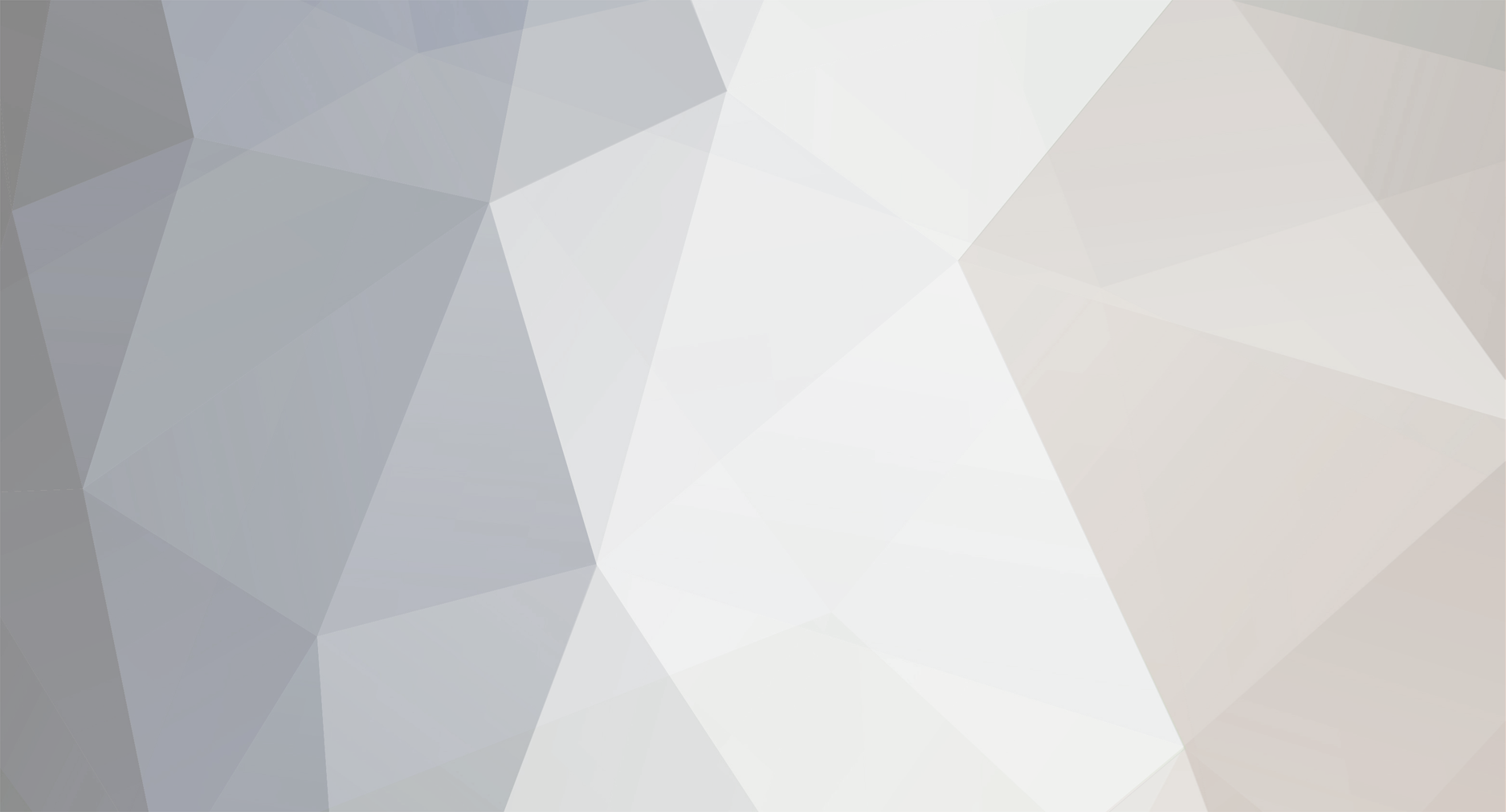
QuickOldCar
Staff Alumni-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Everything posted by QuickOldCar
-
trying a simple login form with complicated results
QuickOldCar replied to jacko_162's topic in PHP Coding Help
You marked this as solved. For one.....never store any type of passwords in a session $_SESSION['password']= $password; I usually use a 1-9 number system for user roles, 9 being admin, 1 is a user, between can specify any other abilities. If not logged in is considered a guest. I think you are complicating things more than needed, creating the following 3 sessions should be fine $_SESSION['user_name'] $_SESSION['user_level'] $_SESSION['user_logged'] $_SESSION['user_name'] = $role['username']; if($role['accessLevel'] == "admin") { $_SESSION['user_level'] = "admin"; $_SESSION['user_logged'] = true; header('Location: index.php'); exit(); } elseif($role['accessLevel'] == "member") { $_SESSION['user_level'] = "member"; $_SESSION['user_logged'] = true; header('Location: tasks.php'); exit(); } else { $_SESSION['user_level'] = "none"; $_SESSION['user_logged'] = false; header('Location: notActive.php'); exit(); } I would also place session_start(); at the top You can check if a user already logged in or not and redirect them elsewhere session_start(); if(isset($_SESSION['user_logged']) && $_SESSION['user_logged'] == true){ header('Location: index.php'); exit(); } You can look here a post I did the other day for a registration form that includes showing some errors. http://forums.phpfreaks.com/topic/292078-why-wont-this-go-to-mysql-form/?do=findComment&comment=1494882 -
On every comment is a "Mark Solved" lower right side. Decide the best answer or just click the last one like some others do.
