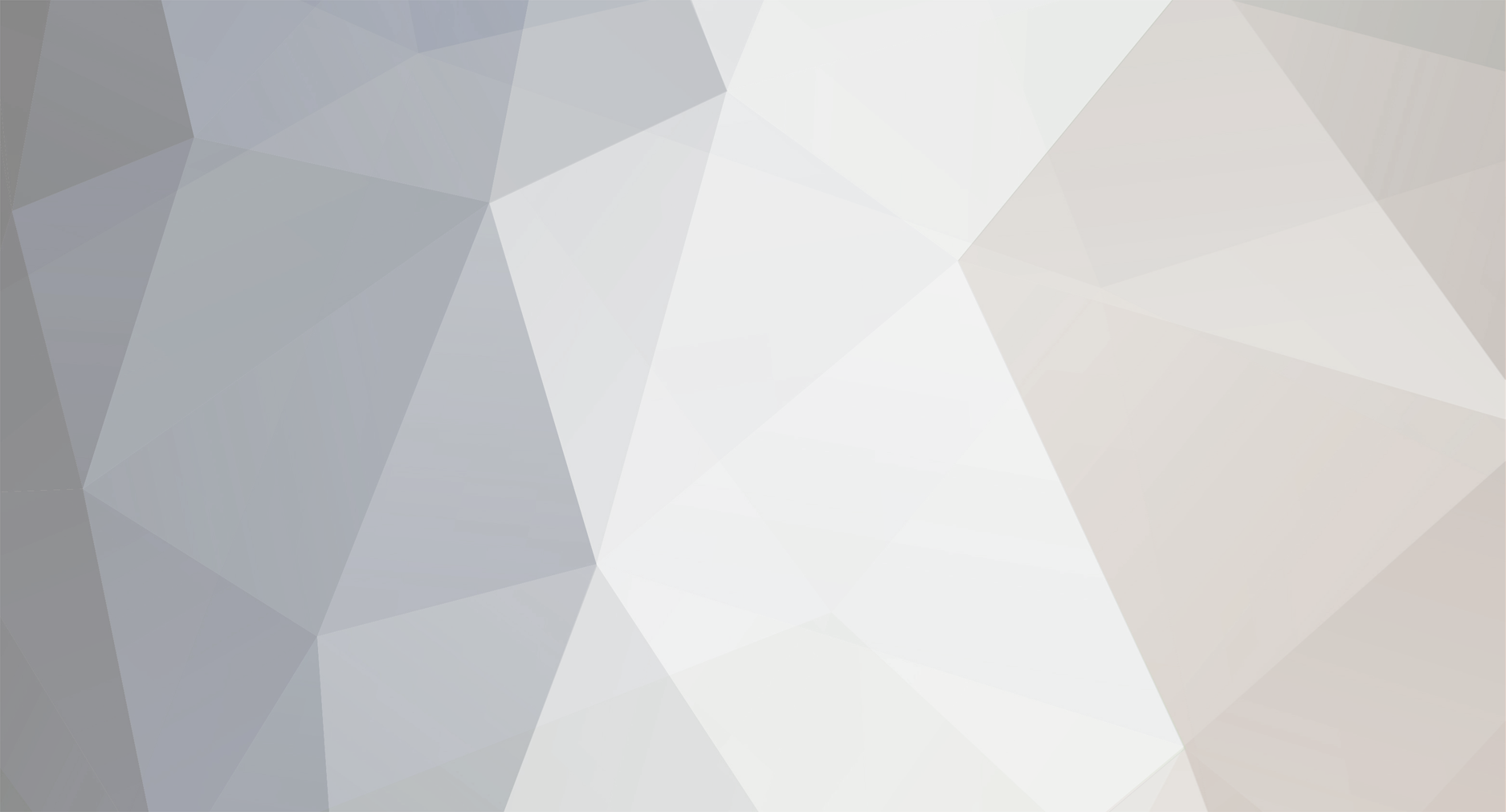
denno020
Members-
Posts
761 -
Joined
-
Last visited
-
Days Won
3
Everything posted by denno020
-
Edit: Appears boompa beat me to the submit button . Well for some proper help you'll need to share your error as well, otherwise we've got nothing to go on.. But I will have a guess that you're getting an error saying that $_GET['action'] isn't set? You need to do it like this: if(isset($_GET['action']) && $_GET['action'] == 'add'){ .... } As for the other part, I'm not sure from looking at it what the error might be, let us know and I'm sure we'll be able to help more. Denno
-
It's not difficult at all. All you need to do is have a field in your products table which is the ID of the retailer in the users table (which I think from your comments is called user_ids). That way the two tables are linked through the retailers ID, and you can get all of the retailers information and all of their products in one hit.
