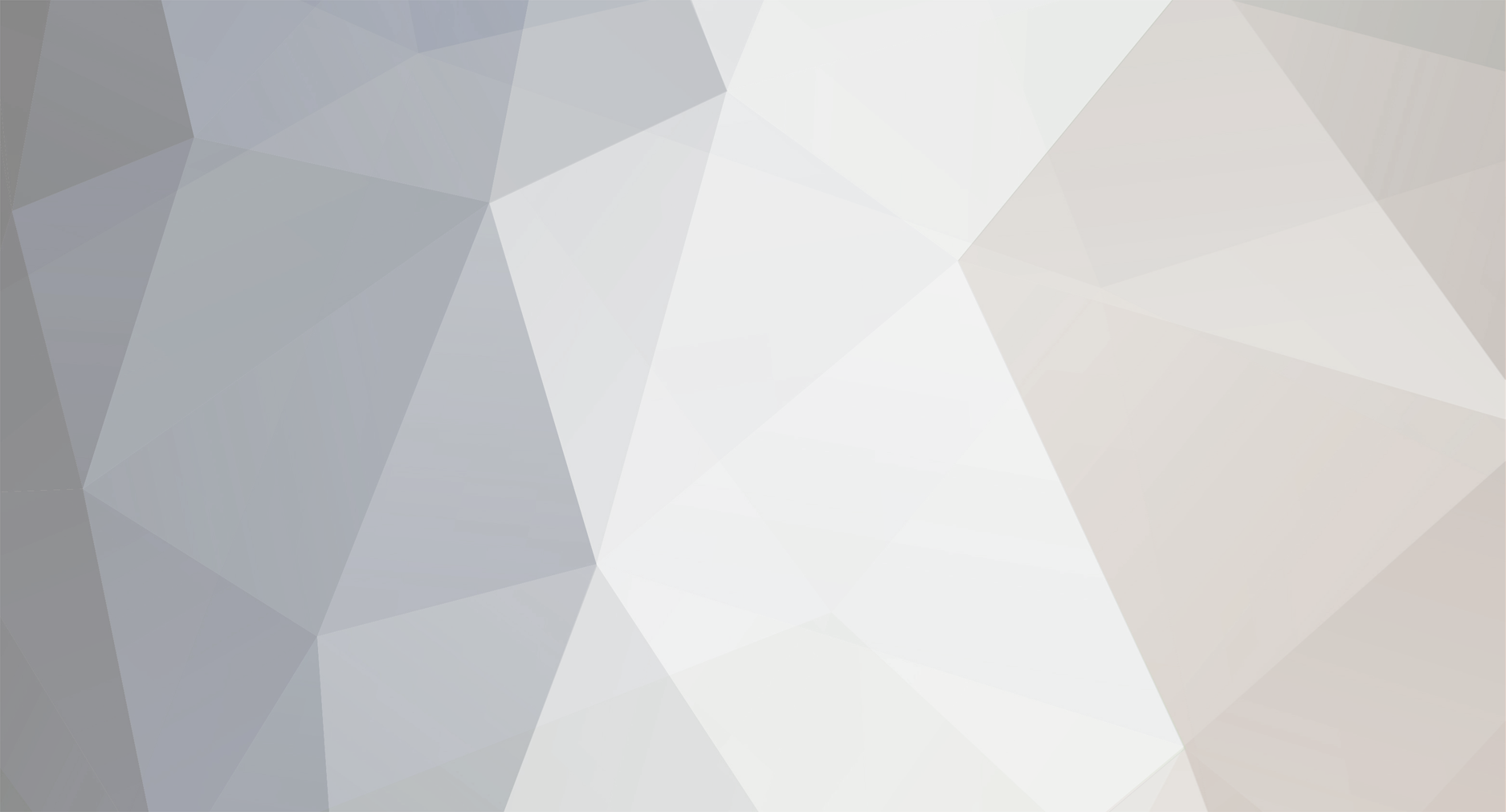
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
You have not asked a question. However, you want to use a JOIN to do this, using the column that is common between the two tables SELECT list, country, type, name, tier FROM tanks JOIN tanks_own ON tanks.name = tanks_own.tanks WHERE tanks_own.player = 'hyster' (I'm not sure what column in "tanks" matches the column "tanks" in "tanks_own", so I took a guess) P.S. When using IN, the sub-query needs to return a single column: SELECT * FROM tanks WHERE tanks IN (SELECT name FROM tanks_own WHERE player = 'hyster' (But the JOIN is probably more efficient.)
