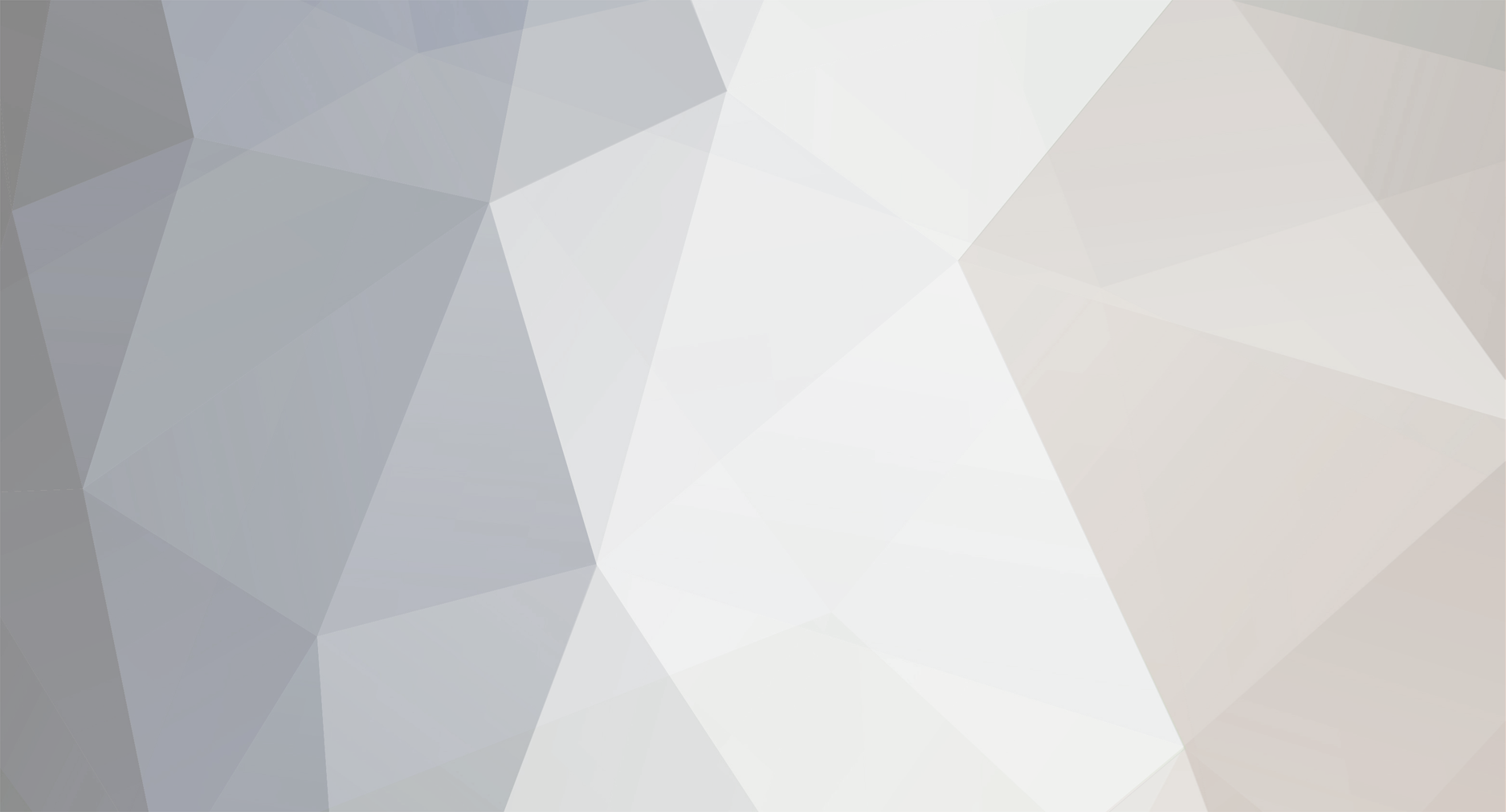
nodirtyrockstar
Members-
Posts
108 -
Joined
-
Last visited
Everything posted by nodirtyrockstar
-
I am trying to create a list with array elements. It should add a comma after every element except for the last. I have the following code: foreach ($srch as $value) { $query .= "`" . $value . "`"; if (next($srch)){ $query .= ","; } } It is adding a comma after everything except for the LAST TWO elements. Can someone help me understand this? I have read the php.net manual about next() and it seems like it should work for this purpose. Also, I realize there are other ways to do this, but right now I would really like to understand next().
