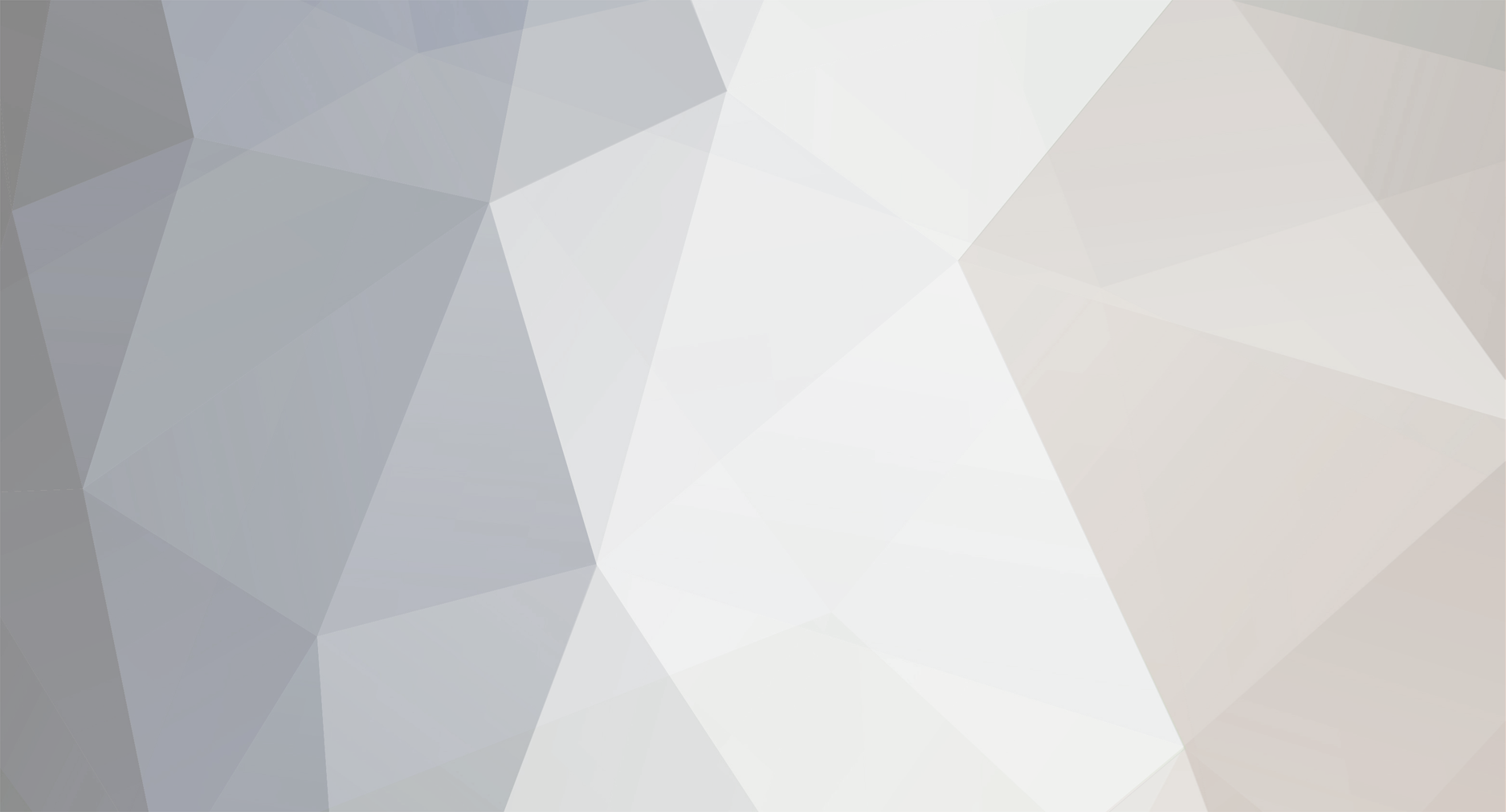
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
copy($_FILES['usr']['name'],$dir); The name element of the FILES array is the basename (filename without the path) of the file that the user uploaded. The tmp_name element is the filename the server used to temporarily store the uploaded file. This temporary file is stored in a directory somewhere on the server (not in your path). The move_uploaded_file function knows where this file is stored, which is one of the reasons you should use that function instead of copy. The chances are very very high that the tmp_name is not the same as the name.
