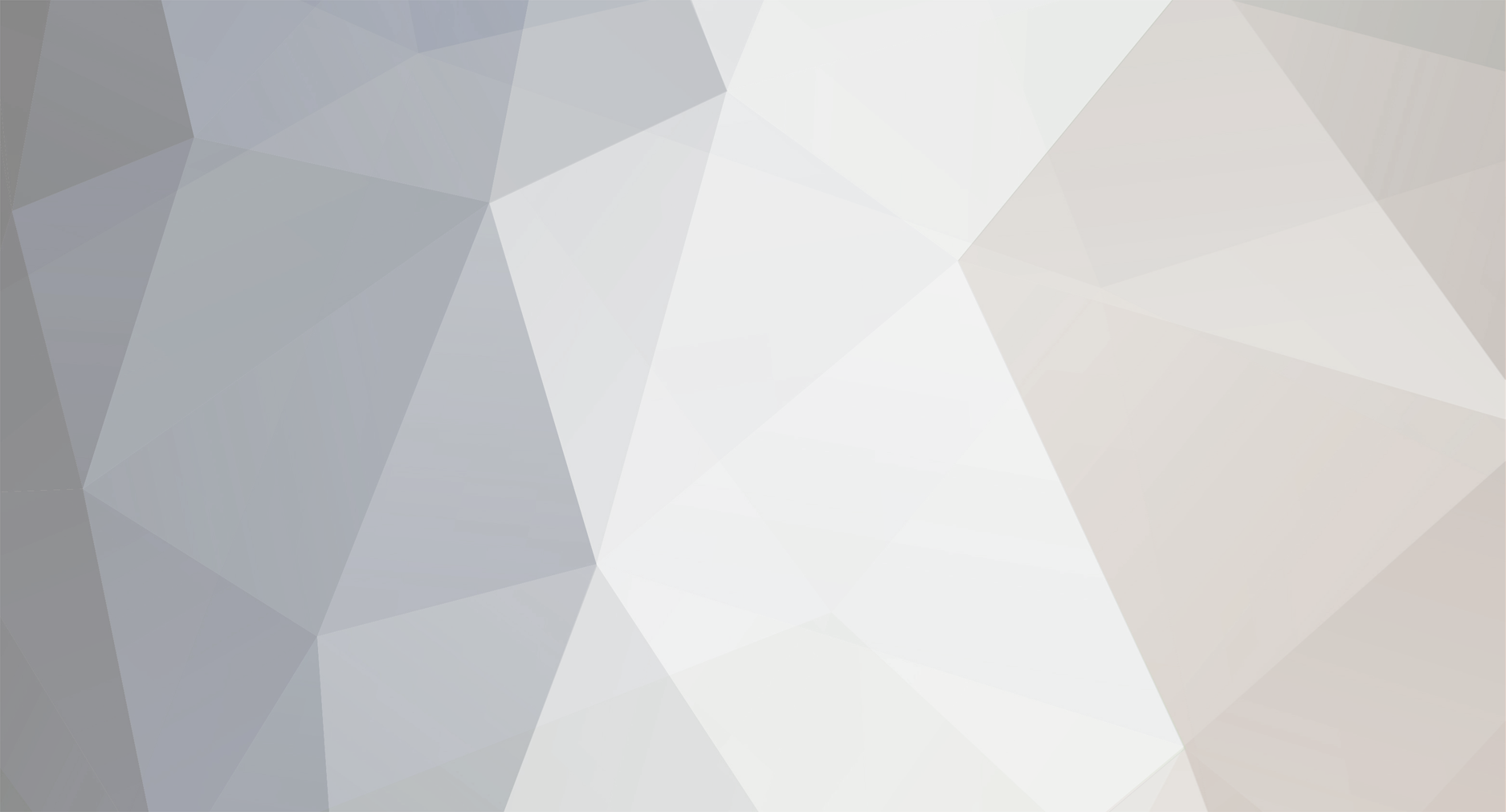
imgrooot
Members-
Posts
383 -
Joined
-
Last visited
-
Days Won
1
Everything posted by imgrooot
-
Say below is my normal html href link. How would you turn this into a pretty URL using .htaccess? Also if I understand it correctly, this link will also have to be changed to match the pretty url. What would the new link look like? <a href="shop.php?type=45&name=belts">Belts</a>
- 5 replies
-
- pretty urls
- url
-
(and 3 more)
Tagged with:
-
I have a Mysql table where I store prices of a product. I have the values in "varchar" format because I needed to include "decimals" in the prices(for eg. 15.30) and "int" doesn't allow that. Having done that, when ever I filter the products by prices in Ascending or Descending order, they don't show up in proper order. It seems like the decimal in the prices is messing up the order. Is there a way to fix this so that the php query can filter the prices in proper order despite the decimals? Here's the eg of the query. $get_records = $db->prepare("SELECT * FROM records ORDER BY records.price DESC");
-
I have it working now. And yes it is a CSS problem. Here's the css code. Note that "Overflow-y" will only work if the position is defined. Without the "position:fixed", my nav-mobile dropdown didn't open when I clicked toggle. .nav-mobile { display: none; *zoom: 1; position: fixed; width: 100%; overflow-y: scroll; -ms-overflow-y: scroll; -webkit-overflow-scrolling: touch; // mobile safari }
-
Right now I have a mobile dropdown that works fine. The only issue I have is that when viewing it on my smartphone, the dropdown gets cut off at the bottom and I am unable to scroll it down to rest of it. Is there a way to fix it so that It's scrollable? Here's my js code of the dropdown. $(document).ready(function() { $(".nav-mobile li a").each(function() { if ($(this).next().length > 0) { $(this).addClass("parent-arrow"); } }) $(".toggle-mobile-menu").click(function(e) { e.preventDefault(); $(this).toggleClass("active-mobile"); $(".nav-mobile").toggle(); }); adjustMenu(); }); $(window).bind('resize orientationchange', function() { ww = document.body.clientWidth; adjustMenu(); }); var adjustMenu = function() { var ww = document.body.clientWidth; if (ww <= 850) { $(".toggle-mobile-menu").css("display", "block"); if (!$(".toggle-mobile-menu").hasClass("active-mobile")) { $(".nav-mobile").hide(); } else { $(".nav-mobile").show(); } $(".nav-mobile li").unbind('mouseenter mouseleave'); $(".nav-mobile li a.parent-arrow").unbind('click').bind('click', function(e) { // must be attached to anchor element to prevent bubbling e.preventDefault(); $(this).parent("li").toggleClass("hover"); }); } else if (ww > 850) { $(".toggle-mobile-menu").css("display", "none"); $(".nav-mobile").hide(); $(".nav-mobile li").removeClass("hover"); $(".nav-mobile li a").unbind('click'); $(".nav-mobile li").unbind('mouseenter mouseleave').bind('mouseenter mouseleave', function() { // must be attached to li so that mouseleave is not triggered when hover over submenu $(this).toggleClass('hover'); }); } }
