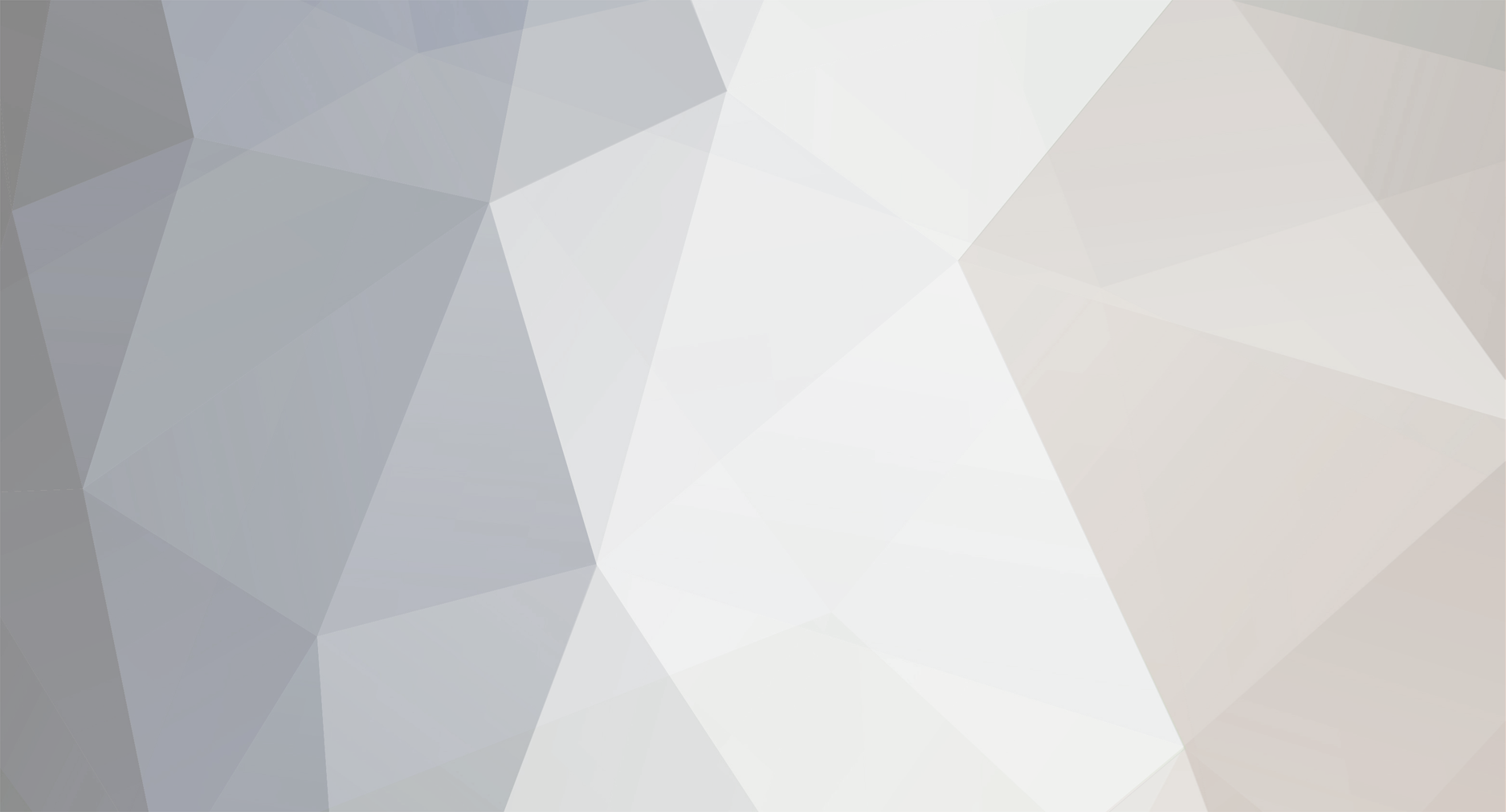
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
How to search data in xls sheet from search options
scootstah replied to gorants1234's topic in PHP Coding Help
Smells like homework. Do you have any code written? Are you stuck somewhere? -
That's silly. As long as you escape input or use prepared statements, you can't have SQL injection. This isn't really necessary, and still doesn't completely protect you from XSS in all cases. You should be escaping HTML on output, not input. You don't (necessarily) need to use strip_tags (although you can if you want), you just need to use htmlspecialchars. Well, that's a new one. And terribly wrong. You need to be escaping input, not encoding it.