-
I do believe that $_SESSION actually saves to the users browser session, so they will only ever have their own user data. I could be wrong, but that's what I've always assumed.. Edit: Just saw your reply. It's not stupid to ask a question if you're unsure. How else will you ever be certain about the answer?
-
Notice: Undefined index: submit in C:\wamp\www\matri\login.php
denno020 replied to anshu's topic in PHP Coding Help
trq is right.. You'll need to bring all of your code here, and highlight which line 74 is.. In the process of doing that though, you'll see where the error is and probably be able to figure out the solution yourself. Denno- 4 replies
-
- undefined index: submit
- undefined index: email
- (and 1 more)
-
Notice: Undefined index: submit in C:\wamp\www\matri\login.php
denno020 replied to anshu's topic in PHP Coding Help
You're doing the isset() test too late. You should assign the variables like this: if(isset($_POST['submit'])){ $form = $_POST['submit']; } //And the same with email and password. (or on one line) $form = isset($_POST['submit']) ? $_POST['submit'] : NULL; That will clear up your undefined index errors. And the reason you're getting the errors is most likely because the form that is submitting to this script either isn't passing the variables through with the same name, or the fields haven't been filled out in the form. Hopefully that'll help you out. Denno- 4 replies
-
- undefined index: submit
- undefined index: email
- (and 1 more)
-
How to print all images stored in a file Directory?
denno020 replied to Jenksuy's topic in PHP Coding Help
glob will return the filenames/paths to all files that match the expression in the directory that you give it as an array. So you will need a foreach loop to loop through each of the files and do with them what you would like: $files = glob(path/to/images/*); foreach($files as $file){ echo $file; //this will echo the complete filename/path } Start playing around with that and post back if you need more help. Luke -
I think it would be more on the database side that you would need to set this up.. Make sure that they field is allowed to have NULL values, and then make NULL the default, so if no value is entered, it will be NULL.. So you do all that in your database manager (which I assume might be phpmyadmin?). Denno
-
In answer to your question about what the SQL query does, it will query the database (ask the database) for any records that have the username that matches what the user put into the form, and a password that also matches. Ideally this would only be one record, as all usernames in the database should be unique. The second line then counts the number of rows (database records) that were returned, if this number is 0, then there is no user with that username, or the password was wrong. If there is 1 record returned, then the user provided login details are correct. Denno
-
I would suggest you set it out like this: <?php $loginError = false; if(isset($_POST['username'])){ //Check if a form has been submitted //Do all your login processing here. //... //... //... //... if($login_check > 0){ //Do all of the processing that you have header('Location: index.php'); exit(); }else{ $loginError = true; //Flag to turn on error messaging below } } ?> <!DOCTYPE html> <html> <head> </head> <body> <?php if($loginError){ echo "There is an error processing your login details. Please try again"; } ?> <form> <!-- form contents --> </form> </body> That will allow you to print out error messages wherever you want them, but placing the if($loginError) section of code wherever you want it to appear, so you could move it inside the form tags, and just before the inputs. Hopefully that will get the ball rolling for you. Denno
-
Redirect users to a certin directory using php with htaccess
denno020 replied to arcangel's topic in PHP Coding Help
If I were to do something like this, I wouldn't be using htaccess at all. Just use PHP to re-direct the user to their directory: //Get the users username by whatever means. This is a simple example of just grabbing it straight from the form POST. $username = $_POST['username']; header('Location: http://blah.com/client/'.$username); That should work fine. -
What you're saying is correct, you're getting a redirect because 'section' isn't set, which is therefore satisfying the first condition of the second if statement. The only way to be able to echo out content related to username even if section isn't set or what it should be is to remove the checking of section..