-
Say I have 2 tables. Table 1: Type(type_id, type_name) Table 2: Records(record_id, record_name, record_type) Under the "record_type" column, is it better to use type_id(eg. 10) or type_name(eg. Clothing & Watches)? If the answer is use the name, is it better to use the original name(Clothing & Watches) or a slug name(clothing-watches)?
-
This is so strange. I have this bit of code that removes the .php extension from pages so that I can visit any page without typing .php at the end. RewriteEngine On RewriteCond %{REQUEST_FILENAME} !-d RewriteCond %{REQUEST_FILENAME}.php -f RewriteRule ^(.*)$ $1.php [L] The above code has always worked for me before. But for some reason today it stopped working on localhost and the live server(ipage hosting). Do you know why that might be?
-
Question 1. I have a form and on submit it redirects to another site. Is it possible to open that site in a new tab instead of the same page? I have tried this js code and it doesn't seem to work. if() { $get_url = 'https://www.google.ca/'; ?> <script> window.open('<?php echo $get_url; ?>','_blank'); </script> <?php } Question 2. Is it possible to have a extend popup to another site using a unique link? Take the above google link for example. Is it possible to add new code at the end of that link url which will show a popup window or something on that google page if only visited through that link?
-
I am using this https://github.com/ircmaxell/password_compat I have no problem using it on a local server. But as soon as I test it on a live server, it gives me an error like this. Parse error: syntax error, unexpected '{' in /home/public_html/sub/snippets/password.php on line 19 The error is on the same line as the beginning of the code in that library. Why is this happening?
-
How do you show php foreach loop results as ajax mousehover?
imgrooot replied to imgrooot's topic in PHP Coding Help
Yep the above works if I have the ".show-details" div as a child of parent div ".product" like this. <div class="product"> <div class="product-title"> <?php echo $get_record_title; ?> </div> <div class="product-price"> <?php echo $get_record_price; ?> </div> <div class="show-details"> <?php echo $get_record_details; ?> </div> </div> -
How do you show php foreach loop results as ajax mousehover?
imgrooot replied to imgrooot's topic in PHP Coding Help
So something like this? <script> $(document).ready(function() { $(".product").mouseover(function(){ $(".show-details").css("display", "block"); }); $(".product").mouseout(function(){ $(".show-details").css("display", "none"); }); }); </script> -
How do you show php foreach loop results as ajax mousehover?
