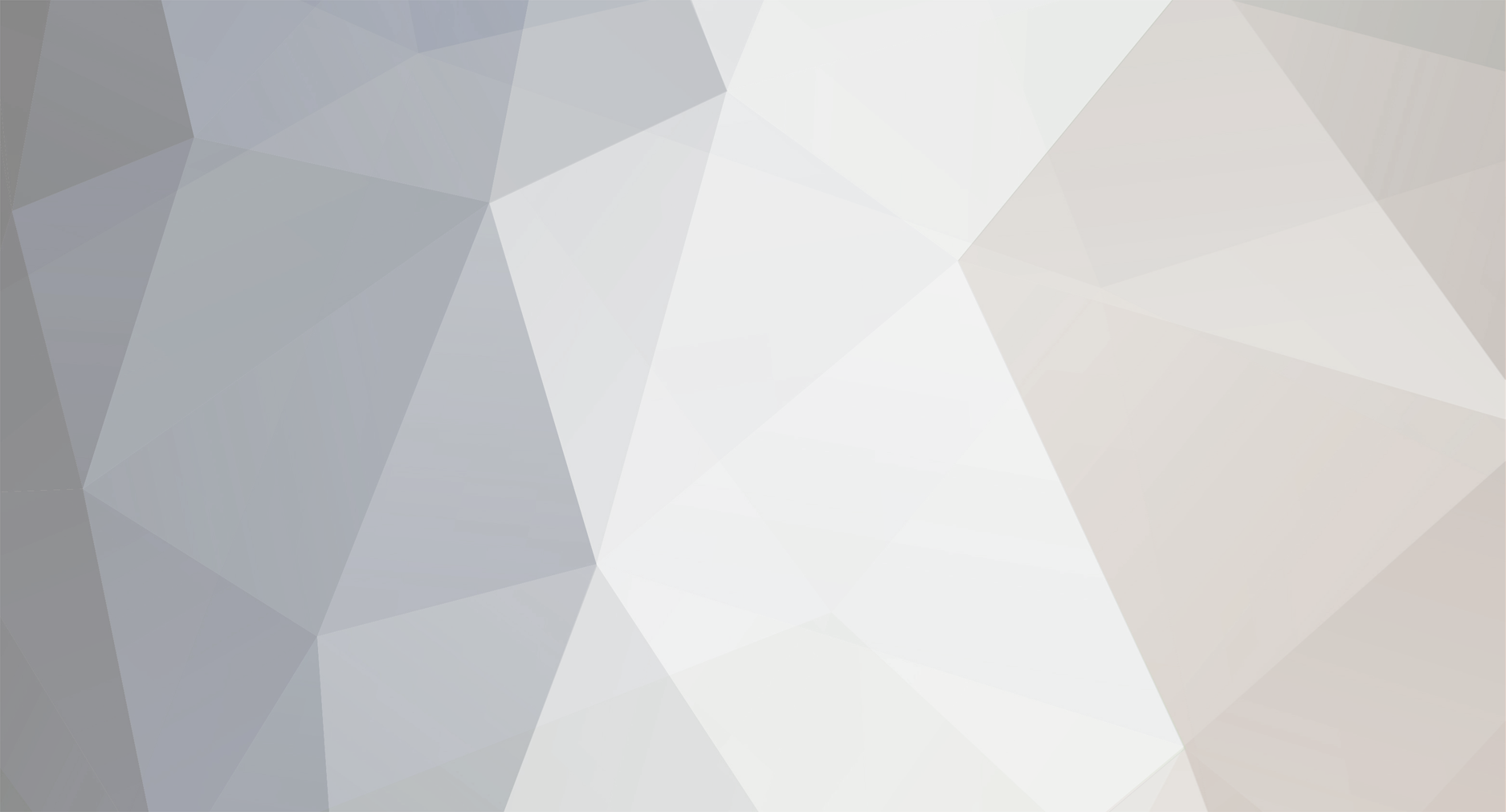
xtopolis
Members-
Posts
1,422 -
Joined
Everything posted by xtopolis
-
Checkboxes when evaluating to true (read: checked) return an array <?php $x = count($_POST['field2']); for($i=0;$i<$x;$i++) { $message .= 'You ordered product ' . $_POST['field2'][$i]; } ?> See return vals of Your Checkboxes
-
If you're asking whether to use MYSQL's PASSWORD() or to use PHP's MD5(), my opinion is this: Why make MYSQL do the work of a scripting language? Mysql is primarily used to store data. It has additional features which allow it to perform specialized queries and computations, but most people will agree that it is better at storing data than crunching numbers. Also, using PHP or any Server-Side-Language will give you more flexibility in your hashing. There are plenty of encryption options out there, you can read a wiki on the best ones to use. MD5 is a common simple one used for 'secure' applications. Things that everyday people like you and I probably use because it's secure enough. If you were designing bank software, I strongly doubt you'd use MD5, more likely SHA2 or WHIRLWIND[i think that was the name]. Make your MD5 more secure: If you have read up on security, you will hear eventually about hackers/crackers using rainbow tables to decrypt MD5 passwords. There is a wiki on this too which was a little beyond me... But the method to beat these rainbow tables was to append/prepend a "salt" to the MD5 string. This means a character/string that you hardcode into your script in order to make your hash data different. Also, you would be careful to do the same to incoming password queries, otherwise they'd always fail. By adding the 'salt' to the MD5, the password 'cat' no longer has the same hash as the word 'cat' would, essentially because the password is really md5('salt + cat'). You might think it's not really saving anything to add a word to your hash, since they might crack your word, but in reality changing 1 character of an MD5 input changes the hash significantly.
-
My mistake TLG, I saw the $_POST['something'] and didn't notice it was being reassigned to the $_POST var, thought it was a straight input. BrianM, you don't need tinytext, varchar(255) will do fine. Md5 actually returns 33 chars.
-
