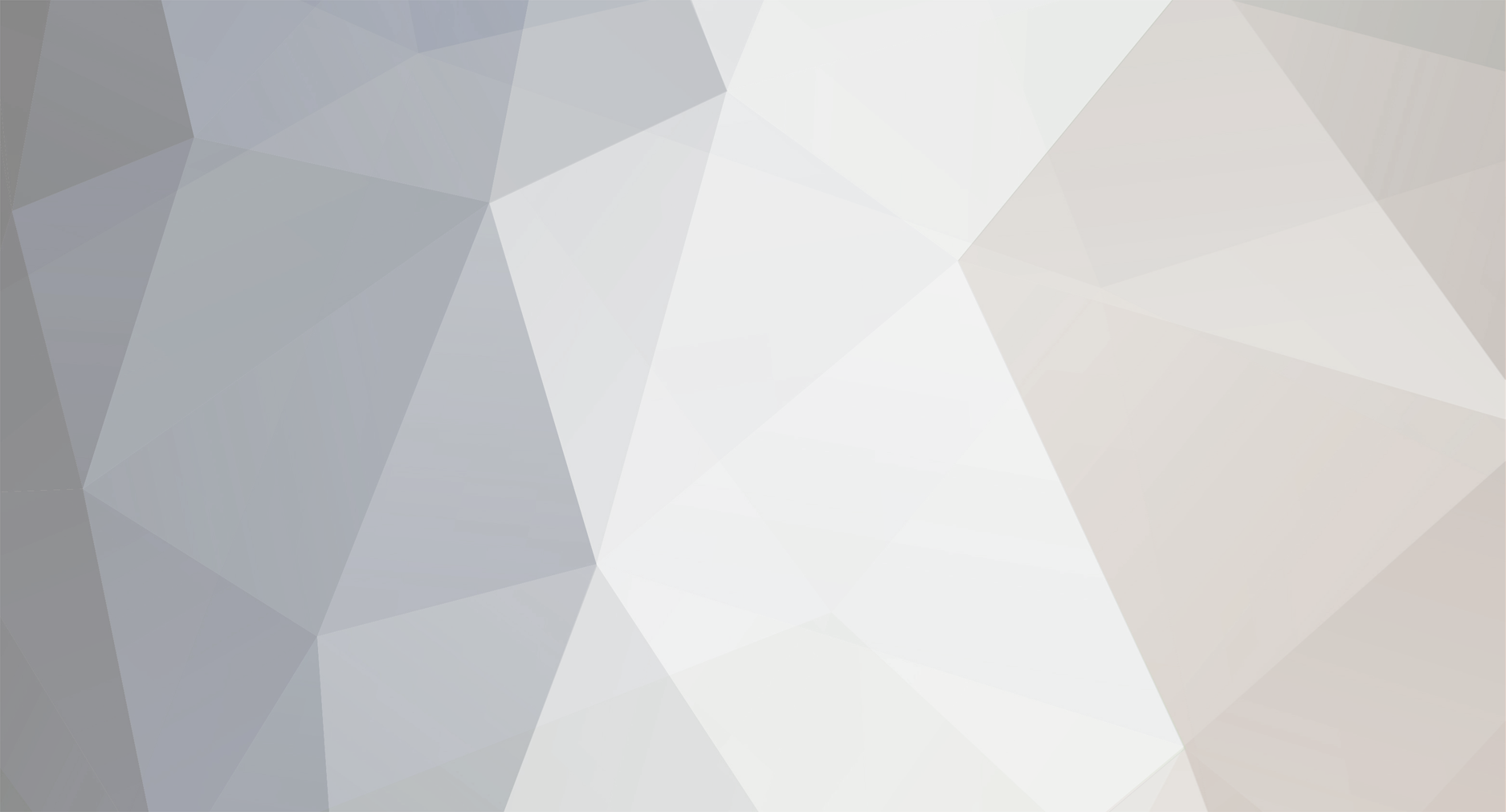
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
That would be because you close the while loop and THEN echo out the data - the data needs to be echoed inside the while loop, otherwise only the last result would be displayed. Try doing this: <?php $sql = "SELECT t.*,user_images.ext as ext,users.ID as pid FROM $tablname as t,users,user_images WHERE t.account = '$user2' AND t.nick=nick AND users.ID = user_images.user_id ORDER BY t.id DESC LIMIT 10"; $result = mysql_db_query($database,$sql,$connection) or die(mysql_error().'<br />Query was:'.$sql); while($row = mysql_fetch_assoc($result)){ $nick = $row["nick"]; $url = $row["url"]; $message = $row["message"]; $datetime = $row["datetime"]; $senton= date("M jS, Y \a\\t h:i A T", $datetime); $id = $row['pid']; $ext = $row['ext']; //echo out as required echo 'Username:'.$nick.'<br />'; echo 'URL:'.$urk.'<br />'; echo 'Message:'.$message.'<br />'; echo 'Time:'.$senton.'<br />'; echo 'Img: <img src="http://www.r.com/members/images/mini/'.$id.'.'.$ext.'" /><br />'; echo "<hr />\n"; } ?> Do the above to verify that you are getting the data you want, then worry about the formatting of it. -
Or even just here on these boards - the quick option to reply and the quick modify buttons are much nicer than having a whole new page reload.