imgrooot replied to imgrooot's topic in PHP Coding Help
The thing is, if I have 10 records on a page and I use the above javascript code to show the hidden div, it will show the div for all 10 records at the same time. I don't want that. I just want it show a div for each unique record one at a time as I hover my mouse over them. So that is why I am asking what the javascript code will look like to make that work? -
Here's what I'm trying to do. I retrieve results using php foreach loop. Within that loop results, I want to show a div(.show-details) on mouse over only. How do I do that? This is my code so far. <style> .show-details { display: none; } </style> $get_records = $db->prepare("SELECT * FROM records WHERE record_id = :record_id"); $get_records->bindParam(':record_id', $record_id); $get_records->execute(); $result_records = $get_records->fetchAll(PDO::FETCH_ASSOC); if(count($result_records) > 0){ foreach($result_records as $row) { $get_record_title = $row['record_title']; $get_record_details = $row['record_details']; $get_record_price = $row['record_price']; ?> <div class="product"> <div class="product-title"> <?php echo $get_record_title; ?> </div> <div class="product-price"> <?php echo $get_record_price; ?> </div> </div> <div class="show-details"> <?php echo $get_record_details; ?> </div> <?php } } else { echo 'no results.'; } <script> $(document).ready(function() { $(".product").mouseover(function(){ $(".show-details").css("display", "block"); }); }); </script>
-
Say I have this loop. foreach($record as $row) { <div class="hello">Hello World!</div> } I want to loop through and add a letter alongside the "Hello World!" above. To do that, I found this code. $azRange = range('A', 'Z'); foreach ($azRange as $letter) { print("$letter\n"); } How can I combine the two loops so that I get a result like this? A Hello World! B Hello World! C Hello World! D Hello World! E Hello World! F Hello World!
-
I am trying out a new script that lets me resize an image before uploading. It is based on this script. http://www.w3bees.com/2013/03/resize-image-while-upload-using-php.html Basically what happens is, it resizes and makes 3 thumbnails and puts them in their relative folder. That works. The part that's giving me the problem is when inserting it into the database. Error says the "image_path" cannot be null. Since it's creating an array of 3 different thumbnails, should I create 2 more fields in the database tabel to account for that? If so, how would I insert the 3 different thumbnail paths into the query? What would it look like? Below is my code. <?php $db_userid = intval($row['user_id']); $get_item_id = intval($row['item_id']); // settings $max_file_size = 5242880; // 5mb $valid_exts = array('jpeg', 'jpg', 'png', 'gif'); // thumbnail sizes $sizes = array(100 => 100, 150 => 150, 250 => 250); // dir paths $target_dir = 'images/'.$db_userid.'/items/'.$get_item_id.'/'; if ($_SERVER['REQUEST_METHOD'] == 'POST' AND isset($_FILES['image'])) { if(!empty($_FILES['image']['name'])) { if($_FILES['image']['size'] < $max_file_size ){ // get file extension $ext = strtolower(pathinfo($_FILES['image']['name'], PATHINFO_EXTENSION)); if(in_array($ext, $valid_exts)) { function resize($width, $height){ global $db_userid; global $get_item_id; /* Get original image x y*/ list($w, $h) = getimagesize($_FILES['image']['tmp_name']); /* calculate new image size with ratio */ $ratio = max($width/$w, $height/$h); $h = ceil($height / $ratio); $x = ($w - $width / $ratio) / 2; $w = ceil($width / $ratio); /* new file name */ $path = 'images/'.$db_userid.'/items/'.$get_item_id.'/'.$width.'x'.$height.'_'.$_FILES['image']['name']; /* read binary data from image file */ $imgString = file_get_contents($_FILES['image']['tmp_name']); /* create image from string */ $image = imagecreatefromstring($imgString); $tmp = imagecreatetruecolor($width, $height); imagecopyresampled($tmp, $image, 0, 0, $x, 0, $width, $height, $w, $h); /* Save image */ switch ($_FILES['image']['type']) { case 'image/jpeg': imagejpeg($tmp, $path, 100); break; case 'image/png': imagepng($tmp, $path, 0); break; case 'image/gif': imagegif($tmp, $path); break; default: exit; break; } return $path; /* cleanup memory */ imagedestroy($image); imagedestroy($tmp); } /* resize image */ foreach($sizes as $w => $h) { if(!is_dir($target_dir)){ mkdir($target_dir, 0775, true); } $files[] = resize($w, $h); } $insert_image = $db->prepare("INSERT INTO images(user_id, item_id, image_path, date_added) VALUES(:user_id, :item_id, :image_path, :date_added)"); $insert_image->bindParam(':user_id', $db_userid); $insert_image->bindParam(':item_id', $get_item_id); $insert_image->bindParam(':image_path', $path); $insert_image->bindParam(':date_added', $item_date_added); if(!$insert_image->execute()) { $errors[] = 'There was a problem uploading the image!'; } else { if(empty($errors)) { $db->commit(); $success = 'Your item has been saved.'; } else { $db->rollBack(); } } } else { $errors[] = 'Unsupported file'; } } else{ $errors[] = 'Please upload image smaller than 5mb'; } } else { $errors[] = 'An image is required!'; } }
-
Basically the "title" and "details" inputs are for showing text on a page. I need CKEditor for the "details" input so that the user can modify the text. That seo_description input is suppose to have the same value as the "details" input. So instead of making the user write the same text again in the seo_page_description textarea, I am having it so that it automatically adds to it from the "details" input. And since seo_page_description is only going to showing in the meta details in the header, I only need it in plain text. One thing I noticed. The textarea that has the CKeditor; it inserts the text(with tags) into the MySql database fine. I can also retrive it from the database fine. It includes all the html tags. However the text is not styled by default. I have to style the tags with css for the text to appear as the user has intended in the Ckeditor. Is this normal?