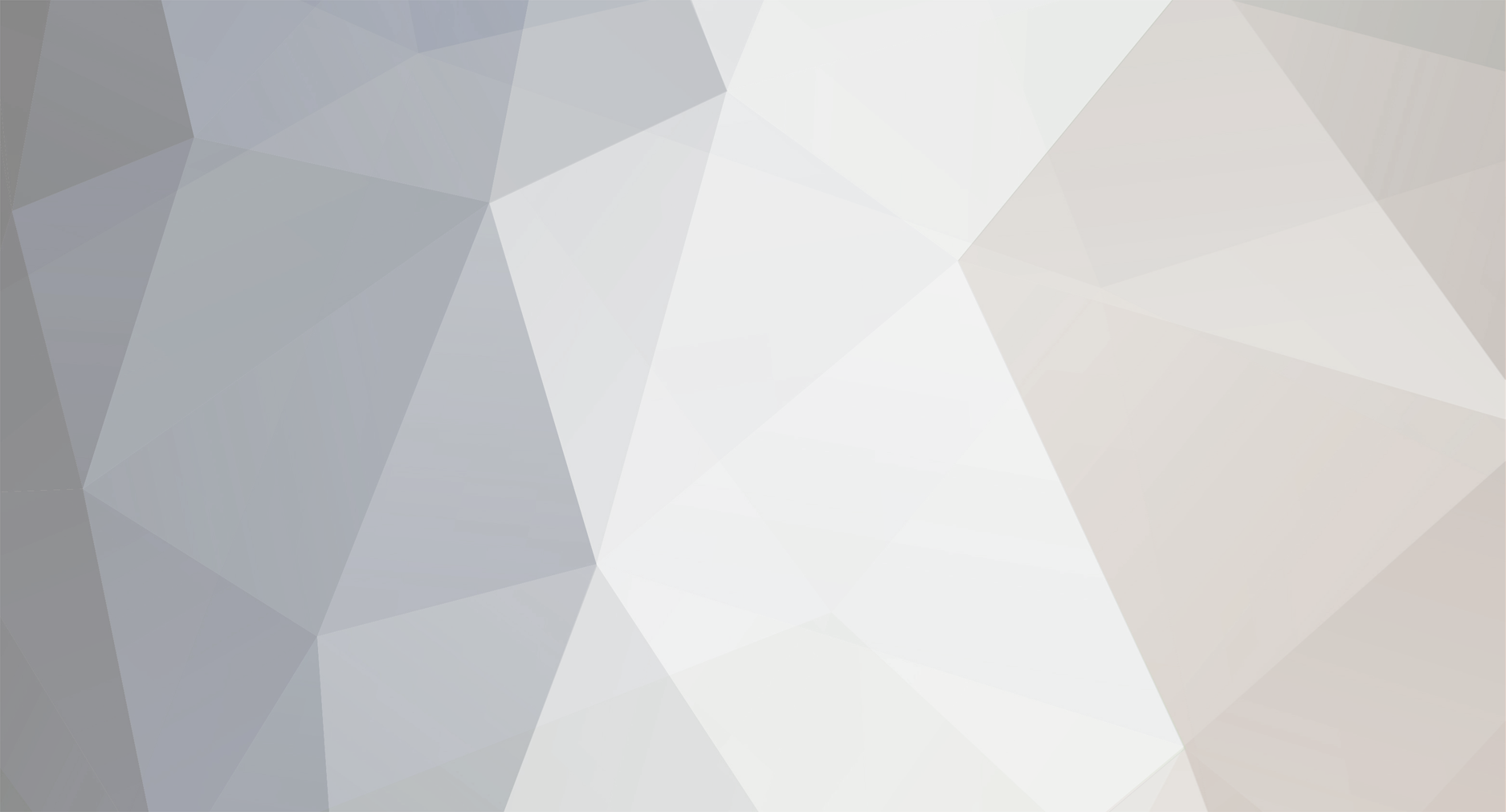
QuickOldCar
Staff Alumni-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Everything posted by QuickOldCar
-
What the other guys said is true. My suggestion would be to use a full-text search, even install sphinx search It's silly trying to categorize every single word and also multiple words in any combination. Instead you can make some search terms that are popular and provide some links in a sidebar or something. Then also have the ability to search anything else. Onto one of your questions as to how to make a letter and number navigation... $characters_array = array("0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"); foreach ($characters_array as $characters) { $upper_char = strtoupper($characters); echo " <a href='yourscript.php?search=".$characters."'>[".$upper_char."]</a> "; } if(isset($_GET['search']) && trim($_GET['search']) != ''){ $search = strtolower(trim($_GET['search'])); //then some sort of query matching results by just the first character $query = "SELECT * from videos WHERE title LIKE '". mysql_real_escape_string($search) ."%'"; } I didn't bother doing the database connection or while loop, you should really not be using any mysql_* functions but using PDO prepared statements or mysqli_* functions If collation of your column is set as _ci, that means is case insensitive meaning a and A will work the same in the LIKE and return both sets of results. If is not set to that you need to run 2 like statements for uppercase and lowercase...or change your column collation to _ci
-
Welcome aboard Maria. Browsing around here seeing others mistakes and then solutions will help a lot. Occasionally some members are nice enough to write something out for you and help you along every issue. Follow the great advice given here and will become easier.