-
1) Check the permissions on the directories you are not getting 2) Show us a list of what you expect and what you get 3) Are any of your directories "hidden" (start with a full-stop ("."))? I'm not sure if glob('*') ignores hidden files or not, seems like I've have trouble with that before. 4) try print_r(glob($path . '*', GLOB_ONLYDIR)) before the foreach loop to see what it reports 5) :'(
-
thumbnail script stopped working after upgrade to PHP version 5
DavidAM replied to daveyp2004's topic in PHP Coding Help
1) Don't use short open tags, some servers have them turned off (which is the default in 5.3, I think) and the code will not execute. So, instead of <? always use <?php 2) Turn on error reporting at the start of your script error_reporting(-1); ini_set('display_errors', 1); 3) Remove the @-sign. In fact, remove it from your memory. This construct HIDES errors, it does NOT fix them. Once you have removed these (and turned on error reporting) you may find that these statements are throwing errors. Fix these errors. --- IMHO a "Notice" is an error, a "Warning" is an error. Your logic should prevent any notices and warnings. If you come across errors that you can't figure out, post them here (along with the updated code) and we'll see what we can do. 4) It looks like this script is used as the SRC of an IMG tag. If that is the case, you will not see the errors or your programmed error message. To see your messages, request the image directly in the address bar of the browser. Note: error_reporting and display_errors reveals information about your site. These settings should be used in development ALWAYS. The should almost always be off in production. -
the specification for what? because the only reliable way I could get a multipart message to send when writing my mail function was to use \n and NOT \r\n RFC 5322 (and its predecessor, RFC 2822) state: and Edit As with most internet content, many readers (i.e. browsers and email clients) choose to interpret the standard loosely. So some readers may not care if the headers have the CR part. But, since the headers have to be read by the email transport servers -- that is, all servers handling the mail along the way -- it is a good idea to follow the standard. Some transport servers may not handle the headers properly without the CR in the line terminator; and some readers (clients) may not handle the headers properly without it.
-
It's been a while since I did this, but: 1) The specification says the headers are terminated with CRLF not just LF 2) The mime message is the BODY not a header (see my comment line below): // Email header $header = "From: ".$from_name." <".$from_mail.">\n"; $header .= "Reply-To: ".$reply_to."\n"; $header .= "MIME-Version: 1.0\n"; // Multipart wraps the Email Content and Attachment $header .= "Content-Type: multipart/mixed; boundary=\"".$boundary."\"\n"; # I BELIEVE EVERYTHING BELOW HERE SHOULD BE THE BODY NOT PART OF THE HEADERS $header .= "This is a multi-part message in MIME format.\n"; $header .= "--".$boundary."\r\n"; // Email content // Content-type can be text/plain or text/html //$header .= "Content-type:text/plain; charset=iso-8859-1\r\n"; $header .= "Content-Transfer-Encoding: 7bit\n"; $header .= "$message\n"; $header .= "--".$boundary."\n"; // Attachment // Edit content type for different file extensions You are putting everything in the headers and sending an empty body. In a raw email stream, the first blank line separates the headers from the body. So putting everything in the headers might work, if the blank line is put in the right place. However, I don't know what PHP's mail() function does with the headers --- does it remove extra blank lines? --- so I would separate them.
-
The <FORM> opening tag is inside your loop; while the closing tag is outside the loop. So either you have multiple forms and all but one are broken; or you have a single form and it is badly broken. If you are trying to have all of the fields submitted as an array in a single form you need to: 1) move the form opening tag outside the for loop; and 2) change the field names that should be repeating to be arrays Something along these lines: <form id="texts" name="texts" method="post" action=""> <?php $items = count($showbasket); for ($i = 0; $i < $items; $i++) { ?> <input name="mainqty[$i]" type="hidden" value="<?php echo $showbasket[$i]['qty']; ?>" /> <input name="productid[$i]" type="hidden" value="<?php echo $showbasket[$i]['productid']; ?>" /> <input name="productqtyid[$i]" type="hidden" value="<?php echo $i; ?>" /> <input name="productsize[$i]" type="hidden" value="<?php echo $showbasket[$i]['size']; ?>" /> Then process them in a loop: foreach($_POST['productqtyid'] as $i) { $productid = $_POST['productid'][$i]; $productqtyid = $_POST['productqtyid'][$i]; $productsize = $_POST['productsize'][$i];
