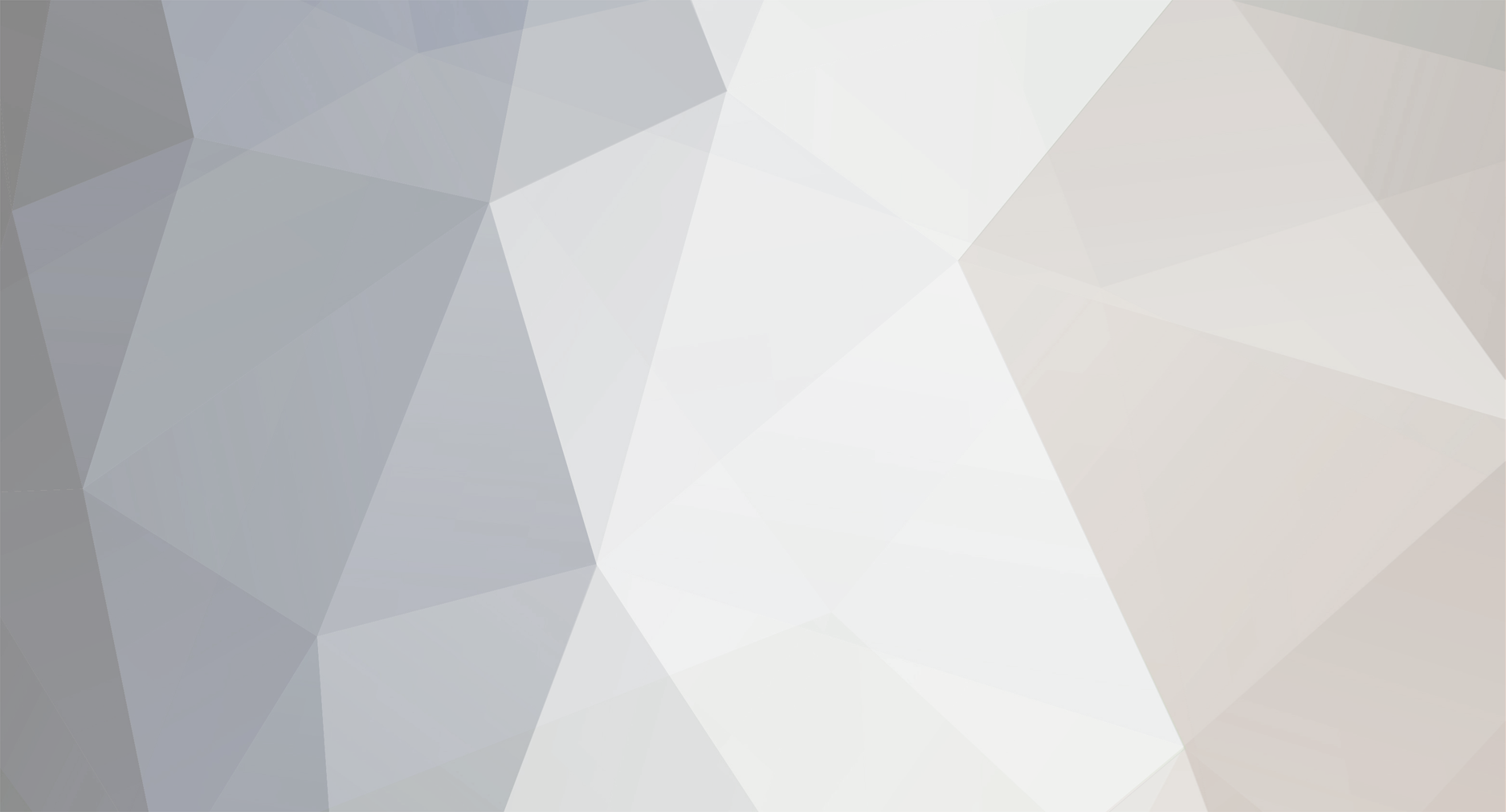
denno020
Members-
Posts
761 -
Joined
-
Last visited
-
Days Won
3
Everything posted by denno020
-
Replace function value depending on what's after function
denno020 replied to crashwave's topic in PHP Coding Help
You could use a combination of is_dir() and file_exists() to determine if the $fork_folder/$fork_file exists. -
If you want to do it using an array, and provided your mysql results array looks like this $results = array( array( "blog_id" => "2", "content" => "Blog2Text", "comment_id" => "4", "comment_text" => "comment4text", ), array( "blog_id" => "2", "content" => "Blog2Text", "comment_id" => "7", "comment_text" => "comment7text", ), //etc. ) Then you can create your array like this: $outputArr = array(); foreach ($results as $result) { //Initialise array for the blog ID of the current $result, if it's not already done so if (!isset($outputArr[$result['blog_id']])) { $outputArr[$result['blog_id']] = array(); } //Append the comment_text for this result to the array for this blog_id $outputArr[$result['blog_id']][] = $result['comment_text']; } $output = json_encode($outputArr); Which will result in an output array looking like this: $outputArr = array( 2 => array( "comment4text", "comment7text", ) ); Hopefully that helps you out. Denno
-
Shouldn't your while be $date < $closing_time
-
Have you called your refresh function?