-
A hash always produces the same length value regardless of the size of the input. W3Schools is garbage. You should be using more up-to-date resources. Also, it's good practice to not store modified data. Your test_input() function is modifying user input. You should be performing those tasks on output instead. Otherwise, you're limiting how your data could be used in the future. For example, what if, later on down the road, you decided that you wanted to allow (filtered) HTML? Well, now your database is full of broken tags and you've created a mess. Another reason is that if you escape on output instead of input, then you know that the data was escaped. The database isn't the only place to get input from.
-
There are some tools, but they're not 100%. Here's a couple good resources off the top of my head: https://www.owasp.org/index.php/PHP_Security_Cheat_Sheet http://www.phptherightway.com/
-
Caching files cause extra server hit.
scootstah replied to NotionCommotion's topic in PHP Coding Help
You might find that you have better luck using an HTTP proxy such as Varnish. The way you are caching, the request still has to be processed by Apache, and execute PHP code. But with something like Varnish it will never even get to Apache. You can even configure it so that the cache stays in memory, and then it won't even hit the disk. -
Implications of $_SERVER['REMOTE_PORT']
scootstah replied to NotionCommotion's topic in PHP Coding Help
Yes, it's normal. Usually the client will pick a random port. -
Need help recognizing the type of php class etc..
scootstah replied to tommytx's topic in PHP Coding Help
Assuming you're talking about this syntax: IDX_Plus::get_data('excerpt'); There are two ways to use classes. You can either instantiate them, or you can call static methods. When you instantiate the class, you create an object variable of that class type. You can then call the class methods, set properties, etc. This object variable will hold the state of the class. When you use static methods, you just call the method directly without instantiating the class or creating an object. Here is how that works in code. Instantiated: class HelloWorld { public function print() { echo 'Hello World!'; } } $helloWorld = new HelloWorld(); $helloWorld->print();Static method:class HelloWorld { public static function print() { echo 'Hello World!'; } } HelloWorld::print();Normally static methods should be avoided. They don't typically utilize good application design. Static methods are typically used with helper classes, where you might not need to instantiate it... you just want to run a quick method. A lot of times though, they're also used to (badly) avoid the need for dependency injection, which makes code really terrible. -
Yikes. I agree with QOC, adding that Javascript is probably the most painless way to handle it. About the sending HTML before headers, well, you could always wrap your output in an output buffer, which will allow all of the PHP to execute before any output is sent. It's an ugly way to do it but it's definitely the least of his problems.
-
What does "doesn't work" mean? Are you getting an error? Having to open a new database connection every time you execute a query is just a ludicrous waste of server resources. Before you said that $link->query was returning false. Did you do what I suggested and look at $link->error? Was there a query error?
-
Try a much more simple script to test if mail functionality is working properly. Simple execute this function: <?php $to = '[email protected]'; // change me mail($to, 'test subject', 'test body');If you don't get an email, then your server is either not configured properly, or the IP is blacklisted due to previous spam abuse. Unless you have tons of money to throw around, your best option for being able to reliably send mail is to let a third party handle it... something like Google Apps. They have a free SMTP relay along with their monthly plans which would allow you to send mail from your server (in the case that it is in fact blacklisted).
-
You're opening up two connections to the same database in the same script. There's absolutely no reason to be doing that. What led you to that conclusion? What is the problem with $link?
