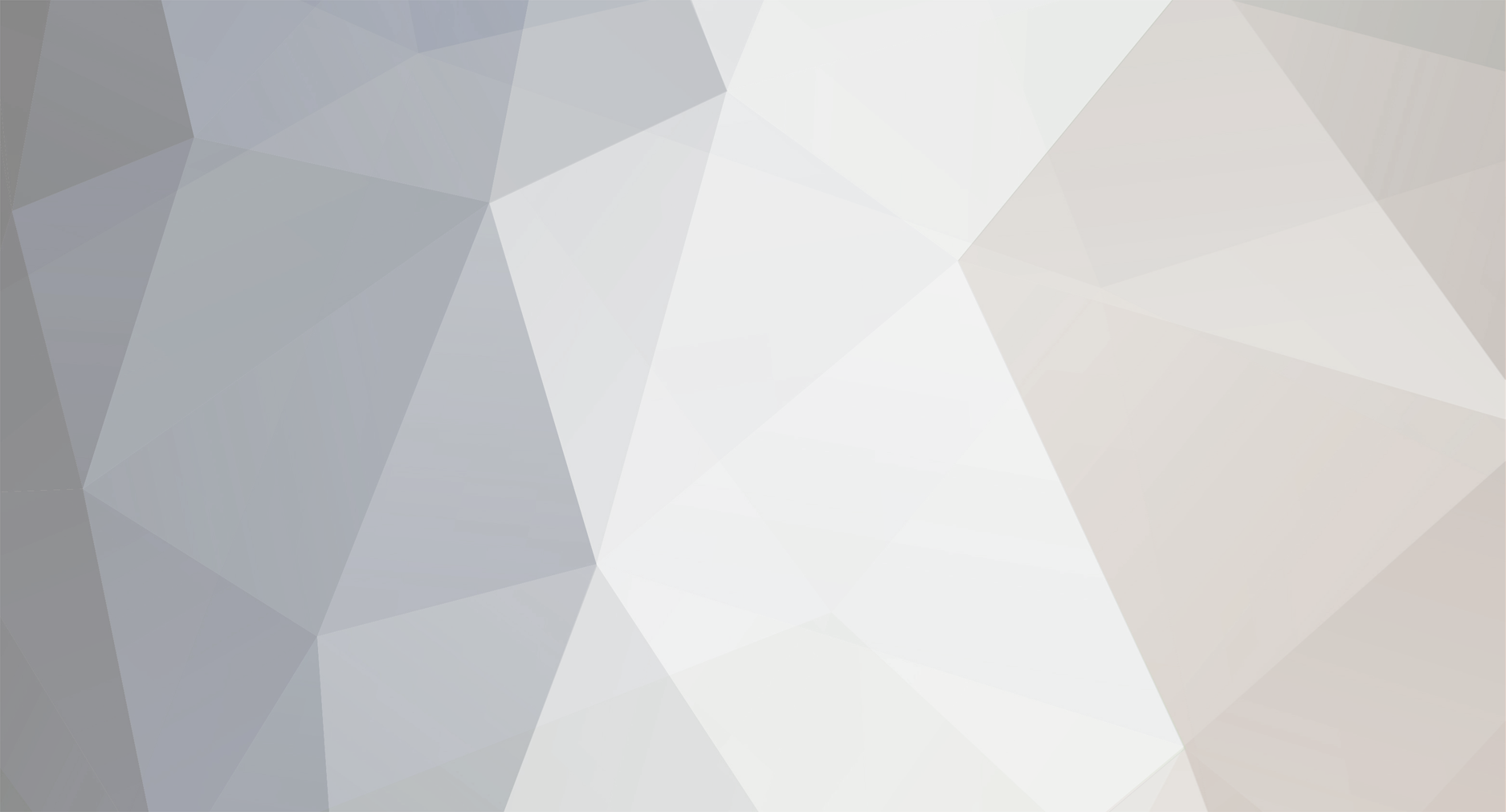
Philip
Staff Alumni-
Posts
4,665 -
Joined
-
Last visited
-
Days Won
20
Everything posted by Philip
-
You're gathering the value in your JS instead of checking to see if it's checked or not. Instead of .value, you need to use .checked on the checkboxes.
-
Yeah, there would only be one matching row. It just seems like its doing a lot of work, but I guess it's okay.