-
MySQL error when single quote or double quotes are used
QuickOldCar replied to barkly's topic in PHP Coding Help
Need to wrap in curly braces $sql="INSERT INTO table (message) VALUES ('{mysqli_real_escape_string($_POST['message']}') )"; or concatenate them $sql="INSERT INTO table (message) VALUES ('" . mysqli_real_escape_string($_POST['message']) . "')"; -
You shouldn't post your real login credentials on the net This is untested, can try it out. <?php //start a session session_start(); //set your credentials for mysql $dbhostname = 'localhost'; $dbusername = 'root'; $dbpassword = 'password'; $dbdatabasename = 'database_name'; //mysqli connection $con = mysqli_connect($dbhostname, $dbusername, $dbpassword, $dbdatabasename) or die("Error " . mysqli_error($con)); $error = ''; //keep this empty //check if form was submitted if (isset($_POST['submit'])) { //checks on each form value if (isset($_POST['name']) && trim($_POST['name']) != '') { $name = strtolower(trim($_POST['name'])); //lower all usernames } else { $error .= "Name not set <br />"; } if (isset($_POST['email']) && trim($_POST['email']) != '') { if (filter_var($_POST['email'], FILTER_VALIDATE_EMAIL)) { $email = trim($_POST['email']); } else { $error .= "Email not a proper format <br />"; } } else { $error .= "Email not set <br />"; } if (isset($_POST['password']) && trim($_POST['password']) != '') { $password = crypt(trim($_POST['password'])); //encrypt the password } else { $error .= "Password not set <br />"; } if (isset($_POST['repassword']) && trim($_POST['repassword']) != '') { //check if password is the same as repassword if (trim($_POST['password']) == trim($_POST['repassword'])) { $repassword = true; } else { $error .= "Both Passwords did not match. <br />"; } } else { $error .= "Repassword not set <br />"; } //check to see if all variables are set to determine doing a query if ($name && $email && $password && $repassword) { //escape any input $e_name = mysqli_real_escape_string($name); $e_email = mysqli_real_escape_string($email); $e_password = mysqli_real_escape_string($password); //check if users name or email already exists, thus disallowing users making new accounts same email $user_query = "SELECT FROM student where name='{$e_name}' OR email='{$e_email}'"; if ($result = mysqli_query($con, $user_query)) { //return the number of rows in result set $rowcount = mysqli_num_rows($result); //if no records exist do the insert if ($rowcount < 1) { //insert query $query = "INSERT INTO student (name, email, password) VALUES ('{$e_name}', '{$e_email}', '{$e_password}')"; $insert = mysqli_query($con, $query); //check if data was inserted if ($insert) { echo "Success! Your information was added.<br />"; //log the user into session $_SESSION['username'] = $name; //or show are logged in $_SESSION['logged_in'] = true; } else { //was unable to insert records //die("Error: " . mysqli_error($con)); $error .= "There was a problem inserting your data. <br />"; } } else { //if rowcount equals 1 or more $error .= "That user or email already exists <br />"; } } else { $error .= "Unable to check if user exists. <br />"; } } } //show if an error if ($error != '') { echo $error; } //if there is a mysql connection then close it if ($con) { mysqli_close($con); } ?> For checking usernames and passwords upon login session_start(); if(crypt($passsword_from_db, $password_from_login) == $password_from_login) { $_SESSION['username'] = $name; $_SESSION['logged_in'] = true; $location = "http://".$_SERVER['HTTP_HOST']."/index.php"; header("Location: $location"); exit; } else { echo "Wrong username or password"; } Checking session logged in on pages and show username session_start(); if($_SESSION['logged_in']){ //do something } if($_SESSION['username']){ echo $_SESSION['username']; }
-
Parse Syntax Error unexpected 'name' (T_STRING)
QuickOldCar replied to bennetta89's topic in PHP Coding Help
I wrote you up using mysqli_* functions instead of the deprecated mysql_* With some additional error checking,messages and escaping the input before the query. <?php //set your credentials for mysql $hostname = 'localhost'; $username = 'root'; $password = 'password'; $database = 'database_name'; //mysqli connection $con = mysqli_connect($hostname, $username, $password, $database) or die("Error " . mysqli_error($con)); $error = ''; //check if form was submitted if (isset($_POST['submit'])) { //checks on each form value if (isset($_POST['name']) && trim($_POST['name']) != '') { $name = trim($_POST['name']); } else { $error .= "Name not set <br />"; } if (isset($_POST['address']) && trim($_POST['address']) != '') { $address = trim($_POST['address']); } else { $error .