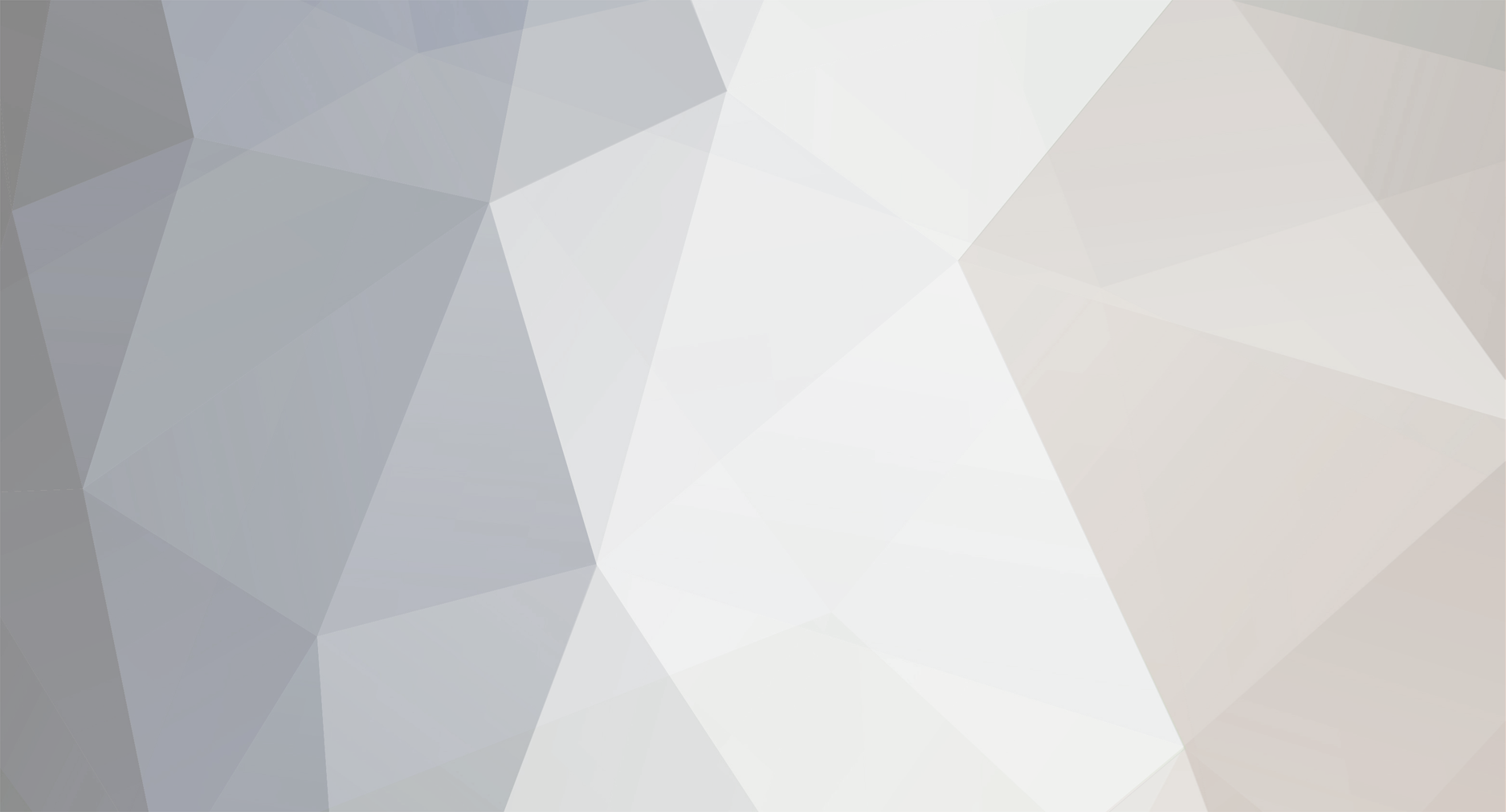
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
http://us2.php.net/manual/en/reserved.variables.files.php http://us2.php.net/manual/en/control-structures.if.php You are welcome!
-
You need a framework BECAUSE you are the only developer in the company. This will make it easy for the company to find someone after you leave or to help you develop it. It's easier to put out a job ad "Knows framework X" then to put a general job ad saying "Knows PHP and willing to learn in-house framework that you will NEVER EVER use again in the rest of your career and is thus of zero-use to you but our business depends on it." Don't be lazy, and don't take shortcuts, learn a framework, I highly suggest symfony as that means half of your application will be written by simply installing a few bundles from KnpLabs and FOS. So, you have all the time you need to learn symfony.
-
LOGICAL OPERATION FOR PHP WITH MYSQL (INVENTORY SYSTEM)
ignace replied to Edmhar's topic in PHP Coding Help
$quantity = 15; $order_quantity = 5; echo $quantity - $order_quantity; -
You would need to write the script it calls as webhook to download the zip..