-
Html Form: Checkbox And Text Inputs
nodirtyrockstar replied to nodirtyrockstar's topic in PHP Coding Help
I made a mistake elsewhere in my code! Thanks, your answer helped me find it. -
I have a table contained by a form. Each row of the table contains the following data cells: <td> <input type='text' name='cart[ccr-01-002]' size='2' /> </td> <td> <input type='checkbox' name='delete[ccr-01-002]' /> </td> Rather than returning whether or not the checkbox is checked (such as ON or OFF), it is instead returning the value of the text input field. Here is how I am trying to reference it: var_dump($_POST['delete']); This prints out the string contents of the text fields instead of ON or OFF. Is it possible (without having to put the checkbox into its own form) to reference the checkbox as a separate entity from the text input? I would like to reference $_POST['cart'], and not have it affect $_POST['delete']. Please let me know if you need any other information!
-
I thought I could use ON DUPLICATE KEY UPDATE, but that is problematic since there are multiple unique keys.
-
I would like to update a table or insert if a specific combination of indices do not already exist. The table is very simple. CREATE TABLE `sessProdLnk` ( `products_id` varchar(20) NOT NULL, `sessions_id` varchar(255) NOT NULL, `qty` int(6) NOT NULL, KEY `products_id` (`products_id`), KEY `sessions_id` (`sessions_id`) ENGINE=MyISAM DEFAULT CHARSET=latin1 So, I would like to search for a match between my data, and any row in sessProdLnk where both the sessions_id and products_id match. Then I would like to update any entries in qty where they match, and insert a new row for any data pairs that do not have matches. I know some of this comparison may have to be done programmatically in the php script, but I am still getting to know MYSQL and was wondering how much of the work it will do for me given these parameters.
-
Thanks for all of the help with answering my question about security for this one very simple function!
- 12 replies
-
- validation
- user input
-
(and 1 more)
Tagged with:
-
@Christian - While I appreciate the design principle lesson, I don't really think you simplified my function. If the array is empty, the function finishes. If the array is not empty, and the input passes validation, it is cast as an integer and the function returns true. Furthermore, the array that this function will return is a list of products, and the only thing stored in the subarray is the desired quantity. Therefore, the array will NEVER have more than one index. @Pikachu2000 - The function is not intended to return data. I will cast my data as int elsewhere. I really appreciate all of the coding advice, but I am really interested in security at this point. Is there another forum I should post to which specializes in security?
- 12 replies
-
- validation
- user input
-
(and 1 more)
Tagged with:
-
I see what you're getting at, but I am working with a 2D array, and each subarray contains only one entry.
- 12 replies
-
- validation
- user input
-
(and 1 more)
Tagged with:
-
Okay. I took all of your suggestions and now I have this: function validate($array){ if (!empty($array)) { foreach($array as $product){ if(ctype_digit($product[0])){ $product[0] = (int)$product[0]; return true; } else{ return false; } } } }
- 12 replies
-
- validation
- user input
-
(and 1 more)
Tagged with:
-
No decimals needed! This is a very helpful tip, thank you. Other than making sure to use the correct function to validate the numbers, can you (or anyone else on here) see any other security concerns?
- 12 replies
-
- validation
- user input
-
(and 1 more)
Tagged with:
-
Start them with an underscore? You can add/remove it dynamically. Or use a letter, or some other allowed character.