-
I wish php was around when I started programming, I was 8 and had to do dos. Wasn't much to call an internet at that time anyway. I'd have to say php is my favorite of every programming language I ever did. Most of the time is fun. Lucky kid.
-
After many years of coding I try for absolute silence. I distract easily and anything can become an excuse.
-
How do I check the statuses of the YouTube videos in my database?
QuickOldCar replied to Fluoresce's topic in PHP Coding Help
I noticed doing id's, missed that. Wanted to say it's most likely youtubes api limits is why seeing them unavailable. If you have many of them it's better to chunk the data and do so many at a time, mark them somehow in the database. Try this out, will show what ones are dead by id and json responses <?php function checkDeadYoutube($yt_id) { if(!$yt_id || trim($yt_id) == '') { echo "<a href='http://www.youtube.com/watch?v=".$yt_id."'target='_blank'>No url inserted</a><br />"; } $oembed_json = "http://www.youtube.com/oembed?url=http%3A%2F%2Fwww.youtube.com%2Fwatch%3Fv%3D" . $yt_id . "&format=json"; $json = json_decode(@file_get_contents($oembed_json)); if (!$json || $json == null) { echo $yt_id." - <a href='http://www.youtube.com/watch?v=".$yt_id."'target='_blank'>Unable to connect</a><br />"; } // echo '<pre>'.print_r($json,true).'</pre>'; $title = trim($json->title); if ($title == "Youtube") { echo $yt_id." - <a href='http://www.youtube.com/watch?v=".$yt_id."'target='_blank'>".$title."</a><br />"; } } //example array $youtube_ids = array("XW99sBf4BUI","MkXVM6ad9nI","baaad","ou2Qk2D_Y4w","hX_yRbpCNVo","73e-tCWydFE","lytzFvAfRRk"); foreach($youtube_ids as $id){ checkDeadYoutube($id); } ?> -
How do I check the statuses of the YouTube videos in my database?
