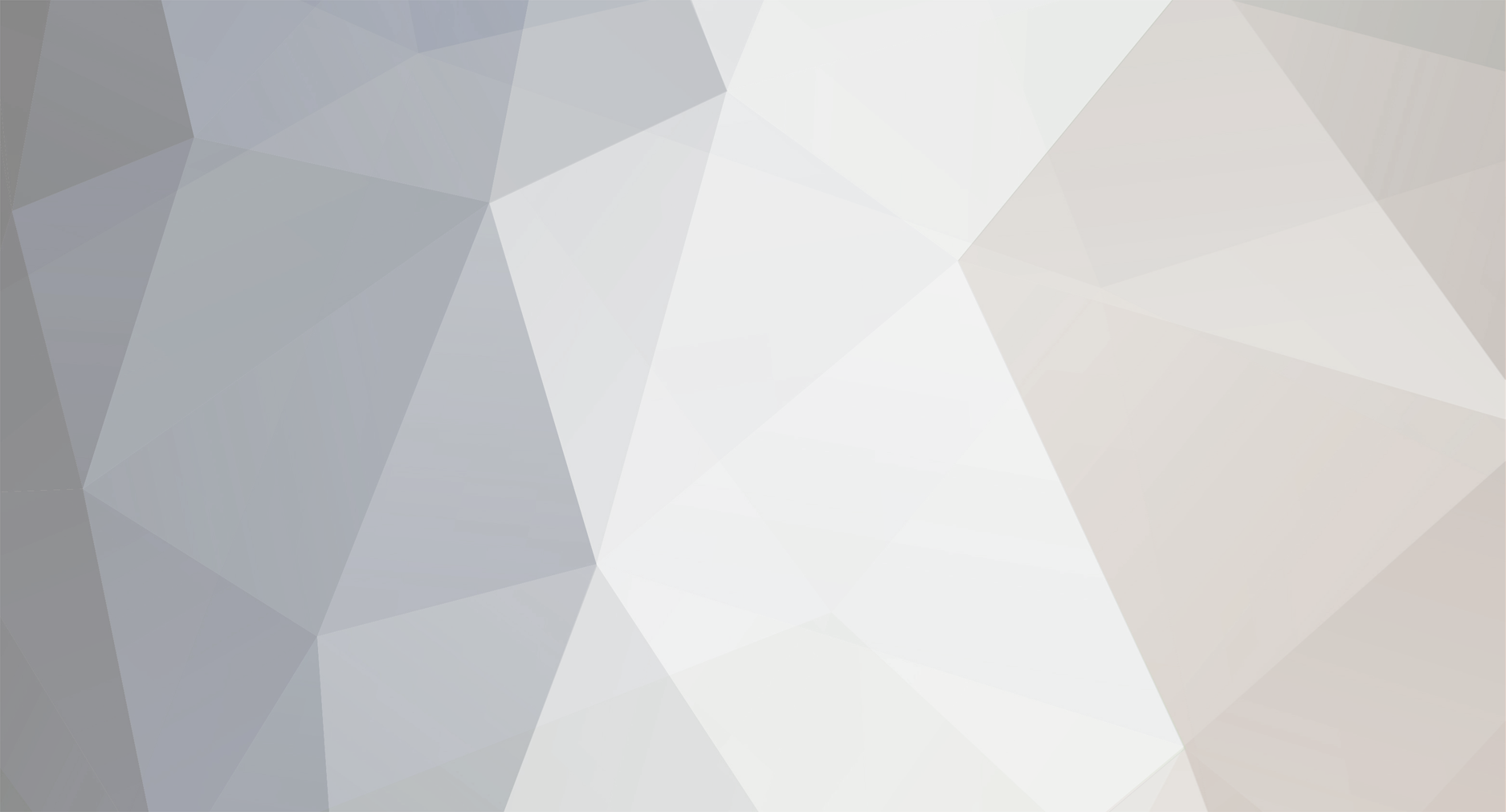
hansford
Members-
Posts
562 -
Joined
-
Last visited
-
Days Won
4
Everything posted by hansford
-
For absolutely no purposeful reason that I can think of.
-
That's a point - I take it for granted all pages are under session management. If you forget to restart a session on some page = you just lost your session. As Mac_gyver pointed out - the definitive way would be to store the attempts in the db. Just remember to delete this data when no longer needed or else everyone who makes a typo on login will be forever redirected to the signup page lol
-
You don't even have to keep track of some temp name - the session id will be unique for that user and the attempts will only pertain to that user's session.