-
At work we use Beanstalk instead of Github. Beanstalk allows you to deploy over FTP when you push new changes to the master branch. You can't use the above library as it assumes you have control over the server, which you don't. So the only way you'll be able to auto-deploy is by writing it yourself and running it from your own computer. That could look something like this (untested): if (file_exists('.revision')) { $deployed_commit = file_get_contents('.revision'); exec('git diff --name-only ' . $deployed_commit . ' HEAD', $output); // $output contains all changed files since $deployed_commit } else { // from scratch, upload all files } exec('git rev-parse HEAD', $output); file_put_contents('.revision', $output[0]);
-
if (isset($_POST['submitbtn'])) { $hasBlank = false; foreach (range(0, as $i) { $val = array_key_exists('box' . $i, $_POST) ? $_POST['box' . $i] : ''; if (empty($val) && $hasBlank === false) $hasBlank = true; $box[] = $val; } function check($box, $x) { // [ 0, 1, 2 ] // [ 3, 4, 5 ] // [ 6, 7, 8 ] // horizontal return ($box[0] == $x && $box[1] == $x && $box[2] == $x) || ($box[3] == $x && $box[4] == $x && $box[5] == $x) || ($box[6] == $x && $box[7] == $x && $box[8] == $x) // vertical || ($box[0] == $x && $box[3] == $x && $box[6] == $x) || ($box[1] == $x && $box[4] == $x && $box[7] == $x) || ($box[2] == $x && $box[5] == $x && $box[8] == $x) // diagonal || ($box[0] == $x && $box[4] == $x && $box[8] == $x) || ($box[2] == $x && $box[4] == $x && $box[6] == $x); } function doComputerTurn(&$box, $x) { $empties = array_keys($box, ''); if (!$empties) return; $key = array_rand($empties); $box[$empties[$key]] = $x; } if ($hasBlank) { echo 'keep playing!'; } elseif (check($box, 'o') && check($box, 'x')) { echo 'draw!'; } elseif (check($box, 'x')) { echo 'x wins!'; } elseif (check($box, 'o')) { echo 'o wins!'; } }
-
// the contents from the file $input = 'a=1,b=2,c=3,d=4'; // the expression $expression = 'a + b - c * d'; // put all variables into $variables parse_str(str_replace(',', '&', $input), $variables); foreach ($variables as $variable => $value) { // replace the variables in $expression with their values $expression = str_replace($variable, $value, $expression); } // assuming this is from a trusted source meaning you or your teacher. eval('?><?php echo ' . $expression . ';');
-
Euhm.. no. I remember shit, I got an IDE that does the remembering for me PHP, Perl, Python, VB.NET, Java, C, C++, Assembler, .. right.. try to remember that!
-
I was wrong, I assumed PHP would change the status to 404 when an error occurs but it doesn't. It simply returns a 202, so did you check all of those one by one? The reason you have this problem is that your front controller is a catch-all, meaning that everything it can't find is loaded through it. For example remove an image from your webroot and now this will hit your front controller, trying to call image.png on controller images, unless you explicitly said it should not run images through it. This is also one of the drawbacks of using a /controller-here/action-here setup, you can avoid this by decoupling your controller/actions from the actual URL. $route = new Route('/hello/world', array('_controller' => '/Path/to/ControllerFoo', '_action' => 'someAction'));Now when you got to /hello/world it will match the route and call the appropriate controller while every call that isn't a route will simply be greeted with a 404.
-
untested class RecursiveArrayWrapper extends \ArrayObject { public function offsetGet($offset) { $data = parent::offsetGet($offset); if (is_array($data)) { return new static($data, $this->getFlags(), $this->getIteratorClass()); } return $data; } // add functionality as needed public function merge($array) { if ($array instanceof self) { $array = $array->getArrayCopy(); } $this->exchangeArray(array_merge($this->getArrayCopy(), $array)); return $this; } } $array = new RecursiveArrayWrapper(array( 'foo' => array('bar' => array('bat' => 'baz')) ), ArrayObject::ARRAY_AS_PROPS); var_dump($array->foo->bar->bat); var_dump($array->foo->merge(array('bar' => array('bat' => 'foo')))->bar->bat);Does exactly what you want but instead of calling it something as meaningless as superGlobal, I called it something so that it can be re-used for something more then just as a superGlobal container (which it really wasn't either). This is kinda more what I would call it: class HttpRequest { public $get; public $post; public $cookie; public $session; public $server; public $files; public $env; public $globals; public function __construct() { foreach (array('get', 'post', 'cookie', 'session', 'server', 'files', 'env', 'globals') as $superGlobal) { if (isset(${'_' . strtoupper($superGlobal)})) { $this->$superGlobal = new RecursiveArrayWrapper(${'_' . strtoupper($superGlobal)}, ArrayObject::ARRAY_AS_PROPS); } } } } $request = new HttpRequest; var_dump($request->get->foo);
-
In your browser press F12 to get the developer console, go to Network tab, reload the page. Sort by Status column and find the one that says 404, that's the one that is causing the < in your error log.
