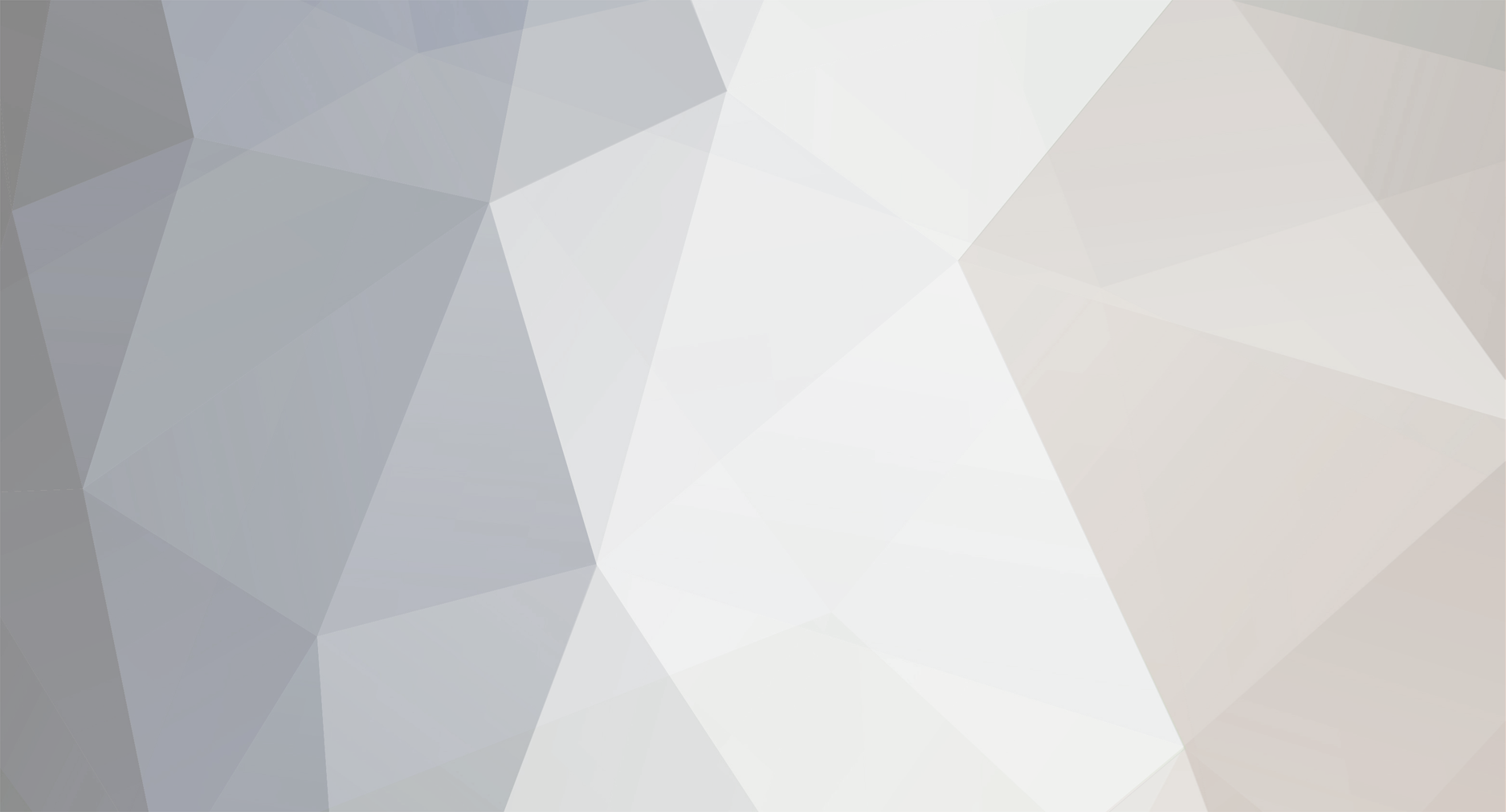
QuickOldCar
Staff Alumni-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Everything posted by QuickOldCar
-
Is your question how to connect to a site and scrape particular data from it...then save.
-
Seems simple enough to use a single table and create a field for each variable. No reason to do different tables and joins on this. The sorting is done either by dynamic variables in the database query or manually inserting one. "SELECT * FROM `computer_db` WHERE `processor` LIKE '%{$processor}' " "SELECT * FROM `computer_db` WHERE `processor` = '{$processor}' AND `ram` = '{$ram}' "
-
As long as the others didn't actually steal the car, I'm sure you can get a claim from their insurance. They will find whoever owns the car. A girl growing up near me stole her parents car while drinking at age 16, during her joyride she wiped out 12 cars. Her parents had to pay for all damages and she couldn't get her license until age 21. She ran as well.