-
Retrieving Data Partially From The Database
nodirtyrockstar replied to heshan's topic in PHP Coding Help
It sounds to me like you want to return the student's full name and reduce the string of their first name so that it displays only their first initial. If that is the case, a simple call to substr() will allow you to truncate the string as you wish. Check it out here: http://www.php.net/manual/en/function.substr.php -
I have a form which contains a dynamic number of text fields that are intended for shoppers to input a positive number only. I separated the validation into a function. It is working well for me so far, but given that security is such a major concern I thought I would ask for comments from the forum. Here's the function: function validate($array){ if (count($array) > 0) { foreach($array as $product){ if(is_numeric($product[0]) && $product[0] >= 0){ return true; } else{ return false; } } } } When the form is sent, the returning page does a little bit of it's own configuring; functions relevant to the page itself and the user's context. Then the script checks to see if input was sent. Then it validates the data before attempting to use it. There are even checks further down in the script that continue to compare it as though it is numeric, and that it is greater than or equal to zero. Is this sufficient?
- 12 replies
-
- validation
- user input
-
(and 1 more)
Tagged with:
-
Here is a row from my merchandise table: <tr> <td>Product ID</td> <td>Artist</td> <td>Title</td> <td>Album Art</td> <td>Label</td> <td>Year</td> <td>Price</td> <td> <input type='text' name='cart[dawn of chaos][]' value='0' size='2'/> </td> </tr> I would like to direct your attention to the input row. In my PHP, the input field actually says "name=cart[$title][]" etc... Then I would like to reference the multidimensional array with PHP. To begin I just added a simple foreach. foreach($_POST['cart'] as $item){ echo $item; } PHP says this: Array ( ) Notice: Undefined index: cart in /you/probably/don't/need/my/filepath/cart.php on line 6 Warning: Invalid argument supplied for foreach() in....
-
Myisam Table: Composite Key As Substitute For Foreign Key?
nodirtyrockstar replied to nodirtyrockstar's topic in MySQL Help
I have a basic grasp on foreign key v composite key now, but I am still struggling with this...so maybe you all can help after all. I am developing a low security shopping cart for an online record shop. It is low security because we are not storing, sending, or processing any customer information. There are no user IDs or passwords that pertain to specific customers. Their "carts" will persist in a database which will be referenced by their PHP session ID. There are three tables: products, sessions, and sessProdLnk. Here is the detail for each of them: CREATE TABLE `products` ( `id` varchar(20) NOT NULL, `artist` varchar(30) NOT NULL, `title` varchar(30) NOT NULL, `artwork` varchar(255) NOT NULL, `label` varchar(30) NOT NULL, `year` year(4) NOT NULL, `price` decimal(6,2) NOT NULL, `qty` int(11) NOT NULL, `agedOff` tinyint(1) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 CREATE TABLE `sessions` ( `id` varchar(255) NOT NULL, `created` datetime NOT NULL, `expired` datetime NOT NULL, `completed` tinyint(1) NOT NULL, `price` decimal(6,2) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 CREATE TABLE `sessProdLnk` ( `products_id` varchar(20) NOT NULL, `sessions_id` varchar(255) NOT NULL, `qty` int(6) NOT NULL, KEY `products_id` (`products_id`), KEY `sessions_id` (`sessions_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 Products will contain the inventory, and can only be changed by my client, the site owner. The sessions table contains cart specific data, such as when it was created, when it expires, whether or not the transaction was completed, and the total price of all the items in the cart. The sessProdLnk table contains the product id, the session id, and the quantity of the item. In other words, this table will actually be capable of referencing the list of products and quantities associated with any particular session. Since the product table has no dependency on the other two tables, I am not worried about it. However the price of a cart relates directly to how many products, and the quantities of each product, that are connected to each session. I assume that this is an issue people have faced before. What is the smartest way to enforce referential integrity in this database? Can you refer me to resources or share your knowledge as to best practices given my parameters? -
Myisam Table: Composite Key As Substitute For Foreign Key?
nodirtyrockstar replied to nodirtyrockstar's topic in MySQL Help
Alrighty, so a little more research leads me to believe that a foreign key is a composite key that links two columns across different tables, and a composite key links two columns in the same table. Does that sound right? After doing a little more research, I think I found that I don't need foreign keys after all. Thanks anyway! -
My shared web host does not allow for InnoDB tables. I was planning to use them to take advantage of the foreign key constraint. Now I am going to try to make do with MyISAM, and it seems like the next best thing is a composite key. Would you agree with that? Where can I find information about how to correctly set that up? In my research, I keep finding endless information about foreign keys, and less about the concept of composite keys. Any help you can offer would be greatly appreciated. Thank you in advance for your consideration.