- 2 replies
-
- jquery
- javascript
-
(and 1 more)
Tagged with:
-
How are you using str_replace? str_replace(' & ', ' ', $variable); Should do the job fine.. Grabbing the space from either side of the & symbol and replacing with 1 space will fix a problem if you simply find the & symbol and replace it with nothing.
-
The first thing I notice is that the if statement doesn't seem to have it's closing curly brace? Apart from fixing that, there are 3 things I would check: 1. var_dump() as the very first thing in the constructor, this will tell you if the object is being instantiated or not (speaking of which, can you show us the code where you create a new validation class) 2. var_dump() is_store_closed() outside of the if statement, to make sure that's returning true. 3. var_dump() the $_SESSION variable, even though doing a var_dump of $this->store_name should display null. I think you problem is either you haven't created a new class properly (you can't just include the script, you actually have to call $validation = new validation() ), or you is_store_closed() function is returning false. Hope that helps. Denno
-
Need help adding TD dymanic attributes in HTML table
denno020 replied to eldan88's topic in PHP Coding Help
Seeing as though $attr is being treated as an array (it's the variable used in the foreach), then you should initialise it to be an empty array, not an empty string. Also, you should indicate that the parameter has to be an array by adding 'array' before it: public function table_rows_and_columns(array $attr=array(),$data_name="",$data="") { Next, if $attr isn't always required, then you need to make it the last parameter in the function signature. These two calls: echo $table->table_rows_and_columns("Name:","<input type='text' name='name' id='name'>"); //First call echo $table->table_rows_and_columns("Phone:","<input type='number' name='phone' id='phone'>"); //Second call effectively do this: $attr = "Name:"; $data_name = "<input type='text' name='name' id='name'>"; $data = "" //First $attr = "Phone:"; $data_name = "<input type='number' name='phone' id='phone'>"; $data = "" //Second So as you can see, you're getting the error because the second two calls to your function don't pass an array as the first parameter. Have a crack at fixing the problem and let us know how you go. Then we can help you further if you need it. Denno Edit: oh and your line $row = "<tr><td{$key.'='.$value}>{$data_name} </td> <td>{$data}</td></tr>"; isn't in the foreach, so it won't use the $key and $value variables.. -
Firstly, I like the message to the user if they're already in the database, but please, update "Your" to be "You're".. Anyway, to your problem. If you var_dump $query2, you'll see that it is actually false, which means you're doing count(false). which gives 1. Therefore, your if condition should be as such if ($query2 !== false) { } //OR if (!$query2) { } Hope that helps. Denno
-
Am I missing something simple with my htaccess?
