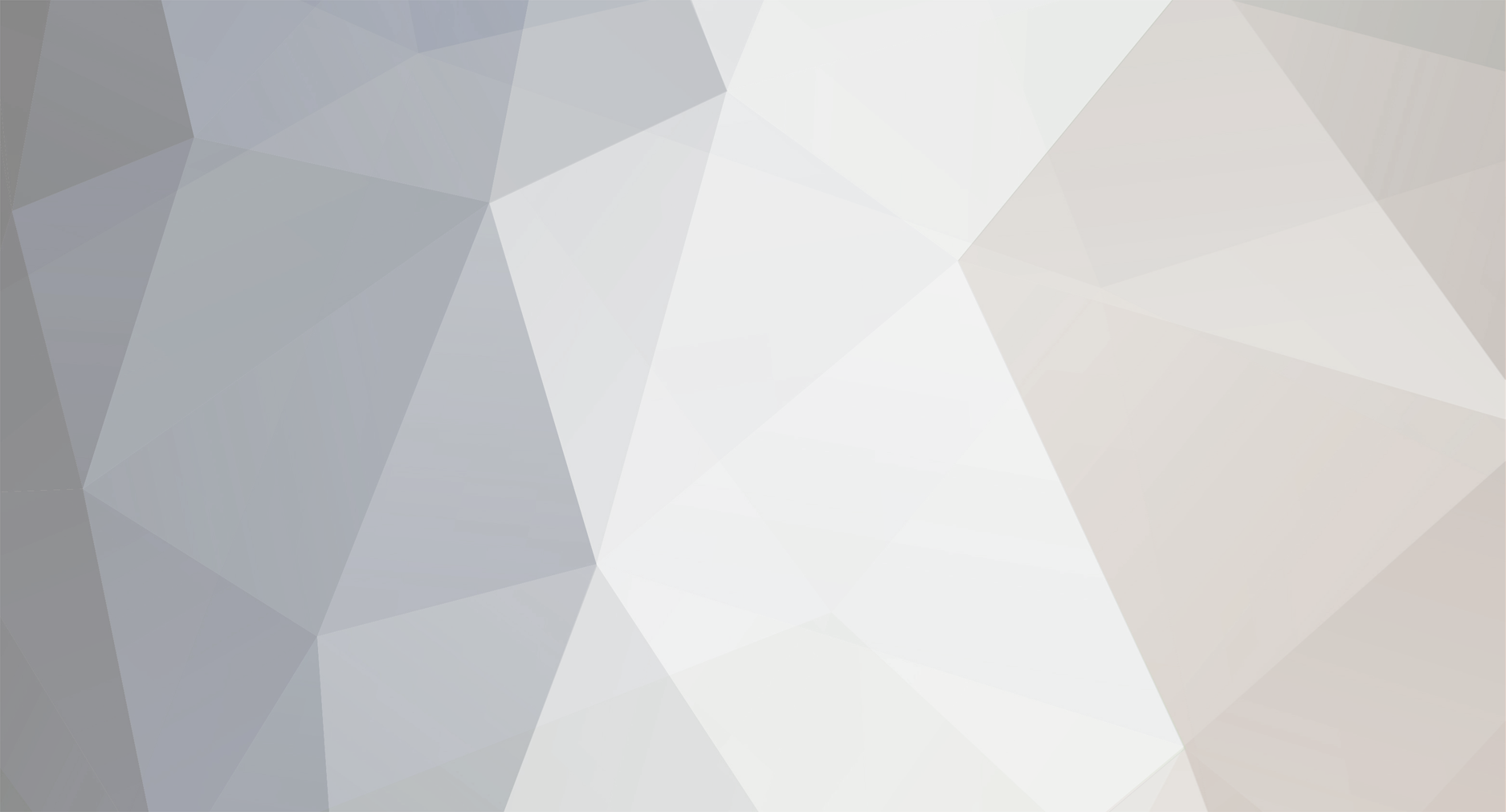
oni-kun
Members-
Posts
1,984 -
Joined
-
Last visited
-
Days Won
1
Everything posted by oni-kun
-
If allow_fopen is on in your PHP.ini settings then you can do something simple like this: $url = file_get_content($url); $logo = preg_match('/<img src=\"\"..../', $contents); And grab it from there, you'll have to learn your own regex though.
-
Create a table to list users who voted, with the columns being their IP and the ID of the poll they voted on. Then you can do this or whatever: $pollID = 4; //Whatever poll it's on. $checkIP = mysql_num_rows(mysql_query("SELECT * FROM voted WHERE ip='".$_SERVER['REMOTE_ADDR']."' AND poll = '$pollID'")); if ($checkIP != 0) { print 'You have already voted on this poll!'; } else { //Do poll.. }
-
Mmm yes, I came a little too late for the C64 era but it was sure fun playing with my DOS console and C64 emulators later on, I quite liked the simplicity of interactivity of the languages. It's why I started VB.NET (when 12/13), which is MS's upped version of well, Visual BASIC, so it was fun and rewarding to make simple console programs and alike. When I first started PHP I hated SQL, It looked ugly and I've seen 300 queries per page being run on simple games and things, I tended to "boycott" it by writing programs purely based on sessions or flatfiles (which were indeed faster), but then I slowly looked at how simple the ..er.. structured queries were and used it from then on! @ the POKEing, I didn't memorize any of that but it was neat to POKE some predefined spaces (which edit colours, or show credits etc)
-
ob_buffer is gone, but i'm not getting errors.....
oni-kun replied to AlexGrim's topic in PHP Coding Help
Do you even know what output buffering is? Make sure error reporting is on at the beginning of your scripts and check again. ini_set('display_errors',1); error_reporting(E_ALL|E_STRICT); You are most likely not sending a problematic header after content if you turn caching off and check this. -
When I looked at the code I didn't see you did it twice. It's always good to check the variables at the beginning (in my example, the isset on $_POST['number']) as you can avoid running ALL that code unless they submit the form. If you look, it nicely goes to the ELSE statement and asks for the number, not running any of the code that doesn't need to be ran.
