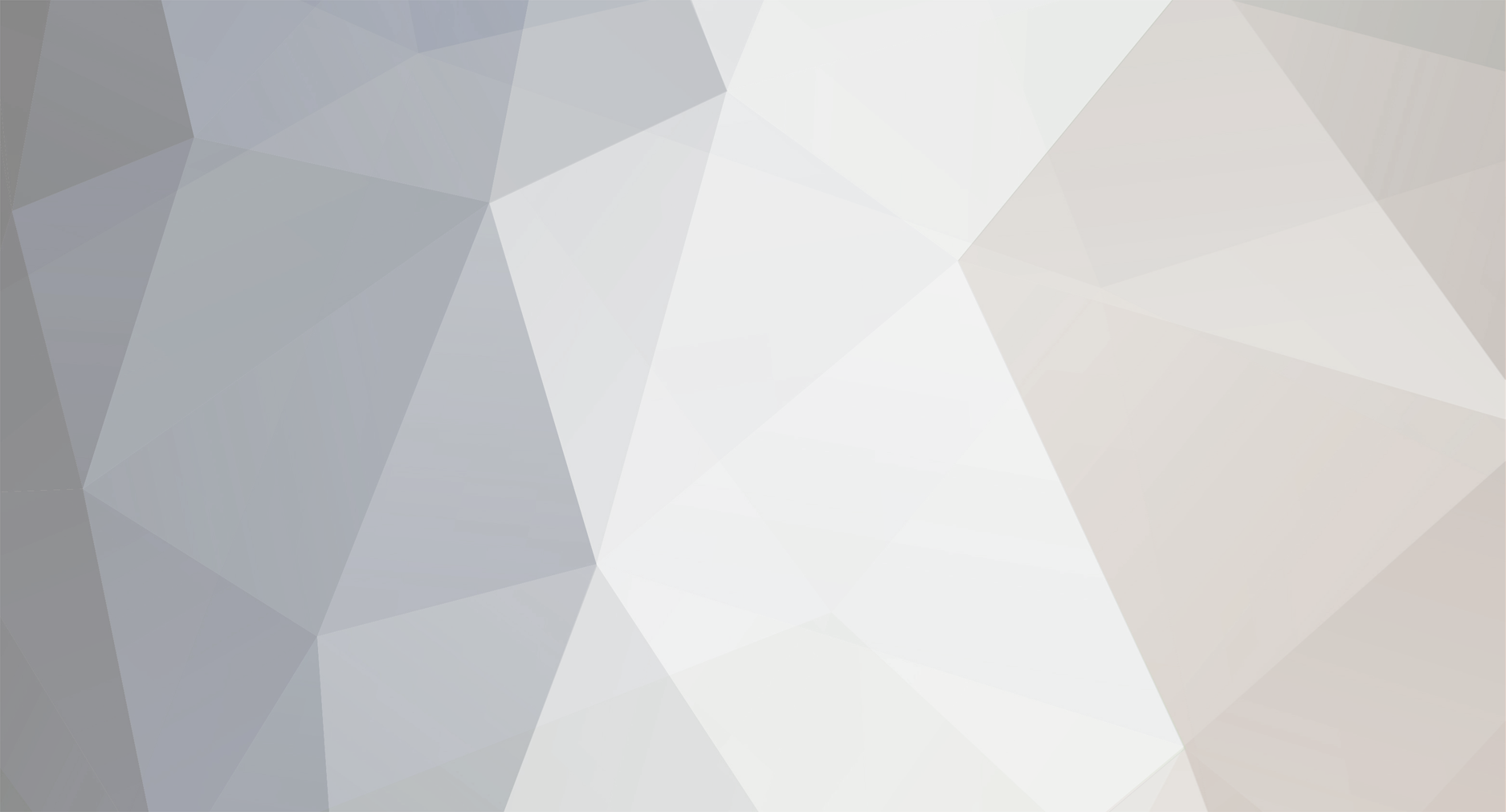
JD*
Members-
Posts
230 -
Joined
-
Last visited
Everything posted by JD*
-
According to php.net, you don't need to close mysql connections or use mysql_free_result...both are handled when the script is finished executing. Can't hurt to include it, but may be unnecessary.
-
PHP Project - TennisPlanner [Guidance Request]
JD* replied to danTheWalker's topic in PHP Coding Help
If I were designing this, I would have three tables: 1 for players, which would have an ID and name 1 for tables/courts, which would have an ID and name/number 1 for registrations, which would hold the playerID(s), tableID(s) and date I'm assuming that someone besides the player will be entering the data, as if everyone is logging in with the same account you won't have an easy way of letting them enter their own data. And that every friday has one available time for each table only? Either way, it would wind up working that when a person enters the site, the database would be queried this way: Query the tables/courts so we can see all of them For each table, query the schedule so we can see who is playing and when. If empty, display nothing, else display a name above and below a table graphic. On clicking the radio button, enter the player's name into the database for the specific date (another set of information either on the main page, or a sub-page for making the reservation). Then shoot out a confirm email. If you'd like more advise on it, let me know in either this thread or a PM and I'll be more than happy to help -
You'll have to figure out if each of those numbers has a class or ID assigned to it and then either make sure your CSS reflects it, or add the id/class to your numbering. Quickest way to find out is to read the source code, or you can install the web developer bar in Firefox and use the Outline->Current element tool, which shows you everything about the current element when you put your mouse over it.