= "Address not set <br />"; } if (isset($_POST['city']) && trim($_POST['city']) != '') { $city = trim($_POST['city']); } else { $error .= "City not set <br />"; } if (isset($_POST['state']) && trim($_POST['state']) != '') { $state = trim($_POST['state']); } else { $error .= "State not set <br />"; } if (isset($_POST['zip']) && trim($_POST['zip']) != '') { $zip = trim($_POST['zip']); } else { $error .= "Zip not set <br />"; } if (isset($_POST['phone']) && trim($_POST['phone']) != '') { $phone = trim($_POST['phone']); } else { $error .= "Phone not set <br />"; } //check to see if all variables are set to determine doing a query if ($name && $address && $city && $state && $zip && $phone) { //escape any input $e_name = mysqli_real_escape_string($name); $e_address = mysqli_real_escape_string($address); $e_city = mysqli_real_escape_string($city); $e_state = mysqli_real_escape_string($state); $e_zip = mysqli_real_escape_string($zip); $e_phone = mysqli_real_escape_string($phone); //insert query $query = "INSERT INTO contacts (contact_name, contact_address, contact_city, contact_state, contact_zip_code, contact_phones) VALUES ('{$e_name}', '{$e_address}', '{$e_city}', '{$e_state}', '{$e_zip}', '{$e_phone}')"; $insert = mysqli_query($con, $query); //check if data was inserted if ($insert) { echo "Success! Your information was added.<br />"; } else { die("Error: " . mysqli_error($con)); } } } //show if an error if($error != ''){ echo $error; } //if there is a mysql connection then close it if ($con) { mysqli_close($con); } ?> Untested, but looks like should work. -
Parse Syntax Error unexpected 'name' (T_STRING)
QuickOldCar replied to bennetta89's topic in PHP Coding Help
Do things like this if(isset($_POST['name']) && trim($_POST['name']) != '') { $name = trim($_POST['name']); } if(isset($_POST['address']) && trim($_POST['address']) != '') { $address = trim($_POST['address']); } Then down below since made a new variable use that if($name && $address){ //run the query } Can also do different ways. if(isset($name) && isset($address)){ //run the query } -
Using Disqus for Ones Own Website with PHP?
QuickOldCar replied to glassfish's topic in Miscellaneous
I never personally used it, make my own comment systems. It probably won't work for outside people on localhost unless you are actually using the local server with a fully qualified domain name or someone knew the ip...port 80 open. Lets say it will work proper on a "live website" -
It's a bad idea, one of the 3 mentioned above. That would not make it more secure and increase the chance of a collision. Is pdo even enabled on that server? ensure the extension is uncommented in the php.ini file extension=php_pdo_mysql.dll Enable error reporting as mac_gyver suggested. Top of your script. error_reporting(E_ALL | E_NOTICE); ini_set('display_errors', '1'); You can wrap the pdo in a try/catch block and see any errors try { $stmt = $pdo->prepare("SELECT * FROM confirm WHERE username=:username AND password=:password"); $stmt->bindValue(':username', $username, PDO::PARAM_STR); $stmt->bindValue(':password', $pass, PDO::PARAM_STR); $stmt->execute(); } catch (PDOException $e) { print "Error!: " . $e->getMessage() . "<br/>"; die(); }
-
Using Disqus for Ones Own Website with PHP?
QuickOldCar replied to glassfish's topic in Miscellaneous
It supports normal html and js too. They call it "universal code". -
Sadly not all browsers support all video types. This means must have ogg and mp4 versions in order to play them all popular browsers. http://html5hub.com/html5-video-on-mobile-a-primer/
-
Making configuration changes in Joomla
QuickOldCar replied to Season's topic in PHP Installation and Configuration
The most direct way is to login with ssh I use putty log in with user, then your password for most linux based servers use the following command sudo reboot -
As far as mysql is concerned a million records isn't too many as long as properly indexed. Maybe the hosting is just sucky. The only way to run a good wordpress site is on a dedicated server, anything else and it's just getting by. I've dealt with wordpress since the beginning, it was never really fast beyond a vanilla install. Their numerous queries to mysql are horrible. Do you have any caching? If not load wp super cache to render html pages P3 plugin performance profiler is a handy plugin Try some page loading tests at Pingdom to see any missing or slow files Any of those fancy image sliders loading many huge images? Try a lazy image loader. If you have a link to the site I would take a look.