-
[*]If the home cost less than 55,0000, you are not charging any tax (not related to the current complaint); [*]If the home is over 400,000, you are not charging the middle tier; [*]If the home is over 500,000, you are not charging the third tire; It would work correctly, like this: # $home_cost = $_REQUEST['home_cost']; $home_cost = 500000.01; if ($home_cost > 0) { $MLTT = min($home_cost, 55000) * 0.005; if ($home_cost > 55000) { $MLTT += min(($home_cost - 55000), (400000 - 55000)) * 0.01; if ($home_cost > 400000) { $MLTT += ($home_cost - 400000) * 0.02; } } } else { echo 'Are you sure you want the home if they have to PAY you to take it?!'; } printf('My Calc #1: %.3f' . PHP_EOL, $MLTT); As an alternative, you can put the values in an array, and then not have to rewrite your code if the tiers change: # $home_cost = $_REQUEST['home_cost']; $home_cost = 500000.01; $breaks = array( array('min' => 0, 'max' => 55000, 'rate' => 0.005), array('min' => 55000, 'max' => 400000, 'rate' => 0.01), array('min' => 400000, 'max' => -1, 'rate' => 0.02)); $MLTT = 0; foreach ($breaks as $vals) { if ($vals['max'] == -1) { /* edge case - the LAST entry should have -1 for the max */ $MLTT += ($home_cost - $vals['min']) * $vals['rate']; } else { $MLTT += min(($home_cost - $vals['min']), ($vals['max'] - $vals['min'])) * $vals['rate']; } } printf('My Calc #2: %.3f' . PHP_EOL, $MLTT);
-
First you need to change the checkbox name in the form so that it POSTs an array of selected ids (note the empty square brackets): <input type='checkbox' name='cars[]' value='" ... Then you need to change your WHERE clause to select multiple cars. SELECT * FROM garage WHERE uci IN (1, 2, 3) I'd show the PHP for that, too, but I don't work with prepared statements, so I'm not sure how it would go. In standard code, it would be $sql = 'SELECT * FROM garage WHERE uci IN (' . implode(',', $_POST['cars']) . ')'; Of course, you'd need to sanitize those inputs, first.
-
You do realize that the 3-digit area-code, 3-digit exchange, 4-digit number is a creation of the North American Numbering Plan Administration (NANPA) which only covers North America, right? If your target audience includes people in other regions, their phone number structure is different.
-
If statement in the middle of string concatenation?
DavidAM replied to brian914's topic in PHP Coding Help
or you can use the ternary operator $address = "<p><strong>Address 1: </strong><br>" . $address1 . "<br>" . (isset($address2) ? $address2 . "<br>" : '') . $city . ", " . $state . " " . $zip . "</p>"; -
Are you sure it is a SMALLINT? That sounds like sorting of a character field that happens to contain "numbers"? Are you doing any manipulations on the field in the query? How about showing us the query? But first, check the column definition.
-
I have not worked with this in a while, but I think the problem is in $fileatt = $_SERVER['DOCUMENT_ROOT']."/home-care-information/test.html"; $fileatt_name = "Application"; # ... $message .= "--{$mime_boundary}\n" . "Content-Type: {$fileatt_type};\n" . " name=\"{$fileatt_name}\"\n" . "Content-Disposition: attachment;\n" . " filename=\"{$fileatt_name}\"\n" . "Content-Transfer-Encoding: base64\n\n" . $data . "\n\n" . "--{$mime_boundary}--\n"; [i broke it up a little to make it easier to see] Where you assign the "name=" and "filename=", you are giving it the value "Application", which does not have an extension. I think you want to put the actual filename here (without any path) as a "suggestion" of the name to save as. So, perhaps $destFile = basename($fileatt); $message .= "--{$mime_boundary}\n" . "Content-Type: {$fileatt_type};\n" . " name=\"{$destFile}\"\n" . "Content-Disposition: attachment;\n" . " filename=\"{$destFile}\"\n" . "Content-Transfer-Encoding: base64\n\n" . $data . "\n\n" . "--{$mime_boundary}--\n";
-
1. Change your second WHERE to AND 2. Use the mySql functions for date/time manipulation DELETE FROM temp_users WHERE book_stage='0' and AND UNIX_TIMESTAMP() > creation + 60 This assumes, of course, that the creation column is an integer datatype.
-
mikosiko is correct. Somehow, I always forget about that. It could be used here, it will be returned as the last row in the result set, with the "type" column being NULL. SELECT type, COUNT(species) AS Total, SUM(IF(fate="released", 1, 0)) AS Released, SUM(IF(fate="incare", 1, 0)) AS InCare FROM animalrecord GROUP BY type WITH ROLLUP type Total Released InCare Birds 5 3 8 Mammals 7 5 12 NULL 12 8 20 Since PHP sees this as just another row in the resultset, you have to "watch" for it in your loop if you want the output to be styled in some way that makes it obvious that it is the "Total" row. Also, you have to be careful if it is possible that (in this case) "type" will ever contain a NULL (in the table).