-
First I will take some time and try to explain api's as best I can by my experience and knowledge about them. Everything I mention can be further researched. REST : Representational state transfer (my simple definition) Uses url or uri over http web The data set to be retrieved could use the url,uri or any query parameter and or their values to access a particular data set. Can use GET, PUT, POST, or DELETE http methods Do local server actions with them or send out a response to a client or server. You can literally return any type of response you want, some popular ones used in api's would be json,xml and html The format of the response can also be determined by a url parameter in the query, such as format=json,format=xml,format=html XML-RPC : (very old) remote procedure call using xml to encode and http transport SOAP : (very basic summary) soap evolved from xml-rpc xml based Has an envelope for defining the message structure and how to process it. Encoding rules to determine each instance of application data types. A method to handle procedure calls and responses. Uses HTTP, SMTP, TCP, UDP, and JMS transport protocols. Create full documentation of your api, your clients need to know how to access your api, what the parameters mean and also any options. If you need to have access keys take a look at oauth A lot of sites that supply an api have information how to obtain and show data. such as: https://developers.google.com/youtube/code https://www.flickr.com/services/api/ Some will have example scripts in a few coding languages. They could even have different resposes such as json,xml,html. Select what you need your particular project that suits your needs. Besides an api, you could also make scripts that can grab from their meta tags, opengraph or oembed per website url. It's also possible to grab from the rss feed data, but over my years have found there is too much malformed xml in them. There is also an issue must detect if is rss 1 or 2, blah, was a nice idea, but not everyone did it nor correct or similar. (coming from a guy that made his own feed aggregator and feedreaders) But if you were interested in feed related take a look at simplepie or magpie Connect to a website: Is various ways to connect to websites. This all varies on the type of data and complexity of what are trying to send or retrieve. curl would be my preference over all of them, you have lots more control over everything. A simple way to connect would be using file_get_contents() For xml can use simplexml_load_file() , I prefer to use curl for connection and responses, then simplexml_load_string() to create an object Once you get the raw data, you need to access the items you want. This would be called parsing. json simplexml dom simplehtmldom preg_match() and preg_match_all() Once you have the data you can either output data to a client or save to your database. Now follow normal website and search methods. As for your question: If the api supports query parameters, you would pass your search terms into there...return or display that particular response. If you want, you can play with my video search api's. Currently they are public requiring no key. In the future they will be domain restricted and public key required. I will explain it all, provide codes how to connect and retrieve the data. Dynavid Video Search using a REST api List of parameters and their options: parameter: [ s ] value: [ word | word +word | +word +word | +word +word] many multiple words accepted default: [ returns latest videos ] description: [ search term keywords - uses advanced search in fulltext boolean mode, supports multiple words using spaces ability to include/exclude by using +/- ] parameter: [ size ] value: [ 100 to 1280 ] default: [ 400 ] description: [ video embed size - optional sizing for video in only html format mode ] parameter: [ startrow ] value: [ 0 to undetermined ] default: [ 0 ] description: [ determines what row to start from in database ] parameter: [ max ] value: [ 1 to 50 ] default: [ 10 ] description: [ maximum number of results shown in data set ] parameter: [ display ] value: [ id | title ] default: [ id ] description: [ group the order of results different ways ] parameter: [ order ] value: [ asc | desc ] default: [ desc ] description: [ direction of the results ascending or descending order ] parameter: [ format ] value: [ json | xml | html ] default: [ json ] description: [ output response format ] 3 links same data showing different formats json response: To me this is best method and always have clean data http://dynainternet.com/dynavid/search/index.php?s=documentary&startrow=0&max=5&display=id&order=desc&format=json xml response: xml tree with tags and encoded data http://dynainternet.com/dynavid/search/index.php?s=documentary&startrow=0&max=5&display=id&order=desc&format=xml html response: for direct display or iframing http://dynainternet.com/dynavid/search/index.php?s=documentary&startrow=0&max=5&display=id&order=desc&format=html We will use the html format and show how can