denno020 replied to denno020's topic in Apache HTTP Server
So I figured out what the problem was, in case anyone was wondering. I had a temporary URL, as I'm moving the website to a different hosting company, and it turns out that when I was reading the http_host in my php file, it was only pulling the ip address in, it wasn't also pulling in the my username which corresponded to my folder on their server. Anyway, that probably doesn't explain it very well, but it's all fixed now, so that's the main thing. -
Is this something like what you were after, http://jsfiddle.net/2ggL6/1/
-
I've been using htaccess in my web projects for a little while now, both at home and at work. I'm currently working on a website for a friend, which is a wordpress one, and I'm trying to set up my htaccess in the root of my website which will handle old links, and forward people on the the new location (they previously had presta shop, which uses a different link structure). Anyway, I've got my public folder on my host, which has my htaccess, an index.php file, and a wordpress folder, which has all of the wordpress stuff. The plan was to capture links that weren't already going to a wordpress subdirectory, pass them to the index.php file, and then that will work out where the user should be redirected to. Sounds simple enough, but for whatever reason, it's just not working and I can't for the life of me figure out why. The following is my htaccess file: # Use PHP5.4 Single php.ini as default AddHandler application/x-httpd-php54s .php RewriteEngine On RewriteCond %{REQUEST_FILENAME} !-d RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-l RewriteRule ^((?!wordpress).)*$ index.php?url=$0 [QSA,L] So as you can see, it's very simple. The first few lines before RewriteEngine are specific for my host. Anyway, this works perfectly on my local server (wamp), however running it on my live server, I continually get "404:File Not Found". The index file is in the exact same location as the htaccess file, so there isn't any problem with that. I've also tried putting junk into my htaccess, to see if it's actually being used, and it is. If I put "some junk" (literally that string) into my htaccess just before the RewriteEngine on directive, I get this message: [an error occurred while processing this directive] So I know my htaccess is being read.. Can anyone see something that I'm missing? Thanks, Denno
-
You have opened a connection to the database haven't you? I'm not sure if you get exceptions thrown if you try and execute a query without first selecting the database..
-
That's what appears to have happened..
-
Are you sure you're actually getting results from your SQL query? Have you got phpmyadmin or something similar that you can use to test your query? Also, can you post your code inside [ code ] tags, makes it much easier to read
-
Then you'll need to add a var_dump($row);die(); just after $row is set, so you can have a look-see at what's actually in that array. Perhaps you're getting your index incorrect..
-
Try this then: echo "<input id='FirstName' type='text' maxlength='20' name='FirstName' value='".$row['FirstName']."' pattern='^[a-zA-Z]{2,20}$' required /><br>"; Oh and don't change all of your input fields, just change one, until you get that one working, then apply the same changes to the rest. Will save you a tonne of time debugging Denno
-
The way you are trying to print the value from $row is like this: $row[FirstName] It needs to be like this: $row['FirstName'] Notice the quotes. Easy to miss that because you have a tonne of single and double quotes going on in your code. Give that a try and see if it helps. Denno
-
When you hit enter whilst entering text in an input field in a form, that form will submit.. The input field needs to be surround by a <form> for this to work. If you don't have <form> tags around your input, then you can use this code that I found from a Google search at stackoverflow: $(document).keypress(function(e) { if(e.which == 13) { alert('You pressed enter!'); } }); And put into practice here: http://jsfiddle.net/Gehtf/1/ Hope that helps Denno
-
What value is currently being echoed into your input fields?
-
How to show static image when there is no dynamic image
denno020 replied to parlanchina's topic in PHP Coding Help
Simply use an if statement to check if the thumbnailUrl array index is set in your $data variable. If it is, echo the value, otherwise, echo the path to your static image. Denno -
First step would be providing a link to your site so we can see the problem for ourselves. What error are you getting when it all fails? Have you included jQuery properly?
-
Can you show me an example outline of the code that you would like to end up with? In your PHPFiddle, the $content variable already has <p> tags.. I don't quite understand where you want them to be.. If you can show me the outline that you want to achieve, I'm sure I'll be able to help you with your loop. Denno
-
The & means it's passing a reference to that variable. Without it, the foreach would need to look like this: foreach($test_array as $index => $value) { if ($value['item'] == '2') { $test_array[$index]['qty'] = 5; break; } }
-
decreasing/increasing number depending on selections
denno020 replied to Spiew's topic in Javascript Help
There are a couple of ways you could do it: Save the base price into the DOM somewhere -> in a hidden div or hidden input or Use ajax to query the server for the base price every time the quantity updates Then, when the quantity is changed, multiply the base price by the new quantity, and set the result into the 'total' field. -
When a radio button is selected, set a cookie or use local storage to store the value of the radio selected. Then when the page refreshes, check to see if the cookie/local storage variable is set, and then use that to set the radio button value. That would be the theory to get the job done. Denno