-
Make sure the From: email address is a valid email address owned by the mail server sending the email These are basically "headers" to this part of the message. They need to be on separate lines (just as if they were in the mail headers). Also, (I think) there should be a blank line between the last "header" and the content. The message body should look something like this: This is a multi-part message in MIME format. --MIME boundary Content-Type: text/plain; charset="iso-8859-1" Content-Transfer-Encoding: 7bit Plain text message here --MIME boundary Content-Type: text/html; charset="iso-8859-1" Content-Transfer-Encoding: 7bit HTML Message here --MIME boundary-- Check the return value of the mail() function to see if it succeeds or fails. Are you certain the message is not sent? Is it in your spam/junk folder?
-
Check Boxes in a Mysql Loop, transforming into $_POST Data
DavidAM replied to parodyband's topic in PHP Coding Help
The only thing I would add to Barand's post, is the need to validate/escape the "id" values before shoving them into the query. Since they should all be integers, you can add array_map in there: $idlist = join(',', array_map('intval', $_POST['id'])); which will force any bogus values to zero. -
Perhaps something along the lines of this: // Associative array keyed by my Document Types (set each to false to start) $types = array_fill_keys(array('Word','Excel','PDF'), false); // Select all doctypes from the database $sql = 'SELECT doctype FROM documents WHERE doctype IN ("' . implode('","', array_keys($types)) . '")'; // Set the array element to -true- for any doctypes we find $res = mysql_query($sql); while ($row = mysql_fetch_assoc($res)) { $types[$row['doctype']] = true; } I used mysql_* functions because I'm not sure what your database object is; but you get the idea.
-
Actually, some of the elements of the $_SERVER array contain data provided by the browser. Those elements cannot be trusted; i.e. they must be validated and/or escaped, depending on how they are being used. Personally, I wish PHP would split this array out; perhaps: $_SERVER and $_CLIENT or something, so we know which elements come from where. [ PHP Manual - $_SERVER ] And, for the record, I ran into one situation where DOCUMENT_ROOT was not defined correctly. While moving a working website from HOST-A to HOST-B, before changing the DNS records, the scripts were loaded into HOST-B so I could test them (using an IP Address since the DNS was not changed). At this time, DOCUMENT_ROOT created all kinds of problems. When I called support, I was told this was because the directory was in a "temporary" location. I never fully understood, or cared, why. At any rate, once we finalized the setup and transferred the DNS, everything was fine. (Yes, HOST-B was a shared hosting server).
-
That indicates that the query failed. You need to take a look at the query (echo $sql;) and see what is wrong with it. You can also add if (! $res) echo mysql_error(); to get the error message from the database server.
-
also, Use htmlspecialchars to convert HTML into displayable entities.
-
You are overwriting the $varlist each time through that loop, so you are not actually collecting the information on any but the last ID. You are also still running that query in a loop. A better approach would be: // ASSUMING UID IS A NUMBER NOT A STRING $idList = implode(',', $_POST['UID']); $sql = 'SELECT * FROM users WHERE UID in (' . $idList . ')'; $res = mysql_query($sql); $varlist = array(); while ($row = mysql_fetch_assoc($res)) { $varlist[$row['UID']] = $row; } Now you have all of the data in an array $varlist. The key to that array is the UID of the record, and the value of each element is an array containing the database data. By the way, you should not use SELECT *, you should specifically list the fields you actually need. In the body of the document, you are trying to retrieve data from the last query, again. You should just be using the data you already retrieved: foreach ($varlist as $UID => $row) { ... } This all assumes that there will be only one record per UID. If there could be more than one, you need to add another level to the $varlist array, and add another foreach inside the one in the body.
-
You have two separate forms. Only one FORM will be submitted, and only the fields in that one FORM will be submitted.
-
disable_functions doesn't work?
DavidAM replied to kenw232's topic in PHP Installation and Configuration
Is PHP being run as a loaded module or as CGI? As I understand it, settings in the Apache config files (including .htaccess) only work when PHP is being run as a module. Otherwise, you have to put the settings in the php.ini file. -
Newbie needs help w/ sample code regarding Update function
DavidAM replied to bajadulce's topic in MySQL Help
Barand's solution is the quickest most efficient way to handle this. But, I thought I'd explain this problem. The correct syntax is: UPDATE tableName SET columnName = newValue Your query, when the variables are replaced with their values would be (something like): UPDATE cars SET blue = red which is obviously not correct. Also, without a WHERE clause in there, your statement would update every row in the table every time it is called. Furthermore, you need to put quotes around the string values. So, you need something like: $sql = "UPDATE cars SET car_color = '{$row[fav_color]}' WHERE name = '{$row[name]}'"; However, as was said above, doing the update in a loop like that is not necessary, and terribly inefficient. -
A little bit of code would help, too. You could be fighting a scope issue here.