manipulate the parameters search horror movie http://dynainternet.com/dynavid/search/index.php?s=horror+movie&size=400&startrow=0&max=5&display=id&order=desc&format=html change the video size http://dynainternet.com/dynavid/search/index.php?s=+horror++movie&size=800&startrow=0&max=5&display=id&order=desc&format=html start at database row 1000 http://dynainternet.com/dynavid/search/index.php?s=+horror++movie&size=400&startrow=1000&max=5&display=id&order=desc&format=html show 20 results data set http://dynainternet.com/dynavid/search/index.php?s=+horror++movie&size=400&startrow=0&max=20&display=id&order=desc&format=html group by title http://dynainternet.com/dynavid/search/index.php?s=+horror++movie&size=400&startrow=0&max=5&display=title&order=desc&format=html show ascending order grouped by title http://dynainternet.com/dynavid/search/index.php?s=+horror++movie&size=400&startrow=0&max=5&display=title&order=asc&format=html For xml parsing.....due to the complexity and websites using different namespaces,parent,children objects and so on, we'll skip that in this post. connect simplexml, retrieve xml data, convert to json and show array <?php $url = "http://dynainternet.com/dynavid/search/index.php?s=horror+movie&size=400&startrow=0&max=5&display=id&order=desc&format=xml"; $data = simplexml_load_file($url); if ($data) { $array = array(); $array = json_decode(json_encode($data), TRUE); var_dump($array); } else { echo "No data"; } ?> file_get_contents, retrieve json data and show array curl results will be similar <?php $url = "http://dynainternet.com/dynavid/search/index.php?s=horror+movie&size=400&startrow=0&max=5&display=id&order=desc&format=json"; $data = file_get_contents($url); if ($data) { $array = array(); $array = json_decode(json_encode($data),true); var_dump($array); } else { echo "No data"; } ?> As for my particular api, lets say if wanted to just grab all the video urls and display them as a hyperlink <?php $url = "http://dynainternet.com/dynavid/search/index.php?s=horror+movie&size=400&startrow=0&max=5&display=id&order=desc&format=xml"; $data = simplexml_load_file($url); if ($data) { $array = array(); $array = json_decode(json_encode($data), TRUE); //var_dump($array['url']); foreach($array['url'] as $video_url){ $video_url = urldecode($video_url); echo "<a href ='".$video_url."'>".$video_url."</a><br />"; } } else { echo "No data"; } ?> connect using simplexml and directly access the objects <?php $url = "http://dynainternet.com/dynavid/search/index.php?s=horror+movie&size=400&startrow=0&max=5&display=id&order=desc&format=xml"; $data = simplexml_load_file($url); if ($data) { //var_dump($data); $href_array = array(); foreach($data->url as $href){ $href_array[] = urldecode($href); } echo '<pre>'; print_r($href_array); echo '</pre>'; } else { echo "No data"; } ?> For more specific questions related to a certain website and their api feel free to ask questions. If your interest is in making your own api, can help with that as well.
-
http://thomasjbradley.ca/lab/signature-pad
-
Probably has a null value first check it if ($map){ if (!$map){ if ($map && $map->count() > 0) { echo "Yup"; } else { echo "No results"; }
-
Trying to modify a Search to use mysqli help
QuickOldCar replied to Mitka's topic in PHP Coding Help
Place this the top of your search.php and see if any errors error_reporting(E_ALL | E_NOTICE); ini_set('display_errors', '1'); You have a $conn instead of $con line 7 in search.php Try that and can look more if need it. -
Is it you that adds the team members? Can't you just insert the users names as Last, First Middle? Sorting the title ASC order should work fine. To change the order 'order' => 'ASC', could be 'order' => 'DESC', Realize that what I proposed will have to reverse all the current names have in database to be last,middle,first, same with permalinks. Then just for display purposes in your gallery you can reverse them again for the post_title so look like normal names. (or can do as barand suggested and leave them as Last, First Middle, no reverse on display required) example of your link now http://testing.merchantlaw.com/team/casey-r-churko/ should be http://testing.merchantlaw.com/team/churko-r-casey/ ASC order for the title should work if the last names in the database are last,middle,first. You may want to do new posts or change them all in wordpress including the pretty permalinks.
- 7 replies
-
- word press
- simple staff list
-
(and 2 more)
Tagged with:
-
Actually if are doing this in a loop from mysql results use preg_match() If it matches either echo the results or save into a new array to output after the loop. Still recommend doing a normal search either with LIKE or use fulltext boolean mode
-
Sure you can use preg_match_all() on those results and it creates a new array.. It would be better to do the proper search queries though and not have all the extra data.
-
It isn't like paint, they don't mix. They are 0 to 255. If you added them up or merged would exceed those values.
-
Password Manager... Which one you guys recommend?