-
You need to include secured.php into your login.php script, otherwise you never grab the username/password from the form. Also, your form uses POST as the method, but you're trying to get the variables using GET. Fix those two problems up and you should start getting some meaningful errors (if there are any), that you can start to debug. Denno
-
No worries at all, we all started somewhere! And also, I've just noticed your signature, very clever! However I'm going to nit-pick on the use of "your".. echo "I will try to help, because your you're still $skill_level , and my skill level is at $my_knowledge"; Sorry to be a jerk! Denno
-
I would say that's because it's a HTML comment and not a CSS comment .. Even though your CSS is internal, when your brower sees the <style> tag, it turns into CSS mode, reading everything up until the </style> tag as CSS.
-
In css, comments start with '/*' and end with '*/'. Try removing your comment with <!-- --> and see what happens . Denno
-
To be honest.. I don't really understand what you're asking?
-
So you want the tooltip to be positioned by the location of the mouse? That will be a bit more involved.. I'm not sure you can do it with just CSS, you'll have to use javascript to constantly update the position of the tooltip div every time the mouse moves, and it's hovered over the button..
-
Well I can imediately see a problem. You're targeting ".tooltip span". That refers to a span inside .tooltip, which is never going to happen, as it's applied to an img. You will need to put a div around both the elements, and apply .tooltip to that div: http://jsfiddle.net/S5J8h/1/ Works straight away. Denno
-
Post your code so we can see what you're attempting this with.
-
After I replied I actually noticed that if you scroll down on that page, they show you some html with exactly how they make it, so you should be able to follow that. The main css property's you're going to use are 'display' or 'visibility'. Do some googling to find out how they work. Essentially you will create a css class, called hidden (or whatever you want), and apply that to the div by default. Then, when the user hovers over the button, use JavaScript to remove that class. As soon as they mouse off the button, your JavaScript should detect that, and apply your css class back to the div. Alternatively, you could do it using just JavaScript. Give your div an id of tooltip. In your JavaScript, hide that div on page load, and then show it again only on muse over. Jquery has some very simple functions already set up if you want to use those, otherwise it's not too hard to do with raw JavaScript. I'm replying on my phone at the moment so apologies for no code examples. If you still can't figure it out, let me know and I'll give you some specific code that will help. Denno
-
This is something I started to put together until I realized that someone else would have surely done it: http://jsfiddle.net/T6PcS/ So the jQuery credit goes to http://stackoverflow.com/questions/5422001/jquery-infinite-loop-for-function user Felix King. Tweak that until you have what you want. Denno
-
Whilst that's called a tooltip, it's probably built using divs and some js to hide/show the div on mouse hover on the button.. Would be fairly simple. Just create a div and style it up how you want it to look (including any images you might want in there). Position it on the page where you want it, and then set it to hidden or display none. Then use some javascript to show that div when someone hovers over the button/link/whatever you want the tooltip to be attached to. Hopefully that'll get you started. Denno
-
you've got $height = $imagesy($img); You need to get rid of the dollar sign before imagesy: $height = imagesy($img); You've done it right on the next line for $width As for the warning, just make sure you have actually got a result by using var_dump($result).
-
Why was I not notified that there was a response here :/.. Anyways, sorry for the delayed reply, but here you go: http://jsfiddle.net/v3DYw/1/ Exceptionally simple, just had to add the a after '#name ul li:last-child', as DavidAM mentioned earlier. Denno