Your md5($password) is returning a string that is longer than 30 characters. You set a limit of 30 characters in your mysql table. Adjust the table to accommodate more characters in the password column. (Why not just go with 255 since you are hashing the values anyway)? Also, read your error message because it told you what was wrong before I did ** I disagree with "The Little Guy". It is considered bad practice to store plain passwords, it comes down to a liability issue. This is up to you whether you want to or not. Everyone has their own opinion. **
-
Just make sure the scripts that people enter into your forms are escaped correctly. Also make sure they are presented/escaped? correctly when shown on the page. Look into things like mysql_real_escape_string, htmlentities, html_specialchars, as well as str_replace. That should get you started.
-
http://www.w3schools.com/Css/pr_pos_overflow.asp div#urDiv { overflow: scroll; } You may have to worry about text wrap if you only want it to scroll vertically.
-
[SOLVED] Question about retrieving database information back into forms
xtopolis replied to lonewolf217's topic in HTML Help
http://www.w3schools.com/TAGS/tag_option.asp You assign the <option> tag to have a standalone attribute of SELECTED.. <option value="cat" SELECTED>Cat</option> How you get the database value to match the appropriate option tag is another story. I have the same problem as you do, how do I 'easily' retrieve and show the selected option from a stored value. One way would be have asp write out the option tags for you and do a check {if (current option'ss value == stored database value) .. write <option value="somevalue" SELECTED> .. else .. write <option value="somevalue">} (you may hardcode an array of possible values and have it print the array) I do not know ASP, but hopefully you understand the pseudo code. Also, if there is an easier way you find or someone else does, tell me, I'd like to know as well! -
I found a 'similar' article relating to <button> tags for safari... but it's from 2006 Here You should debug and test that when you select NO (even though it should be disabled) that it is actually getting submitted. This means that maybe it is disabled functionally, but it might have the same visual bug where it doesn't show up as disabled. You test this by getting the value of q2 after submitting the form when q2_no should be disabled. If it returns the value of 'no', then it doesn't disable... if it returns false or null then it is disabled.. but graphically shows incorrectly. (which at that point you would either remove it completely using DOM, or add a style to it to grey it out and hope people get the picture.) Hope this helps.
