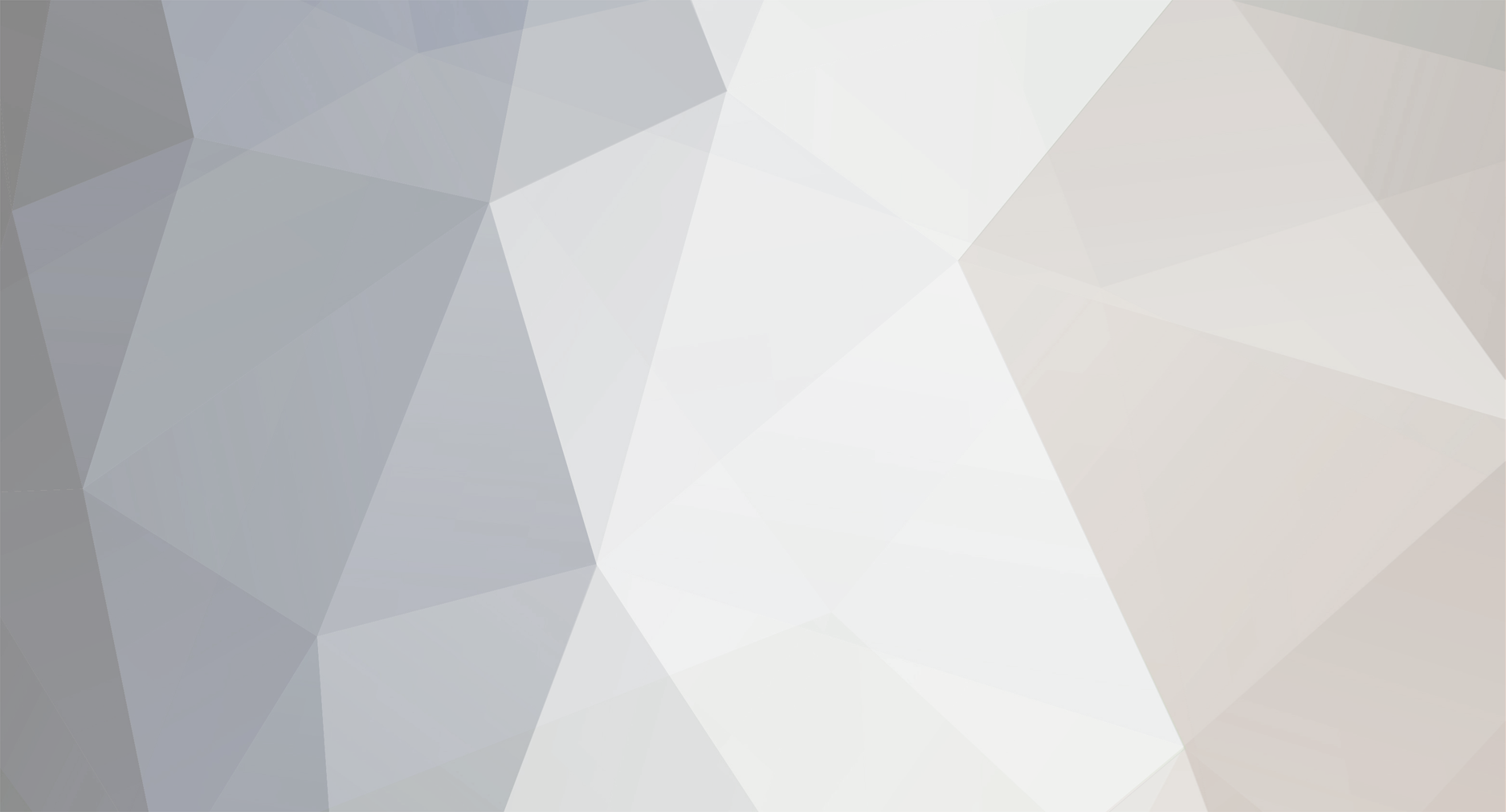
Hall of Famer
Members-
Posts
314 -
Joined
-
Last visited
Everything posted by Hall of Famer
-
There is a site called phpclasses.org, I use it and its really good.
