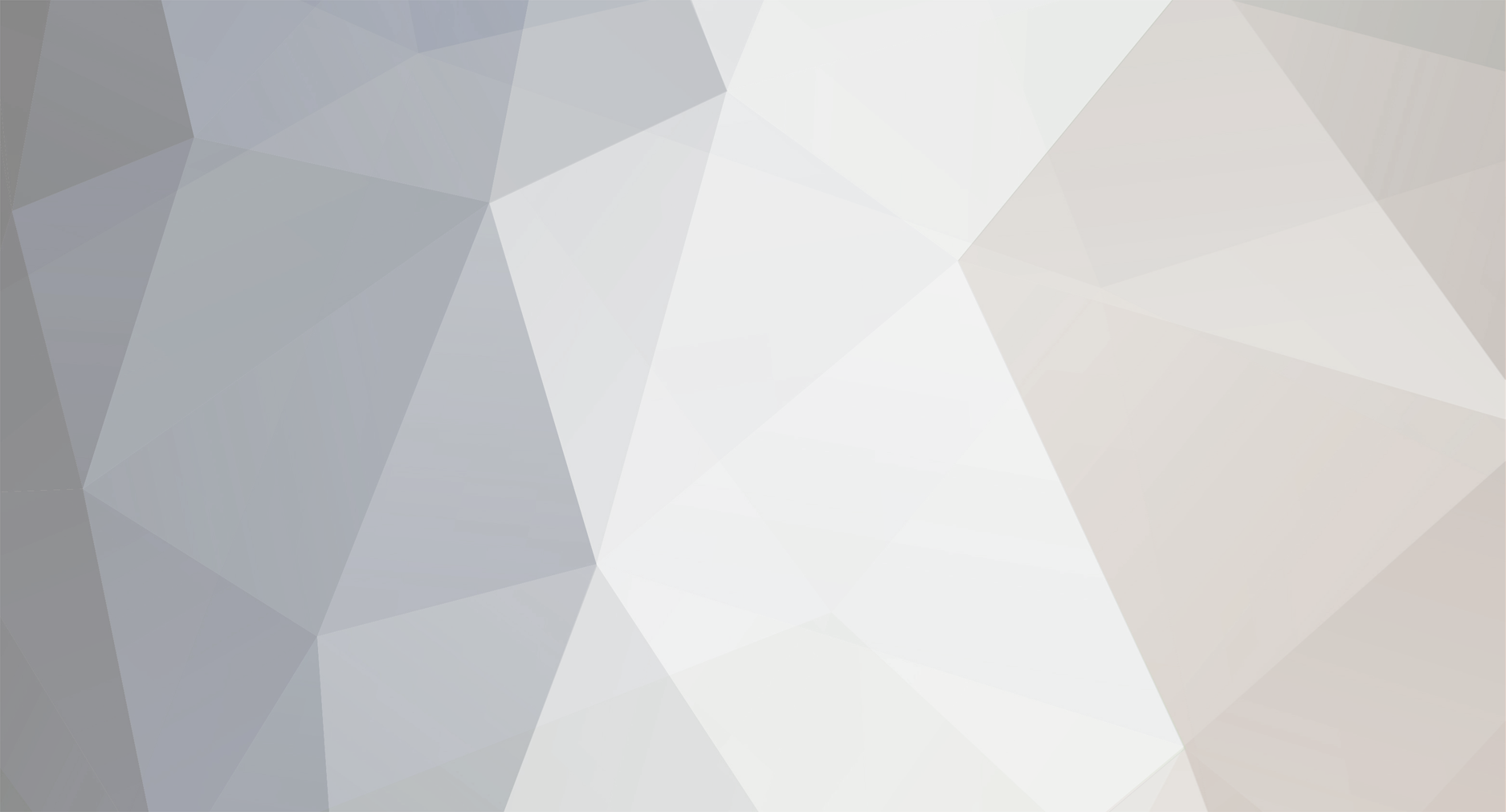
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
Syntax guidance to use mysql datetime for html table with php
DavidAM replied to ThisisForReal's topic in PHP Coding Help
First convert the data to a unix timestamp using strtotime(), then use the date() function to format it: $datetime = strtotime($meals['mealDateTime']); $date = date('M j', $datetime); $time = date('g:i a', $datetime); -
Redesign the database. Cancelled, State, Status, and so forth should probably be individual columns in the table, not embedded in a text field. As it stands you need to do two things: 1) Change HAVING to AND - HAVING is optimized for GROUP BYs and will not filter the rows being selected, it filters the rows being returned. A subtle difference, but it basically means that it will not be considered until all other conditions are met. 2) Make sure that eventStartDate and eventEndDate are individual indexes on the table. They do not have to be unique indexes. LIKE will never use an index, so you have to limit where it is looking. By changing the HAVING and letting the engine use an index, you should get better performance. You are most likely doing table scans right now. I don't think there is anything else that can be done since all the other conditions are using LIKE.