-
Cookies or sessions. You could probably use javascript for it. PHP sessions might be a bit much just for saving display states, but, still a valid option.
-
I'm looking at in FF3 ... don't really notice it. If you are using FF as well, it may be the slight movement that appears because the scroll bar comes up (the content is longer than the main screen). Sorry if I'm missing it, I'm picky as well, but don't notice it.
-
Do not try to secure the URL, try securing the output data. Make it so someone has to be logged in in order to see the data by use of sessions and authentication with a database. It depends how secure you need your data to be. Very secure, sessions + database auth. Not very secure, single password hard coded into the page. Anything you might try to do the url can be reverse engineered, so that is why I do not recommend trying to secure it.
-
a:hover { color: blue; } You can modify the "a" properties anyway you like. a:link, a:visited, a:hover, a:active Although, if I recall, you need to define them in a certain order... Not sure.
-
<?php echo count($array); ?> You can also assign it to a variable, good to use in for loops. <?php var $x = count($array); for($i=0;$i<$x;$i++) ..... as oppossed to : for($i=0;$i<count($array);$i++) ?> saves having to do the math for the same thing x number of times.
-
Depends on what your code is... If that picture represents a bunch of <td></td>s next to each other, you could probably just apply a border to the table only. If those are all tables next to each[not the best idea], you could have table { border: 1px solid black; } table.nRB { border-right: 0px; } table.nLB { border-left: 0px; } just class stack the inside tables , and add the appropriate class to the first and last. <table name="table1" class="nRB"><table name="table2" class="nLB nRB"> .. .etc same idea for if they are TDs.
-
Changing an ajax-triggered field in Firefox doesn't work properly
xtopolis replied to Debbie-Leigh's topic in Javascript Help
We would need some relevant code. Are you passing element IDs or using event values? -
Example So, I spent about 1 hour writing and figuring this out because I wanted to know too, lol. I used the following: 1) One hidden and disabled <input> field. 2) onfocus, pass the current value to the hidden/disabled input value 3) onblur, validate (i used a regular expression) , if it fails, replace the value with the one stored in the hidden/disabled It should accomplish the basics of what you want, enjoy. <html> <head> <style type="text/css"> input#hide { display: none; } </style> <script type="text/javascript"> function MouseEvent(e) { if(e.target) { this.target = e.target; }else{ this.target = e.srcElement; } } // mouseevent function clearField(e,tID) { var e = new MouseEvent(e); var y = e.target; var x = document.getElementById(tID); x.value = y.value; y.value = ''; return false; } function checkField(e,tID) { var e = new MouseEvent(e); var x = document.getElementById(tID); var y = /\w{1,}/.test(e.target.value); if(!y) { e.target.value = x.value; } return false; } </script> </head> <body> <form name="myForm"> <input type="text" name="fn" value="Firstname" onfocus="return clearField(event,'hide')" onblur="return checkField(event,'hide')" /> <input id="hide" value="Test" DISABLED /> </form> </body> </html>
-
<?php echo '<meta http-equiv="refresh" content="0;url=http://www.someurl.com/" />'; exit(); ?> This can be outputed at anytime and will attempt to redirect the user to someurl. It's best to put exit(); after calling this so that a non regular browser (ie: PERL masquerading as a browser) will not get any additional info.