QuickOldCar replied to mikosiko's topic in Miscellaneous
I keep them all on paper. Print them out from notepad or something if want multiple copies. Long ago I used a program similar and the harddrive screwed up, scratched the platter up and was gone forever. -
Printing the IP Address From Which the Site is Viewed on Screen?
QuickOldCar replied to glassfish's topic in PHP Coding Help
or add an if and replace to 127.0.0.1 if($_SERVER['REMOTE_ADDR'] == "::1" || $_SERVER['REMOTE_ADDR'] == "localhost"){ $ip = "127.0.0.1"; } else { $ip = $_SERVER['REMOTE_ADDR']; } echo "Your IP Address: ".$ip."<br/>"; -
Found the issue <form action='login.php' methody='post'> <?php error_reporting(E_ALL | E_NOTICE); ini_set('display_errors',1); session_start(); include('connect.php'); if (isset($_POST['name']) && trim($_POST['name']) != '' && isset($_POST['password']) && trim($_POST['password']) != ''){ $name = trim($_POST['name']); $password = trim($_POST['password']); $escape_name = mysql_real_escape_string($name); $escape_password = mysql_real_escape_string($password); $result = mysql_query("SELECT * FROM users WHERE user='{$escape_name}' AND password='{$escape_password}'"); if($result){ $_SESSION['name'] = $name; header("Location: admin.php"); exit(); }else{ header("Location: login.php"); exit(); } }else{ ?> <html> <head> <title>Admin Insert page!</title> </head> <body> <form action='login.php' method='post'> Username: <input type='text' name='name'/><br /> Password: <input type='password' name='password'/><br /> <input type='submit' name='submit' value='Login' /> </body> </html> <?php } ?>
-
You should not be using any mysql_* functions, instead use mysqli_* or PDO Saving the raw password into a database is not good either, but at least escape any data inserting into mysql. Try this <?php error_reporting(E_ALL | E_NOTICE); ini_set('display_errors',1); session_start(); include('connect.php'); if (isset($_POST['name']) && trim($_POST['name']) != '' && isset($_POST['password']) && trim($_POST['password']) != ''){ $name = trim($_POST['name']); $password = trim($_POST['password']); $escape_name = mysql_real_escape_string($name); $escape_password = mysql_real_escape_string($password); $result = mysql_query("SELECT * FROM users WHERE user='{$escape_name}' AND password='{$escape_password}'"); if($result){ $_SESSION['name'] = $name; header("Location: admin.php"); exit(); } }else{ ?> <html> <head> <title>Admin Insert page!</title> </head> <body> <form action='login.php' methody='post'> Username: <input type='text' name='name'/><br /> Password: <input type='password' name='password'/><br /> <input type='submit' name='submit' value='Login' /> </body> </html> <?php } ?>
-
The author of the plugin used the built in posts system instead of making a new table. If there was a new table can save each first,middle,last names would be able to query and sort by however wanted to. Essentially now you must pull results by post title and will go by first character.
- 7 replies
-
- word press
- simple staff list
-
(and 2 more)
Tagged with:
-
An easier way is to use this reverse code just gave on the $_POST["_staff_member_title"] at line 52 in admin-save-data.php Then use the same reverse code for display purposes (normal names) <?php /* // Save Post Data //////////////////////////////*/////// Register and write the AJAX callback function to actually update the posts on sort. ///// add_action( 'wp_ajax_staff_member_update_post_order', 'sslp_staff_member_update_post_order' ); function sslp_staff_member_update_post_order() { global $wpdb; $post_type = $_POST['postType']; $order = $_POST['order']; /** * Expect: $sorted = array( * menu_order => post-XX * ); */ foreach( $order as $menu_order => $post_id ) { $post_id = intval( str_ireplace( 'post-', '', $post_id ) ); $menu_order = intval($menu_order); wp_update_post( array( 'ID' => $post_id, 'menu_order' => $menu_order ) ); } die( '1' ); } ////// Save Custom Post Type Fields ////// add_action('save_post', 'sslp_save_staff_member_details'); function sslp_save_staff_member_details(){ global $post; if ( !isset( $_POST['sslp_add_edit_staff_member_noncename'] ) || !wp_verify_nonce( $_POST['sslp_add_edit_staff_member_noncename'], 'sslp_post_nonce' ) ) { return; } if ( defined( 'DOING_AUTOSAVE' ) && DOING_AUTOSAVE ) return $post->ID; $name_parts = array(); $name_parts = explode(' ',trim($_POST["_staff_member_title"])); $name_parts = array_reverse($name_parts); $reverse_name = implode(" ",$name_parts); update_post_meta($post->ID, "_staff_member_bio", $_POST["_staff_member_bio"]); update_post_meta($post->ID, "_staff_member_title", $reverse_name); update_post_meta($post->ID, "_staff_member_email", $_POST["_staff_member_email"]); update_post_meta($post->ID, "_staff_member_phone", $_POST["_staff_member_phone"]); update_post_meta($post->ID, "_staff_member_fb", $_POST["_staff_member_fb"]); update_post_meta($post->ID, "_staff_member_tw", $_POST["_staff_member_tw"]); } ?>
