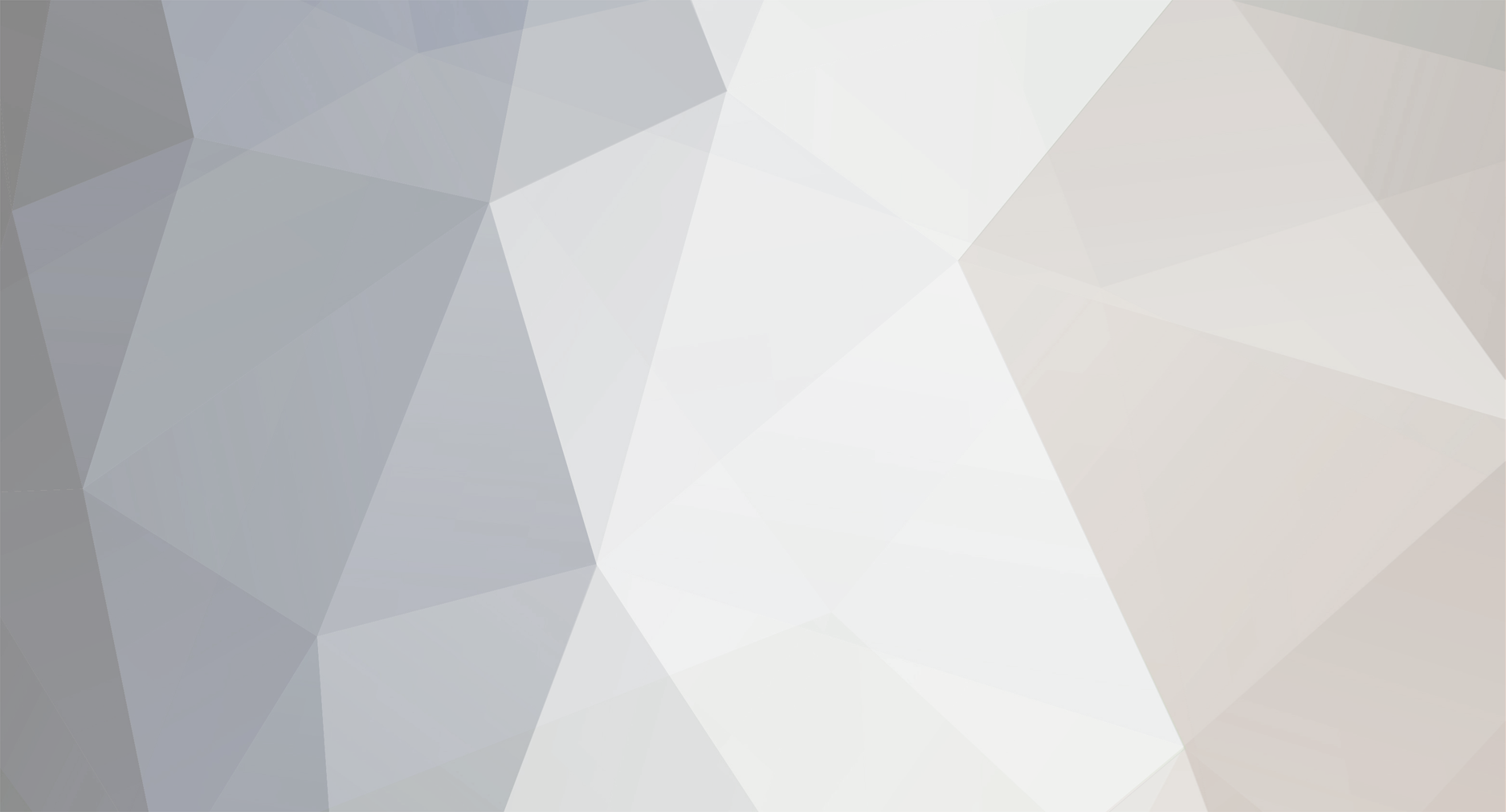
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
All jokes aside. What I was referring to is: http://www.phptherightway.com/#databases_abstraction_layers More specifically: https://github.com/auraphp/Aura.Sql It provides you with a simplified interface (Facade) to work with PDO, hiding it's 'complex' parts which might be a good starting point. Dutch: Van waar uit vlaanderen ben je? ik ben van vlaams-brabant.