-
Try not to use transaction script unless you do not have a choice, instead apply the data mapper design pattern to make the best use of OOP. Transaction script is not even OOP to begin with, it is an anti-pattern with objects written in procedural code.
-
New Job! Im Learning..But I Desire To Impress My Boss!
Hall of Famer replied to JoshB312's topic in Introductions
umm this thread should belong to another section, right? Anyway, buy a book about advanced OOP concepts will help, Id recommend Matt Zandstra's Object, Patterns and Practices, it has everything you will need to further improve your skills. -
PDO prepare: binding a parameter after SELECT
Hall of Famer replied to junkomatic's topic in PHP Coding Help
Your design looks flawed to begin with. When you have as complex domain logic as yours, Id say using a domain model and data mapper, following the OOP enterprise architecture design pattern. If all of your functions/methods accept the same argument $pdo and $username, it is a strong implication that refactoring is necessary. -
Again I was just trying to prove that many of the benchmarking test is overexaggering the performance issue in large frameworks like Zend. I never said Zend would be faster than other frameworks like Symfony or Yii, just not that much slower. Nor was I referring to the benchmarking test the OP brought up, its for all benchmarking artices I've seen in general. But anyway if you cant be convinced or refuse to be convinced, I am fine with it. All I have to say then is that I respect your opinion, but I have to accept our differences and stop here. I do not live my life trying to please everyone, especially when there's no actual benefit for me to even do this.
-
http://www.thedeveloperday.com/framework-battles-yii-vs-zend-framework/ Well heres an interesting article if you want to take a look. Just as I stated before, frameworks like Zend do tend to be slower, but the severity of such performance issues is overexaggerated. I have nothing against Yii, and in fact I quite like it. However, doing benchmark evaluation based on 'Hello World' is never a good idea. In fact, if all your script does is to output 'Hello World' on the screen, you are better off just using plain HTML. Why bother with server-side programming languages at all in this case? XD Here is another benchmarking article I found, also with flaws: http://www.techempower.com/blog/2013/03/28/framework-benchmarks/ Sure it seems to be using more realistic code this time, but because it is evaluating frameworks among many different platforms, the coding style becomes an issue here. You cant write java-like code with programming languages like Python to claim that the latter is much slower. Not saying it is not slower, but definitely not that much slower. With PHP, Cake is one of the slowest frameworks available, why not use Yii or Codeigniter instead? If I were to choose the slowest framework for java servlet and node.js, and compare them with the fastest PHP frameworks, the difference will be much smaller or even invisible.
-
Are you sure you are even defining a variable at all? the keyword define is used to declare PHP constants, these aint variables since their contents cannot be modified after you create the constants.
-
Hey, I need help with the following Classes syntax
Hall of Famer replied to elme's topic in PHP Coding Help
Well you can try this: static function create_user(){ return new static; } The self keyword references to the very specific class in which the method is defined, in your case it is the parent class personal_detail, this is why the property $gender is undefined when you call this method. The static keyword, on the other hand, points to the very child class that calls this static method. Read this article for more details on the variation between self and static: http://stackoverflow.com/questions/5197300/new-self-vs-new-static Anyway incase you have to, Id recommend against calling parent's static method to create objects of its child classes, static properties/methods dont work well with inheritance and composition, they aint true OOP at all. Try rewriting the classes with instance properties/methods using factory pattern is the better idea, and its better done in a specialized factory object. -
The error means that the method new_request::the_suppliment() does not exist. Since you only show a fragment of your code its difficult to help you with. Are you sure the method the_suppliment() is defined inside class new_request? You dont seem to have made a typo though, so thats the only clue I have for now.
-
I doubt about that though, as I mentioned before my CS professors are all strong OO advocates, cant say I am not at least a little bit influenced. As far as I know, Drupal 8 will be highly object oriented, especially considering it will integrate with Symfony. Apparently the developers have realized their past mistakes, and learned that OO is the right way to go when your application gets larger and larger. Man I am kinda excited, Drupal 8 is gonna be really professional amazing object oriented software.
