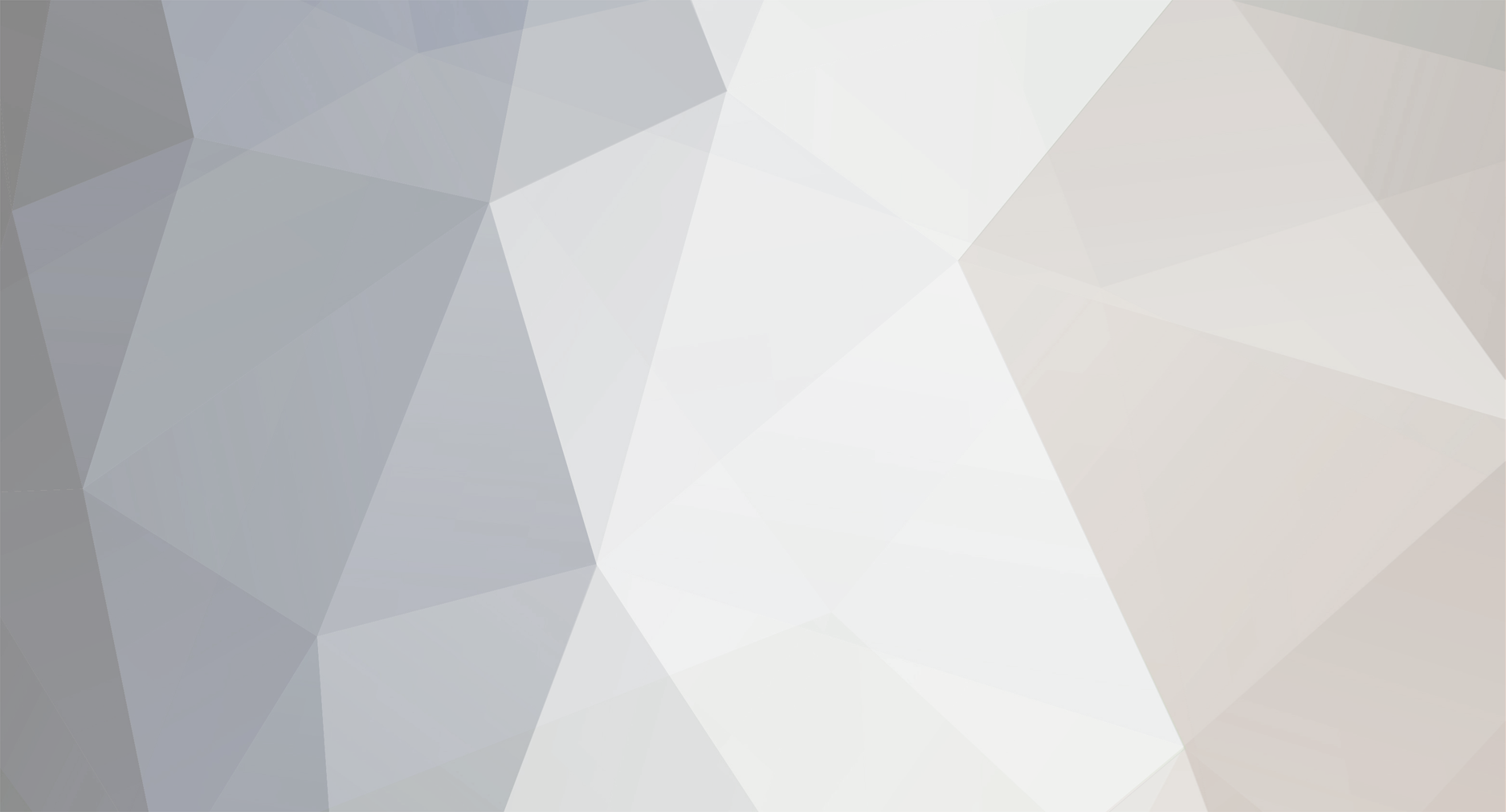
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
You can't do a progress bar with PHP uploads, you'd have to use something like cgi.
-
I suppose you could do something like this: <?php function getRand($low, $high) { $main = Array(); for($i = $low;$i <= $high;$i++) $main = array_merge($main, array_fill(0, $high-$i+1, $i)); return $main[array_rand($main)]; } echo getRand(1, 7);
-
use mysql_real_escape_string() on all input. To remove html you can use strip_tags()
-
You still are missing quotes around $page, since it's not a number. $q = "SELECT * FROM blogs WHERE post_page = '$page' AND post_is_sticky = $is_sticky";
-
Flash fails to connect to PHP Socket when Online!!!
