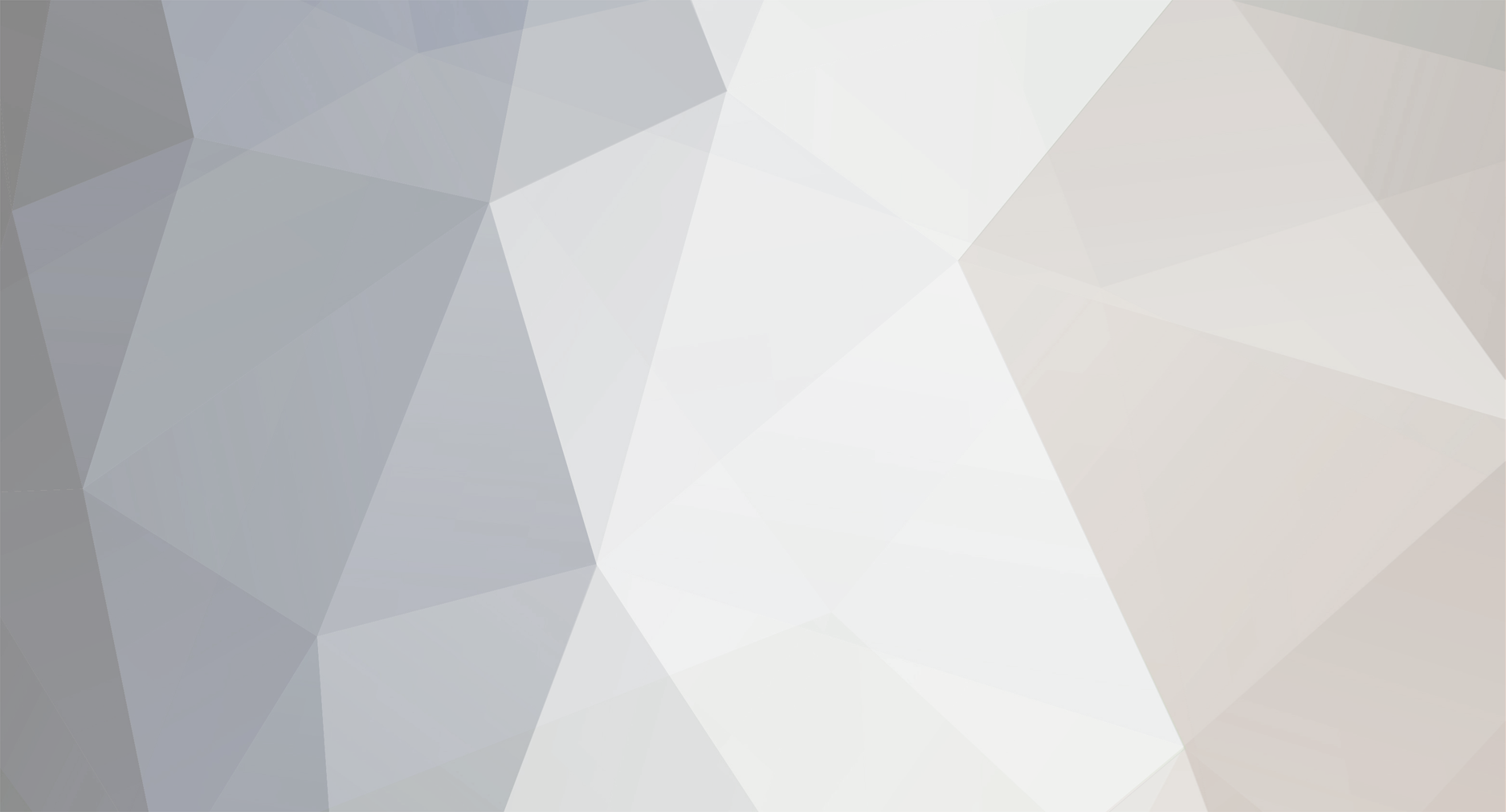
denno020
Members-
Posts
761 -
Joined
-
Last visited
-
Days Won
3
Everything posted by denno020
-
The only reason you would need the magic getter or setter functions is if you're trying to access private variables from another class. Also, seeing as though class User extends class Auth, then instead of creating a new Auth, just create a new User. You will have access to the variables username, password etc because they are inherited to the User class from the Auth class. Hopefully that makes sense.. Denno
-
Copy your code here and I'll see if I can figure out what's breaking it.