- 1 reply
-
- 1
-
-
Welcome to phpfreaks Chazy
-
Are you talking using html5 video versus flash?
-
Down below you have a lot that look like <?=$variable?> change them all to <?php echo $variable;?>
-
Try using full <?php tags versus short <? tags, some servers are not configured for it. change these width: <?= $width?>px; height:<?= $height?>px; to width:<?php echo $width;?>px; height:<?php echo $height;?>px; css errors can cause the white page as well with no error messages If you need to see the errors you can enable debug in wp-config.php define('WP_DEBUG', true); define('WP_DEBUG_LOG', true); define('WP_DEBUG_DISPLAY', false); @ini_set('display_errors',0); The log will be saved in wp-content as debug.log
-
how to compare the radio button value stored in database.
QuickOldCar replied to areeshkkj's topic in PHP Coding Help
You are going to want to store the users selections in a session or even a file if you wanted to. Then you can just look at all the session data instead of saving to a database. If you need to save results to a database as well, then you can compare session results to database results as well. If you are using all GET requests, I can see just looping through $_GET and saving them into a session session_start(); if(isset($_GET['testid']) && trim($_GET['testid']) !=''){ foreach($_GET as $key=>$value){ $_SESSION[$key] = trim($value); } } Now when you get your results from mysql, you can check them against the session values. as an example if($_SESSION['key'] == $row['key']){ $correct = true; } -
Bind the parameters and get rid of the stripslashes and mysql_* everything Let PDO escape it. bindValue bindParam $stmt->bindValue(':username', $username, PDO::PARAM_STR); $stmt->bindValue(':password', $pass, PDO::PARAM_STR); $stmt->execute(); More on encryption. Take a look at password_hash() , crypt or bcrypt
-
Besides it not working... md5 is not secure enough to use, also don't save plain text passwords into a session Edit: Don't save any password info, plain text or encrypted. Creating something like $_SESSION['logged_in'] == true or even $_SESSION['username'] is enough Consider adding user levels to know a user from an admin
-
Is just like basic html making the table, except in your while loop for mysql you echo out the <td></td> while ($row = mysqli_fetch_assoc($result)) { echo '<td>'.$row['cpu'].'</td>'; echo '<td>'.$row['ram'].'</td>'; }
-
For sure, parents hide those keys!!
-
I think we misunderstood one another. You want to make a form and store that post data to a database Do you have the form created? to post into a form and insert using PDO you can try this <form action="" method="post"> cpu: <input type="text" name="cpu"><br> ram: <input type="text" name="ram"><br> <input type="submit" value="Submit"> </form> <?php if (!empty($_POST)) { if (isset($_POST['cpu']) && trim($_POST['cpu']) != '') { $cpu = trim($_POST['cpu']); } if (isset($_POST['ram']) && trim($_POST['ram']) != '' && ctype_digit($_POST['ram'])) { $ram = trim($_POST['ram']); } if (isset($cpu) && isset($ram)) { //save the data $dbname = "my_database"; $dbuser = "username"; $dbpass = "password"; $tablename = "my_table"; try { $pdo = new PDO("mysql:host=localhost;dbname=$dbname", $dbuser, $dbpass); $sql = "INSERT INTO {$tablename} (cpu,ram) VALUES (:cpu,:ram)"; $q = $pdo->prepare($sql); $q->execute(array( ':cpu' => $cpu, ':ram' => $ram )); } catch (PDOException $e) { print "Error!: " . $e->getMessage() . "<br/>"; die(); } } } ?>
-
Websites frown on other websites scraping their data. Do you own this site or have permission to do so? Use curl or file_get_contents() to connect to the website Parse the html data whichever method suits you simplehtmldom dom simplexml preg_match() or preg_match_all() display or store the data you discovered
-
I wish we had a button for what am about to write next.... You should not be using deprecated mysql_* functions, instead use PDO or mysqli_* functions
-
Or check for $_REQUEST and use $_GET For one you should first see if $_GET["id"] is set, also not empty before you define a variable to it. $part = $_REQUEST['id']; if(isset($_GET["id"])){ if( isset($_GET['id']) && trim($_GET['id']) != '' && ctype_alnum($_GET['id']) ) { //is this supposed to be alphanumeric? make sure it is one $part = trim($_GET['id']); } else { //stop from continuing the script die('stop right there is nothing more to do!!!'); }