Alex replied to reapermedia's topic in PHP Coding Help
Btw, I reviewed that tutorial, and I found a problem. Because that's an old tutorial this problem wouldn't have occurred when it was made, but now it will. Within the PHP server where a new client is added "array_push($read_sockets, $client)", you'll have to send flash a response telling it that it's a legit response. As a measure of security this was added in like Flash 9.x. After that line add: socket_write($client, '<?xml version="1.0"?><cross-domain-policy><allow-access-from domain="*" to-ports="*"/></cross-domain-policy>' . chr(0x00)); I don't believe this is related to your direct problem posted in this thread, but you would encounter it next. -
Yea, something like this.. <?php $imgs = Array(Array('mariza', 'main.jpg'), Array('anaraquel', 'main.jpg'), Array('joicy', 'main.jpg'), Array('rita', 'main.jpg')); shuffle($imgs); echo "<tr>"; foreach($imgs as $img) { echo '<td><div align="center"><a href="' . $img[0] . '.html" target="_blank"><img src="imagens/' . $img[0] . '/' . $img[1] . '" width="220" height="240" border="0"></a></div></td>'; } echo "</tr>"; Then just add more content to the Array to display more. The thing is, you'll have to edit that so it'll work for adding more rows.
-
You could do something like.. <?php $imgs = Array(Array('mariza', 'main.jpg'), Array('anaraquel', 'main.jpg'), Array('joicy', 'main.jpg'), Array('rita', 'main.jpg')); shuffle($imgs); ?> <tr> <td><div align="center"><a href="<?php echo $imgs[0][0]; ?>.html" target="_blank"><img src="imagens/<?php echo $imgs[0][0] . '/' . $imgs[0][1]; ?>" width="220" height="240" border="0"></a></div></td> <td><div align="center"><a href="<?php echo $imgs[1][0]; ?>.html" target="_blank"><img src="imagens/<?php echo $imgs[1][0] . '/' . $imgs[1][1]; ?>" width="220" height="240" border="0"></a></div></td> <td><div align="center"><a href="<?php echo $imgs[2][0]; ?>.html" target="_blank"><img src="imagens/<?php echo $imgs[2][0] . '/' . $imgs[2][1]; ?>" width="220" height="240" border="0"></a></div></div></td> <td><div align="center"><a href="<?php echo $imgs[3][0]; ?>.html" target="_blank"><img src="imagens/<?php echo $imgs[3][0] . '/' . $imgs[3][1]; ?>" width="220" height="240" border="0"></a></div></td> </tr>
-
Yes, it's possible. You'll have to put the images in an array and randomly place them in the table. You'll have to show us your source for more help.
-
Make sure session_start(); is at the top of every page you want to access the session variables from.
-
$query = "SELECT * FROM quotes ORDER by RAND() LIMIT 1"; $result = mysql_query($query); $row = mysql_fetch_assoc($result); echo $row['quote']; Edit: Also change this: mysql_select_db(db); to mysql_select_db(DATABASE_NAME, $db);
-
[SOLVED] Invalid argument supplied for foreach
Alex replied to heldenbrau's topic in PHP Coding Help
$sortedvotes isn't an array. -
[SOLVED] Calculate (add) all integers from mysql rows
Alex replied to keldorn's topic in PHP Coding Help
There's no need for a loop. $line = mysql_fetch_assoc($Hits); $count = array_sum($line['hits']); -
What editor do you use? (Notepad, Notepad+, etc...)
Alex replied to kratsg's topic in PHP Coding Help
I use Notepad++. -
if($logged['level'] == 'owner' || $logged['level'] == 'head mod' || $logged['level'] == 'admin' || $logged['level'] == 'mod')
-
You could do something really simple like this: $templateObjects = Array('somefile' => Array('{REPLACE_ME1}', '{REPLACE_ME2}')); class Template { private $template; function __construct($title) { $this->template = str_replace('{TITLE}', $title, file_get_contents('template/html/main.html')); } public function setContent($section, $content) { $this->template = str_replace($section, $content, $this->template); return true; } public function display() { echo $this->template; return true; } } function getContent($template, $content) { global $templateObjects; $structure = @file_get_contents('template/html/' . $template . '.html'); if(!$structure) return 'Error: Invalid template id (' . $template . ')'; return str_replace($templateObjects[$template], $content, $structure); } Then to use it: template/html/main.html defining your doctype etc.. everything that will be the same on every page.. <head> <title> {TITLE} </title> <html> <body> {MAIN_CONTENT} </body> </html> template/html/somefile.html This is some file.. {REPLACE_ME1} {REPLACE_ME2} file.php //Include class file $template = new Template('Some title'); $content = getContent('somefile', Array('Hello', 'World')); $template->setContent('{MAIN_CONTENT}', $content); $template->display(); It's just a quick example, should you get started.
-
encrypting $_GET variables - does that make sense?
Alex replied to jeffz2008's topic in PHP Coding Help
It's possible, of course.. But usually not necessary. You probably shouldn't be sending data that's so sensitive it can't be validated through a simple check. I've only ever done this once myself; and it was for something stupid anyway. I was creating an image water-marker, but I didn't want to save a new image, and I didn't want the url of the water-marked image to be public. So I encrypted the URL of the image within the query string for the water-marking file. -
try: <Files "somefile.html"> AddType application/x-httpd-php .html </Files> If that doesn't work you'll have to change some other configuration.