-
Helping Determining how Challenging a Project is
DavidAM replied to guinbRo's topic in Application Design
Your opening statement sounds like you have a single file of UN-normalized data you want to load into an empty normalized database. So I am answering from that perspective. In this situation, I usually create a table matching the import file, with some added columns (in this case) for MarketID, ContactID, EventID, etc. These added columns default to NULL. Then import the CSV file so all of my data is in one place. Then I use a series of SQL statements, directly against the database (phpmyadmin) to review and process the data. First, check the market data: SELECT DISTINCT MarketName FROM ImportData using whatever makes the market data unique. If the result looks reasonable, and returns the expected number of rows, great. If there is a problem, fix the import data and re-import (or fix it in the ImportTable as well), then try again. I fix the source data (if possible) in case I have to drop the import table and start over. Once I'm happy with the data, I insert it into the final table: INSERT INTO MarketData (column, names, here) SELECT DISTINCT column, names, here FROM ImportData Here is where the added columns come in. Once I have inserted the data, they now have a unique ID from the AUTO_INCREMENT column of the final table, so update the import table with this ID (we will need it to do the next level (Contact)). UPDATE ImportTable FROM MarketData SET ImportTable.MarketID = MarketData.ID WHERE ImportTable.MarketName = MarketData.MarketName Then move on to the next level -- Contacts. As I do this, I save every SQL statement that is used, including the ones I use to review the data, along with copious comments on what it does and why. This way, if I have to start over, I can either copy/paste the SQL instead of creating it again; or I can put the conversion SQL in a file and execute the SQL script to do multiple steps quickly. I would not bother to write a PHP script to handle this, unless there is a significant amount of conditional updates. "You can't automate a process that doesn't exist" --- I was told that by a guy I worked with/for many years ago, and it makes sense. Doing this manually in SQL will be faster than trying to write a process to do it, since there will be some trial and error. Once you have the process down, if it will have to be repeated --- because the file comes to you every month or whatever --- then you can automate it and you will know what you are trying to accomplish. Even then, I would likely build a single SQL script file to be run against each new file, rather than write a PHP script.- 1 reply
-
- php
- application design
-
(and 3 more)
Tagged with:
-
encoding issue (?) after ajax post request
DavidAM replied to peppericious's topic in Javascript Help
The Content-Type META tag simply tells the browser what kind of text it is about to receive; it does not magically change the text you send. Your script should send text that matches what you tell the browser. If the data in your database is stored as UTF-8, then you should not need to convert the encoding. But you do need to tell the browser you are sending UTF-8 on the AJAX request. 1) Make sure your database (tables/columns) are defined as UTF-8. 2) Make sure your database connection include file sets the connection to UTF-8 immediately after openning it: $dbc->set_charset("utf8") 3) You should send a header from PHP to indicate UTF-8 text will be sent. header('Content-type: text/html; charset=utf-8'); 4) I have not worked a great deal with non-ASCII text, but the following PHP settings are also of interest in this case. Have a look at the manual book.mbstring mb_internal_encoding('UTF-8'); mb_http_output('UTF-8'); mb_http_input('UTF-8'); mb_language('uni'); mb_regex_encoding('UTF-8'); -
You can format it when you execute the query using the mySql function DATE_FORMAT(). Or you can format it in PHP using date and strtotime echo date('m/d/Y g:i:s A', strtotime($row['DateColumn']));
-
@Jessica --- although you don't deserve it any more than I do. @Backslider At the risk of sounding immodest, I have over 25 years experience in computer programming and consulting. A good part of that spent in database design and administration. I have designed and managed databases that handle hundreds of millions of dollars and millions of barrels of oil, just to name a couple. I know a table scan when I see one, and I've never seen one that I liked. Jessica is no troll, but I think she hit the nail on the head about you. You have clearly demonstrated your ignorance --- and before anyone charges me with name calling, that simply means "lack of knowledge" --- in this area. "CODD Hell" as you called it, is a fairly simple and straight-forward description of the most efficient means of storing and accessing data in a relational environment. If it scares you so bad that you consider normalization "hell", then you need to find someone else to design your database for you, you obviously don't have the ability to handle it. Oh, and by the way, Jessica did not say she knew how to do it. I, on the other hand, know exactly how to do it; a solution that works in edge cases, at that. But I am not going to tell you. Such knowledge, while sometimes necessary (in very rare cases), is too dangerous to place into the hands of a heretic.