QuickOldCar replied to Fluoresce's topic in PHP Coding Help
Youtube has opengraph and oembed data can look at. Youtube always returns a 200 response, they change the content on the page. If the title is "Youtube" it's dead. You can preg_match the opengraph for title Use urls similar to these for json or xml results for oembed http://www.youtube.com/oembed?url=http%3A%2F%2Fwww.youtube.com%2Fwatch%3Fv%3DfnpNFvcJS2k&format=json http://www.youtube.com/oembed?url=http%3A%2F%2Fwww.youtube.com%2Fwatch%3Fv%3DfnpNFvcJS2k&format=xml Can spend a few days each method and cleaning youtube urls to all be standard or just grabbing the id's. Here is a simple function to show a message or a video using json oembed <?php function checkDeadYoutube($url) { if(!$url || trim($url) == '') { echo "No url inserted"; exit(); } $oembed_json = "http://www.youtube.com/oembed?url=" . urlencode($url) . "&format=json"; $json = json_decode(@file_get_contents($oembed_json)); if (!$json || $json == null) { echo "Unable to connect"; exit(); } // echo '<pre>'.print_r($json,true).'</pre>'; $title = trim($json->title); $video = $json->html; if ($title != "Youtube") { echo $video; } else { echo "Dead Video"; } } // usage checkDeadYoutube("http://www.youtube.com/watch?v=fnpNFvcJS2k"); ?> -
So you are placing a pile of text into a form and trying to get all the relevant data. In some of those lines it may or may not contain multiple whitespace. This is the first place where I see you grab all the lines, so try this here Replaces any whitespace if more than one with a single whitespace. foreach ($file as $k) { $k = preg_replace('/\s+/', ' ',trim($k)); If still have problem areas add a trim as well. Is some questionable statements in this script if ($v == "") continue; what else would it do? $v = explode (" ", $v); now $v variable is $v array if (count($v) != 2) die("Incorrect values in POINTS input (check spaces!)<br>"); is it ever not 2? what about the rest of data when it dies? if (strstr(strtolower($v[1]), 'points')) continue; so checking if $v[1] equals points, will it ever not be? maybe should be checking all the $v array for what you want? lets say want to find if $v[0] is actually a number, then that is players points, otherwise is zero is_numeric() for numbers including decimals ctype_digit() for just numbers if(ctype_digit(trim($v[0]))){ $player_points = trim($v[0]); } else { $player_points = 0; } Maybe just grab the number from $v array and make it points if not sure is in 1st position? Be better to know exactly where and what is needed, but here it is anyway. foreach($v as $val){ $val = trim($val); if(ctype_digit($val)){ $player_points = $val; } else { $player_points = 0;//or handle this another way } }
-
That script is exploding many times and sometimes by spaces. There won't be an easy one time and all values get trimmed. Your best bet is to just wrap any variables directly before the insert query with trim() Additionally can also use mysql_real_escape_string() at the same time. I'll do the first one as an example, and didn't look for blank values or certain data types $c_Host = mysql_real_escape_string(trim($Host)); $c_At = mysql_real_escape_string(trim($At)); $c_PC = mysql_real_escape_string(trim($PC)); $c_p1 = mysql_real_escape_string(trim($p1)); $c_e1 = mysql_real_escape_string(trim($e1)); $c_p2 = mysql_real_escape_string(trim($p2)); $c_e2 = mysql_real_escape_string(trim($e2)); $c_p3 = mysql_real_escape_string(trim($p3)); $c_e3 = mysql_real_escape_string(trim($e3)); $c_p23 = mysql_real_escape_string(trim($p23)); $c_e23 = mysql_real_escape_string(trim($e23)); mysql_query("insert into tourne_report values ('$c_Host', '$c_At', $c_PC, '$c_p1', '$c_e1', '$c_p2', '$c_e2', '$c_p3', '$c_e3', '$c_p23', '$c_e23')") or die (mysql_error());
-
What happens when try http://127.0.0.1/phpmyadmin Additionally another service could be using your ports, make sure apache and mysql start first then any others may need. Programs like skype or teamviewer
-
In addition are missing the mysql_query() so not saving them to database $sql=mysql_query("INSERT INTO images (url) VALUES ('$num')"); if (!$sql) { die('Error: ' . mysql_error()); }
-
Besides using outdated mysql_* versus PDO or mysqli_*... You should always sanitize/filter/escape all data saving to your database. Even doing checks for the proper data you expect.