-
You would have to use a meta tag redirect and set it to like 1 second. This is because the JavaScript doesn't send the information to Google Analytics until after/during the page is loading. Which doesn't occur if you're php header('Location:');
-
Btw, you should probably use this: class Image { private $image, $ext, $file; const CENTER = 0, CENTER_LEFT = 1, CENTER_RIGHT = 2, TOP_CENTER = 3, TOP_LEFT = 4, TOP_RIGHT = 5, BOTTOM_CENTER = 6, BOTTOM_LEFT = 7, BOTTOM_RIGHT = 8; public function __construct($im=NULL) { if(empty($im)) return true; $info = @getimagesize($im); $backtrace = debug_backtrace(); if(!$info) return die('<b>Fatal error:</b> \'' . $im . '\' is not a valid image resource on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $ex = explode('/', $info['mime']); $this->ext = $ex[1]; $func = 'imagecreatefrom' . $this->ext; $this->image = @$func($im); $this->file = $im; return true; } public function setImage($im) { $info = @getimagesize($this->file); $backtrace = debug_backtrace(); if(!$info) return die('<b>Fatal error:</b> \'' . $im . '\' is not a valid image resource on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $ex = explode('/', $info['mime']); $this->ext = $ex[1]; $func = 'imagecreatefrom' . $this->ext; $this->image = @$func($im); $this->file = $im; return true; } public function resize($width, $height, $porportional=FALSE, $percent=NULL, $max=NULL) { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->resize, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $info = Array(imagesx($this->image), imagesy($this->image)); if($porportional) { if(empty($max) && empty($percent)) return false; if(empty($max)) { $new_width = $info[0] * ($percent/100); $new_height = $info[1] * ($percent/100); } else { if($info[0] < $max && $info[1] < $max) return false; $new_width = ($info[0] > $info[1]) ? $max : ($info[0]/$info[1]) * $max; $new_height = ($info[0] > $info[1]) ? ($info[1]/$info[0]) * $max : $max; } } else { $new_width = $width; $new_height = $height; } $new_image = imagecreatetruecolor($new_width, $new_height); imagecopyresampled($new_image, $this->image, 0, 0, 0, 0, $new_width, $new_height, $info[0], $info[1]); $this->image = $new_image; return true; } public function crop($width, $height, $x, $y, $position=NULL) { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->crop, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $info = Array(imagesx($this->image), imagesy($this->image)); if($width > $info[0] || $height > $info[1]) return false; if(!empty($position) && $position >= 0 && $position <= { switch($position) { case CENTER: $x = ($info[0] - $width)/2; $y = ($info[1] - $height)/2; break; case CENTER_LEFT: $x = 0; $y = ($info[1] - $height)/2; break; case CENTER_RIGHT: $x = ($info[0] - $height); $y = ($info[1] - $height)/2; break; case TOP_CENTER: $x = ($info[0] - $width)/2; $y = 0; break; case TOP_LEFT: $x = 0; $y = 0; break; case TOP_RIGHT: $x = ($info[0] - $width); $y = 0; break; case BOTTOM_CENTER: $x = ($info[0] - $width)/2; $y = ($info[1] - $height); break; case BOTTOM_LEFT: $x = 0; $y = ($info[1] - $height); break; case BOTTOM_RIGHT: $x = ($info[0] - $width); $y = ($info[1] - $height); break; default: return false; break; } } $new_image = imagecreatetruecolor($width, $height); imagecopyresampled($new_image, $this->image, 0, 0, $x, $y, $width, $height, $width, $height); $this->image = $new_image; return true; } public function save($loc, $compression=100) { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->save, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $func = 'image' . $this->ext; return $func($this->image, $loc, $compression); } public function output() { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->output, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); header('Content-type: image/' . $this->ext); $func = 'image' . $this->ext; $func($this->image); } } It just reflects the changes and fixes the problem(s) that igance pointed out.
-
How is "->" used? As in, $variable->function(). Newbie here.
Alex replied to teraparsec's topic in PHP Coding Help
Yes, that's all correct. If you wanted to preform another function on $xml you could do something like.. $xml = somefunction($xml); -
Oh, well in that case you should use two tables. You could store it as 1,2,3, but that's a horrible database structure. 1 Table should be Categories, it'll just have an ID field (auto_increment primary key), and the name of it. Then Articles should have a auto_increment primary key (as well), a category_id, and any other information you need. Then you'll just link the two by the category id in the articles table that'll correspond to a row from the Category table.
-
It depends on how complicated things are going to get, and how much flexibility you need. Regardless, your first method is horrible. Why don't you just create a single column named "category", then within that colunm you just enter the name (or id) of that category. Then go from there.
-
How is "->" used? As in, $variable->function(). Newbie here.
Alex replied to teraparsec's topic in PHP Coding Help
That's PHP OOP (Object Oriented Programming). $variable represents an object, and function() is a method of that object. Here's an example: <?php class someClass { private $a; public function someClass() // Constructor, called once a new object is made { $this->a = 'Hello World'; } public function displayA() { echo $this->a; } } $variable = new someClass(); $variable->displayA(); // Hello World Look here There are plenty of examples. -
This is often caused by forgetting to close a curly brace }. Maybe somewhere higher in your code one isn't being closed properly.