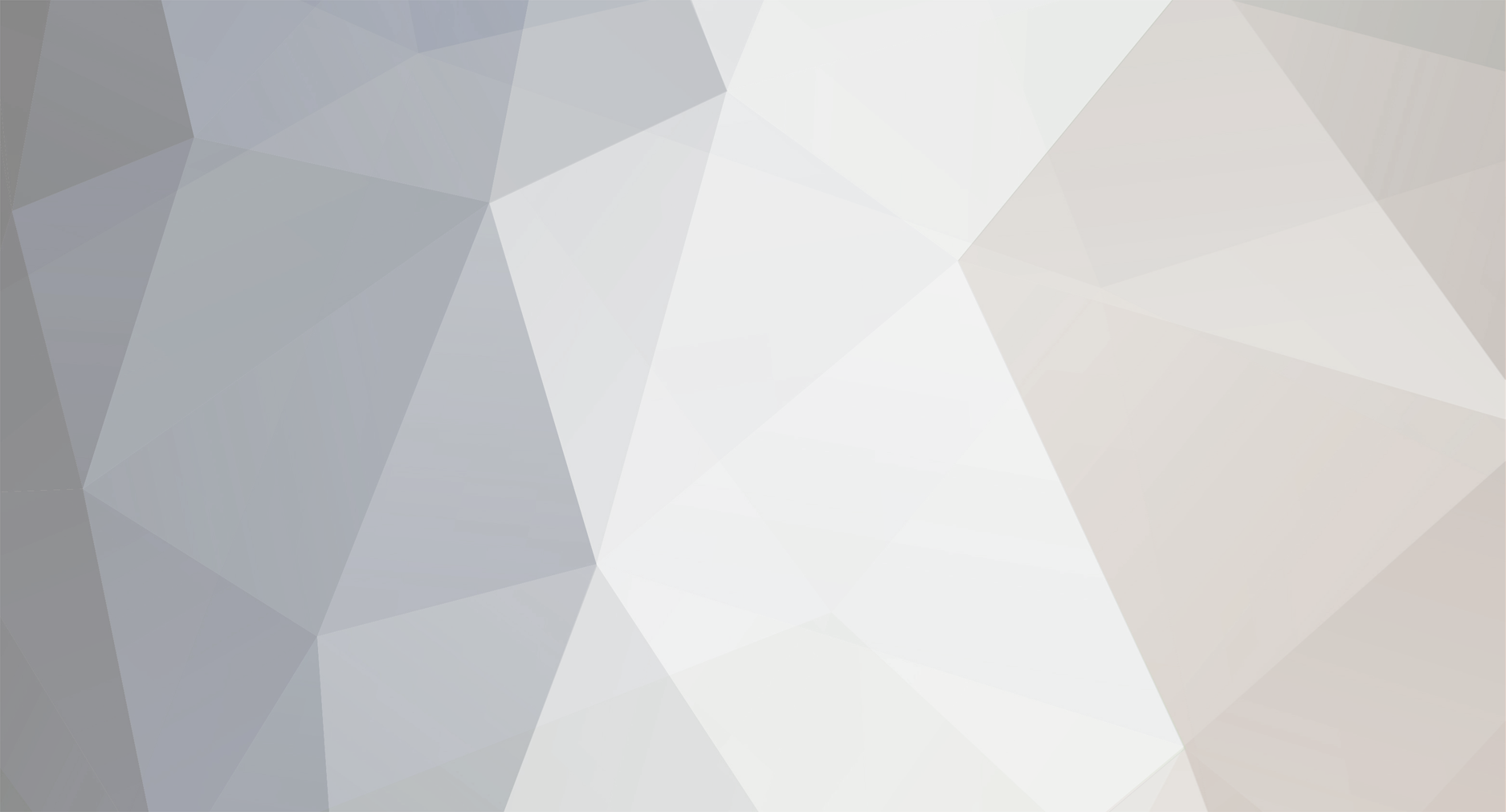
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
Can we see more code, and maybe a snippet of the relevant XML? It seems as if the $f == 'F' condition is always true.
-
Do you have a properly configured mail server? EDIT: Or SMTP relay or something. How is your server configured to send mail?
-
No! You don't want to be executing SQL queries in a loop if at all possible. Instead you can use batch insert syntax. $insertValues = array(); $kategorija = mysql_real_escape_string($_POST['kategorija']); foreach ($_FILES["uploaded_file"]["error"] as $key => $error) { if ($error == UPLOAD_ERR_OK) { $tmp_name = $_FILES["uploaded_file"]["tmp_name"][$key]; $name = $_FILES["uploaded_file"]["name"][$key]; move_uploaded_file($tmp_name, "$uploads_dir/$name"); $url = mysql_real_escape_string($_FILES['uploaded_file']['name'][$key]); $insertValues[] = "('" . $kategorija . "', '" . $url . "'"); } } $sql="INSERT INTO galerija_slike (kategorija_id, url_slike) VALUES " . implode(',', $insertValues);This will produce a query that looks something like:INSERT INTO galerija_slike (kategorija_id, url_slike) VALUES ('some category', 'some filename'),('some category', 'some other filename'),('some category', 'yet another filename')
-
You need to check the MIME type of the uploaded file. No, that doesn't mean look in the $_FILES data for it. You need to use something like finfo. For additional security measures, I like to disable script execution in the upload directory. You can do this in your Apache config, although I don't know the syntax off the top of my head.