-
You'd probably better off storing less unique data in your session. Take a look at my login script: if(check_required2("user_name, password")) { $_SESSION['error'] = "required_missing"; unset($_POST); redirect("http://admin.mysite.com"); exit(); } $result = mysql_query("SELECT * FROM permissions WHERE userName = '".addslashes($_POST['user_name'])."'") or die(mysql_error()); if(mysql_num_rows($result) != 1) { $_SESSION['error'] = "nosuchuser"; unset($_POST); display_login(); exit(); } if(md5($_POST['password']) == mysql_result($result, 0, "userPassword")) { $_SESSION['id'] = mysql_result($result, 0, "ID"); $_SESSION['logged_in'] = 1; unset($_POST); redirect("?action=home"); exit(); } else { unset($_POST); $_SESSION['error'] = "badcombo"; display_login(); } So in here, I do check their name and password, but then I store their unique user_id into a session. Then, on each page, I can query that ID so that they only see their own information. Also, I have a custom function for my error reporting and login, so you can ignore those. session_register, by the way, is depricated, so you'll want to steer away from it in the future.
-
I'm trying to figure out if I can structure a query so I can replace one column of data, while leaving the rest alone. My query looks like this: SELECT * FROM database.survey WHERE building = '4' And the result looks like ID Building Teacher question_1 question_2 ... 10001015423613... So what I'm looking to replace is the "teacher" column, which right now only contains an ID. I have a staff table that has their name, so I'm wondering if I can replace their ID with their name, but still keep my query with the "SELECT *", as it's a pain to have to add to the select statement every time someone decides to add another question (and most of these questions have sub questions). I know I can do this easily in PHP with a loop, but I want to push as much processing on the server as possible.
-
I have a PHP function that is half built that does this. What happens is when a user submits the form, in the processing logic it runs a check_required("field_name_1, field_name2, field_name3"). check_required sees if the $_POST['field_name'] is empty or not, and if it is, puts it into a session called "required" and returns 1; Back on the form logic, if check_required returns 1, it redirects them to the same page. Each of these pages has a function called display_error() that checks for the session['error'] and prints out a message and explodes the "required" session. One last function called "fill_in_field" will grab fields that are already filled and, when reloading the form, fills them back in. It's not as easy to use as javascript, but it's a lot more reliable for multiple browsers. If you're interested, I'll post the source.
-
Yeah, in my production version there is my error display function to help them figure out what went wrong. In our future, as we transition from multiple login servers to one master, we'll be making it so that if a user logs in with their name and there is more than one user, we'll give them a list of names with IDs, which will then be used, with the password, to try and complete the bind. I don't know that we've used account locking so far, but that is also something interesting to look into.
-
I got it figured out, thanks to all of your suggestions. What I do now is, when they submit their credentials, I do a search based on whatever it is they submit (ID Number OR Common Name). Then I pull their actual UID, unbind, and then rebind with the UID and password. This way, at one building where the UID is lastnamefirstinitial, and another where it's firstname.lastname and so on, I can use the same code and just toss in the proper DN. Following is the function I made tot allow this: function ldap_auth2($server=NULL, $username=NULL, $password=NULL, $dn=NULL) { error_reporting(0); // Surpress the error message when an account fails to bind $ds=ldap_connect($server); // must be a valid LDAP server! ldap_set_option($ds, LDAP_OPT_PROTOCOL_VERSION, 3); // Set our protocol or else this won't work // Search the server for user with the information they submitted if ($ds) { $r=ldap_bind($ds); $sr=ldap_search($ds,$dn, (is_numeric($username) ? "uidnumber=" : "cn=").$username ); } $ldapResults = ldap_get_entries($ds, $sr); $username = "uid=".$ldapResults[0]['uid'][0]; // Get their UID ldap_unbind($ds) // close this connection $connect=ldap_connect($server) or die("Could not find server"); ldap_set_option($connect, LDAP_OPT_PROTOCOL_VERSION, 3); // The above is anonymous, but we can only guarantee access if they can rebind to the server with the uid and password. if(ldap_bind($connect, $username.",".$dn, $password) == 1) { return 1; } else { return 0; }; ldap_unbind($connect); } And then the item that calls the function works like this: case "Log In": //Do LDAP login if(ldap_auth2('my.server.com', $_POST['username'], $_POST['password'], "cn=users,dc=my,dc=server,dc=com") ==1) { echo "You did it!"; } else { echo "You didn't do it"; } Thanks guys!