-
The query would look something like this: SELECT c.id, c.posted, c.comment, c.profile_name, u.user_id FROM profile_comments c JOIN site_users u ON u.user_username = c.profile_name ORDER BY c.id DESC LIMIT 5
-
Calling a function from inside a function - possible ?
DavidAM replied to MacRedrum's topic in PHP Coding Help
MacRedrum: do you have the global variables defined anywhere OUTSIDE of the functions? Or are they being "created" by the function. I have seen problems with a function creating a variable that has been defined as global (inside the function) not actually becoming global. For Instance: function doWhat() { global $something $something = pickOne(); } But if you just declare the variable outside the function scope it seems to work $something = ""; // This variable is global because it is outside the function scope function doWhat() { global $something $something = pickOne(); } It does not make sense to have to do it, and maybe I was fighting a different problem at the time, but it is worth a try. If you get this working with all of these globals, you are going to want to review it and design a better approach, whether it is object oriented or not. In fact, you may spend more time getting this to work as you have it than if you stop right now and redesign. And before I get a bunch of hate mail: yes, there may be a better way to do this without having all of those global variables. But PHP is loaded with globals and we ALL use them ALL the time; $_SERVER[] comes to mind, and oh yeah, $_SESSIONS, and maybe a few others? There are times when a global is the right solution (IMO). -
Since you are using a condition on a column in the COMMENTING table, you are going to have to have that condition NOT exclude the POSTS with no comments: 'SELECT POST.ID,Subject,Body,Name,GivenName,Surname,UpdatedAt,AuthID, COUNT(COMMENTING.ID) AS NrOfComments FROM POST INNER JOIN USER ON POST.AuthID = USER.ID INNER JOIN FAMILY ON USER.FamilyID = FAMILY.ID INNER JOIN CATEGORY ON POST.CatID = CATEGORY.ID LEFT JOIN COMMENTING ON COMMENTING.CommentableID = POST.ID WHERE (CommentableType='post' OR CommentableType IS NULL) GROUP BY POST.ID' When you do an outer JOIN you get NULLs for all columns in the table (in this case COMMENTING) if there is no match; even if the columns are specified as NOT NULL, the query will return NULL. Since you specified only count the rows with CommentableType = 'post' (why was that a ? in your code?) the POST rows without comments were not in the resultset. By adding the OR ... IS NULL we allow these POST rows and the count will be zero.
-
Pretty much. It assigns an empty array to $topicIDs. If anything was there before (whether an array or a scalar) it is lost. If it did not exist before, it will now. That adds the value in $row['topic_id'] on to the end of the array ($topicIDs). It is the same as $topicIDs[] = $row['topic_id']
-
It is unlikely that PHP is being "lazy". It is more likely that the files being loaded are short enough that randomizing them returns the same value often enough to be noticed. I would suggest an alternate approach, for that reason as well as efficiency. You are reading the same file into an array over and over again. Why not pull that outside the loop: $file1 = file("1.txt"); $file2 = file("2.txt"); $file3 = file("3.txt"); shuffle($file1); shuffle($file2); shuffle($file3); $var_a = 1; $var_b = 50; while($var_a <= $var_b) { $one = array_pop($file1); $two = array_pop($file2); $three = array_pop($file3); echo $one . ", " . $two.", ".$three; $var_a++; } Of course if there are less than 50 entries in any of the files, that will produce an error.