-
If you are using PHP 5.4, Id recommend you to take a look at the session handler class. It is an object oriented way of playing with PHP sessions, you may extend its functionality by subclassing it: http://us1.php.net/manual/en/class.sessionhandler.php
-
Well design patterns are only applicable in OOP. Sure procedural code can try to 'mimic' some OO patterns, but usually does poorly and never feels the same way as you use the OO approach. I've seen many scripts who have claimed to be using MVC while being procedural, none of them are even half as impressive as true MVC with OOP. It feels like they are trying to force their way into MVC to make their application 'better-looking', but well... You dont use MVC for the sake of using MVC, dont you agree? I am a graduate student at an Ivy league university, whats your point? I also took many CS courses already for various programming languages, and my CS professors are all OO programmers and strong OO advocates.
-
Thats true, after you learn the basic OO techniques, it is about time to move on to design patterns, and then MVC architecture and the entire framework. Note the fact that you can work with objects does not indicate that you are already an OO programmer. The true beauty of OOP lies in the idea of OOA(Object oriented analysis) and OOD(object oriented design), without them you can easily write amateurish procedural code even if each line of your code has objects in it. To write true OO code, your entire script as a whole needs to be object oriented. Its not an easy process, may take a while to finally get there. Other than the whatever those advanced programmers already mentioned in previous posts, Id also recommend you to look into ORM(object relational mapping). Martin Fowler's book on Enterprise architecture patterns is a very good one to read, while you can also buy Matt Zandstra's Object, Patterns and Practices to see how Fowler's patterns get implemented into the world of PHP. The patterns are generally universal across all programming languages that support OO, but the actual implementation may vary slightly.
-
I do not recommend you to downgrade either. Like Requinix said, chances are the script works with PHP 5.3 too, you can always give a try. The required version PHP 5.2 may as well be the minimum requirement. If the script indeed does not work on PHP 5.3+, its not really worth using at all. In this case, either contact the script developer to ask for a newer version of it, or just try an alternative.
-
Question about using array_shift with OOP
Hall of Famer replied to eldan88's topic in PHP Coding Help
I am not quite familiar with Pear, but yeah I do remember they have a base mapper class. In most cases though, you will need to design individual data mappers for each different domain object, unless you have a really simple structure and that every database table shares exactly the same behavior. -
Question about using array_shift with OOP
Hall of Famer replied to eldan88's topic in PHP Coding Help
Well you can use dependency injection. The best way to instantiate a user class is to design a domain model and a data mapper. The beauty of this is, well, its truly object oriented. Heres an example from Anthony Ferrara: $mapper = new PersonDataMapper(new MySQLi(...)); $people = $mapper->getAll(); As you see, the database extension used here is MySQLi(quite similar to PDO though), and Anthony uses dependency injection to pass this database object into the Mapper object. The mapper then encapsulates all the processes of data retrieval, and mapping of data into person model or people collection. Note the above example fetches all users in the database, which is why you see the method getAll(). If you know the user id, simply use $user = $mapper->findByID($id) and the mapper returns a domain model object nicely. The implementation of Mapper classes can be a bit complex for beginners and intermediate skilled programmers though, its a step for intermediate skilled programmers to improve into advanced programmers. -
I've been reading Martin Fowler's book about enterprise architecture patterns for a while now. From what I find about Transaction script, it is not object-oriented at all. Its basically procedural code despite the fact that it may use objects in every line of its code.This code below, for instance, is purely procedural despite the fact that it works with objects in every line: <?php /* Execute a prepared statement by binding PHP variables */ $calories = 150; $colour = 'red'; $sth = $dbh->prepare('SELECT name, colour, calories FROM fruit WHERE calories < :calories AND colour = :colour'); $sth->bindParam(':calories', $calories, PDO::PARAM_INT); $sth->bindParam(':colour', $colour, PDO::PARAM_STR, 12); $sth->execute(); ?> Yeah this is not a complete picture of Transaction script pattern, and you can actually wrap up the code inside a transaction object's method(if you care to design one such object for each transaction). Still, this does not change the fact that the approach as a whole is procedural, its not object oriented. For this reason, transaction script is an anti-pattern in the object oriented world. Its usage should be limited to really simple/small script and for newbies to get used to database extensions such as PDO and MySQLi classes. The better solution is to use a domain model instead, coupled with a data mapper with unit of work/identity map/lazy load/query object and such. OOP is mostly about OOA(object oriented analysis) and OOD(object oriented design), which fits well with domain model and data mapper. To create object oriented script, we will need to take this necessary design approach. Fortunately most large application frameworks do have domain models and data mapper, which makes object oriented design much easier and less time consuming. Here is an example of Domain model with Data mapper, as illustrated by Anthony Ferrara. Its just a small fragment of the client code, with the detail of implementation hidden inside the domain object and mapper. It is truly object oriented: $mapper = new PersonDataMapper(new MySQLi(...)); $people = $mapper->getAll(); Using transaction script, however, the code is actually procedural in disguise. The usage of object operator -> does not make it professional object oriented code: $m = new MySQLi(...); $res = $m->query($query); $results = array(); while ($row = $m->fetch_assoc($res)) { $results[] = $row; } So what do you think? Should professional coders all stop using transaction script if they havent, since its actually an anti-pattern? Perhaps there are times when people are comfortable with using an anti-pattern, but I doubt there is any justification for at least large softwares to apply it.