-
Your code is full of many errors and typos, I put an action on the <form> element (as required for POST) and put your code in a proper IF statement: <html> <head> <title>Array Finder</basic> </head> <body> <h2>Geuss all the numbers in the array!</h2> <br /> <form action="<?php print $_SERVER['SCRIPT_NAME']; ?>" method="POST"> <input name="number" type="input"> </input> <input name="submit" type="submit"> </input> </form> <br /> <?php if (isset($_POST['number'])) { $array1 = array(5,9,10,45,98,101); $maxArray = max($array1); $minArray = min($array1); $findNumber = $_POST['number']; echo "<br />"; if(in_array($findNumber, $array1) == true) { echo "Yes, that number is in the array." . "<br />"; } else { echo "No, that number is not in the array." . "<br />"; } if((max($array1) == $findNumber)) { echo "That is the largest number in the array." . "<br />"; } else { echo "No, that is not the largest number in the array" . "<br />"; } if((min($array1) == $findNumber)) { echo "Yes, that is the smallest number in the array." . "<br />"; } else { echo " No, that is not the smallest number in the array." . "<br />"; } } else { echo "Please enter a number." . "<br />"; } ?> </body> </html> That should fix your problem, it works.
-
That fills in a bunch, I coulda sworn my dejavu about this being around was correct, thanks.
-
It's pointless to list code without you looking through the documentation or examples first on how to handle these things. If you're wanting exact sizes, Calculate the aspect ratio: $aspect = ($width / $height); $towidth = (400 / $aspect); Therefor, the new width will be exactly the same proportion to the height, but the height will always be 400. There'll be no distortion.
-
Single quotes will write the dollar symbol without needing for escaping. You can do this as well: $content = "Some content." $variable = "\$variable = $content" And as for your permissions, it's as simple as opening up your FTP client and CHMODding the file/folder to 755/777 permissions to be able to write to the folder.
-
I've always wanted to know how many people have got an interest in the very beginning. I've coded for VB.NET/console programming for roughly a year designing random applications when I was younger, but as I became more interested a few years ago in web development a good friend of mine was going to university for web programming, PHP. I gave up on it when I first tried, but once I got the hang of it I became great ever since, I purchased my own site and started having fun developing many many random projects over time, such as having fun with GD to writing spiders. Where/when did you begin, and with what languages?
-
If your server has no SMTP logs then you're most likely using a shared host, if so then records sometimes need to be set or changed for proper use of PHP's mail function from the host itself. I'd recommend pinging your server (port 26 usually) over time and see if it's reachable, such as your MX records, but you really should ask your host to check if everything is alright on the record end.
-
The ELSE is fine. Your code seems to be correct (as long as mysite.com is replaced with your actual site in the headers) and should send atleast at the software level. The best chance of your e-mails not sending is either the SMTP server has error authenticating the headers, or is/was not reachable at the time it is sent. Try looking in logs.
-
It'll always fail. You don't have $checkresult defined as well. $checkresult = mysql_query($query) or die('Cannot execute query'); I believe you wanted.
-
You're still not testing to see if $_POST['...'] exists. if (isset($_POST['number'])) { $findNumber = $_POST['number']; } else { $findNumber = 0; //Or whatever you wish to do, such as exit() or trigger_error to tell no number is defined. } Also: $findNumber is not defined until later on in the script, you can't access it yet.
-
Just note a Windows system will require \r\n, And as well it has to be within double quotes to parse "\n" else it won't escape into the character @ OP.
-
functions working on http://www but not on http://
oni-kun replied to WeirdMystery's topic in PHP Coding Help
Remove all cache and restart your browser. What error possible 'injection' error can you be seeing? http://pyrohawk.net/board.php?id='\'\'\ Would become: http://pyrohawk.net/board.php?id=\'\\'\\'\ And shouldn't return much, as the DB wouldn't know what record that is, AS LONG AS YOU ARE USING mysql_real_escape_string. -
Don't you mean \n?
-
If you don't have SSH access, you can hit manual install on the PEAR library and plug it in manually to your script's directory.
-
Upon looking more, it looks like it's changed the way the salt is implemented in the Unix DES library, possibly using another modified algarithm. You can look online (user contrib. notes) for samples of this; I'm sure someone else has run into this problem. But you're most likely in a bad place, as you've generated defective/depricated one way hashes.
-
The only method you'll get to achieve compatibility is to batch decrypt the passwords and hash them like they were designed to be in the database. *SQL provides md5() and sha1() commands (without using PHP), as they're standard. If you're wanting to know the exact differences, I've made a textdiff between the two versions here: http://zwap.to/0023g
-
Now that it's actually out, I'll end up downloading a W7 distro if my HD ever fragmented to hell again. I mean some things can't run (especially some .net 1.0/2.2 related programs) on it, but I don't use Windows, so for general gaming it should be better than XP. I'm glad DX10.1 has an actually decent background OS finally. The RC2 (7077 I believe I had) wasn't good at all, there were many GUI errors on my laptop's integrated card, which isn't all that bad. Hope that's all fixed.
-
Why do you want this done? There's no cross browser method for 'any' browser. You could detect the browser and send them to the proper url, or if automated viewsource.php?http://...
-
I agree with Boom.dk, What on earth is encryption used for? And with a salt? Use sha1/md5