-
In your test form, add this just before you look at $_POST: <?php echo '<PRE>'; print_r($_POST); echo '</PRE>'; if (isset($_POST['submit'])) { I think you will see that "submit" is not defined in IE. I'm not sure, but I think this is one thing they actually do according to the spec. In other browsers the "submit" is passed (if I remember correctly) but IE sends only the X and Y position of the mouse within the image. Look at what keys are being consistently set in $_POST between the different browsers and decide how to figure out if the form was posted. You might even consider just using if (isset($_POST)) since $_POST should not exist unless the form was actually posted.
-
The code tags recognize full PHP tags ( <?php ) but not short ones, which you should not be using anyway. The problem with the code is at the top: /* Let's build the query string from a variable that is not defined yet (so it has no value) - Move this line down below then one that sets $trimmed */ $query = "SELECT * FROM widgetlist WHERE cname LIKE '%$trimmed%'"; $numresults=mysql_query($query); $numrows=mysql_num_rows($numresults); // Get the search variable from URL $var = @$_GET['q'] ; $trimmed = trim($var); //trim whitespace from the stored variable // PUT THE $query line here
-
Page Redirect after Error Message is Output to the Browser
DavidAM replied to makamo66's topic in PHP Coding Help
As PFMaBiSmAd said, the best approach is the have the form and the process in the same file (usually). Users will get tired of having to retype everything they put in the form just because one field has a typo. But if I absolutely have to display a message and then redirect, I use the META REFRESH tag. <meta http-equiv="refresh" content="2;url=http://domain.com/destination.php"> It goes in the HEAD of the HTML document. The "2" in the content attribute is the number of seconds to wait before loading the page specified in the "url=" portion of the attribute. This gives the user time to read the message. <HTML> <HEAD> <META http-equiv="refresh" content="2;url=http://webdesign.about.com"> </HEAD> <BODY> <P>That was a very bad thing to do ...</P> </BODY> </HTML> Using Javascript to PRE-validate the form is OK, but the user can turn off Javascript, so you HAVE to validate on the SERVER as well. Of course, since the META REFRESH is done by the browser, I suppose it is possible for this feature to be disabled as well. So you should also have a link in the page that the user can click to go to the page you want them to go to. -
You are getting that loop when you login? Show the code for the index.php from the directory (privilege) that you a trying to log in to.
-
Change your getRandomFile() function to return an array of the image files in the directory (and maybe change the name to getFileArray() or something). Then shuffle() the array (which randomizes it). Call this new function BEFORE your loop starts. Then in your loop, use array_pop() to get an element from the array (which will be a random filename). Since array_pop() removes the entry from the array, you will not get the same filename a second time. Calling your current function inside the loop means you are scanning the directory 3 times. As the directory gets bigger (more files) this scan will take longer. By calling it only once, you are preventing a problem in the future. Also, you do not need to create an image (imagecreatefromjpeg()) just to find out its size. I think the getimagesize() function would be more efficient here.
-
Use of an $id array to include page content from directory.
DavidAM replied to tbint's topic in PHP Coding Help
Something more like this might work: if (isset($_GET['id'])) { $id = $_GET['id']; if (isset($page[$id])) { // the page exists include($page[$id]); } else { // the page is not in the array include ('pages/404.txt'); } } else { // No id specified include ('pages/home.txt'); } Also, rather than using two separate arrays that you have to keep in sync, I would be tempted to write it this was: <?php $pages = array ( 0 => array ('file' => 'pages/home.txt', 'title' => 'sourfly.us'), 1 => array('file' => 'pages/home.txt', 'title' => 'Home'), 2 => array('file' => 'pages/about.txt', 'title' => 'About'), // and so forth 404 => array('file' => 'pages/404.txt', 'title' => '404 Page Not Found!') ); if (isset ($_GET['id'])) { $pageID = $_GET['id']; if (! isset($pages[$pageID])) $pageID = 404; } else { // No page specified send them home $pageID = 1; } include($pages[$pageID]['file']); echo $pages[$pageID]['title']; // show the title ?> Then your page list is all in one place. If you want to add a page later, you only have to change that one array. Also, use full php tags ( <?php ) some servers do not support the short ones ( <? ) and if you move to a new host you might spend a few hours trying to figure out why your site does not work there. -
A blank page usually indicates that the HTML is screwed up or not being output. Post the code of the page that is producing this problem, all of it (at least through the first few lines after the BODY tag). Also, when you get this blank page in your browser, use the "View Source" feature of the browser to see what HTML is actually being sent. That might point you to the problem.
-
You need to take a close look at your create_dropdown() function. You have a slight logic error there that is generating invalid HTML which could lead to problems: return $dropdown; echo "</select>"; } you are returning the SELECT code you just generated without the closing tag, and then echo-ing the closing tag. I suspect the closing tag is appearing in the HTML before the actual SELECT tag. Which is probably why you added a closing SELECT tag after your call to this function in the main document. The reason you get that javascript message is that these two statements are using variables as the element IDs and the variables are not defined. pickup = document.getElementById(searchpickup).selected; dropoff = document.getElementById(searchdropoff).selected; you probably meant to put those in quotes: pickup = document.getElementById('searchpickup').selected; dropoff = document.getElementById('searchdropoff').selected;
-
Ok, I'm an idiot ... well, maybe not an idiot, but that parenthesis we took out, should have been there, we were just missing the openning paren on the isset() function. // This line if (! isset$_SESSION['privilage']) { // User not logged in //Should be if (! isset($_SESSION['privilage'])) { // User not logged in by the way, for future reference, post the full error message; or at least the line number from the end of the message, and maybe the filename (near the end). If we are looking at a long piece of code, the line number helps us figure out where to start looking for the problem. Oh, and yeah, this is php code, so it will have to be in php tags ( <?php ... ?>)
-
ignace: Looks like you were calculating disk space, not number of entries. And you did not include any overhead for the indexes; of course, you assumed all of the keywords are going to be 255 characters long (varchar only uses the space needed for the contents plus a little overhead for the length of the content), so I guess that makes up for it. razta: You are storing each keyword only once, right? I mean if I search for "wine" you INSERT it into the database with a count of 1. When the next guy searches for "wine", you UPDATE that entry and increment the count to 2. You are not doing a second INSERT, right? You should probably just let the table grow until it becomes a problem. But if you develop the archive process before the problem comes up, you will be ahead of the game. One thing to make note of, that auto_increment key is not going to do you much good at all. And you need to have the keyword indexed so you can locate it and update it. You might want to consider making the keyword your Primary Key and throw away the auto_number. Also, since your will be querying for the top 50, you might consider putting an index on the count column so that query will be more
-
Oops, there is an extra parenthesis in the first if statement. // This Line if (! isset$_SESSION['privilage'])) { // User not logged in // Should actually be if (! isset$_SESSION['privilage']) { // User not logged in There may be other syntax errors, I just typed that in the post to give you an idea of how it could work. The session_start() function has to called before ANY output to the browser, so yes it has to go before the <HTML><HEAD> code. Are these other pages PHP or just plain HTML? If they are HTML, you're going to be adding PHP into them, so they will probably have to be renamed from page_name.html to page_name.php Renaming the folders on the server will depend on how you access it. If it is a Linux server and you have command line access, the command is mv oldname newname. If it is a hosted server, you probably access through a control panel which should have a command somewhere to manipulate files and folders. You will also need to review your code, and make sure you change any references between pages: for instance <A href="../../receptionists/phonelist.php">View Phone List</A> in the manager's section may have to change. It might be easier to change your privilege names to match your existing folder names. As for the code tags; when you are posting a message, there are a series for formatting buttons above the edit box. One of them has a hash-mark (#) on it and next to it is one with PHP on it. The # button inserts code tags {code}{/code} (but with square brackets [] not curly) The PHP button inserts php tags {php}{/php} (but with square brackets [] not curly) everything between the open and closing tag is rendered as code, looks pretty and is much easier to read.