-
Take A Look At This Query Real Quick
nodirtyrockstar replied to computermax2328's topic in PHP Coding Help
Your variable ('$table') is in quotes. I don't know if that matters since I can't see the rest of your script. Also, have you tried plugging the query directly into MySQL? It helps to start with a good query, and then troubleshoot the PHP. -
I actually got it all to work, so I decided to post it in case someone comes across this thread. I used the singleton pattern to extend mysqli. Here's my class: class Database extends mysqli{ private static $database; private function __construct(){ global $dbhost, $dbuser, $dbpass, $db; parent::__construct($dbhost, $dbuser, $dbpass, $db); if ($this->connect_error) { die('Connect Error (' . $this->connect_errno . ') ' . $this->connect_error); } } public static function getInstance() { if(!self::$database) { self::$database = new Database(); } return self::$database; } public function __clone() { die(__CLASS__ . ' class can\'t be instantiated. Please use the method called getInstance.'); } } Then you can reference the class like so: $mysqli = Database::getInstance();
- 3 replies
-
- mysqli
- connection
-
(and 1 more)
Tagged with:
-
Well, I now see this is much more complex than I originally thought, and I am unable to edit or delete the first post...unless I am missing something. So please disregard.
- 3 replies
-
- mysqli
- connection
-
(and 1 more)
Tagged with:
-
I have multiple scripts referring to one another and it is getting a little confusing with database calls. I stumbled upon the singleton method, and I am trying to use it to create a Database class that will make sure there is only one connection at a time. This is what I have: class Database{ //Store the single instance of Database private static $m_pInstance; private function __construct(){ $mysqli = new mysqli($dbhost, $dbuser, $dbpass, $db); if ($mysqli->connect_error) { die('Connect Error (' . $mysqli->connect_errno . ') ' . $mysqli->connect_error); } } public static function getInstance() { if(!self::$m_pInstance) { self::$m_pInstance = new Database(); } return self::$m_pInstance; } } My connection definitions are stored in a cfg.php file which is required by this script. For the most part I understand how this works, but not well enough to understand why it isn't working. Here is the reference to this database: $mysqli = Database::getInstance(); Can someone help me figure out what I am doing wrong? The connection fails when I run it. It works when I put the above reference into a comment and simply connect with this: $mysqli = new mysqli($dbhost, $dbuser, $dbpass, $db); if ($mysqli->connect_error) { die('Connect Error (' . $mysqli->connect_errno . ') ' . $mysqli->connect_error); } Thanks.
- 3 replies
-
- mysqli
- connection
-
(and 1 more)
Tagged with:
-
Php Mysqli -- Database Connection Failure
nodirtyrockstar replied to nodirtyrockstar's topic in PHP Coding Help
Good idea. Now that the connection errors are done screaming at me, I can work through what should be happening inside the loop. I'll post more specific questions about what I am dealing with now. Thanks for your help everyone. -
Php Mysqli -- Database Connection Failure
nodirtyrockstar replied to nodirtyrockstar's topic in PHP Coding Help
Oh I was referring to the first script. I guess that was confusing. The following queries: $query = 'SELECT `id`,`artist`,`title`,`artwork`,`label`,`year`,`price` FROM `products` WHERE (`qty` <> 0) AND (`agedOff` <> 1)'; $query = 'SELECT `id`,`artist`,`title`,`artwork`,`year`,`price` FROM `products` WHERE (label = "cobra cabana records") AND (`qty` <> 0) AND (`agedOff` <> 1)'; only have two differences. I would like to figure out how to create them dynamically. -
Php Mysqli -- Database Connection Failure
nodirtyrockstar replied to nodirtyrockstar's topic in PHP Coding Help
Yes, I want to pull things out of the loop. Maybe I am being dense, but I got hung up on the fact that one queries seven fields, and the other only six. So if I use your $query = "INSERT INTO myCity (Name, CountryCode, District) VALUES (?,?,?)"; Then how do I get around a variable number of placeholders?