-
<?php date_default_timezone_set('America/New_York'); if(isset($_POST['submit'])) { if(!isset($_POST['gui'])) { $_POST['gui'][0] = 'No'; } } //remove these braces } and { below, leave the other stuff alone #### { //these look out of place ####### $date = date('m-d-Y'); $time = date("g:i a"); ?> <?php // $gui = $_POST['gui']; <-- old way $gui = $_POST['gui'][0];//dont forget it's now an array index // $killspam = $_POST['killspam']; <-- old way $killspam = $_POST['killspam'][0]; //dont forget it's now an array index ?>
-
The checkboxes won't be set at all if they are not checked. You have to do a if(isset($_POST['gui'])) { //yes, they have a value }else{ //no, make them have a diff value; } Consider this code: show as an example here: http://xtopolis.com/z_phpfreaks/checkbox/checkbox.php <?php if(isset($_POST['sub'])) { echo '<b>POST BEFORE DETERMINING CHECKBOXES</b><br />'; print_r($_POST); if(!isset($_POST['food'])) { $_POST['food'][0] = 'no'; } echo '<br />Post after checking if checkbox was set<br />'; print_r($_POST); echo '<br />Do I want chicken? <b>' . $_POST['food'][0] . '</b>'; }else{ ?> <form method="post"> Chicken ? <input type="checkbox" name="food[]" value="Yes" /> <input type="submit" name="sub" /> </form> <?php } ?> <br /><a href='checkbox.php'>Reload</a> Following this example and putting it in your code, you should get the results you want. Let me know of your results.
-
[SOLVED] First time connecting to mysql database: PHP error
xtopolis replied to NovaArgon's topic in PHP Coding Help
It can't find the include file. Make sure the path you supply it "includes/connect.php" is referenced correctly to the location of the script running it. A Temporary test might be to supply it the FQDN , like include("http://www.tarantuladatabase.com/path/to/connect.php"); to see if it works, then if it does, work on getting the "../includes/connect.php" type path correct. Solve that first and the mysql stuff should go away. -
You can try changing DISABLED to READONLY. I don't think DISABLED values get $_POSTed. Then change your checkbox name='s to this: for Gui: <input type="checkbox" name="gui[]" value="Yes"> for killspam: <input type="checlbox name="killspam[]" value="Yes"> The only thing I changed on them was adding [] to the end of their name. Read this page to find out why: http://www.tizag.com/phpT/examples/formex.php/ and click continue on that page at the bottom to see the example code. That should allow you to read those 3 values. Let me know if this helps. Gl.
-
http://www.mediacollege.com/internet/javascript/page/scroll.html window.scrollBy(0,50); // horizontal and vertical scroll increments A thought of how it could be done: -Image on screen, with mousedown and mouseup event. -Mousedown (records start position of clientx/y) -Mouseup takes the end position of clientx/y) From there you could determine how much the mouse moved and make it a ratio to your page height/length and apply the window.scroll. However I am not sure how to apply this to a circular method.
-
No. As long as the submit button is inside the <form></form> tags, it can be ahead of any fields afaik.
-
http://www.ampsoft.net/webdesign-l/image-button.html Go here to see the CSS you need. <form action="http://www.google.com/search" method="get"> <input type="Text" name="q" size="45"> <button id="replacement-1" type="submit">Search with Google</button> <!-- <div><button style="display:block" id="replacement-1" type="submit">Search with Google</button></div> --> </form> <h4>Using padding-top for image replacement:</h4> <form action="http://www.google.com/search" method="get"> <input type="Text" name="q" size="45"> <button id="replacement-2" type="submit">Search with Google</button> </form>
-
That depends, will the codes expire once they've been used? If one person uses TQ01, can no one else use TQ01 again? Either way, first if the determined format is TQ + #(up to 4#s), you would use a regular expression to match. Check this site out for a decent regular expression explanation. I tend to favor preg_ style btw. http://www.webcheatsheet.com/php/regular_expressions.php <?php $code = $_GET['value'];// YOU WOULD WANT TO FURTHER VALIDATE/TYPE SAFE THIS VALUE $pattern = '/^TQ([0-9]{1,4})/'; //This pattern looks for: "Starts with "TQ" "Followed by 1 to 4 #s" only. It is case sensitive. //To make it insensitive (TQ == tq) add the letter "i" at the end $pattern = '/^TQ([0-9]{1,4})/i'; if(preg_match($pattern,$code)) { //Do something more to check code } ?> If codes are reusable, you could probably just discount the price right there. If they get burnt right after use, you would store those in a database after successful entry. And in the //do something more to check section, you would create a query looking for if that code already exists in the database. If yes, you would error : "code has already been entered, please enter a different one", otherwise you would write it to the database, and apply the discount.