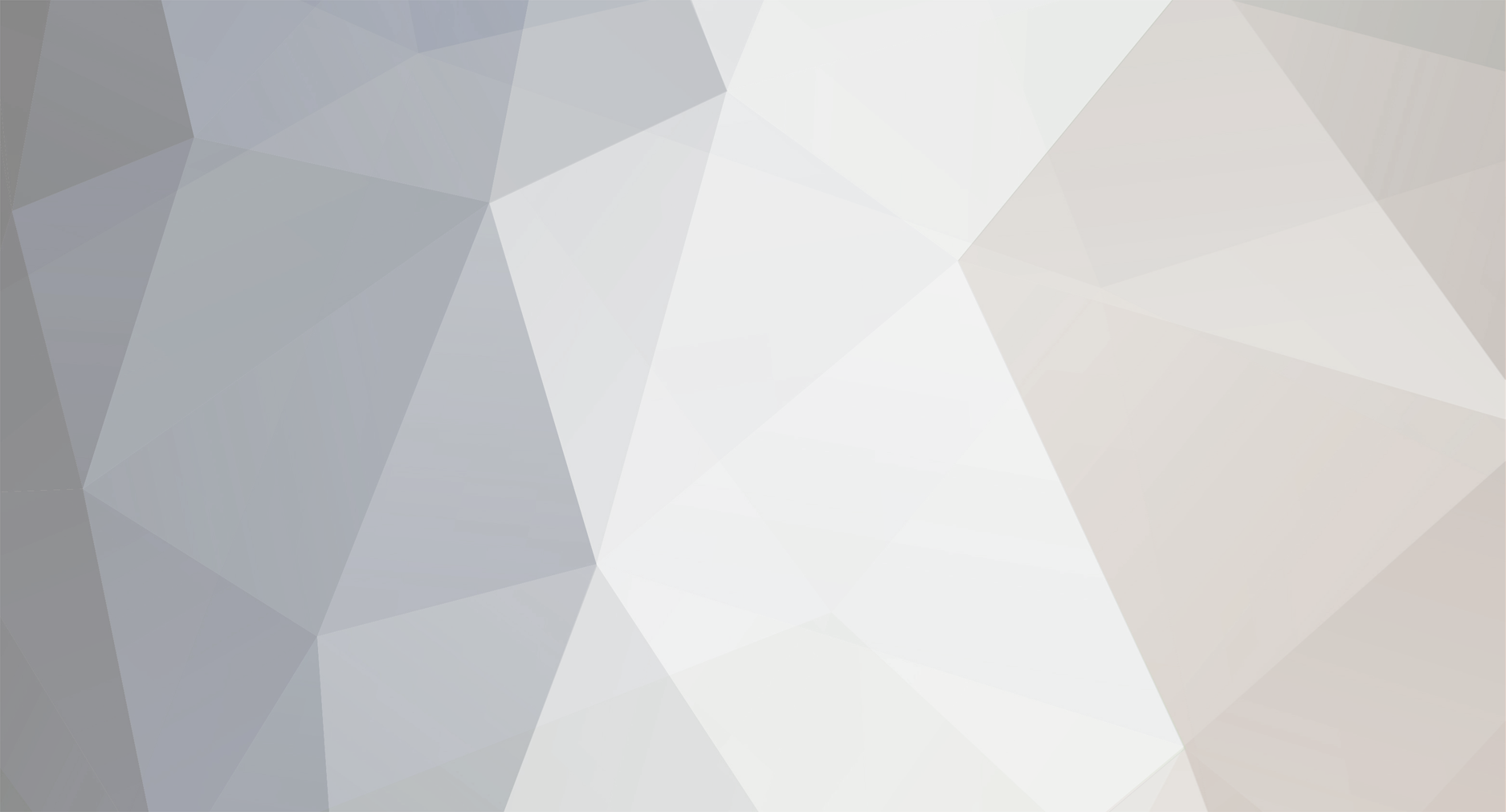
zq29
-
Posts
2,752 -
Joined
-
Last visited
-
Days Won
1
Posts posted by zq29
-
-
You could ask your client to ask their host to configure their server properly... Alternatively, you could use the IMAP functions.