-
Okay guys, I can do it this query using subqueries - but I'm wondering if there is a more efficient way to do this: Settings table: VariableValue Setting11 Setting2999 Members Table: IDNameCode1Code2 1Phil751000 2Bob065 3Joe53210 Let's say I want to: Select members.id, members.name from members, settings where members.code1 > settings.setting1 and members.code2 < settings.setting Right now, above query is obviously invalid, since the field name is "Variable" and "Value" (and not "Setting1"/"Setting2"), so following works: SELECT `id`, `name` FROM `members` WHERE code1 > (SELECT `value` FROM `settings` WHERE `variable`='Setting1') AND code2 < (SELECT `value` FROM `settings` WHERE `variable`='Setting2') Which would return ID 3 - Joe. Is there any way to simplify or make the above query more efficient. The real query I want to do would have 4+ sub queries, which I know probably wouldn't be too ideal, performance wise. Or is it okay to multiple subqueries?
-
.name { Put main stuff here } .sub1 { little changes between types } .sub2 { little changes between types } class="name sub1" class="name sub2"
-
Well, if you're wanting it to return PHP code and then execute it on the first page - it won't. Remember, PHP is server side, not client side like javascript. Maybe I'm still not understanding it?
-
I'm not sure what you're really wanting, when you say "send post data into the div tags" - do you want to show the variables you're sending or receiving show into a div area?
-
Well, you could still use GET, but you're going to eventually be limited by the size of the text. Or we can switch to POST, which would allow a lot more text sent. Using post is just as easy as GET, but allows for more flexibility. Your js code: function ajaxFunction() { var ajaxRequest; // The variable that makes Ajax possible! try{ // Opera 8.0+, Firefox, Safari ajaxRequest = new XMLHttpRequest(); } catch (e){ // Internet Explorer Browsers try{ ajaxRequest = new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { try{ ajaxRequest = new ActiveXObject("Microsoft.XMLHTTP"); } catch (e){ // Something went wrong alert("Your browser broke!"); return false; } } } // Get our variables into nice variable names var title = document.getElementById("title").value; var typ = document.getElementById("typ").value; var story = document.getElementById("story").value; var queryString = "title=" + title + "&typ=" + typ + "&story=" + story; // Start the Request process ajaxRequest.onreadystatechange=function(){ // This function will run whenever PHP says something back to our script. if (ajaxRequest.readyState==4 && ajaxRequest.status==200){ // If the ready state is final return, and the http status is OK alert(ajaxRequest.responseText); /* Of course you could change the above to show in a div on the page but for now, we'll just show it in an alert box */ } } /* THIS IS WHAT WILL CHANGE FROM GET -> POST */ // Open the page, ready it for posting. ajaxRequest.open("POST", "php_ajax_input_script.php", true); // Lets tell the header what we are ajaxRequest.setRequestHeader("Content-type", "application/x-www-form-urlencoded"); // Now let's send the parameters. ajaxRequest.send(queryString); } -- In your PHP script (<? and ?> just for highlighting), change: <?php $title = $_GET['title']; $newstype = $_GET['typ']; $news = $_GET['story']; $poster = "whatever"; ?> <?php $title = $_POST['title']; $newstype = $_POST['typ']; $news = $_POST['story']; $poster = "whatever"; ?> Oh and the typos: <?php $title = mysql_real_escape_string($title); $news = mysql_real_escape_strong($title); ?> to: <?php $title = mysql_real_escape_string($title); $news = mysql_real_escape_string($news); // notice the $news instead of $title in the second string ?>
-
Okay, since I can't see anything wrong at the moment - let's see what PHP says when it returns. Your js should be now: function ajaxFunction() { var ajaxRequest; // The variable that makes Ajax possible! try{ // Opera 8.0+, Firefox, Safari ajaxRequest = new XMLHttpRequest(); } catch (e){ // Internet Explorer Browsers try{ ajaxRequest = new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { try{ ajaxRequest = new ActiveXObject("Microsoft.XMLHTTP"); } catch (e){ // Something went wrong alert("Your browser broke!"); return false; } } } // Get our variables into nice variable names var title = document.getElementById("title").value; var typ = document.getElementById("typ").value; var story = document.getElementById("story").value; var queryString = "?title=" + title + "&typ=" + typ + "&story=" + story; alert(queryString); // See what the queryString says // Start the Request process ajaxRequest.onreadystatechange=function(){ // This function will run whenever PHP says something back to our script. if (ajaxRequest.readyState==4 && ajaxRequest.status==200){ // If the ready state is final return, and the http status is OK alert(ajaxRequest.responseText); /* Of course you could change the above to show in a div on the page but for now, we'll just show it in an alert box */ } } // Open the page via get ajaxRequest.open("GET", "php_ajax_input_script.php" + queryString, true); // We don't need to send anything, since we're using GET and not POST ajaxRequest.send(null); } I added in the onreadystatechange function - which will run whenever PHP returns a value
-
You might want to edit out your password
-
Can you post the whole request code? There might be something up with it
-
Your input id doesnt match what Javascript is looking for. var title = document.getElementById('title').value; Should be: var title = document.getElementById('titles').value; Also, move the alert(queryString); above the line ajaxRequest.open("GET", "php_ajax_input_script.php" + queryString, true); Now after you run it - check in the database to see if anything is in there. There should be something.