-
writing error log but I'm not sure how to organize it
denno020 replied to et4891's topic in PHP Coding Help
At the end of each strings that you fwrite to the error log, add '\n' (no quotes). This is a new line character. Hopefully this will translate over to the browser as well, but if not, then you'll need to use '<br>' instead. And as for printing the link to the error log, I wasn't aware that this was something extra that had to be done, but you could do it after the foreach loop, and use the $datePrinted variable again. So if $datePrinted == true, then that means there must be been an error, as the date had to be printed, so echo the link to the error file. Again, this if statement will be outside of the foreach loop (after it to be exact). Hope that helps? Denno -
writing error log but I'm not sure how to organize it
denno020 replied to et4891's topic in PHP Coding Help
I don't understand this? You'll want an error printed for each line there is an error wouldn't you? Anyway, this code should do it for you: $datePrinted = false; $error_log = fopen("./courses/path/error.log","a+") or die ("File can not be opened"); foreach($fileContents as $row){ $column = preg_split("/,/", $row); if(!(isEmailAddressWellFormed($column[3])) || !(isStudentNumberWellFormed($column[0]))) { echo "error"; if(!$datePrinted){ fwrite($error_log, date("F t, Y (h:i:s a)") . PHP_EOL); $datePrinted = true; } if(!(isEmailAddressWellFormed($column[3]))){ fwrite($error_log, "Improper email address from " . $_GET["filename"] . " :" . PHP_EOL); fwrite($error_log, "$column[2] $column[1] $column[3]" . PHP_EOL); } if(!(isStudentNumberWellFormed($column[0]))) { fwrite($error_log, "Improper student numbers from " . $_GET["filename"] . " :" . PHP_EOL); fwrite($error_log, "$column[2] $column[1] $column[0]" . PHP_EOL); } } }//closing foreach fwrite($error_log, "=============================" . PHP_EOL); fclose($error_log); Give that a try -
writing error log but I'm not sure how to organize it
denno020 replied to et4891's topic in PHP Coding Help
Sorry put it as the first thing inside your first if error check.. outside of the "if date not set, set it" if thing -
writing error log but I'm not sure how to organize it
denno020 replied to et4891's topic in PHP Coding Help
Put the fopen as the first thing inside your foreach. The error is appearing because the second time that the foreach loops, the file has been closed (at the end of the first loop), but won't be opened again because $datePrinted is set to true, so it won't go into that if block that contains fopen. Fingers crossed that will fix all problems Denno -
writing error log but I'm not sure how to organize it
denno020 replied to et4891's topic in PHP Coding Help
Directly before the foreach, put $datePrinted = false; Then inside the first if, with the two conditions, have the "if date not printed, print it" if statement that I wrote previously. -
writing error log but I'm not sure how to organize it
denno020 replied to et4891's topic in PHP Coding Help
a simple way could be to just use a flag.. So outside of the foreach, set $datePrinted = false, Then inside the foreach, and inside an error detecting if, you can do this: if(!$datePrinted){ //print the date to file etc $datePrinted = true; } That way the date will only be printed once, no matter how many times the foreach loop runs . Denno -
window.setInterval(yourfunction, 10000); I just did a quick search and found that. Looks like it'll be able to do what you want.