-
Right now the $dn variable is set to be static, so I'm not too worried about that, I'm more concerned with being able to use a user's name. I'm just not sure how to go about that. As it stands, we're using OS X servers as our LDAP, so their name is "lastname, firstname", but their short name is their ID, so they can log into a computer using either, so I'd like to make it so that they can log into our website using either.
-
Hello all, I'm trying to work with a flexible LDAP setup for logging in to alternate servers. I have the code working for logging in with an ID number, but I'm trying to get it working with a username. I have a set of code that shows me the LDAP attributes on my server, but so far I can't get the cn (as username) to work. The following is the code that I'm using, hopefully it'll help: $connect=ldap_connect($server) or die("Could not find server"); ldap_set_option($connect, LDAP_OPT_PROTOCOL_VERSION, 3); $bind = ldap_bind($connect, (is_numeric($username) ? "uid" : "cn")."=$username,$dn","$password"); if($bind == 1) { return 1; } else { return 0; } ldap_unbind($connect); Any help would be greatly appreciated.
-
Sorry Barand, tried to work in some PHP stuff but it was mostly JS and CSS.
-
Yes. I choose to use sessions, but you can do it any way you want, check the following: session_start(); $result = mysql_query("SELECT * FROM db"); for($i=0;$i< mysql_num_rows($result);$i++) { $_SESSION['content'].=mysql_result($result, $i, "text").", ".mysql_result($result, $i, "text2"); } header("Content-Disposition: attachment; filename=pdexpressimport.txt"); header("Content-type: text/plain"); header("Pragma: no-cache"); header("Expires: 0"); print $_SESSION['content']; That will give you the option to download the text file when you visit the page. If you want to write to a file on the server, read the following: http://www.tizag.com/phpT/filewrite.php
-
I'll try to answer your questions in order: It's fairly easy to make a link to each page and then have one other page (or the same page) that can read a URL variable and pull the appropriate data from the database. You can even combine a mod_rewrite rule to hide the actual URL variable and make it look nice (but save that until later). As for your "related titles"...never done anything like it, but I'd imagine you'd want to do a query along the lines of SELECT * FROM movies where rating = (this_movies_rating) OR director = (this_movies_director)... etc, where you'll replace the stuff in () with the actual movie information (dynamically). As for the star rating, yes, there are easy scripts out thre for ajax start rating systems, or making a regular one, which isn't very hard.
-
Also, via css: tr { background: white; } tr:hover { background: #FF0000; }
-
[SOLVED] Possibly a simple PHP image manipulation question
JD* replied to Petsmacker's topic in PHP Coding Help
Check out http://www.codewalkers.com/c/a/Miscellaneous/Overlapping-Images-with-GD/1/ -
No, it would be more like: $result = mysql_query("SELECT COUNT(y) AS count from table WHERE Test='y'"); echo mysql_result($result, 0, "count");
-
Problem with $title and active menu item styling...? (newbie)
JD* replied to MischiefManaged's topic in PHP Coding Help
You have to do it like this: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <? include("required.php"); ?> <title><?php echo $title; ?></title> and then in your required, set all your variables, like $thispage and $title. You can make them dynamic (ie say $thispage = basename($_SERVER['REQUEST_URI']); ) because they are included onto the page, then executed, then stored into the variable space. -
Sorry, didn't realize there was a PHP integration for this. Check out http://us2.php.net/chmod
-
exec("Chmod -R /directory");
-
Sounds like you need to look into a perl script that can emulate the behavior of a user agent. Start at google looking for WWW::Mechanize
-
In the SQL statement, do SELECT COUNT(Y) from database
-
Problem with $title and active menu item styling...? (newbie)
JD* replied to MischiefManaged's topic in PHP Coding Help
I do not see $thispage defined anywhere but in your menu and the body code. But if the body code is executed after the menu is included on the page, then the menu will never get the variable, as PHP is executed in a linear fashion, ie. top to bottom, left to right. You're best bet would be to define the $thispage variable as the first thing on your page, before you do any of your includes. -
Those are variables. You can use those to pass information to a page (using $_GET) to do database queries or affect other code on the page. For example, if I wanted to make something that displays a news article, my URL may look like article.php?id=15 then in my page I will have code like mysql_query("SELECT * FROM news WHERE ID = '".$_GET['id']."'") or die(mysql_error()); That will make my query dynamic, only selected the record with an ID of what I want at the time.
-
Are you trying to have it updated on the same page, or after a post? If after a post, you can access it by $_POST['testName']. If it's on the same page (ie, select from the dropdown and then the value changes on a different part of the page) you'll need to update it with a javascript function that will change the innerhtml of a div
-
For that kind of interaction, you'll need to look into using a database along with php. If you have specific questions, I'll be more than happy to help, but general tutorials on inserting and updating rows will get you about 90% of the way.