-
Right, but the 'q' part is outside of the foreach loop. So, you're only looking at the data from one node.
-
You can get rid of that Notice with: if (!session_id()) { session_start(); }So it works now? Awesome! I'm not really sure what the problem was, without experiencing it myself and being able to step through it.
-
Okay, so we have just confirmed that your sessions are working properly between pages. This is good. That's a bit strange then however, as like I said your script works perfectly fine for me. Let's try making the code a little smaller with less moving parts. Give this a whirl, and let's see what happens: <?php session_start(); if (!isset($_SESSION['REQUEST_URI'])) { $_SESSION['REQUEST_URI'] = array(); } $_SESSION['REQUEST_URI'][] = time() . ',' . $_SERVER['REQUEST_URI']; var_export($_SESSION['REQUEST_URI']);Also, as stated in the manual $_SESSION is a superglobal and is not affected by scope. It does not matter if it is in a function or not.
-
Adding "Login with Facebook" to existing app
scootstah replied to arbitrageur's topic in Application Design
Facebook uses an Oauth2 API. So, you'll need to be familiar with how that tech works. I'm not familiar with that UserCake system, so I can't really say what kind of work that will be. But unless someone has already done it, or unless there is already support for Oauth2, you'll probably have to dig very deep into that library and make significant changes. -
Do this simple test for me please. Create two files: page1.php and page2.php, with the following: page1.php <?php session_start(); echo '<pre>'; echo '------- PAGE1 OUTPUT -------<br />'; echo 'session_id: ' . session_id() . '<br />'; $_SESSION['foo'] = 'bar'; echo 'session data:<br />'; var_export($_SESSION); echo '<br />----------------------------<br />'; echo '</pre>'; page2.php <?php session_start(); echo '<pre>'; echo '------- PAGE2 OUTPUT -------<br />'; echo 'session_id: ' . session_id() . '<br />'; echo 'session data:<br />'; var_export($_SESSION); echo '<br />----------------------------<br />'; echo '</pre>';Put these files in the same directory. View page1.php, and then view page2.php. Copy/paste the output from each page in your reply.
-
Should I bother with ActiveX Object?
scootstah replied to moose-en-a-gant's topic in Javascript Help
HA! HAHA! One could only dream of such a wonderful utopia. But, thankfully, IE6 has very little users now. IE8 is the new IE6. Like maxxd said above, you can use jQuery for its AJAX library, which will make things a lot easier. It abstracts away the browser incompatibility stuff and has some nice shortcut methods. However, if you're only installing jQuery for the sole purpose of using AJAX, well then there are other, smaller libraries that will do the job as well. jQuery is a bit of a beast. -
Add this right underneath session_start(): echo session_id();Does this string change on every page load, or stay the same? If it changes, you might have the session cookie domain and/or path incorrectly configured.
-
Passing two variables via URL but not able to GET one of them...
scootstah replied to Jim R's topic in Applications
If you're still stuck, take a look at this answer: http://stackoverflow.com/a/20228400 Seems to be what you want. -
If it returned false then you should be able to get an error message with $link->error right after the query call.
-
webcam.js iphone/safari not supported?