- 7 replies
-
- word press
- simple staff list
-
(and 2 more)
Tagged with:
-
Here is how you can reverse the first and last names. <?php $name = "Firstname Middlename Lastname"; $name_parts = array(); $name_parts = explode(' ',trim($name)); $name_parts = array_reverse($name_parts); $reverse_name = implode(" ",$name_parts); echo $reverse_name; ?>
- 7 replies
-
- word press
- simple staff list
-
(and 2 more)
Tagged with:
-
Php itself can not do it, you must exec something else to do it on the server. Some sort of actionscript or java. commandline to a program that can do it. Have a look at this and try it. http://sourceforge.net/projects/flazr/
-
Does your server have mod_rewrite module enabled? Did you create the pagination yourself? Any additional info could help, such as the pagination code or if it's a plugin are using, your .htaccess rules
- 1 reply
-
- wordpress
- custom page
-
(and 1 more)
Tagged with:
-
New to PHP, looking for a couple basic fixes
QuickOldCar replied to dd4814's topic in PHP Coding Help
A lot of what you want seems to be more style related like css. Your module most likely has css file you can edit. Is piles of css tutorials and examples on the net. You can use style right there if wanted to though. define('MODULE_PAYMENT_PAYPALWPP_MARK_BUTTON_TXT', "<b>Checkout with PayPal.</b>"); Can't you just replace the image with yours? define('MODULE_PAYMENT_PAYPALEC_MARK_BUTTON_IMG', 'https://www.anywebsite.com/myimage.png'); Really you should place that image inside a divider or span, if it already is then make a class for it for style Just an example, no idea how need this styled or positioned. echo "<div style='position:relative;top:200px;right:50px;margin:0;padding:2px;border:0;'><image width='70px' src='https://www.anywebsite.com/myimage.png' /></div>"; -
Multiple feeds into wordpress better way to do this?
QuickOldCar replied to flyBeta's topic in PHP Coding Help
I used to run like 1,000 or so feeds auto posting through a multi-wordpress years ago. Had each subdomain a main category, imported categories and tags from original articles. The server at that time was around 120 a month (7 years ago?), it handled it fine, Intel quad Q6700 and used 4 out of the 8 gig available memory. Page caching for the visitor traffic was a necessity. I would imagine that doing this on a shared host with low specs will have issues. For what you are planning seems like nothing less than a dedicated server and well planned out. Constantly hitting feeds and new posts will certainly use lots of resources. To me wordpress got severely bloated and not as good as used to be. Consider making your own rss aggregator using simplexml. Here is a simple example using simplxml and getting the results into an array so you can then insert into a database. <?php $url = "http://science.jobs.net/RSS/"; $xml = @simplexml_load_file($url); if($xml){ $json = json_encode($xml); $array = array(); $array = json_decode($json,TRUE); echo "<pre>"; print_r($array); echo "</pre>"; } else { echo "No Data"; } ?> Feel free to use my feed search to find feed urls. http://dynaindex.com/feed-urls.php?search=jobs -
Take a look at ffmpeg and ffserver instead, well documented.
-
Wordpress, Gravity Forms, and RepairShopr API
QuickOldCar replied to klee1981's topic in PHP Coding Help
To concatenate the $_POST data you do it like this $ticket_subject = $_POST['device'] . " - " . $_POST['phonemake']; Up higher in the form I would check all these post values and make a string variable. Can even do an array and implode characters between each additional if you wish. $ticket_subject = ''; if(isset($_POST['device']) && trim($_POST['device']) != ''){ $ticket_subject .= trim($_POST['device'] . " - "; } if(isset($_POST['phonemake']) && trim($_POST['phonemake']) != ''){ $ticket_subject .= trim($_POST['phonemake'] . " - "; } $ticket_subject = rtrim(trim($ticket_subject), "-"); Then you have this 'ticket_subject' => $ticket_subject,