-
[SOLVED] using XML to take ease off database?
Philip replied to XpertWorlock's topic in Javascript Help
Yeah, that's what I would do. I'm no server load guru or anything, but it would make sense to split it up. Like you said, if you have 5000 users, its highly unlikely that they will be loading the same file at the same time. Whereas with mysql, you could run into a 'too many connections' error, even if they aren't loading the same part of the map. I'd recommend putting something like 9 squares of the map in each xml file (you decide how big the squares are) - that way when the user walks into an outside square you know which direction they are heading and you can preload the next images. Example (look at your numpad): 1 2 3 4 5 6 7 8 9 -
[SOLVED] using XML to take ease off database?
Philip replied to XpertWorlock's topic in Javascript Help
Thats why you could break it up into sections, not all into one file. -
[SOLVED] using XML to take ease off database?
Philip replied to XpertWorlock's topic in Javascript Help
Using xml would probably be better in this case - unless you were interacting with other users, then it could get complicated and I would switch to database. -
Yeah, as xtopolis suggested, get a great Javascript book, not ajax specific. If you know how to do some server side scripting, then you're set with just getting a JS book. I have Javascript The Definitive Guide from O'Reilly, and it's pretty handy to have around
-
I'd suggest reading the working example in this forum http://www.phpfreaks.com/forums/index.php/topic,115581.0.html There are 2 different ways to send values from a form to a script. First is with GET, where the variables are in the url. Downfalls of using this are limited sending space, each browser limits how long the url can be. Nonetheless, an example: foo.com/bar.php?variable=value Second is with POST, where the variables are sent in the HTTP headers. I believe the max you can send is 2MB of data. (correct me if I'm wrong somebody.) Whichever way you decide, make sure both ends match.
-
This should be a good article to read up on: http://jennifermadden.com/javascript/dhtml/dynamicLayerContent.html
-
$_POST['title'] vs $_GET['title'] are 2 different things, considering your ajax code is using GET: XMLHttpRequestObject.open("GET", dataSource);
-
I typically do the input name + the number, for both the name/id So, you have a dropdown for X amount of "names" <input name='name1' id='name1'> <input name='name2' id='name2'> .... Then <?php if(isset($_POST['name1']) && !empty($_POST['name1'])) { // stuff } // OR // Change $i = to your max num of inputs you have. for($i=3; $i>0; $i--) { $name = 'name'.$i; // Set the name correctly, and then see if that variable is set. if(isset($_POST[$name]) && !empty($_POST[$name])) { // do stuff } } Just one way of many to do that.
-
Why not just <?php //php stuff here ?> HTML STUFF <?php //more php ?>
-
Okay I see your problem now. You're pushing GET variables (the ones in the URL: somepage.php?var1=value) and not POST variables. You can do POST in ajax, its just a little harder. http://www.openjs.com/articles/ajax_xmlhttp_using_post.php
-
adding checkbox value to php array on click
Philip replied to tryingtolearn's topic in Javascript Help
Well, you wouldn't put all of the checkboxes into the URL. Just the ones from the recent page. Then store them into a session array. Whenever your pulling the results - see if the checkbox ID is in the array, if so echo out CHECKED, else do nothing. -
adding checkbox value to php array on click
Philip replied to tryingtolearn's topic in Javascript Help
How are you getting the next page, by submitting a form, or by clicking a link? -
So, the backend side of it - does it require any inputs? Right now, dataSource is just upalbum.php - do you need to post any variables to get a return? Also, try putting in an alert to see if it is even sending the data XMLHttpRequestObject.onreadystatechange = function() { alert("State: "+XMLHttpRequestObject.readyState); if(XMLHttpRequestObject.readyState == 1 && XMLHttpRequestObject.status == 200) { obj.innerHTML = '<img src="../images/loading.gif" />'; } if(XMLHttpRequestObject.readyState == 4 && XMLHttpRequestObject.status == 200) { obj.innerHTML = XMLHttpRequestObject.responseText; } }
-
Yes, look into using ajax, its exactly what you're going to want to use. Login info (via JS) -> PHP check response -> Display error/success (via JS) However, if you did, make sure your users can still login if their browser has javascript disabled.