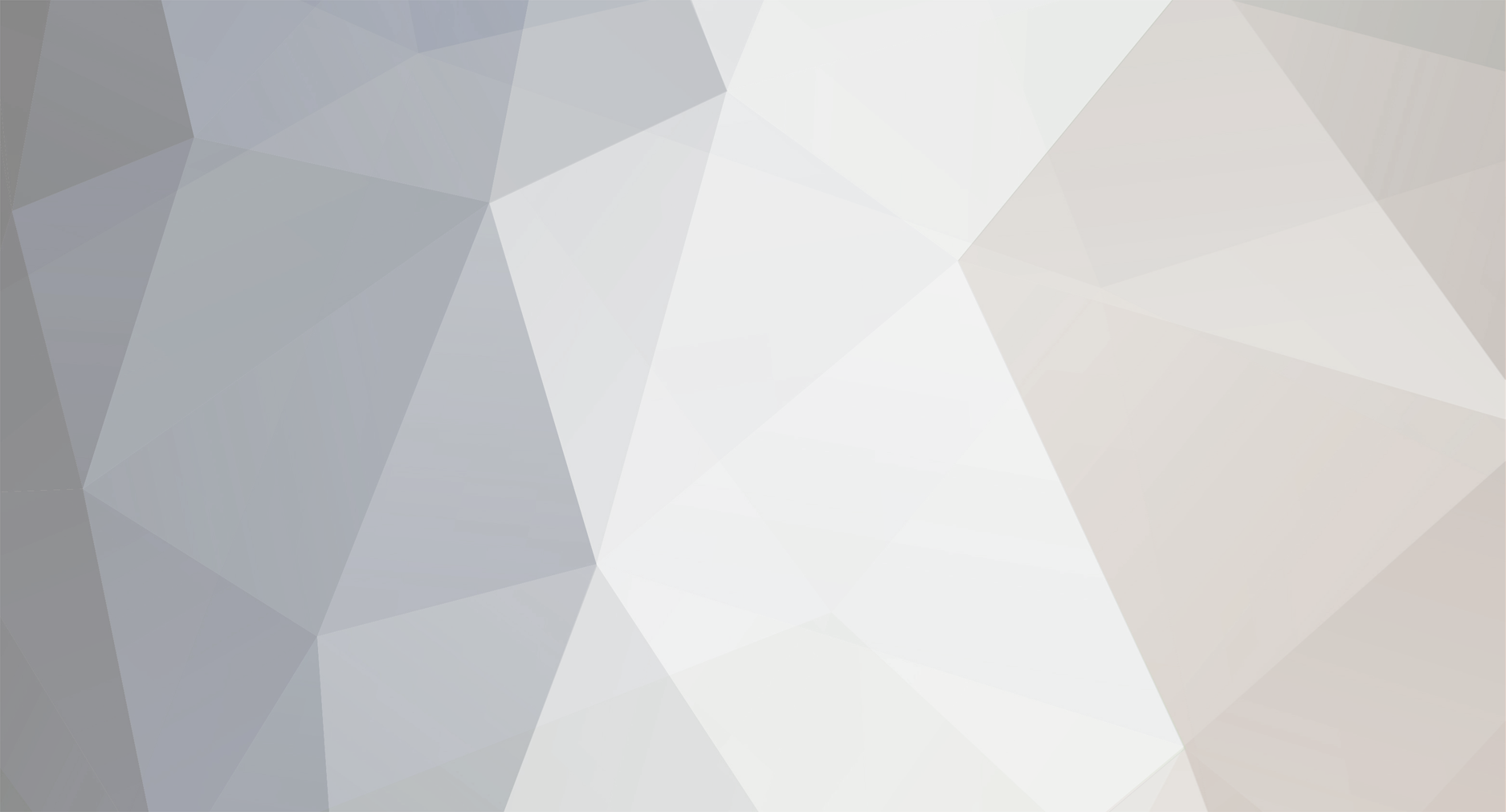
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Ok so I had to make too much tweaks to the above function to make it re-usable across multiple scenario's. I also dropped the multi-dimensional array and made it single-dimensional. And I was looking for a Iterator-like solution because storing them meant possible memory issues. Also using an Iterator-like solution allows me to 'page' through them. This is what I ended up with: /** * Original author: * @author http://stereofrog.com/blok/on/070910 * * Preserved by: * @author http://stackoverflow.com/a/3742837 */ class Combinations implements Iterator { /** * @var array */ private $offsets = null; /** * @var array|string */ private $set = null; /** * @var integer */ private $length = 0; /** * @var integer */ private $width = 0; /** * @var integer */ private $key = 0; /** * @param array|string $set * @param integer $width */ public function __construct($set, $width) { if (is_array($set)) { $this->set = array_values($set); $this->length = count($this->set); } else { $this->set = (string)$set; $this->length = strlen($this->set); } $this->width = $width; $this->rewind(); } /** * @return CombinationsMemento */ public function getMemento() { return new CombinationsMemento($this->set, $this->length, $this->key, $this->offsets, $this->width); } /** * @param CombinationsMemento $memento */ public function setMemento(CombinationsMemento $memento) { $this->set = $memento->getSet(); $this->length = $memento->getLength(); $this->key = $memento->getKey(); $this->offsets = $memento->getOffsets(); $this->width = $memento->getWidth(); } /** * @return integer */ public function key() { return $this->key; } /** * @return array|string */ public function current() { $result = array(); for ($i = 0; $i < $this->width; $i++) { $result[] = $this->set[$this->offsets[$i]]; } return is_array($this->set) ? $result : implode('', $result); } /** * */ public function next() { if ($this->_next()) { $this->key++; } else { $this->key = -1; } } /** * */ public function rewind() { $this->offsets = range(0, $this->width); $this->key = 0; } /** * @return boolean */ public function valid() { return $this->key >= 0; } /** * @see http://mysite.verizon.net/res148h4j/javascript/script_combinations.html#the%20source%20code * @return boolean */ protected function _next() { $i = $this->width - 1; while ($i >= 0 && $this->offsets[$i] == $this->length - $this->width + $i) { $i--; } if ($i < 0) { return false; } $this->offsets[$i]++; while ($i++ < $this->width - 1) { $this->offsets[$i] = $this->offsets[$i - 1] + 1; } return true; } }What I am doing here is as a combination is generated, I inspect it and if it's valid I store it in a fixed array. Once the array is filled, it retrieves the memento and stores the fixed array and memento in the database. At a later time the last memento is retrieved and the process proceeds.
-
getID3 is a PHP function. You can install it locally through composer: https://packagist.org/packages/james-heinrich/getid3 Or you can simply download it as a .zip and extract it in your project root: https://github.com/JamesHeinrich/getID3/archive/master.zip
-
Something like this: $cars = array(); foreach ($list as $car) { $year = substr($car, -4); $model = substr($car, 0, strlen($car) - 5); $cars[$model][] = $year; } foreach ($cars as $model => $years) { echo $model . ' '; if (count($years) == 1) { echo $years[0]; } else { echo min($years) . '-' . max($years); } echo '<br>'; }
-
Recommendation on Error Handler Library FOSS
ignace replied to Firemankurt's topic in Other Libraries
Here's a nifty one for during development: https://github.com/filp/whoops For production you can do something like: set_error_handler(function($errno, $errstr, $errfile, $errline, $errcontext) { throw new ErrorException($errstr, 0, $errno, $errfile, $errline); }); set_exception_handler(function($exception) use ($pimple) { $pimple['logger']->error($exception, array('exception' => $exception)); $pimple['twig']->display('error500.html.twig', array('exception' => $exception)); exit; });'logger' is:https://github.com/Seldaek/monolog/tree/master/src/Monolog -
Pimple container -vs- defined constant for SITEPATH etc...
ignace replied to Firemankurt's topic in PHP Coding Help
Though a constant is perfectly fine keep in mind that you don't create any hard dependency between your global constant and any class. class Foo { private $bar = GLOBAL_CONSTANT; }Foo now has a hard dependency on GLOBAL_CONSTANT, pass the constant through the constructor if it needs it's value: $foo = new Foo(GLOBAL_CONSTANT); -
That's not what he said, he said to do this: echo 'this var was undeclared before this';
-
In your signature it say's: And you do the opposite. You counsel your fears for instructions.
-
Well, this is not a relationship counsel but a programming forum. But I think you are being unreasonable to let him make such promises. A relationship is about trust, if you don't trust your boyfriend with another girl around him (which happens when he is with friends, at work, ..) then you should end the relationship.
-
A wise man once said: 1) Increase success by lowering expectations 2) Only make promises you can keep. So my new year resolutions only include things I am already doing. Looking back, I have realized all of my new year resolutions for the last 5 years. My new year resolutions: 1) GetKeep in shape. 2) Stay ahead of the pack (colleagues). 3) Have sex 3 times a week (I kept this vague for good reasons, it doesn't mention with girlfriend, achieve by any means necessary). 4) Be awesome.
-
No bi-directional relationships are fine. Though your example is awkward at best, why not simply call addit() on LCMSCore instead of creating a separate class to do that for you? Also, separation of concern. Why does LCMSCore create the Access class? What if their will be multiple ways to build the Access class in the future? Will you add all of these methods on LCMSCore? And what if you need to do more then simply add? multiply? divide? substract? namespace Math; interface Operation { public function execute(); } class Operand implements Operation { private $value; public function __construct($op) { $this->value = $op; } public function execute() { return $this->value; } } abstract class AbstractOperation implements Operation { protected $op1; protected $op2; public function __construct(Operation $op1, Operation $op2) { $this->op1 = $op1; $this->op2 = $op2; } } class Add extends AbstractOperation { public function execute() { return $this->op1->execute() + $this->op2->execute(); } } echo (new Add(new Operand(1), new Add(new Operand(2), new Add(new Operand(1), new Operand(2)))))->execute(); // 6If you need to add multiplication: class Multiply extends AbstractOperation { public function execute() { return $this->op1->execute() * $this->op2->execute(); } } No. That's not how you locate a memory leak. You have to understand how it works, and how it 'leaks'. Search for 'php find memory leak' and you will find good resources to tools that help you find memory leaking code.
-
I am not sure what it is you expect from us. But, I would use a Dependency Injection container, because: Nothing has to be globally accessible. It's bad practice for a class to be globally accessible. You solve this by using a DI container: $pimple = new Pimple(): $pimple['ini'] = function($c) { // read and return configuration values }; $pimple['db'] = function($c) { $config = $c['ini']; // create database }; $pimple['access'] = function($c) { return new Access($c['db']); }; $pimple['some_module'] = function($c) { return new MyModule($c['access']); }; // ...As you can see from this container I can pass any required information to whichever class that needs it without having to be globally accessible.
-
Create simple things. Read from a text file. Read from a database. Store things in sessions. Change that so that everything you write to a session is stored in the database. Make a form with some input fields and submit it, then add, subtract, divide, or multiply the input and display it on screen. Get your hands dirty. It's the only way you learn how to program.
-
Handling 'Pear Mail to txt' truncated messages
ignace replied to 2outspoken's topic in Other Libraries
On the verizon website it says that name is part of the 140 char limit. So simply limiting your message to 140 chars is insufficient, you need to do: $sender = 'Some Name'; $charsLeft = 140 - strlen($sender);$charsLeft is the maximum length of your message. -
Core is pretty abstract for a class name. And unless you are really into God objects (yes no christians among programmers, we use the name in vain), it would be better if you broke it down further into smaller re-usable components. (see EDIT2 below) Otherwise simply pass the Access class through the constructor, or unless it can operate without it, which I doubt, through a setter. class Core { private $access; public function __construct(Access $control) { $this->access = $control; } protected function getAccess() { return $this->access; } }It's also a good practice not to use public variables on a class since that way you lose all control. Protected should be avoided as well if possible (what public is to the outside 'world' is protected to the child classes). If child classes need it make it available through a getter as shown. Singletons should be avoided as well, it's the same as the global keyword. If a class needs another object to operate, simply pass it through the constructor. EDIT2: For example, the $_Ini could be a Config object that was created by a Factory that could read from different possible sources .ini .xml .json .. Take a look at: https://github.com/zendframework/zf2/tree/master/library/Zend/Config Your hardcoded mysqli inside Core could be passed through the constructor. Though I would advice to use PDO: class Core { public function __construct(PDO $pdo) { $this->driver = $pdo; } }PDO is a data access layer, a database abstraction layer is available at:https://github.com/zendframework/zf2/tree/master/library/Zend/Db The difference is that Zend/Db allows you to write abstract queries: $select = new Select('users'); $select->where(array('username' => 'ignace'));You can use these libraries instead of writing them yourself. If you look around it's quite possible some folks have already done what you want to do and you can simply use their effort and make it your own.
-
Thx Barand. So simple, I really need to quit pulling an all-nighter and head off to bed before I am completely embarrassing myself. EDIT: Awesome, with a few tweaks, running a gazillion permutations (don't need to store all of them just specific ones) on a 128M system
-
Hi, Does someone know of a recursive way to permute $data? Currently I am using iteration and that sucks.. I tried without luck, online examples were only about 1-dimensional array's. $data = [ [1,3,4], [11,15,16], [22,24,25,28], [36,38], [44,48,50], [1,2,3], [9,10,11], ];So that it permutates to: [] = [1,11,22,36,44,1,9] [] = [1,11,22,36,44,1,10] [] = [1,11,22,36,44,1,11] [] = [1,11,22,36,44,2,9] [] = [1,11,22,36,44,2,10] [] = [1,11,22,36,44,2,11] [] = [1,11,22,36,48,1,9] [] = [1,11,22,36,48,1,10] [] = [1,11,22,36,48,1,11] ..
-
Well I am truely sorry that you only have 1 page projects. I was under the assumption you did real projects. My bad, in that case you are completely right. Carry on.
-
By adding more time writing/figuring it (out) yourself instead of using available libraries? That's what I am gonna say to my boss for the next project. Oh no, we don't use frameworks they add too much crap that we don't need. Instead I am gonna write my own and add another 3 months of programming and 6 months of debugging to the project. He's gonna LOVE it!
-
You may want to learn JavaScript first before continuing on your journey. You will safe yourself hours of frustration. You don't simply burst into China and then hope to learn their language. You learn chinese first and then you go to China. <!DOCTYPE html> <html> <head> <title>Test Music App/title> </head> <body> <select name="Songs" height="150" onchange="playsong(this.options[this.selectedIndex].value)"> <option>Nicki Minaj - Five-O</option> <option>text2</option> <option>text3</option> <option>text4</option> <option>text5</option> </select> <script> function playsong($song) { alert('Playing ' . $song); var container = document.createElement('div'); container.innerHTML = '<audio controls>\ <source src="http://www.inzernettechnologies.com/StreamIT/media/music/'+$song+'.mp3" type="audio/mpeg">\ <embed height="150" width="400" src="http://www.inzernettechnologies.com/StreamIT/media/music/'+$song+'.mp3">\ </audio>'; document.body.appendChild(container); } </script> </body> </html>
-
A typical case of Not Invented Here Syndrome
-
Doctrine comes in 3 packages. You only need the DBAL package. Since you already use Composer, installing doctrine is easy: $ php composer.phar require doctrine/dbalSetting up is as easy as: http://docs.doctrine-project.org/projects/doctrine-dbal/en/latest/reference/configuration.html
-
Best way to tackle conditional methods oop?
ignace replied to RuleBritannia's topic in PHP Coding Help
I don't understand why everyone seeking help always ends up giving canned examples. A canned example is a canned answer. Tells us what you are doing, and why you want to do it this way. -
OOP is more then simply introducing one class into your project. Or a few classes for that matter. So if you want to use a class for it or go with a function, the choice is ultimately yours. You can avoid having to create the handle over and over by passing the handle to the functions that require them: function login($ch, $other, $parameters) { // code }Personally I would prefer a class to signify the cohesion between the functions and not having to pass the same data around between functions, in a class they would simply share the information. But don't take my word for it though, because I am biased.
-
Even if you would be able to see how an expert builds a website. You would probably learn nothing, and/or consider yourself really dumb because you don't understand half of what he wrote. The same way you wouldn't learn chinese if you see someone write it. What you need is the mindset of an expert and that is to understand how he solves problems. A project usually has 2 categories. 1) The part you know how to do or can easily google to find out how to do them; 2) The part you have no clue whatsoever how to do; Number 2 is the interesting part. And before anything else you should take into account their importance to the project. So if you have no clue how to do something but the importance of it is optional then you won't invest any time in it at all. And the problem is solved. If however it's critical to the project then it's your primary focus, and solving this before anything else is paramount to the success of the project. Avoiding the complex parts of an application until the end usually means project failure. Furthermore the expert identifies the different components of the project on a high-level, which is the same as categorizing cohesive (=related) portions of the project. For a blog you could identify: Authorization (Access Control) Authentication Blog Post Authoring & Publishing Among others, from this high-level view of your project, you would drill down further categorizing. The advantage here is that it's easier to focus, as the scope is smaller, and after the project has been going for a while you will have a neat whiteboard as a top-down view with all these components and the links between them making it easier to see the big picture and add/factor-in new functionality. And I think that after you reading this I have proved my first point.
-
objnoob is on to the right idea. function setSettingsForBrand($brand) { switch ($brand) { case 'intel': setIntelSettings(); break; default: setDefaultSettings(); break; } }So in the case of where you have a brand with no corresponding function, or misspelled the brand name, your application wouldn't error out but instead simply apply the default. Magic is cool, but not in your code. Because in the end it will pull it's greatest trick on you.