-
It has: http://wulijun.github.io/php-the-right-way/
-
You are correct. The correct way of doing it is by 1) using doctrine to do your table to object mapping: http://docs.doctrine-project.org/en/2.0.x/reference/xml-mapping.html#example 2) or (and this is the lesser option) writing your own mapper: http://www.sitepoint.com/integrating-the-data-mappers/#highlighter_536159 I would not follow adam_bray's advice, because 1) it couples your entity to the database 2) it introduces globals (you can no longer control who has access to the database, even your View can start executing queries) 3) it uses a singleton, which is an anti-pattern 4) in those rare cases you need to work with more then 1 database connection you have a problem. -- Keeping your entities decoupled from the database means that they will have more cohesive methods and less clutter (db specific methods like find*() and save() or something).
-
That is because the course you are following is outdated. A good place to start is this: http://www.phptherightway.com/
-
.html is not parsed by PHP. You have to name your file with a .php for it to work.
-
Why do so Many Beginners Use a lot of Bad Practices?
ignace replied to mogosselin's topic in Miscellaneous
Psycho nailed it. -
<?php print "Hello world, I need a good brain!"; ?>
ignace replied to phpallwinner's topic in Introductions
Obviously the introductions forum should not be used to ask for help. To answer your question. Learn HTML, PHP and XPath (or Regex) to query the DOM of the returned HTML from Google, Amazon, and e-bay. Learn CSS optionally to make your website and the search results look nice.- 4 replies
-
- php search
- php search engine
-
(and 1 more)
Tagged with:
-
Now that we are talking about Symfony. Symfony has it's own CMS of some sort called CMF (if you click on one of the slides, use the arrow keys). It incorporates the ideas outlined in Decoupling Content Management. One of the key blocking factors that I've seen with other developers adapting to Symfony is Doctrine and the fact that they still think in terms of database tables and columns. Anyone having done some OOP and written some mapping classes can tell you all about the Object-Relational Impedance Mismatch and the problems associated with it. Thus as to better yourself in OOP you need to remove the idea of a database and think in objects, how they will interact and what messages (the type of data they will return) they will send. You know you have a good OOP model when you have to apply the techniques outlined here. People change and so do requirements. A field you did not have to search on at first is suddenly critical for sprint#n. Thus always keep in mind that change is inevitable and your OOP model will change. Keep everything therefor as simple as possible as to make these changes easy and refactor code pieces that are slowly building a complexity momentum.
-
Everything Jacques1 said is spot on. @OP What I see from your classes is that you think far too narrow for OOP. You create one class to solve multiple needs at once. Instead you should figure out who the actors are on the stage, define them and then use composition to solve your need.
-
What possien proposes is not OOP but procedural programming masked as 'OOP' by abusing the class keyword. Since you will be working with images you will have an object Image that you want to support an operation resize(). Since resizing an Image constitutes a new image, your code should act accordingly: class Image .. { public function resize(..) { return new Image(..); } }Now for the actual resizing of the Image we can identify several methods: 1) A fixed width/height combo 2) Only a width to widen the image 3) Only a height to heighten the image size 4) Increase the image size by a multiplier 5) A ratio for scaling Obviously we will be foolish to force all of this on one method, and we will probably require this on other occassions. A new object is born. class Box { public function __construct($width, $height) {} public function widen(..) {} public function heighten(..) {} public function increase(..) {} public function scale(..) {} }Now add this to our Image::resize() method: class Image .. { public function resize(Box $box) { // do the image resizing using $box->getWidth(), $box->getHeight() return new Image(..); // done! } }The API in action: //client code $image = new Image(..); $thumb = $image->resize($image->getSize()->scale(0.5)); header(..); print $thumb;Obviously there is more to OOP then I can tell you in one post. It is also for example possible that one of your classes support an operation that is too big for one class to handle or is not part of it's responsibility (like the above resizing methods). In this case you create multiple classes yet these classes are not known to the 'client' (your controllers that access the public interface of your class) and are never exposed. Which improves maintainability and re-usability. For example you could have a TwitterGateway class that reads your twitter feeds. To do this it requires an OAuth handle. The OAuth handle is a separate class that is never exposed through the public interface of the TwitterGateway class to your client (ie getOauthHandle()), yet it uses this to achieve it's goal (read your feed). The OAuth handle class can be used by other classes though, to access oauth protected resources, thus the OAuth handle class provides re-usable behavior not specific to Twitter. If you still have questions, just ask them.