-
How do I search mysql databse for a value?
GingerRobot replied to des1017's topic in PHP Coding Help
The manual really is the best place to answer that. The number refers to the row number of the result set. As im sure you can imagine, a lot of queries return more than one row, so the function needs to know which row you are looking at. The manual would also help you with ths kind of thing: If you check the page for the function (http://www.php.net/mysql_result) and look at the section called return values, it would help you answer the above question. -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
This line: $ID = $row['id']; Should be: $ID = $row['pid']; -
Yes, exiting the script will stop all following output. Futhermore, if you're changing location then there wont be any output anyway! Die and exit are equivalent - it makes no odds which one you use.
-
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
Well I for one am not going to sit here and unpick your logic. As far as I can see there are missing braces all over the place. Short and concise code (i.e. with just the one query) is much easier to debug. If you insist on following your version, i suggest the first step would be to fix the indentation and get rid of the unnecessary whitespace. It'll be easier to follow the flow of the code that way. -
[SOLVED] How can I make this more secure?
GingerRobot replied to Cory94bailly's topic in PHP Coding Help
I wouldn't escape a username - use regex to make sure only certain characters are allowed. For example, to allow alphanumeric characters plus spaces and underscores and to set a minimum of 3 and a maximum of 20 characters, use: if(!preg_match('|^[a-z0-9 _]{3,20}$|i',$user)){ //username not ok - alert user and do not enter into database } There would then be no need to use mysql_real_escape_string() on this field. -
How do I search mysql databse for a value?
GingerRobot replied to des1017's topic in PHP Coding Help
You want to obtain a count of the number of rows where the username field is equal to the supplied username. This can either be done with the mysql_num_rows() function or, more efficiently, with the sql COUNT function: $result = mysql_query("SELECT COUNT(*) FROM yourtable WHERE username='$username'") or die(mysql_error()); if(mysql_result($result,0) > 0){ //user name already taken }else{ //user not taken -insert into database } -
There seems to be quite a few examples of this in the user contributed notes in the manual.
-
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
Seem to have an extra as ext in there for some reason, not sure how that got there. Try: <?php $sql = "SELECT t.*,user_images.ext as ext,users.ID as pid FROM $tablname as t,users,photos WHERE t.account = '$user2' AND t.nick=users.nick AND users.ID = user_images.user_id ORDER BY t.id DESC LIMIT $howmany"; $result = mysql_db_query($database,$sql,$connection) or die(mysql_error().'<br />Query was:'.$sql); while($row = mysql_fetch_assoc($result)){ $nick = $row["nick"]; $url = $row["url"]; $message = $row["message"]; $datetime = $row["datetime"]; $senton= date("M jS, Y \a\\t h:i A T", $datetime); $pid = $row['pid']; $ext = $row['ext']; //echo out as required } ?> -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
Well i'll start with the full stop. You use a full stop to separate a table and a field name. Hence user_images.ext is the ext field from the user_images table. The t is an alias. It allows you to do alias.fieldname rather than longtablename.fieldname. You set an alias with the AS keyword. You can also use aliases for the names of the selected fields. This is useful both to eliminate the table name or function from the result set (that is, so you dont have to use $row['tablename.fieldname'] or $row['COUNT(*)'] - instead you could just use $row['fieldname'] or $row['count'] ) and for the use of the selected fields in more complex queries. -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
As i say, i suggest you rewrite the above and join your tables. It'll be something like: <?php $sql = "SELECT t.*,user_images.ext as ext,users.ID as pid as ext FROM $tablname as t,users,photos WHERE t.account = '$user2' AND t.nick=users.nick AND users.ID = user_images.user_id ORDER BY t.id DESC LIMIT $howmany"; $result = mysql_db_query($database,$sql,$connection) or die(mysql_error()); while($row = mysql_fetch_assoc($result)){ $nick = $row["nick"]; $url = $row["url"]; $message = $row["message"]; $datetime = $row["datetime"]; $senton= date("M jS, Y \a\\t h:i A T", $datetime); $pid = $row['pid']; $ext = $row['ext']; //echo out as required } ?> -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
A blank page generally indicates that you have display_errors set to off, but do have errors in your page. The errors in question are unclosed braces - you have if statements that have not been closed. However, it is not necessarily a question of just adding the closing braces - they may have been required earlier in the script. Just placing them at the end will more than likely result in logic problems. All that said, you're really going a long way round of doing this. Using a join in the query would be much simpler and more efficient. Speaking of which, you shouldn't select all the columns from a table if you only need one or two. -
To be honest, i dont think there would be a lot of point in having people creating their own competitions. If there were more than one people run I dont think people would actually bother to enter. And those that did would more than likely not produce anything decent. That said, I would like to see the competition idea back.