-
There are a couple of ways to do this. If there are a small number of "fates" and they are not likely to change, then we can do it in the query. As the number of "fates" increases the query becomes cumbersome to maintain. In that case, I would group by the type and fate, and then handle the "crosstab" in PHP. We can start with your base query SELECT type, COUNT(species) FROM animalrecord GROUP BY type and add counters for the known "fate" SELECT type, COUNT(species) AS Total, SUM(IF(fate="released", 1, 0)) AS Released, SUM(IF(fate="incare", 1, 0)) AS InCare FROM animalrecord GROUP BY type In this query, we are adding (SUM) one (1) for each row that meats our "fate". As you can see every "fate" you add, requires an additional line in the query. So, when there are many "fates", or we need to be able to add to the list often, it would be easier to group on fate and have PHP build the table: SELECT type, fate, COUNT(species) AS Total FROM animalrecord GROUP BY type, fate As to the bottom totals for the table, I think you will just have to accumulate the values (in PHP) as you output them, and then print the totals from the accumulators.
-
see number_format and/or money_format
-
It is possible that you are including a php file that is itself including config.php. If this is the case, you can either change config.php to not start a session if one has already been started, or change all of the include('config.php'); statements in all of your files to be include_once('config.php'); It is also possible that your php.ini is setup to automatically start a session for all requests. In this case, you can either disable this setting in the ini file, or (again) change the config.php to not start a session if one has already been started.
-
fopen($myFile, 'a'); The "a" tells it to append to the file if it exists. See the manual for fopen.
-
Note: trigger_error generates a NOTICE if no error level is specified. If error_reporting is not on, or is excluding NOTICE's, that page could produce absolutely nothing. 1) Do you have error reporting turned on? error_reporting(E_ALL); ini_set("display_errors", 1); 2) Is your table really called "tablename"? Edit You probably should check the result of the select db statement as well: if (! mysql_select_db($database, $con)) { trigger_error(mysql_error(), E_USER_ERROR); }
-
strip it out $newhtml = preg_replace('~</body></html>$~i', '', preg_replace('~^<!DOCTYPE.*<body>~i', '', $doc->saveHTML())); I'm no RegExpert, and I'm pretty sure some of those characters need to be escaped, but unless DOCDocument provides a way to retrieve it without the tags, you will just have to strip them out.
-
Neither extract(), nor your original code changes, is going to make the site work. extract() is a one-way process. While it will create the "session" variables in global scope (such as $ADMINNAME), it is not linked to the session, so any script that modifies $ADMINNAME or tries to assign a value to it, is not affecting the session value, which means it will revert to the old value on the next page load. This process will also not pickup any "session" variables that are created in other scripts. My recommendation is to setup the site on a development machine, turn on full error reporting, and start fixing the "undefined variable" errors. I think the closest you can get to replicating the behavior would be to "reference" the $_SESSION values: foreach ($_SESSION as $key => $value) { $$key = &$_SESSION[$key]; // Note the & that makes the new variable a reference } Then everywhere (in all scripts) that there is a session_register() call, change it to: session_register("ADMIN"); $ADMIN = &$_SESSION["ADMIN"]; // Again with the & to make it a reference I have never tested this, and I'm not 100% sure that it will make the site 100% functional. I also Do Not Recommend Doing This. But that is my best guess at how to make things happen.
-
That's what you told it to do. for ($i=1; $i<count($files); $i++) Numeric arrays start at zero, unless you intentionally start it somewhere else.
-
In order for ON DUPLICATE KEY UPDATE to work, you must be inserting a value into a field that has a unique index and that value already exists in that index. I don't see a field in your INSERT statement that looks like a "key" field. As an example -- assume that the Slug column is defined as a unique index INSERT INTO Users (Slug, FirstName) VALUES ('DavidAM', 'David') ON DUPLICATE KEY UPDATE FirstName = 'David'; INSERT INTO Users (Slug, FirstName) VALUES ('DavidAM', 'Wrong') ON DUPLICATE KEY UPDATE FirstName = 'Wrong'; The first INSERT statement will INSERT the correct data (assuming the "Slug" does not already exist). The second INSERT statement will UPDATE the table, making my name Wrong. There is no reason to assign a value to Slug in the UPDATE since I know that that is the column that caused the duplicate (in this case). mySql does not care which unique index causes the duplicate. So if there were a unique index on FirstName as well, and there is already a FirstName of "Wrong" in the database, the second update statement would fail with a duplicate key error. Also, in that case, I would have needed to have the "Slug" column in the UPDATE statement since I could not be sure which index triggered the DUPLICATE KEY
-
How to get the time the file was last modifed at in a PHP script
DavidAM replied to APD1993's topic in PHP Coding Help
Yes. The function is filemtime. If you show the code you tried and any error messages, we can help you fix it. The most likely reason it failed (IMO) is that the glob function returns just the filenames (without the path portion), but you need to specify the path in the call to filemtime(). -
There is an explanation of the calculation in the manual under Row Size Limits And here is an example of how helping others teaches me things: