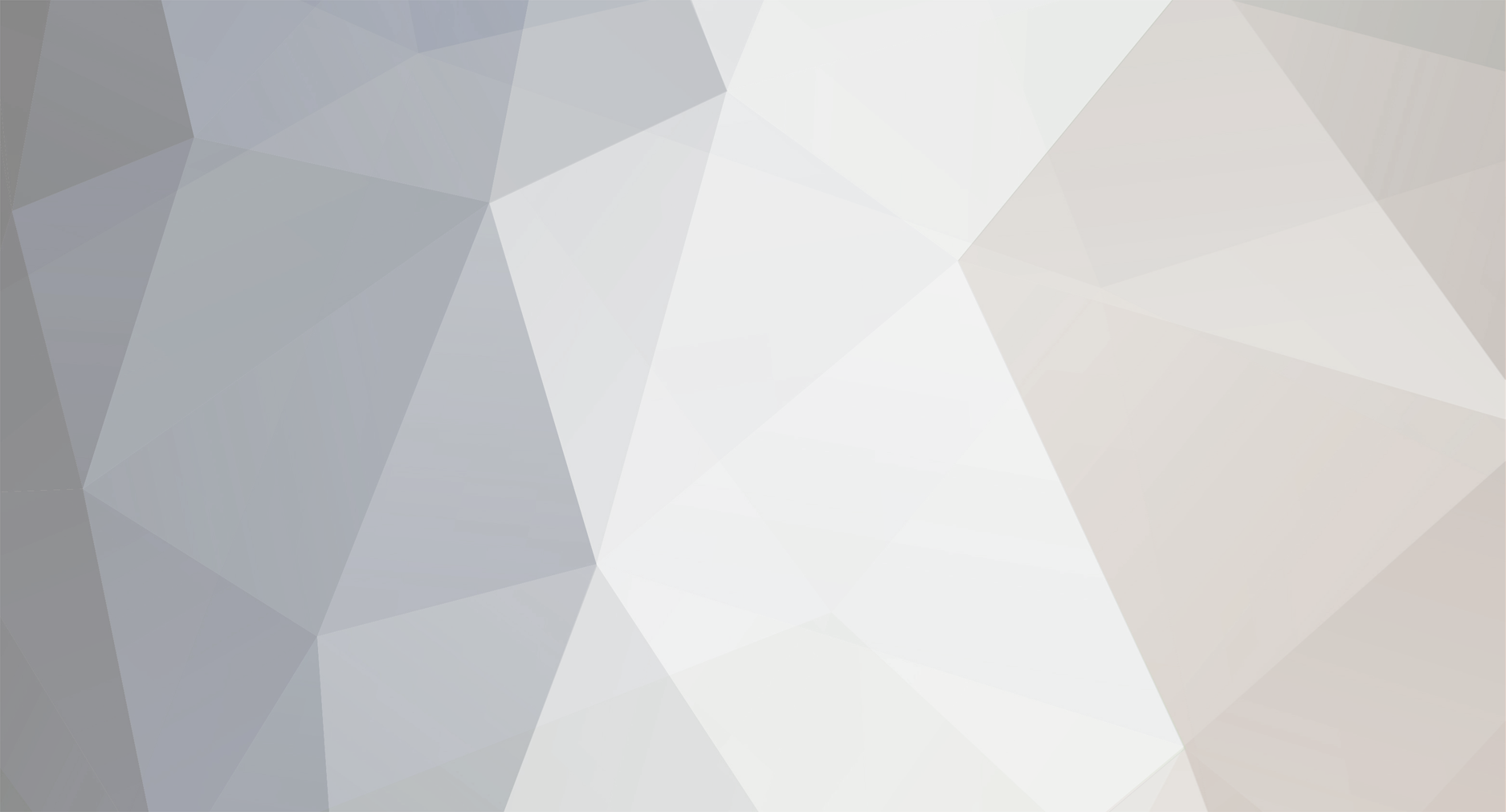
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
If $time is always a string with a colon in it: function roundMe ($time, $upDown) { list($hrs, $mins) = explode(':', $time); // Is it already on a 5-minute boundary? if (($mins % 5) == 0) return $time; if($upDown==1) { //round up $mins += 5 - ($mins % 5); } else { //round down $mins -= ($mins % 5); } if ($mins < 10) $mins = '0' . $mins; return $hrs . ':' . $mins; }
-
It would help if you indicated which line the error was reported on. I think your problem might be in this line: print "<td><image src=\"{$result['image_path'].$result['image_name']}\" height=\"{$result['image_height']}\" width=\"{$result['image_width']}\" /></td>"; I'm not sure, but I don't think you can use the dot operator inside the curly-braces. I'm not sure, because I almost never use curly-braces. This type of statement is very difficult to read when working with your code, and prone to errors. There are a couple of different ways to clean it up. First, instead of escaping double quotes inside a double-quoted string, switch to single quotes for one or the other. My favorite method is to use the printf() function: printf('<td><image src="%s" height="%d" width="%d" /></td>', $result['image_path'] . $result['image_name'], $result['image_height'], $result['image_width']); I have switched to using printf() and sprintf() for HTML and SQL generation almost entirely. The reason is clear. If you look at that code, you can see the HTML you are going to generate with the placeholders for the variables, then the variables are listed in the order they are applied. Or use single quotes for the print and concatenate the variables: print '<td><image src="' . $result['image_path'] . $result['image_name'] . '" height="' . $result['image_height'] . '" width="' . $result['image_width'] . '" /></td>'; If you really like the curly-braces, I think the correct way would be to remove the "." between the image_path and image_name and replace it with more curly-braces (they are separate varaibles): print "<td><image src=\"{$result['image_path']}{$result['image_name']}\" height=\"{$result['image_height']}\" width=\"{$result['image_width']}\" /></td>";
-
First, I have never worked with wordpress, so I don't know what might be happening in the include files that could cause a problem. But I can make a couple of suggestions When using this method to hide images (or other files) it can be difficult to debug. However, you can enter the url in your browser. If it works you will see the image (or whatever); if it does not work, you will see the error messages (so make sure you turn on error_reporting(E_ALL) in the script). So if, for instance, your image tag is: <IMG src="http://mydomain.com/showimage.php?id=1234"> you can enter http://mydomain.com/showimage.php?id=1234 into the address bar on the browser and view the image or see the errors. It might be that one of the includes is sending content, so you get an error when sending the headers. Requesting the script directly should show you any errors that are creating that problem. Now, in your code, you are sending a header that says you are about to send a JPEG file. But then you send a GIF, PNG, or JPEG. I would think you need to specify the correct Content-type header based on the type of file you are sending. I'm not sure how picky browsers are, but personally, I would send this header after I determine which type of file I have. Also, you do not need to create an image in memory just to send it to the browser. This is useing resources and taking time that is just not necessary. You can use readfile($filename) to read the file and send it directly to the browser.
-
Track the last player you processed and only print the name if it is different from the current one. $database="tarb89_ausus"; mysql_connect ("localhost", "tarb89_admin", "leccums"); @mysql_select_db($database) or die( "Unable to select database"); $result = mysql_query( "SELECT * FROM characters ORDER BY `player`") or die("SELECT Error: ".mysql_error()); $num = mysql_num_rows($result); if ($num > 0 ) { $i=0; $lastPlayer=''; // TRACK THE LAST PLAYER TO PREVENT HEADINGS while ($i < $num) { $character_name = mysql_result($result,$i,"character_name"); $breed = mysql_result($result,$i,"breed"); $gender = mysql_result($result,$i,"gender"); $base_color = mysql_result($result,$i,"base_color"); $markings = mysql_result($result,$i,"markings"); $genetics = mysql_result($result,$i,"genetics"); $sire = mysql_result($result,$i,"sire"); $dam = mysql_result($result,$i,"dam"); $other = mysql_result($result,$i,"other"); $player = mysql_result($result,$i,"player"); $id = mysql_result($result,$i,"id"); // PRINT PLAYER NAME IF DIFFERENT FROM THE LAST ONE if ($lastPlayer != $player) { // IF YOU WANT A BLANK LINE BETWEEN GROUPS if ($lastPlayer != '') echo "<br>"; echo "<b>$player</b><br>"; $lastPlayer = $player; } echo "Horse ID Number: $id - $character_name - $breed - $gender - $base_color - $markings - $genetics - $sire - $dam - $other<br><br>"; ++$i; } }
-
Actually, that error message came from the database server. The 'line 1' is referring to line 1 of the query. Since your query contains only one line, it is not helpful here. The problem is that there is a quote character missing just before the comment string. The code should be: $sql = "INSERT INTO Comments (user, comment) VALUES ('".mysql_real_escape_string($_REQUEST['user'])."', '".mysql_real_escape_string($_REQUEST['comment'])."')"; or use sprintf to build the query, I've recently discovered this helps keep the code cleaner and easier to read $sql = sprintf("INSERT INTO Comments (user, comment) VALUES ('%s', '%s')", mysql_real_escape_string($_REQUEST['user']), mysql_real_escape_string($_REQUEST['comment'])); EDIT: I mean the line number is not helpful. The error message was very helpful.
-
@disciple10 The problem I have had (and may others if you google it) is that IE (at least up to version 6, I have never tried with anything later) will submit ALL BUTTON tags regardless of which one you clicked. It also submits the button text (between the open and close tags) instead of the value (which FF and others use). I don't know what the standard says, but I have not found a way to use multiple BUTTON tags that is reliable across multiple browsers. <button type='submit' name='copy' value='1'>Copy</button> <button type='submit' name='copy' value='2'>Copy</button> <button type='submit' name='copy' value='3'>Copy</button> // If you click the second button, // In IE you get $_POST = array ('copy' => 'Copy'); // In FF you get $_POST = array ('copy' => '2'); It is easier to see what happens if we use different buttons: <button type='submit' name='cmdCopy' value='doCopy'>Copy</button> <button type='submit' name='cmdDelete' value='doDelete'>Delete</button> <button type='submit' name='cmdEdit' value='doEdit'>Edit</button> // If you click the second button, // In IE you get $_POST = array ('cmdCopy' => 'Copy', 'cmdDelete' => 'Delete', 'cmdEdit' => 'Edit'); // In FF you get $_POST = array ('cmdDelete' => 'doDelete'); There are many cases where I would prefer to use BUTTON over INPUT type=submit -- styling, non-string value attribute, etc. -- but IE just makes it impossible
-
$sqlstr="select * from abc for var1='John'"; $sqlres=mssql_query($sqlstr); $foundrecs=mssql_num_rows($sqlres); $ABCresultststable = mssql_fetch_array($sqlres, MSSQL_BOTH); while ($ABCrestultstable = mssql_fetch_array($sqlres, MSSQL_BOTH)) { $owner=$ABCresultstable[1]; echo " <input type='text' value='$ABCresultstable[2]'> ....... other HTML stuff ......."; $sqlstr="select * from xyz for var1='$owner'"; // THIS NEXT LINE WIPES OUT THE RESOURCE FROM THE OUTER LOOP // ** USE A DIFFERENT VARIABLE HERE - AND WHEREVER YOU REFERENCE IT $sqlres=mssql_query($sqlstr); $foundrecs=mssql_num_rows($sqlres); $XYZresultststable = mssql_fetch_array($sqlres, MSSQL_BOTH); echo " <input type='text' value='$XYZresultstable[3]'> ....... ......."; }; You are trashing your Outler Loop query resource (see comment in code) with the second query. Also, just before your loop, you call fetch_array() but you never use the data. So your loop is not processing the first record in the result.
-
Are you using INPUT type="submit" tags or are you using BUTTON tags? I have had more luck with the INPUT than with the BUTTON tags. I had a similar situation a while back. Since the value attribute is actually displayed, we can't really use it to indicate which button was pressed. But, since we can send an array to PHP using the name tag: name="cmdSubmit[]", we can use the row number as the index to the name: <INPUT type="submit" value="Copy Items" name="cmdCopy[1]"> <INPUT type="submit" value="Copy Items" name="cmdCopy[2]"> <INPUT type="submit" value="Copy Items" name="cmdCopy[3]"> if you click on the second button, you should get: $_POST['cmdCopy'][2] = 'Copy Items' Then we can check which button we received: if (isset($_POST['cmdCopy'])) { $group = array_keys($_POST['cmdCopy']); $group = $group[0]; We should only get one button (if you are using the INPUT type=submit) so that should tell us which one it is. Edit: I have not tested this thoroughly. But I think that was how I did it.
-
I notice in your OP you defined the function as isServer(), but then a few lines lower you called it as isProductionServer(). Are you doing this in your code, or was that a typo in the post?
-
You are not checking for errors on any of your queries. So they could be failing and you don't know it. I don't use the mysqli object, so I'm not sure what the exact process is, but after you do '$this->query(...)' you should be checking for any mysql error and displaying it or handling it. I suspect that if you add error checking you will find that some of the string data you are adding contains a single-quote mark: like "12' snake" or "O'Roark something". If there is any possibility that a string will contain a single-quote mark - and there is unless YOU personally built the data and made sure it did not - you need to escape the string. It will not hurt to escape the string if there are no special characters, so just do it. Again, I don't use mysqli, but with plain vanilla mysql it is mysql_real_escape_string(). $this->query("INSERT INTO spicyvib_product SET model = '" . mysql_real_escape_string($data['model']) . "', quantity = '1', minimum = '1', subtract = '0', stock_status_id = '7', image = '" . mysql_real_escape_string($data['image']) . "', date_available = NOW(), manufacturer_id = '0', price = '" . (float)$data['price'] . "', cost = '" . (float)$data['cost'] . "', weight_class_id = '5', length_class_id = '3', status = '1', tax_class_id = '0', date_added = NOW()"); by the way, it is usually easier to debug, if you build the sql into a string and then execute the string. That way, you can echo the string and see the query that is being built to help figure out what is wrong with it: $sql = "INSERT INTO spicyvib_product SET model = '" . mysql_real_escape_string($data['model']) . "', quantity = '1', minimum = '1', subtract = '0', stock_status_id = '7', image = '" . mysql_real_escape_string($data['image']) . "', date_available = NOW(), manufacturer_id = '0', price = '" . (float)$data['price'] . "', cost = '" . (float)$data['cost'] . "', weight_class_id = '5', length_class_id = '3', status = '1', tax_class_id = '0', date_added = NOW()"; echo $sql; $this->query($sql);
-
is this a Magic Quotes problem with PHP Array?
DavidAM replied to ThunderVike's topic in PHP Coding Help
Glad I could help. Sorry we took the long way around. I couldn't really tell where we were trying to go at first. The change we made to the function passed a reference to the variable instead of a copy. That allowed us to modify the variable INSIDE the function and have those changes remain OUTSIDE the function. Read up on variable SCOPE. Basically, anything defined inside the function is local to the function and has nothing to do with the stuff outside the function. This is a key concept in programming. Take this example: <?php /* Here is a function definition - It does not matter where this is in the code, it is DEFINED here but NOT executed. */ function fred($input) { echo 'INSIDE1: ' . $input . '<BR>'; $input = 'green'; echo 'INSIDE2: ' . $input . '<BR>'; } // MAIN PROGRAM LOGIC STARTS HERE $someVar = 'sky'; echo 'OUTSIDE1: ' . $someVar . '<BR>'; // Here we call the function to execute the code inside it fred($someVar); echo 'OUTSIDE2: ' . $someVar . '<BR>'; the output from this is: OUTSIDE1: sky INSIDE1: sky INSIDE2: green OUTSIDE2: sky Notice the following: [*]When we call the function, we include a variable (in the parenthesis) whose value is passed to the function, this is called a parameter; [*]In the function definition, we used a different name for the parameter. That's fine because inside the function has nothing to do with outside the function; we can use the same name if we want, it just does not matter; [*]We changed the parameter's value INSIDE the function; the two echos inside show we received the original value AND we changed that value; [*]The last echo, after the function call - OUTSIDE the function - shows that the change we made INSIDE the function had no affect on the variable OUTSIDE the function. That's because PHP actually passed a copy of the variable's value. However we can add just one single character to this code and make a dramatic change: <?php /* Here is a function definition - It does not matter where this is in the code, it is DEFINED here but NOT executed. */ function fred(&$input) { // <== ONE SINGLE CHARACTER ADDED HERE echo 'INSIDE1: ' . $input . '<BR>'; $input = 'green'; echo 'INSIDE2: ' . $input . '<BR>'; } // MAIN PROGRAM LOGIC STARTS HERE $someVar = 'sky'; echo 'OUTSIDE1: ' . $someVar . '<BR>'; // Here we call the function to execute the code inside it fred($someVar); echo 'OUTSIDE2: ' . $someVar . '<BR>'; the output from this is: OUTSIDE1: sky INSIDE1: sky INSIDE2: green OUTSIDE2: green Notice that OUTSIDE2 now shows "green". That's the value we set INSIDE the function. This works because the ampersand ('&') we added in the function definition tells PHP to pass the parameter BY REFERENCE. Which means everything we do to that parameter INSIDE the function is affecting the ORIGINAL variable that was passed in. You will note that it still does not matter if we use the same name or not. Version Note: This works in PHP 5. In PHP 4 we had to put the ampersand (&) at the function call and in the definition. So the function call would have been: fred(&$someVar); I'm not sure if this is an improvement or not. I liked the PHP 4 way in some respects, because I could choose to pass a copy or a reference depending on what I needed in a specific call. But, such is life. If you use this "call by reference" in PHP 5, you get a notice that it is depricated. -
is this a Magic Quotes problem with PHP Array?
DavidAM replied to ThunderVike's topic in PHP Coding Help
Ok, David, seeing a little more of the code might have helped me understand what is (and should be) happening. So "* This is step 2 of 3 for the ad submission form" starts processing the POSTed fields, and loading them into an array named $postvals so you can submit them to the update process later. By the way, is there a typo in this section? if (!empty($_FILES['image'])) $postvals = cp_process_new_image(); should that be $postvals['image']? Because otherwise it is overwriting whatever is already there and the next assignment is overwriting that? Of course, I don't know what cp_process_new_image() is doing so I could be wrong. So, when you get through processing the non-checkbox fields, you call cp_show_form_checkboxes() to handle the checkboxes (which are POSTed as an array and need to be imploded). Now, inside this function you load the checkbox values into $postvals. BUT REMEMBER, you are inside a function, so this is NOT THE $postvals that you were loading OUTSIDE the function. Functions do not have access to the variables that are defined OUTSIDE the function. If I read this correctly, you want to be adding the imploded checkboxes to the SAME $postvals you used before. There are a couple of ways to accomplish this. The "recommended" way is to pass a reference to that variable into the function. So your function definition would be: function cp_show_form_checkboxes($catid, &$postvals) { // We use & to get the reference Then you can take out the 3 returns I added at the end of the function. And take out the foreach loop I added after the function Finally, change your call to the function to this: } // THIS IS THE CLOSING BRACKET ON THE FUNCTION DEFINITION $catid = ($_POST['cat']); cp_show_form_checkboxes($catid, $postvals); // NOTE: IF YOU ARE NOT USING PHP 5 you will need to change that to // cp_show_form_checkboxes($catid, &$postvals); /* REMOVE THIS STUFF - I GUESS I SHOULDN'T HAVE BEEN GUESSING foreach ($postVals as $key => $value) { update_option($key, $value); } ** END OF REMOVE THIS STUFF */ // A print_r() here should show EVERYTHING in $postvals // print_r($postvals); //these are the lines below that wrap things all up for the submit form // the oid is the session and the cp_is to distinguish all these values from regular Wordpress $option_name = 'cp_'.$postvals['oid']; update_option($option_name, $postvals); Of course there are other ways to do this. You could just add $postvals to your global statement INSIDE the function. Typically, you want to avoid the use of globals inside functions, though. Does all that make sense with what you are expecting to accomplish? I'll look through this again when I get home (in about 2 hours) and let you know if I see something different. -
It should remain set and available (depending on how you set it) unless 1) You change domains (including going from www.domain.com to domain.com) 2) You use the setcookie() function to erase it 3) You change directories 4) The cookie expires - what expire date did you put on the cookie? Sometimes, when working with cookies, it is helpful to use your browser to delete the cookies for the site, close the browser and start again. Can you post more (or all) of the index.php (especially any part that uses setcookie()). Also states.php (again, especially where it uses setcookie()). Is states.php a compete page in its own right? Should you be using a header() redirect instead of the include? This could be important if there is anything happening before the include. Do you have error_reporting() and display_errors turned on? Maybe you're getting an error setting the cookie.
-
is this a Magic Quotes problem with PHP Array?
DavidAM replied to ThunderVike's topic in PHP Coding Help
I understand the frustration. There's nothing like having a computer do exactly what you tell it to do, instead of what you want it to do; and then trying to figure out why. It does not look like you did anything with the $postvals array after you built it. First, check to see if the values are put into the array as expected. Immediately after the endforeach add this line: endforeach; print_r($postvals); } it should dump the array to the screen so you can see the keys and values. Post them here if they don't look right. What are you expecting to happen with the $postvals after to fill it up? Are you trying to use it in the update_option() function? You may need to add a return $postvals; to the function to get the array out of it, then process it. So maybe something like this: $catid = ($_POST['cat']); // queries the db for the custom ad form based on the cat id and shows any checkboxes function cp_show_form_checkboxes($catid) { global $wpdb; $fid = ''; //$catid = '129'; // used for testing // get the category ids from all the form_cats fields. // they are stored in a serialized array which is why // we are doing a separate select. If the form is not // active, then don't return any cats. $sql = "SELECT ID, form_cats " . "FROM ". $wpdb->prefix . "cp_ad_forms " . "WHERE form_status = 'active'"; $results = $wpdb->get_results($sql); if($results) { // now loop through the recordset foreach ($results as $result) { // put the form_cats into an array $catarray = unserialize($result->form_cats); // now search the array for the $catid which was passed in via the cat drop-down if (in_array($catid,$catarray)) { // when there's a catid match, grab the form id $fid = $result->ID; // put the form id into the post array for step2 $_POST['fid'] = $fid; } } // now we should have the formid so show the form layout based on the category selected $sql = $wpdb->prepare("SELECT f.field_label, f.field_name, f.field_type, f.field_values, f.field_perm, m.meta_id, m.field_pos, m.field_req, m.form_id " . "FROM ". $wpdb->prefix . "cp_ad_fields f " . "INNER JOIN ". $wpdb->prefix . "cp_ad_meta m " . "ON f.field_id = m.field_id " . "WHERE f.field_type = 'checkbox' " . "AND m.form_id = '$fid' " . "ORDER BY m.field_pos asc"); $results = $wpdb->get_results($sql); if($results) { foreach ($results as $result): // now grab all ad fields and print out the field label and value $postvals[$result->field_name]= implode(',', $_POST[$result->field_name]); endforeach; return $postvals; // Return the values so we can process them } else { echo __('No checkbox details found.', 'cp'); return array(); // Nothing found so return empty array } } else { return array(); // Nothing found so return empty array } } // RIGHT HERE I EVEN REFERRED IMMEDIATELY TO FUNCTION TO SEE IF THIS WOULD MAKE A DIFFERENCE // WITH OR WITHOUT THIS LINE BELOW IT MAKES NO DIFFERENCE // THERE ARE NO ERRORS, JUST NO IMPLODED ARRAYS $postVals = cp_show_form_checkboxes($catid); foreach ($postVals as $key => $value) { update_option($key, $value); } I added a couple of return statements and another else (for the last return statement) to the function. Then changed the processing of the returned array. -
is this a Magic Quotes problem with PHP Array?
DavidAM replied to ThunderVike's topic in PHP Coding Help
What I was trying to say is that you do not need the quotes. IF $result->field_name returns cp_checkbox_1 WITHOUT QUOTES, then $postvals[$result->field_name] = implode(',', $_POST[$result->field_name]); is exactly the same statement as $postvals['cp_checkbox_1'] = implode(',', $_POST['cp_checkbox_1']); // IF the field_name property of the $result object contains "cp_checkbox_1" ... $result->field_name = 'cp_checkbox_1'; echo $result->field_name; // WILL print: cp_checkbox_1 // AND echo implode(',', $_POST[$result->field_name]); // WILL print the EXACT SAME THING AS echo implode(',', $_POST['cp_checkbox_1']); We put quotes around string literals (cp_checkbox_1) because they are NOT variables ($fieldname) and not function names (implode()) and not operators (+), etc. That way the compile or interpreter will have a chance at knowing what it is. YOU DO NOT NEED THE QUOTES AROUND THE VARIABLE! You could put the quotes inside the variable as part of its value, but then it will not be the same thing: $result->field_name = 'cp_checkbox_1'; // IS NOT THE SAME AS $result->field_name = "'cp_checkbox_1'"; So the correct coding from your first post should be: foreach ($results as $result) { // now grab all ad fields and print out the field label and value $postvals[$result->field_name]= implode(',', $_POST[$result->field_name]); } assuming, of course, that $results is an array of objects that have a property called field_name. If you absolutely want/need the quotes around the value of the variable, then the correct code would be: foreach ($results as $result) { // now grab all ad fields and print out the field label and value $postvals["'" . $result->field_name . "'"]= implode(',', $_POST[$result->field_name]); } but that will NOT result in the same arrangement we had with the hard-coded values. In the solution of your other topic, the quotes ARE NOT PART OF THE ARRAY KEY they are simply there to indicate we are using a literal (hard-coded) value for the key. Go back to your other code, and dump the array using print_r(): $postvals['cp_checkbox_amenities']= implode(',', $_POST['cp_checkbox_amenities']); $postvals['cp_checkbox_days']= implode(',', $_POST['cp_checkbox_days']); $postvals['cp_checkbox_3']= implode(',', $_POST['cp_checkbox_3']); print_r($postvals); you will see that the quotes ARE NOT in the array keys. -
is this a Magic Quotes problem with PHP Array?
DavidAM replied to ThunderVike's topic in PHP Coding Help
You are correct. The first uses a literal string as the array index, the second is specifying a defined constant as the array index. However: $_POST['cp_checkbox_amenities'] = "Hello"; // AND $pickBox = 'cp_checkbox_amenities'; $_POST[$pickBox] = "Hello"; // ARE EXACTLY THE SAME // ALSO $pickBox = "cp_checkbox_amenities"; $_POST[$pickBox] = 'Hello'; // IS THE SAME THING, TOO // OH YEAH, THIS IS THE SAME THING TOO. $pickBox = "cp_checkbox_amenities"; $newValue = "Hello" $_POST[$pickBox] = $newValue; and by "SAME THING", I mean you get the same result. The reason for the single-quotes around the checkbox name is that we are using a literal value. If you use a variable that contains the name, no quotes are needed in the array reference. The major difference between single-quotes and double-quotes is how PHP treats the stuff inside the quotes. With double-quotes, PHP will scan for and replace variable names and control characters; with single-quotes, it does not. I personally always use single-quotes unless I need to use double-quotes for parsing or because there are single-quotes in the string. -
You have to change it at the location you moved it TO: move_uploaded_file($_FILES["file"] ["tmp_name"], "../Graphics/" . $_FILES["file"] ["name"]); chmod("../Graphics/" . $_FILES["file"] ["name"],0644);
-
Keeping to your code example: $insertCount=0; foreach($results[1] as $curName) { if($insert){$insertCount++;} echo <<< END $insertCount $curName<BR> END; } Although, I would probably do this: $insertCount=0; foreach($results[1] as $curName) { if($insert){$insertCount++;} printf('%d %s<BR>', $insertCount, $curName); }
-
There is a little-used second parameter to print_r() called $return. Set this to true to have the string returned instead of output to the browser: $myplugin_debug_test.= "\n[GETTING COOKIES...\n"; $myplugin_debug_test.= print_r($myplugin_all_cookies, true);//THIS IS LINE 38 $myplugin_debug_test.= "\n... DONE]<br/>\n";
-
Yes. I have not played with IFRAME much, and only on FireFox when I did. So, I'm no expert. However, my experience leads me to believe that an IFRAME is treated as if it were a separate browser window. Any link you click in the IFRAME loads in that IFRAME. So, I don't see any way, other than javascript, to affect the "parent" window. And I'm not sure what the DOM relationship is (if any) or if it is cross-browser friendly. Maybe someone with some IFRAME experience can help
-
//THIS IS ON index.php PAGE $cookie_state = $_COOKIE['state_nvc']; if(!isset($cookie_state)) { In this snippet, $cookie_state is set (you just set it). You need to test the actual cookie (and you don't need the elseif that tests the exact opposite; just use else). Also, the first line is/should throw an error (ok, a NOTICE, but those come from logic errors) if the cookie is not set. Take that line out and only assign the value once you know the cookie is set. //THIS IS ON index.php PAGE if(!isset($_COOKIE['state_nvc'])) { include "states.php"; } else { $cookie_state = $_COOKIE['state_nvc']; run code for index.php }
-
also, look at the parse_url() function
-
If you are going to a spot on the same page (not loading a new page) you ONLY need the hash. You do not need the filename or the query string <A href="#start">Top</A> Also, be sure to use both the ID and the NAME in the destination anchor, I had trouble with one browser wanting the ID and other browsers wanting the NAME. I'm not sure what the standard says. <A id="start" name="start"></A> Be aware that ID must be unique on the page. Also, this anchor is NOT visible, but is placed at the top of the window, so it should appear immediately above or before the first thing you want to be visible: <!-- RIGHT WAY --> <A id="start" name="start"></A> <H1>This is the Top</H1> <!-- WRONG WAY --> <H1>This is the Top</H1> <A id="start" name="start"></A>
-
1) Is the 'username' field actually the ID field and is it numeric? Is this field indexed? 2) I'm not sure that sending 500 ID's in an IN phrase is a good idea. Are these in a database table as well? Because a JOIN might actually perform better. 3) Don't copy the array and then implode it, just use it: $x= implode(',',$friend_id_array); $q.=$x . ',' . $me['id']; $q.= '))'; of course, you'll want to be sure to handle the case where it might be empty 4) I think you want DAY(thedate) instead of DATE(thedate). DATE() returns YEAR-MONTH-DAY so there is no sense in testing the YEAR(thedate) after that. DAY() is a synonym for DAYOFMONTH() which returns a value between 1 and 31. 5) Having said that, using the functions in the WHERE clause will prevent any index use on 'thedate' and will use more resources. You might be better off calculating the date range and using it in the query; like for instance: AND thedate BETWEEN '2010-08-08' AND '2010-08-14' // IF thedate IS A DATETIME FIELD USE AND thedate BETWEEN '2010-08-08 0:00:00' AND '2010-08-14 23:59:59' and possibly adding an index on 'thedate' 6) I don't see that you are "resorting" the array. You are retrieving the data from the database, which you have to do. You'll need to look at the query plan (see EXPLAIN) to see where the query is getting bogged down. Or post the results here for review. You may need additional indexes on the table. You'll want to look at the plan for several or all of the 6 different calls you make to this function. Also, you might want to profile the code to see if this function is in fact causing the bottleneck. Note: A query can only use, at most, 1 (one) index per table. You have to look at the ways that you query the data and choose the column(s) that get you closest to the final data. One last point. You know your application and database (well, at least more than I do). Consider the possibility of making a single call to the database to get all of the data you need for the 6 calls you make now. Add some flags to the data that is returned to indicate where it is needed. Then sort it out to the six places on the front end. It will add more front-end (PHP) processing; but might provide an overall performance improvement. Or at least consolidate some combination of calls. It might not be possible, but it's worth a thought.
-
// update data in mysql database $sql="UPDATE $tbl_name SET Day='$Day', Month='$Month', Year='$Year', Year='$Song', Year='$Data' WHERE id='$id'"; $result=mysql_query($sql); You are referencing variables that have not been defined in your script. Specifically $Day, $Month, $Year, $Song, $Data and $id. It would appear that you expect these to be coming from the form. However, the register_globals functionality of PHP has been turned off and will be removed from a soon-to-be-released version of PHP. It was a major security whole. You need to retrieve these variables from the $_POST array before referencing them. You should also sanitize them to prevent SQL injections. And check that they are valid $day = mysql_real_escape_string($_POST['day']); // etc. etc., etc., // OR if they are integers, you can use $day = intval($_POST['day']); // which might end up as zero if it was not a valid number Note: event the "id" value which you pass as a hidden field needs to be sanitized and validated.