-
I agree with CroNiX. Regex for this is a bit slow. However, since you asked for it, something like this should work: <a class="stripped-link lightblue-link profile-page-link" href=".*">(.*?)<\/a>You can adjust it depending on what kind of specificity you need. If you just wanted to match any old anchor tag, then maybe more like:<a.*>(.*?)<\/a>
-
There is very little reason to start from a blank slate with PHP today. I'm not sure what kind of customization you want, but I'm willing to bet that an off-the-shelf solution will do most of what you want, from the sounds of your post. Sure, there's no reason that wouldn't be possible. Come up with some basic wire frames which illustrate what kinds of data and workflow you want to have. If you know what you want, the developer should be able to pull it out of you. The important thing is knowing what you want before you get the developer.
-
Usually something like this works fairly well: $errors = array(); if(empty($FirstName)) { $errors['FirstName'] = '<p class="errText"> Please enter a value</p>'; }elseif(!preg_match('/^[a-z]+$/i',$FirstName)){ $errors['FirstName'] = '<p class="errText">Name may not start with a dash. Letters, spaces and dashes are accepted.</p>'; } // after validation... if (empty($errors)) { // there were no errors, carry on... // SQL inserts here... } else { // there was errors! display the form again and show errors }
-
How to extract information from an existing Array to a new Array?
scootstah replied to pescatore's topic in PHP Coding Help
array_search doesn't work on multidimensional arrays like you have. You'll have to loop over the outermost array first and then search on the inner one. Try something like this: $original = array( 1234567 => array('name' => 'john'), 3456 => array('name' => 'johnny'), 45673 => array('name' => 'james'), 987 => array('name' => 'jamie'), 5628721 => array('name' => 'Simon'), ); $filtered = array(); $search = 'jo'; foreach ($original as $index => $o) { if (stripos($o['name'], $search) !== false) { $filtered[$index] = $o; } } -
Is there any query errors? Are you sure your variables hold the data that you think they do? I would try changing it around a little like this: $sql = "UPDATE member SET rank= '$rank' WHERE username='$sql_user'"; mysqli_query($connection, $sql); if (($error = mysqli_error($connection)) != '') { echo 'mysql error: ' . $error . '<br />'; echo 'query: ' . $sql; }This way, your query is in a variable and you can output the exact query that is being executed. Also, note that you were using the wrong error function: mysql_error() instead of mysqli_error().
-
You are just using strings in the query values, instead of variables. $query = "UPDATE `bencobricks`. `users` SET `username` = '$username', `password` = 'NULL', `email` = 'email', `membership` = 'membership', `firstName` = 'firstName', `lastName` = 'lastName', `gender` = 'gender', `dateOfBirth` = 'dateOfBirth', `date` = 'NULL', `sets` = 'sets', `checkbox` = 'checkbox', `adminFlag` = 'adminFlag' WHERE `userID` = '$id'";
-
If you don't use a database, then the session data will only be stored on one server. Both servers could read the session cookie, but only one of them has the corresponding data. If you stored it in a database then both servers could access it. You could also do something like a network share, but that will probably be a lot slower.
-
No, it is short hand for this: $labels = array('B','KB','MB','GB','TB'); // $x will be one of: 0, 1, 2, 3, 4 $labels[$x]It's actually pretty clever.
-
And they always say bigger is better.... hmm....
-
No, just change your comparison to an int as well. if ($value !== 0) Ha, trust me we've all been there. We've all been stuck on stupid little problems like this. Sometimes you just need to walk away and do something else for a bit.
-
Well, then it won't work. Then your value is an int but you're comparing it to a string. Also, if your value was a non-int string before hand then it will be converted to int 0, which might be breaking your logic.
-
How is longone() supposed to be getting executed?
-
You should use strict type comparison. if ($value !== '0')This will look for string '0'.
-
You can have output, you just need to output the headers first.
-
If it's taking that long to arrive to Apache, then it seems like a network issue. It hasn't even reached your script yet.
-
Yes, you've pretty much got it. This is shorthand for array_push. Because, parse_url will give us the parts of the URL so that we can use them in the socket connection. Yes, parse_url parses a URL and returns an array of the components that make it up. Not random info, these are request headers which describe the request. Yes, which is what makes this non-blocking. My only problem with this approach is that your script can only assume that everything went as planned. You cannot get any response data, so you have no idea if the script had errors or not.
-
No, not necessarily. It depends on your traffic of course. If you only have a few dozen concurrent connections, you'll probably be fine with a smallish VPS. If you have hundreds or thousands of concurrent connections, then it's not going to be very cost effective to stay with VPS's. If you'd like to get a dedicated, check out http://www.soyoustart.com/us/essential-servers/ They have servers starting at like $42. The only caveat is that you basically have 0 support. You need to be able to completely manage the box for yourself. The support will help out if there is something completely out of your hands, like a disk fried or something. But they will not help with software issues.
-
Keep in mind that if your script takes too long, it might hit the script execution time limit which is set to 30 seconds by default. Another solution is to use a job scheduler such as Gearman. This would allow you to spin off a background task which will be non-blocking. But with that solution you would have to use polling to check the status of the operation.
-
If it makes you feel better, you can use session handlers to read/write sessions from wherever you want. You can write to the database, write to a cache store, whatever you want. However, before you go to all that work, have you actually profiled your application? Have you seen data that suggests that your page is slow because of iowait? If not you're jumping the gun a bit. Don't solve problems that don't exist yet.
-
Yes, once the $_SESSION is populated it will live for the life of the session (or until PHP expires it). So, you're free to rely on it through AJAX requests, assuming that the user has been authenticated prior. To deal with session expiry, your server will have to return an error that your javascript app can capture and handle. You will probably have to have some logic to queue anymore requests, while simultaneously calling an endpoint to re-authenticate the user, and finally executing all of the queued requests. Or, if you don't care about queued requests, you can just redirect the user and make them re-do the action.