scootstah replied to moose-en-a-gant's topic in Javascript Help
After a quick Google I found this: http://jsfiddle.net/2wZgv/ It seems to work on Android, but I don't have an iOS device to test. For your other question, generally you want to use the strictest permissions possible. 777 means that any user on the system can read/write/execute. Does every user need to be able to do that? No, not in most cases. Usually, only the Apache user needs only read+execute permissions on directories, and read permissions on files. Obviously if you want to be able to write within a directory then you will need write access, and/or if you want to write to a specific file you'll need write access to that file. But only the Apache user needs it. There are some exceptions, like if you're running CLI scripts or CRON jobs or something. -
Your script mostly worked for me. I changed two things: if (is_array($_SESSION['REQUEST_URI'])) {This gave an "Undefined index" notice on the first go. So I changed to:if (!empty ($_SESSION['REQUEST_URI']) && is_array($_SESSION['REQUEST_URI'])) {And:array_push($_SESSION['REQUEST_URI'], array($REQUEST_URI));This was creating (what I think is) undesired nesting, so I changed to:array_push($_SESSION['REQUEST_URI'], $REQUEST_URI);I get this result:Array ( [0] => 1422932734,/index.php [1] => 1422932737,/page.php [2] => 1422932741,/index.php )Note that it doesn't stop at 3, as you have no logic for that. It will just keep racking up. Did you make sure to put session_start() at the top of all your pages?
-
One of the best pieces of advice that I can give to beginners, is to make a habit of formatting your code properly. Your random/inconsistent spacing and indentation makes it hard to follow your code. You should always use consistent styling everywhere in your code, because it makes maintaining that code much easier later on. Plus, when you need other people to read it (like right now), it makes it that much easier for those people. Here is your code cleaned up: if (empty($errors)) { $userrname = test_input($_POST['userrname']); $email = test_input($_POST['email']); $newpassword = test_input($_POST['newpassword']); $hash = password_hash($newpassword, PASSWORD_BCRYPT, array("cost" => 9)); $stmt = $link->prepare("SELECT username,hash FROM User where username=? And email=?"); $stmt->bind_param("ss",$userrname,$email); if ($stmt->execute()) { $stmt->bind_result($username_from_db,$hash_from_db); if ($stmt->fetch()) { $_SESSION['user']=$username_from_db; $query = "UPDATE User SET hash='$hash' WHERE email='$email' And username='$username_from_db'"; if ($result=$link->query($query)) { $_SESSION['status_message'] = "Password has been reset"; } } else { echo "no good"; } $host = $_SERVER['HTTP_HOST']; $uri = $_SERVER['REQUEST_URI']; // the path/file?query string of the page header("Location: http://www.parsemebro.com/userpanel.php"); exit; $link->close(); } }Now that that's out of the way... Your problem should be pretty obvious. There are two most likely culprits here: either the query is failing, or the query does not contain the correct data (matching the wrong rows/no rows). So, step one is to make sure that the query actually executed without errors. If we look at the manual for mysqli::query, we can see that it returns FALSE when the query fails. So, you can check if the query failed and then show an error. if (($result=$link->query($query)) !== false) { $_SESSION['status_message'] = "Password has been reset"; } else { echo 'Query failed!<br />'; echo $link->error; }If the query is successfully executing, then the next most likely problem is that the query is not matching any rows, or not matching the rows that you expect it to. In that case, you should print out the $query variable and see exactly what the query is that is executing. One thing to note: you are using proper prepared statements in one spot, but are open to SQL injection in another spot. Bad!
-
So, I'm a little confused. Here you are looping over $xml->gms->g foreach($xml->gms->g as $game) { // if (!empty($game['vtn'])) { $games[(string) $game['gt']][] = $game; } }But here (right below it) you are not: if (($xml->gms->g['q']) == 'F') { $final = 'Final'; } else if (($xml->gms->g['q']) == 'FO') { $final = 'Final Overtime'; }You are only comparing the last index of $xml->gms->g (since you just iterated over it), which happens to be "F". I can't see what you're trying to do with $final, but I think you got some flawed logic in there.
-
I wasn't sure, so I just made a quick test. I found a jpeg image that had phpinfo() embedded. It is both a valid jpeg image and a valid PHP script. If I do include 'image.jpg';, it will execute phpinfo(). If I view the image, it is still a valid picture. I used imagecopyresampled and saved a new image which removed the PHP code and is now only a valid image. So, it seems that indeed recreating the image with PHP would remove any malicious code. The important thing though is that you only ever treat an image as an image. As long as you do that it shouldn't really matter.