-
When a user attempts to login. session_start(); // attempt to authenticate user with login // if login fails that's strike one $_SESSION['attempts'] += 1; if($_SESSION['attempts'] >= 3) { // you're being redirected to crack some rocks } else { // congrats you made it! }
-
On a side note, there is usually a login link and sign up link which most people understand these days. If they keep attempting to login and failing...they should be able to click the forgot username/password link and get a hint or temp password emailed to them. A session will expire, but depending how long a cookie is set, they could forever be going to the sign up page even though they are just forgetful members. Let them try to login all day if they want because eventually they will: A) remember their login details B) click the link sign up link C) click the forgot username/password link
-
I wouldn't attempt to store the data in a cookie. I would use something like $_SESSION['temp_username']['attempts'] to keep track of how many attempts. At the beginning of your script just check this value and redirect accordingly.
-
This recommendation will save you countless hours and you can drag the code over and easily apply it to every client you have. A simple example: <?php require('common_functions.php'); ?> <html> <head> </head> <body> <?php $dropdown_to_add = get_state_dropdown_menu('menu_name'); echo "<select name=\"menu_name\">\r\n"; foreach($dropdown_to_add as $name => $value) { echo "<option name=\"$name\" value=\"$value\">$value</>\r\n"; } echo "</select>\r\n"; ?> Anyways...you can create generic functions to return an array of the data you need or they can even return the html with the data, name etc. just passing it arguments of what you want.
-
converting strings from foreach into a single array
hansford replied to tarquino's topic in PHP Coding Help
Difficult to determine what you're after unless you post some code. There are many ways to convert strings into arrays. The whole string or just words/parts of the string. Post some code and a clear explanation of what you're trying to accomplish and let's get the party started! $string = "This is a string, whatever"; $args = explode(' ', $string); print_r( $args ); // output: Array ( [0] => This [1] => is [2] => a [3] => string, [4] => whatever ) $string = "Sentence number one. Sentence number two. Sentence number 3."; $args = explode('.', $string); $sentence_array = array(); foreach($args as $sentence) { if($sentence.length < 3) { continue; } $sentence_array[] = trim($sentence) . '.'; } print_r($sentence_array); // output: Array ( [0] => Sentence number one. [1] => Sentence number two. [2] => Sentence number 3. ) -
Pay yourself with the challenge and reward of coding it yourself. Your friends will be amazed, want to borrow money and drop off all of their old, porn and malware affected machines at your doorstep for you to fix. The hot neighbor girl will drop off her....computer as well. Mac_gyver and those guys will be much more willing to help if they see you've put in some effort first (posting your effort thus far - the code you've written/attempted) <?php // set up database variables or import them from config file $dbname = "database_name"; $user = "super_developer"; $pass = "man_o_steele"; $condiments_selected = array(); if( isset($_POST['condiments']) ) { foreach($_POST['condiments'] as $condiment) { $condiments_selected[] = $condiment; } // create query $query = 'INSERT INTO tablename(column_name_1, column_name_2, column_name_3) VALUES(:choice1, :choice2, :choice3)'; // query check // echo $query; // fire up the database...vrrrm! vrrrm! try { $con = new PDO("mysql:host=localhost;dbname=$dbname", $user, $pass, array(PDO::MYSQL_ATTR_INIT_COMMAND => "SET NAMES utf8")); con->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); $stm = $con->prepare( $query ); } catch(PDOException( $e )) { echo($e->getMessage()); } $stm->bindValue(':choice1', $condiments_selected[0]); $stm->bindValue(':choice2', $condiments_selected[1]); $stm->bindValue(':choice3', $condiments_selected[2]); $stm->execute(); } // end if( isset($_POST['condiments'] )
-
Assuming I understand the question...you have members who refer other people who themselves become members.. You have a "Members" table which holds the member ID's and date & time of when they became members. Members ------------------ Member_Id name email etc etc Date_Time You have the "Referrals" table which holds the Member Id of the now member and the Referrer Id of who referred them and the date & time of when they were referred or actually signed up. Referrals ------------------- Member_id email etc etc Referer_Id Date_Time You can traverse by following a member -> any members that have a row/s in the referral table -> RECURSION I'll step aside and see what the senior members have to say. It appears like a recursion problem to me at first glance.