-
HTML inside PHP or PHP inside HTML, what to do?
GingerRobot replied to Ge64's topic in PHP Coding Help
It's almost entirely user preference, though it does depend on the situation. For situations with lots of HTML and little PHP, i close the PHP tag and only echo variables where i need them. For something with lots of variables, i'd echo everything. As i say though, this is personal preference. There are of course other altenatives, like the heredoc syntax. -
Someone was going to spend £10,000 on apples?! Thats going to be one hell of a pie!
-
Maths based ones would be even easier for a bot to sovle...
-
Not entirely true - with PHP 5.2.2 and a windows environment, you could use the imagegrabscreen or imagegrabwindow functions. Never used them myself, so im unsure of the limitations - but it's worth a look.
-
Last time i looked, they only did for their premium accounts(or whatever they were called). No idea if they still even have those. Switch to googlemail! Edit: Looks like that's still the case: http://get.live.com/mailplus/features#ID0EIKAC
-
Retrieving data from MySQL specific fields in a cycle
GingerRobot replied to Kyrus's topic in PHP Coding Help
Stick the variables in an array and cycle through till you find a non-empty one: <?php $var1 = ''; $var2 = ''; $var3 = 'foo'; $var4 = 'bar'; $vars = array($var1,$var2,$var3,$var4); foreach($vars as $v){ if(!empty($v)){ echo $v; break; } } ?> -
Pesonally, i think you'd make it much more user friendly if you did use some AJAX - i would have it set up so that the link showed a small text field just below the drop down box, with a button. Once the button is clicked, you could update the drop-down box, and use AJAX to add the option to the database.
-
Retrieving data from MySQL specific fields in a cycle
GingerRobot replied to Kyrus's topic in PHP Coding Help
The idea would be to check to see if the description field is empty - if it isn't, print out the description. If it is, print out the model and size etc. So it would be something like: <?php $sql = "SELECT id,description,model,size FROM yourtable"; $result = mysql_query($sql) or die(mysql_error()); while($row = mysql_fetch_assoc($result)){ echo 'Product ID: '.$row['id']; echo '<br />'; if(!empty($row['description'])){ echo $row['description']; }else{ echo 'Model: '.$row['model'].' Size: '.$row['size']; } echo '<br /><br />'."\n"; } ?> P.S. Welcome to the forum -
That's because its a VARCHAR field. An auto-increment field should be an INT field.
-
[SOLVED] Extract & Parse Form Field Values from External Site
GingerRobot replied to phoenixx's topic in PHP Coding Help
You can just insert new rows based on the contents of the array: <?php $data ='<!-- Sample Data --> <input type="hidden" name="U2FsdGVkX18goKhw3PNp1hoNW1XtlDrynp2vpujLUk91T-mrCFgp_zPaHUQ0ntTK1qkDcubva4Y" value="test"> <input type="hidden" name="U2FsdGVkX185P0eiDtlWUsO1e5nu-uKU9zRVqCup_wQaMp55L082EJ9d1z8AGEd9bOEQRrJII0U" value="Test"> <input type="hidden" name="test" value="v335d"> <input type="hidden" name="postingKey" value="uLLKc4c9H4h5QZoe"> <input type="hidden" name="U2FsdGVkX1_tQgrdT02gqfhvLU9azdevjvwPNGRYZ1bLpPLQrw6n3Aq4q2uqoUC6" value="C"> <input type="hidden" name="U2FsdGVkX18L31E19JPgAISK-7vJbKFiOvwvOIL0ffYr-vWaUykl9OM7NOqr8cYz" value="Test"> <input type="hidden" name="FromEMail" value="samples@mydomain.net"> <input type="hidden" name="Type" value="B"> <input type="hidden" name="mix" value=""> <input type="hidden" name="contactEmail" value=""> <!-- End Sample Data --> '; preg_match_all('/<input.*?name="([^"]*)".*?value="([^"]*)"/is',$data,$out); $d = array_combine($out[1], $out[2]); $insert_into = array(); foreach($d as $k=>$v){ $insert_into[] = '('.$k.','.$v.')'; } $insert_into = implode(',',$insert_into); mysql_query("INSERT INTO yourtable(fieldname,fieldvalue) VALUES $insert_into") or die(mysql_error()); ?> -
They get the PHP source code? I expect you're using the short style opening tags (<?) but have them disabled in your php.ini. Either enable the short_open_tags option, or switch to full opening tags (<?php). The latter is better in my opinion, since it's more portable. Alternatively, the server may have previously been set up to parse other file extensions(other than .php that is) as PHP files. If this is no longer so, then you would again see the source code.