-
Question about using array_shift with OOP
Hall of Famer replied to eldan88's topic in PHP Coding Help
Globals are bad not just in OOP, they are considered evil everywhere. You can easily overwrite a global variable, and break the rest of your code that depends upon it. You should only use them when you absolutely have to, most likely with metadata. If you can use dependency injection, do it, its way better than using globals. Otherwise, at least get around with a Registry object. Statics are not OOP at all, they can be called and accessed without using objects and object relations. Inheritance and Polymorphism are important for OOP, which are nonexistent in static context. Also you can bring back the horror of global state with statics, especially for public static properties/methods. Theres nothing wrong with private properties though, and in fact properties should be private or protected while public properties are poor OOP practice. -
So what are you trying to say? Pro-framework or pro-nonframework? I am kinda confused.
-
Object-Oriented Programming Clarification?
Hall of Famer replied to Strider64's topic in PHP Coding Help
Thats really great to know, congratulations. In a perfect script everything is an object, you are making a huge step into writing a professional application. If I may suggest, your next step can be building a framework with object oriented design. This way your entire application as a whole is also object oriented, which makes you further appreciate the beauty of OOP. -
Well I am not saying large applications will actually run faster with frameworks, there is always an overhead cost for frameworks especially ORM. I am merely suggesting that the difference in performance is exaggerated since most do not really write a complete object-oriented software to compare the speed. Large applications with proper optimization will still run slower with frameworks like Zend, but wont be 4-6 times slower. You can say its slow, but its not notoriously slow.
-
Well OP may want to take a look at this article, the comments are quite worth reading too. http://blog.ircmaxell.com/2012/07/oop-vs-procedural-code.html But regarding your class, yes Jess is right that your SQL statement is wrong. It may be a good idea to use a query object to build queries if you are prone to mysql syntax errors/typos.
-
One problem with these benchmark articles is that a lot of them(cant say all, but definitely most) use simple script like 'hello world' or a small application to test the performance. Sure it is easy and less time-consuming to write performance tests, but this gives misleading results as large frameworks tend to be way too slow. In reality larger applications the difference becomes less and less significant, and using complex frameworks turns out to be more beneficial the bigger your application becomes. Truth be told, if your script only displays a sentence of hello world on the screen, you are better off just using HTML. Frameworks are for sites/applications that expect to grow large in future, they are not for extremely small fansites that do not even need OOP to survive.
-
Well if you are very new to PHP, congratulations, you are able to write decent OOPHP in just a short period of time. Keep up the excellent work, you will improve from an amateur to professional coder soon. A friendly advice though, use netbeans or eclipse to load your script and it can detect syntax errors immediately for you. In most cases the blank page is caused by syntax errors, in a large program it may not be easily detectable. If there is no syntax error, you will have to enable error reporting and track the problem deep down.
-
If string contains #mudder Add to the database
Hall of Famer replied to runnerjp's topic in PHP Coding Help
Nope, I'd say it was the constructor method below, which uses PHP4 signature instead of public function __construct($username, $count = 20). function myTimeline($username, $count = 20) { $this->feedUrl = 'http://api.twitter.com/1/statuses/user_timeline/'.$username.'.rss'; $this->count = $count > 20 ? 20 : $count; $this->username = $username; }