-
Live server not displaying the same as local WAMP
hansford replied to travisco87's topic in PHP Coding Help
Congratulation Travisco87 - you kept working the problem. We can only assist with what we see code-wise. We cannot see your environment and anything you don't show us. You have a good future because you keep working the problem regardless. -
Risks of allowing users to upload files to the server
hansford replied to NotionCommotion's topic in PHP Coding Help
You know you're getting some good information when you get two experienced developers going back and forth. Soak it up peeps. Programmers, in my experience, rarely agree. However, there is more than one way to approach a problem and come to a suitable solution. Many times, a "right" answer cannot be necessarily determined in programming. It's not a black and white science. You can run tests a thousand times on a piece of code and get the correct testing answer, but still have serious flaws in your logic that will cause it to fail given such and such data. The worst thing a new programmer can do is stick steady to the first way they learned how to accomplish a task. It feels good to see results, but dig deeper and see how others are tackling the problem. Look into best practices at the time. If you post to the board and several people are telling you such and such is the wrong way to do something - this should be a clue that maybe it's the wrong approach. Having a healthy ego is good, but being stubborn will only keep you stuck as a mediocre programmer at best - at worst, a poor programmer who has never been challenged and only thinks they are good. A good programmer never stops progressing and continues to grow and learn. -
Live server not displaying the same as local WAMP
hansford replied to travisco87's topic in PHP Coding Help
You just found your answer from validation. <input id="newsbutton" type="submit" name="Submit" value="Signup"> </ul> </form> You can't put a submit button in an Unordered list as a child element as it is expecting a list element. Place it after the Unordered list. -
I'll second that vote. PHPMailer is very reliable and maintained code that will assist you with many client needs down the road. As far as setting PHP up on your computer. Wamp or Xampp is the way to go. You'll have to remember to stop the Windows IIS server if your using a Windows machine when those are running or they will clash. http://www.wampserver.com/en/
-
Data is not inserted to database after submit
hansford replied to osherdo's topic in PHP Coding Help
FYI - I find that named parameters are easier to keep track of and match to the columns your inserting into the database. This is especially true when you have many columns to fill. I name the parameter similar to the real column name, so it's easier to track down when you mistakenly leave out one and you get an Sql error letting you know that your parameter count doesn't match your column count. -
Here's something to get you started. If I read your post correct you wanted a multiple select drop-down. If not then remove the "multiple" tag from the select and the value the user selected won't be in an array, but in the $_POST['condiments'] value itself. HTML <form name="form1" method="post" action="process_form.php"> <select name="condiments[]" multiple> <option value="olives">Olives</option> <option value="pickles">Pickles</option> <option value="onions">Onions</option> </select> <input type="submit" value="submit"> </form> PHP <?php if( isset($_POST['condiments']) ) { $condiments_selected = array(); foreach($_POST['condiments'] as $condiment) { $condiments_selected[] = $condiment; } // here you can build your sql statement to insert into database } ?> Hopefully others will chime in on the database insert part - if not, I'll post that part later as well.
-
Space - depends on what they are uploading and how many developers are storing there and how much available storage space you have. If it's just plain code and other text files then a decent server space should be able to accommodate tons of these types of zips. Just give them each there own file space within a master file. Have a cron job that runs once a month and mails you the results of the master file and then each developers file size. Video and other heavy files are what can eat up server space quickly.
-
Why are you arguing and resisting what these senior level members are telling you? You're logic makes no sense and they have pointed out why a switch is not the solution in this instance. They aren't out to steer you in the wrong direction - they wouldn't waste their valuable time if that were the case. They are here to help you. I have been programming for years and I still learn from them.
-
I haven't used this old deprecated code in years, but I'm going to try and take a crack at it off the top of my head. $result = mysql_query($sql); $likecount = mysql_num_rows($result); while($row = mysql_fetch_array($result)) { $status = $row['stream_status']; echo $status . 'Likes: ' . $likecount . '<br /><br />'; } Learn the new stuff because we can assist you better and because, one day, you're code will magically just stop working on any server. And that time is coming faster than you think.
-
Data is not inserted to database after submit
hansford replied to osherdo's topic in PHP Coding Help
Which button is submitting the form - the one actually in the form or the one outside of the form. Does the code following this condition ever get executed? if (isset($_POST['SubmitDetails'])) { put an echo right after just to see. Better yet - loop through what values are actually in the $_POST array and echo them out. foreach($_POST as $post) { echo $post . "<br />"; } -
I put all files like that in a password protected directory. When I have to do site maintenance that requires a site be down for a specified period, I redirect all traffic to the "Scheduled Maintenance" page except my own IP address. And as mentioned, any directory or file that you don't want published to the world should be a noindex in the robots.txt file.
-
Is there a reason you need to change locations. Why not just require that file since the current file is apparently done processing. require('install/index.php');
-
I would first check the error logs,as was mentioned earlier, and then work on tackling that php page. I don't see an action url set in your form, which means by default it just submits to the same page. Make sure they are otherwise you need to set the action attribute in your form to where the script is located.
-
Detect whether the page was refreshed
hansford replied to NotionCommotion's topic in PHP Coding Help
Well, you have sessions. You can store the last page name/url in a session variable. Whatever page they visit, this session variable gets updated. Then you will always know what the last page visited was. -
Detect whether the page was refreshed
hansford replied to NotionCommotion's topic in PHP Coding Help
When you load the page check for some cookie value. If it's not there, set some cookie value; If the page gets refreshed it will check for that cookie value again. If it is there, you know the page has been revisited/refreshed. You will have to delete the cookie once it is no longer needed. It will be reset every time they visit that page.