-
No, it won't. You can't access cookies from another domain. You can however use AJAX to an external source or include an external *.js file through <script/> to get the user's login info. The other domain however will have to allow you to make these calls using Access-Control-Allow-Origin.
-
Cool.
-
You need to lex your templates as seen here: https://github.com/fabpot/Twig/blob/master/lib/Twig/Lexer.php Once your template is lexed, you have a token stream (to see how that might look like see token_get_all), this token stream can then be used to validate your templates as well as to start parsing your template. A good book about lexing/parsing see http://www.amazon.com/Language-Implementation-Patterns-Domain-Specific-Programming/dp/193435645X
-
What to do when your code gets messy and confusing?
ignace replied to FrogWarrior's topic in Application Design
Hall of Famer is this you? When the project is small, it does not matter which pattern you use, or if you use patterns at all (and I am not saying you should), since the codebase is small and change is easy. And no matter the size of the project, change is a constant in every project. So you won't get it right the first time, therefor it is best you simply build the simplest thing that could possibly work as to not make it harder on yourself when you got it wrong, and you will. Iteratively refactor your code as your domain knowledge of the project evolves. -
How do you send a bad email, and how do you get the info back.
ignace replied to njdubois's topic in Applications
Or use a service like Mandrill to send your e-mails. That way you can see exactly what happened to each e-mail you send. -
function getPeaks($array) { if (($count = count($array)) < 3 ) { // not enough neighbours return false; } $peaks = array(); // $i starts at the second element, $max points to the last element that still has a neighbour // array( // 0 => .. // 1 => .. <-- $i // 2 => .. <-- $max has neighbours 1 and 3 // 3 => .. // ) for ($i = 1, $max = $count - 2; $i <= $max; $i++) { if ($array[$i - 1] < $array[$i] && $array[$i + 1] < $array[$i]) { $peaks[] = $i; // both neighbours of $i are smaller } } return $peaks; } function getFlags($array) { // number of peaks is the required distance between flags $distance = $count = count($array); if ($count < 2) { return false; } // flag positions, add first array element $positions = array($array[0]); // $i is current element position // $j is last flag position to compare against for ($i = 1, $j = 0, $max = $count; $i < $max; $i++) { if (abs($array[$j] - $array[$i]) >= $distance) { $positions[] = $array[$i]; $j = $i; // move position to new flag position } } return $positions; } header('Content-Type: text/plain'); $peaks = getPeaks(array(1,5,3,4,3,4,1,2,3,4,6,2)); echo 'Peaks:', PHP_EOL; var_dump($peaks); echo 'Flag positions:', PHP_EOL; var_dump(getFlags($peaks));
-
if( $this->real_escape_string_exists) { // PHP v4.3.0 or higherCracks me up when I see this and they use public function. Public, private, protected is available only in PHP5, so stop doing checks for things that were added in PHP4.
-
// what would be the absolute minimum you would charge for this project? $minimum = ..; // what would be the absolute maximum you would charge for this project? $maximum = ..; $range = range($minimum, $maximum); echo 'This projects total costs: ', $range[array_rand($range)];Adding some randomness to your project makes for on-time and within-budget projects. You'll also feel much better because you won't feel like your the one ripping your client off as you did not set the price.
-
no question.
-
They even disable error reporting and displaying in your honor! XD
-
Indeed. Just what we need another Texan! Why not change the name from phpfreaks to phptexans?! XD Just kidding! Congrats! I voted!
-
You can also just: $ curl http://www.your-domain.top/The only way to block people from viewing your source is to do this: <?php exit;At the start of every script. This effectively blocks anyone from viewing source or anything else for that matter. Another option to block people from viewing source is to change your DNS entry: your-domain.top A 88.88.88.88You can change the IP to whatever you like, it really does not matter. It may take a few hours though before they are no longer able to view your source. Though it is more effective then the previous described technique. The third option saves you money to stop people from viewing your source. Simply stop paying your hosting company. And you'll see that after some time, they won't be able to view source. Cost effective, but slow.
-
uhm.. right. A few pointers: 1) Always write <?php not just <?. The short notation may be turned off and then your code is displayed in the browser. 2) Always properly indent your code. 3) Separate HTML and PHP. <?php // PHP processing here // include the HTML include 'posts.view.php'; Inside posts.view.php you would write something like and use PHP's alternative syntax:http://php.net/manual/en/control-structures.alternative-syntax.php <?php foreach ($posts as $post): ?> <div class="post <?php alternate('class1', 'class2'); ?>"> .. $post here .. </div> <?php endforeach; // notice this tag here ?>which makes for maintainable code.
-
They stood it up for adoption, that's how much they like it themselves.