-
writing error log but I'm not sure how to organize it
denno020 replied to et4891's topic in PHP Coding Help
What error are you getting then? Have you used some echo's or var_dumps to check that you're actually entering the if code blocks? -
writing error log but I'm not sure how to organize it
denno020 replied to et4891's topic in PHP Coding Help
Your first if statement would need two pipes || for the or, you've got just the one.. That could be a problem -
writing error log but I'm not sure how to organize it
denno020 replied to et4891's topic in PHP Coding Help
On way you could do it is to nest your if statements inside a bigger if statement. This bigger one checks all the conditions, and if any of them are true, then we move into the if code block, print the date to the error log, then use the if statements you already have to print whatever error(s) there are., This would mean the date would only be put in there once. Does that make sense? Denno -
Selecting Checkboxes based off MySQL Field that is an Array
denno020 replied to jay7981's topic in PHP Coding Help
Oh so the implode happens before the data goes into the database? That makes sense then, I thought this was the beginnings of your script to extract the data from the database. Anyway, another thing you could do then which could be simpler is to use strpos as such: $actionItems = //query to get the results from the database - this will be a string, comma separated, as you've already said $open = (strpos($actionItems, "Open") !== false) ? "checked" : ""; //this is a one line if statement, if the string "Open" is in $actionItems, this will be it's to be checked, so set $open to checked //do the same for the other two actions //Print out the checkboxes echo "<td>Action</td>"; echo "<td>"; echo "<input type='checkbox' name='action[]' value='open' $open/>"; //This will place either 'checked' or nothing to the end of the input tag echo "Open"; //again, do the above for the other two options. echo "</td>"; I'm pretty sure that will get you want you want.. Denno -
Selecting Checkboxes based off MySQL Field that is an Array
denno020 replied to jay7981's topic in PHP Coding Help
I assume that all the data is already in the same field? So the field could contain "Open, Close", or "Open", or all three "Open, Close, Break"? I'm not sure why you're using implode(), as that will join things together, and I don't think $action is an array. Anyway, what you could do is explode $action into another variable, using comma as the delimiter. Then do a for each that loops through the exploded array. Something like this: $action = $res['action']; $actionArr = explode(",", $action); echo "<td>Action</td>"; ecoh "<td>"; foreach($actionArr as $actionItem){ $actionItem = trim($actionItem); //Remove any leading/trailing white space echo "<input type="checkbox" name ="action[]" value="$actionItem" checked />"; echo $actionItem; } echo "</td>"; That will print the checked items as you want, however it won't print the others that aren't checked, but it's not hard to figure out how to add that in. Hope that helps Denno -
Do you have to use fwrite? Another way you could do it is by reading the file contents into an array using file(). Then use preg_grep to match the line return tooltips (this will return an array with the data as a value, and the key is the index in the array that the data is - so basically the line number in the file). Then you grab the array key, using a simple foreach loop $file = file("test_file.text"); $fileArray = preg_grep("/^return tooltips$/", $file); $line = 0; //This will store the line number of 'return tooltips' foreach($fileArray as $index => $data){ //This will most likely only run once, but that's fine, we're only grabbing the array key $line = $index-1; //Store the index number just before the the line you searched for } Then you use array_splice to put data into the array at position $line. You may need to use a loop and increase $line each time you add to the array, so it adds it _after_ each previous line that you add, otherwise it will print backwards (last line will appear first, first line will appear last) Then you can use file_put_contents("new_file.txt") to put the array back into a file. Hopefully that's understandable. Denno
-
<?php while($row = mysql_fetch_array($result)){ //Opening curly brace?> <td><?php echo $row['id']; ?></td> <td><?php echo $row['lastname']; ?></td> <td><?php echo $row['cellphone']; ?></td> <td><?php echo $row['email']; ?></td> <?php } //closing curly brace?> Everything is the curly braces will be run for every row in your mysql result set. Personally, I would write it like this too, especially seeing as though you're already using echo: <?php while($row = mysql_fetch_array($result)){ //Opening curly brace echo "<td>".$row['id']."</td>"; echo "<td>".$row['lastname']."</td>"; echo "<td>".$row['cellphone']."</td>"; echo "<td>".$row['email']."</td>"; } //closing curly brace ?>
- 11 replies
-
- connecting to server
- php
-
(and 1 more)
Tagged with:
-
using SESSION and after header redirect SESSION is gone
denno020 replied to loren646's topic in PHP Coding Help
session_start() must be the very first line in any php script that you want to use to access the session variables. -
Just a quick note, you've got your default case, that will run if nothing else matches, so you could have put your "That page doesn't exist" page there..
-
Where is the while loop supposed to finish? Looking at your code, it will only run on the first line.. and not loop over any code block.. You should use curley braces to wrap the code block that you actually want to loop over. Denno
- 11 replies
-
- connecting to server
- php
-
(and 1 more)
Tagged with:
-
if($myfield != ""){ //there is content, so do something with it }else{ //There is no content }