-
Then you should post your code where you create these values, are they from a form like $_POST? $_POST = array_map('trim', $_POST); Now the array named $_POST would all be trimmed, or in a foreach loop. foreach($_POST as $key => $val){ $_POST[$key] = trim($val); }
-
This person does and looks like explains it all. http://kencochrane.net/blog/2012/01/developers-guide-to-pci-compliant-web-applications/
-
how to get the page count of a tiff and pdf file
QuickOldCar replied to my3cents's topic in PHP Coding Help
Using ImageMagick identify (for multi-tiff, pdf is a lot slower with this) Using packages xpdf-utils/xpdf-tools or poppler/poppler-utils/poppler-tools pdfinfo (for pdf and lots faster) -
http://dev.maxmind.com/geoip/
-
That site you linked to must use a youtube downloader such as youtube-dl on their host. Then grab x many frames with ffmpeg/avconv and turn it into a gif file. For a very basic tutorial showing some code. http://www.atrixnet.com/animated-gifs/ To me is a large hassle to go through all this for a preview animated gif image. You could just show an image preview using one of the following urls as the image source. Use the video id where see red. http://img.youtube.com/vi/youtube_video_id/0.jpg http://img.youtube.com/vi/youtube_video_id/1.jpg http://img.youtube.com/vi/youtube_video_id/2.jpg http://img.youtube.com/vi/youtube_video_id/3.jpg http://img.youtube.com/vi/youtube_video_id/default.jpg http://img.youtube.com/vi/youtube_video_id/hqdefault.jpg http://img.youtube.com/vi/youtube_video_id/mqdefault.jpg http://img.youtube.com/vi/youtube_video_id/sddefault.jpg http://img.youtube.com/vi/youtube_video_id/maxresdefault.jpg
-
You should always get permission grabbing other websites data. If they want to share it they can tell or make you methods to access it.
- 1 reply
-
- php
- server-side
-
(and 2 more)
Tagged with:
-
True, but lots of hosts use older php versions.
-
Requesting suggestion about my programming future
QuickOldCar replied to Digitizer's topic in Miscellaneous
I would have told that professor that most diamonds are not formed from coal. I don't believe age matters at all. It's knowledge and the ability to get tasks completed efficiently and correct that will get you places. The schooling with the paper helps you land a job. You can go your own route and just do freelancing. -
Use full tags <?php and not short <? tags in your post title. Ha, I thought that was amusing, welcome.
-
I know this is an older post, but I didn't see any replies. When it comes to coding a lot can not be copyrighted. It would be difficult to do something new that isn't already copyrighted by someone previously. Unless you have something spectacular I wouldn't bother about the copyright aspect. Even small changes it becomes new code, but in that same respect if examined as the whole could fall into a previous copyright. If it was a language that compiles would be options to distribute the object code versus the source code. Try to focus on contracts and licensing agreements. Something like they can use the code but not have any rights to the code itself as in using parts of it or reselling any of it. Possibly another option is to let them have the code and able to modify it for a price. If you are trying to protect scripts is pretty hard. You can try obfuscation to make it more difficult to duplicate. Force a section of important code to be included from your server upon usage...otherwise it doesn't work. To me the best way to protect your code is to never share it. Try turning it into a web service if possible. Something like an api or generated code they access with a key and/or domain restriciton.
-
This is easier echo "<tr><td>".$i."</td><td>".$v['player']."</td><td>".$v['points']."</td></tr><br />";
-
Not fetching player from the table so it won't show as $v['player'] in the results You are returning the sum only Use a subquery, * is all...you can return only what you need $r = mysql_query("select *,(select sum(Points) as total ) from player_points where date_entered > '$d' Group by player order by total DESC") or trigger_error(mysql_error());
-
It's most likely a js issue and hashtag/fragments are being ignored. One should have approval from the website owner to scrape their data. I read their link policy which is pretty strict, i doubt they want people scraping information. http://www.window.state.tx.us/linkpolicy.html