-
Is it worth having a DB class? Yes. Pdo is such an existing class which brings you the advantage of being able to switch between vendors (mysql, postgre, sqlite, ..). Is it worth writing this yourself? No, unless for learning purposes, it's better to use an existing solution written by people who know their stuff. Most libraries simply extend PDO with their own functionality like Doctrine's DBAL layer. What you probably are looking for is a way to centralise your queries, so instead of having these spread out over your entire application, possibly even duplicating them, you would write a few cohesive classes that will hold your queries allowing you to change these in one place. abstract class AbstractRepository { protected $pdo; public function __construct(PDO $pdo) { $this->pdo = $pdo; } } class UserRepository extends AbstractRepository { // all queries executed by this class at the top for quick editing private static $QUERY_RECENT_REGISTERED_USERS = 'SELECT .. FROM users WHERE registered_at >= ?'; public function getRecentRegisteredUsers($interval = '-5 hours', $timezone = null) { $datetime = $timezone ? new DateTime($interval, $timezone) : new DateTime($interval); $stmt = $this->pdo->prepare(self::$QUERY_RECENT_REGISTERED_USERS); // execute statement, get users return $users; } }
-
Well you could achieve that using the ExpressionLanguage component and short-circuit logic: (product.makeEquals('Ford') and seller.idEquals(12345)) and product.changeCategory('Truck')Or using ternary operators: (product.makeEquals('Google') and product.modelEquals('Glass')) ? product.removeColor()But this assumes your use-case won't get complexer than this and someone with some programming background is around to write these rules. Not to mention that if there is someone with a programming background around he could aswell simply write it in PHP. You don't want your business logic in your database.
- 6 replies
-
- inference engine
- rules engine
-
(and 3 more)
Tagged with:
-
Can you give an example of said rules? And how will these rules be defined? Can a programmer intervene to program said rules and does the end-user merely be able to 'chain' these rules? If this is true then the answer is the Specification Pattern: http://en.wikipedia.org/wiki/Specification_pattern Or something like: http://symfony.com/doc/current/components/expression_language/syntax.html
- 6 replies
-
- inference engine
- rules engine
-
(and 3 more)
Tagged with:
-
I would like to see a forum for that as well. Consider this my upvote
-
Nyan cats seem to be all the rage.
-
Radius of Latitude and Longitude Logic & Maths Scope - Postcodes
ignace replied to Ansego's topic in PHP Coding Help
HAVING should be WHERE -
Trying to convert my existing code to an OOP framework.
ignace replied to p2bc's topic in PHP Coding Help
Why reinvent the wheel? Pdo comes installed with PHP and supports a various set of vendors (mysql, postgresql, mssql, oracle, ..). Preferably you use a true database abstraction layer like Doctrine to make your code portable between the different db vendors. -
It is great that you teach other people though I imagine the teaching may go much better if the one teaching actually knows something.
-
What question? You still haven't asked a question. The only question marks you posted are on the start and end php tags.
-
A search engine can't read a video. The description helps users to find video's that are related to their search query.
-
Augury if you are not going to ask a question, I am going to lock this thread. And then you and your thread can have the intimate experience you both crave.
-
1) You are declaring a class Shape but you are not using it? 2) You have one class Shape to represent all your shapes shows you haven't been paying attention in math 101 because assuming that for the squares and rectangle you have a method getSides() then what do you return for a circle? Similarly what do you return for getRadius() on a square? You already have the form you simply have to add some validation and add the proper classes. For File I/O you can create a simple File object. Take a look here for the functions required to perform file operations: http://php.net/manual/en/book.filesystem.php I have no idea what is meant with collection of shapes perhaps a simple array? $shapes = array( new Circle($radius), new Square(), new Rectangle(), );But as since these have no common ancestors it's hard to iterate over them and execute something meaningful on them.
-
How about the showshapes.php file?
-
Post your code here.
-
$current_page = isset($_GET['page']) ? intval($_GET['page']) : 1; $items_per_page = 20; $offset = ($current_page - 1) * $items_per_page; $items = glob("entries/*.php"); $total_items = count($items); $total_pages = ceil($total_items / $items_per_page); foreach (array_slice($items, $offset, $items_per_page) as $entry) { include $entry; }