-
I'm not sure if it is possible when creating the array, unless you manually define them to have the same value, but then affecting one won't affect the other.
You could do it with a reference though:
<?php $foo = array(1 => 'bar'); $foo[0] =& $foo[1]; echo "<pre>",print_r($foo),"</pre>"; ?>
EDIT: Beaten to it.
-
Do you get what you expect when running the following on your live server?
echo $_SERVER['REQUEST_URI'];
-
It's not working because of the double quotes you have sprinkled around in there, either echo it with single quotes:
echo '<v6.52><e0> @Normal=<Ps100t-2h100z9.4k0b0cMf"Helvetica"> @.LIST Bold=<Ps100t-4h110.001z8.7k0b0cKf"InterstateNL-BlackCondensed"> @.BODY=[s"",".BODY"]<*J*h"Standard"*kn0*kt0*ra0*rb0*d0*p(0,7,0,10,0,0,G,"U.S. English")Ps100t-2h100z9.4k0b0cKf"PoynterOSTextTwoNL-Roman"> @Normal=[s".BODY",".BODY","Normal"]<> @.GLANCE Hed 100K=[s"",""]<*L*h"Headline"*kn0*kt0*ra0*rb0*d0*p(0,0,0,+0,10,0,g,"U.S. English")Ps100t-4h100z16k0b0cKf"InterstateNL-BlackCondensed"> @.GLANCE Text normal=[s"",".GLANCE Text normal"]<*L*h"Standard"*kn0*kt0*ra0*rb0*d0*p(0,5.25,0,10,0,0,G,"U.S. English")Ps100t-2h110.001z9.4k0b0cKf"InterstateNL-LightCondensed"> @.LIST Subtopic label=[s"",""]<*C*h"Standard"*kn0*kt0*ra0*rb0*d0*p(0,0,0,10,4,2,g,"U.S. English")PKs100t-3h100z8.7k0b0cKf"InterstateNL-BlackCondensed"> @.LIST Body no indents=[s"",".LIST Body no indents"]<*L*h"Standard"*kn0*kt0*ra0*rb0*d0*p(0,0,0,+0,0,3,g,"U.S. English")Ps100t-4h110.001z8.7k0b0cKf"InterstateNL-LightCondensed">' ;
Or use the HEREDOC syntax:
echo <<<FOO <v6.52><e0> @Normal=<Ps100t-2h100z9.4k0b0cMf"Helvetica"> @.LIST Bold=<Ps100t-4h110.001z8.7k0b0cKf"InterstateNL-BlackCondensed"> @.BODY=[s"",".BODY"]<*J*h"Standard"*kn0*kt0*ra0*rb0*d0*p(0,7,0,10,0,0,G,"U.S. English")Ps100t-2h100z9.4k0b0cKf"PoynterOSTextTwoNL-Roman"> @Normal=[s".BODY",".BODY","Normal"]<> @.GLANCE Hed 100K=[s"",""]<*L*h"Headline"*kn0*kt0*ra0*rb0*d0*p(0,0,0,+0,10,0,g,"U.S. English")Ps100t-4h100z16k0b0cKf"InterstateNL-BlackCondensed"> @.GLANCE Text normal=[s"",".GLANCE Text normal"]<*L*h"Standard"*kn0*kt0*ra0*rb0*d0*p(0,5.25,0,10,0,0,G,"U.S. English")Ps100t-2h110.001z9.4k0b0cKf"InterstateNL-LightCondensed"> @.LIST Subtopic label=[s"",""]<*C*h"Standard"*kn0*kt0*ra0*rb0*d0*p(0,0,0,10,4,2,g,"U.S. English")PKs100t-3h100z8.7k0b0cKf"InterstateNL-BlackCondensed"> @.LIST Body no indents=[s"",".LIST Body no indents"]<*L*h"Standard"*kn0*kt0*ra0*rb0*d0*p(0,0,0,+0,0,3,g,"U.S. English")Ps100t-4h110.001z8.7k0b0cKf"InterstateNL-LightCondensed"> FOO;
-
We have an IRC channel on irc.freenode.net - ##phpfreaks - Should you wish to have a more "chat-like" environment.