-
You are not passing the "case" value to tell the script which value to return. Try $.getJSON("Saturday.php?ajax=true&case=place", function(data) { But, why, oh, WHY are you retrieving all of the data from two tables, just to return one of them. You are wasting a lot of resources: if (isset($_GET['ajax'])) { $case = (isset($_GET['case']) ? $_GET['case'] : false); switch($case) { case 'place': $sql = 'select * from tblRestaurants order by RestName ASC'; break; case 'cuisine': $sql = 'select * from tblCuisines order by CuisineName ASC'; break; } if (isset($sql)) { $res = mysql_query($sql); while ($row = mysql_fetch_assoc($res)) { $date[] = $row; } echo json_encode($data); } die(); } Or something like that. (untested, off the top of my head)
-
To insert into a mySql DATETIME (or TIMESTAMP) column, the value must be formatted as YYYY-MM-DD hh:mm:ss There is no real reason to put this field on the form (unless you want the time that they REQUESTED the form page). When you do the INSERT, you can use the mySql function NOW(): INSERT INTO myTable (UserName, theDate) VALUES ('DavidAM', NOW())
-
Two terms to know: "Copyright" and "Terms of Service" If you are scraping the site to present the data on another site, you are likely violating the Copyright of the site. If you are scraping the site for any reason, you are likely violating the site's Terms of Service. Most of them say that you are not allowed to access the site through any automated method. You need to read the site's policies and be sure that your actions are within the policies. You may want to contact the site administrator and see if they have an RSS feed or an API for people who want to retrieve the data for their own use.
-
With your table structure, in order to execute the query you are asking about, mySql (or any other database server, for that matter) will have to search every one of those 25,000 rows every time (that's called a "table scan" and is really bad for performance). With a normalized database, and the CountryID indexed, the server quickly finds the rows of interest using indexes -- a whole lot faster. For the same reason that PHP used to have magic_quotes, and register_globals; to support lazy/un-knowledgeable programmers! Just because a language supports something, does not mean it is a good way to do it. Also, FIND_IN_SET is useful for SET-type columns. The reason you are here, presumably, is to learn how to accomplish something from someone who knows more than you --- there is nothing wrong with knowing that there are people that know more than you, it is why I came here in the first place (and I still don't think I've caught up with some of the folks here) --- we would be remiss if we did not provide professional-grade advice when we see someone doing something that is considered bad-practice. If you can't or don't want to change your design, you can simply say so --- it would be polite to thank the person for the expert advice, as well --- but there is no reason to attack someone for trying to help.
-
Soo much easier when the requirements are clearly stated. Can we mark this thread solved?
-
Trying to update table using isset() function shows nothing
DavidAM replied to psonawane's topic in PHP Coding Help
I don't know why you chose "update" when you added that line of code. But that line is supposed to be checking to see if the form was submitted. Usually, I use the name of the submit INPUT element there. In your code, that would be "submit". Except that your second post did not include the form that was in the first post, so ... You need to check if one of the fields from the form isset (in the $_POST array) which means the form was submitted, before you do any database updates. When you switched to empty, you effectively reversed the IF test so you should have added NOT -- if (! empty($_POST['submit'])) (However isset() is the way to go.) Note: session_start needs to be called before anything is sent to the browser. By the time you call it you have already sent the HEAD so, your session may not be working properly. For form processing, you generally want to move the processing code above the HTML anyway. It would go something like this: 1. Start a Session (if one is needed) 2. If the form was submitted ... A. Validate the data B. If the data is valid update the database 3. Build the form page Now you can include any error messages from the validation on the page -
Your first post said you wanted to output the user only once. Your most recent post complains that you output the user only once. What is it that you actually want? I'm a little confused about what you are attempting to do. 1) Is follow_user_id the ID of the user being followed or the user doing the following? 2) I would presume that the follow table has two user IDs in it -- the follower and the followee. What are the names of these columns and what does each represent? 3) What are you trying to list? The other users being followed by the logged-in user? Or the other users that are following the logged-in user?
-
You may want to do the same with $headers. As it is, you are adding another set of headers for each subsequent message. If you (later) add something specific to the headers of one email, it will carry to the next.