-
At the top of each page, just test the privilege value and if they are not in that directory, send them where they belong: session_start(); if (! isset$_SESSION['privilage'])) { // User not logged in header('Location: ../../index.php'); exit(0); } $privilage = $_SESSION['privilage']; if ($privilage != 'receptionist') { // or what ever directory this page is in if ('receptionist' === $privilage) { header('Location: ../../receptionists/index.php'); exit(0); } if ('manager' === $privilage) { header('Location: ../../managers/index.php'); exit(0); } if ('administrator' === $privilage) { header('Location: ../../admin/index.php'); exit(0); } } of course, if you change the system so the privilage and directory names are the same, you can simplify that: session_start(); if (! isset$_SESSION['privilage'])) { // User not logged in header('Location: ../../index.php'); exit(0); } $privilage = $_SESSION['privilage']; if ($privilage != basename(dirname(__FILE__))) { $rdir = '../../' . $privalage . '/index.php'; header('Location: ' . $rdir); exit(0); } By the way, put your code in code tags when you post, it makes it easier to read. Disclaimer: This code is untested and offered as an example.
-
Insert all of the search words into the table, but add a column for LastSearch date. Then run a cron job daily or weekly (or whatever) that deletes any entries that have a low count and the LastSearch date is over 1 week or 1 month or whatever old. This way, you are only tossing out words that have grown "stale". Another option would be to create a second table to "archive" these deleted words to. Then when you get ready to delete, you can check to see if the word is in the archive and if it is, add the archive count to the live count before deciding what to delete. If you delete a word, insert or replace it in the archive table with the updated count. You can have a cron job scan this table periodically to see if any words have a high enough count to be moved back into the live table.
-
how can i forward emails to PHP script on shared hosting account?
DavidAM replied to hoolahoops's topic in Linux
I did this on my development system once. Basically, you put a .forward file in your home directory which contains a pipeline that receives the email on stdin. I don't know if there are any hosting providers that support this. All I can say is that it can be done on a linux system. Talk to technical support at your webhost and see if they will. -
I would think the easiest way to do this would be to have a column in the user table called, oh, I don't know, "LastAccess". Every time the user accesses any page, update this column to NOW(). When you want to know how many users are logged in, select a count of users where LastAcccess is greater than NOW() - 5 minutes (or whatever time frame you want). As for the 3-a-day thing. That needs 2 columns in the user table, LastFightDay, and DayFightCount. If LastFightDay is today and DayFightCount is less than 3, then let them fight and increment the count. If LastFightDay is before today, set the count to zero, change the Day to today, let them fight and increment the count.
-
Here's where we use all that algebra we were taught in grade school: The ratio is one side over the other, so pick one ... width / height We want the ratio to stay the same when we change one value so: new_width cur_width ---------- = ---------- new_height cur_height To calculate the new width, we multiply both sides by new_height: new_width = cur_width / cur_height * new_height; Or, we can calculate new height new_height = new_width / cur_width * cur_height
-
A variable defined in one function does not exist in other functions. In this case the $db_conn defined in function a() is not the same $db_conn you are trying to reference in function b(). There are two ways to resolve this problem: 1-Recommended (though not necessarily by me) function a(&$db_conn) { $db_conn = new MySQLi('localhost', 'root', '', 'db'); } function b(&$db_conn){ $result = $db_conn->query("INSERT INTO users(name) VALUES('$name')"); } a($dbc); b($dbc); 2-Not Recommended (but I use it often for my db connection) function a() { global $db_conn; $db_conn = new MySQLi('localhost', 'root', '', 'db'); } function b(){ global $db_conn; $result = $db_conn->query("INSERT INTO users(name) VALUES('$name')"); } $db_conn = null; a(); b();
-
$s.='</table>'; echo "$s\n"; the dot-equal is adding the closing table tag to the current contents of $s. At this point $s still contains the last row from the while loop, so you are outputting it again. This line should be: $s ='</table>'; echo "$s\n"; although, I would just echo '</table>'; Also, you are not closing any of your columns or rows. You need to add a '</td>' after each cell content and a '</tr>' after the last cell (closing tag) of each row. Some browsers might not render content that follows the table correctly if things are not closed properly. One more thing, instead of using '<td><b>Column Head</b></td>' try using '<thead><th>Column Head</th></thead>'. This does a couple of things: 1) It bolds the contents of the cell so you don't have to; 2) it centers the contents; 3) some browsers will repeat the heading row(s) when a page is printed and runs over to multiple pages.