-
Thats a bit long winded SemiApocalyptic. To remove anything bar a number you could simply use
Good point.
-
Alternatively, if the input is not always the same, you could do something like this:
<?php $str = "R$100.000,00"; function numbers_only($in) { $out = ""; for($i=0; $i<strlen($in); $i++) if(is_numeric($in{$i})) $out .= $in{$i}; return $out; } echo numbers_only($str); ?>
Off the top of my head, I can't think of a built-in function that can do this...
-
Regular Expression?
echo (preg_match("/^([A-Z]+)$/i",$str)) ? "Alpha" : "Not Alpha";
-
thanks, i couldent find where to look in teh manual
How about the link that GingerRobot posted?
-
apart from on experts exchange, and I refuse to pay to use that service because it was a load of crap when I used to use it!!!
You know, you can scroll down to the bottom of the page on Experts Exchange to see all of the posts you "have to pay for".
-
-
echo $predate[1];
-
You could name each button differently and use PHP to check which one was pressed:
<?php if(isset($_POST['button_1'])) { //do something } elseif(isset($_POST['button_2'])) { //do something else } elseif(isset($_POST['button_3'])) { //do another thing } ?> <form name="foo" action="" method="post"> <input type="submit" name="button_1" value="Button 1" /> <input type="submit" name="button_2" value="Button 2" /> <input type="submit" name="button_3" value="Button 3" /> </form>
-
People sit at computers and write them? Or do you mean, how do they work?
In a nutshell, they visit a page and save all of the content into a database, looking for all links on the current page, visit them, store them, look for more links, and so on. This is called spidering and indexing. A user typing a search term queries this database and is given results.
Obviously, there is a lot more complexity under the hood. This is a basic overview.
-
Like I said I was running Ubuntu in VmWare Fusion, the only problem is that Vm ware is such a resource hog.
Dual booting is not the same as virtualization. When dual booting, you're not loading one OS within the other, you're loading it instead of the other. It's running native, not wasting resources on emulation or virtualization.
But, in response to your question about what would be a good native (to OS X) compiler, I don't know.
-
Dual boot GNU/Linux along side OS X?
-
I wouldn't know what to do with one of these things about 5 minutes after sitting at it. When I built my i7 box, the first thing I did was conduct a race between it, and my previous machine, to see how quickly it could merge a couple of Gigs of avi files. After being suitably impressed, I just got on with my coding...
-
You're not blind, it's gone. It didn't get enough traffic to justify its existence.
-
How many super computers do you know that are still up and working 28 years after they come online? it's already obsolete in terms of technology...
I wonder what they do with them whey they're "obsolete"... Donate them to a university, government, chuck it on eBay?
-
Something like this?
<?php $image = imagecreatefrompng("/path/to/file.png"); $width = imagesx($image); $height = imagesy($image); for($y=0; $y<$height; $y++) { for($x=0; $x<$width; $x++) { $rgb = imagecolorat($image,$x,$y); echo "Pixel $x , $y colour: $rgb\n"; } } ?>
-
Processor type: 32-bit PowerPC 450 at 850 MHz.
Isn't PowerPC like...An ancient Macintosh processor?
The PowerPC is an IBM processor, though, it was previously used by Apple. I guess they're using an "old" chip for a number of reasons - Easier to cool, cheaper to build, consumes less power, and it was probably a new-ish chip in the design stages.
-
2) If you have a generic coding question, ask it in an actual coding help board, not the board where we talk about general garbage and non-coding topics.
I moved it here - It had no PHP specific questions and was a bit generic to fit any other board.
-
-
As much as I don't think that is the case, I'm not a fan of Java either. Platform independent code is great, but at the cost of performance, I'd rather find an alternative.
JSON
in Javascript Help
Posted
JavaScript Object Notation
http://lmgtfy.com/?q=JSON