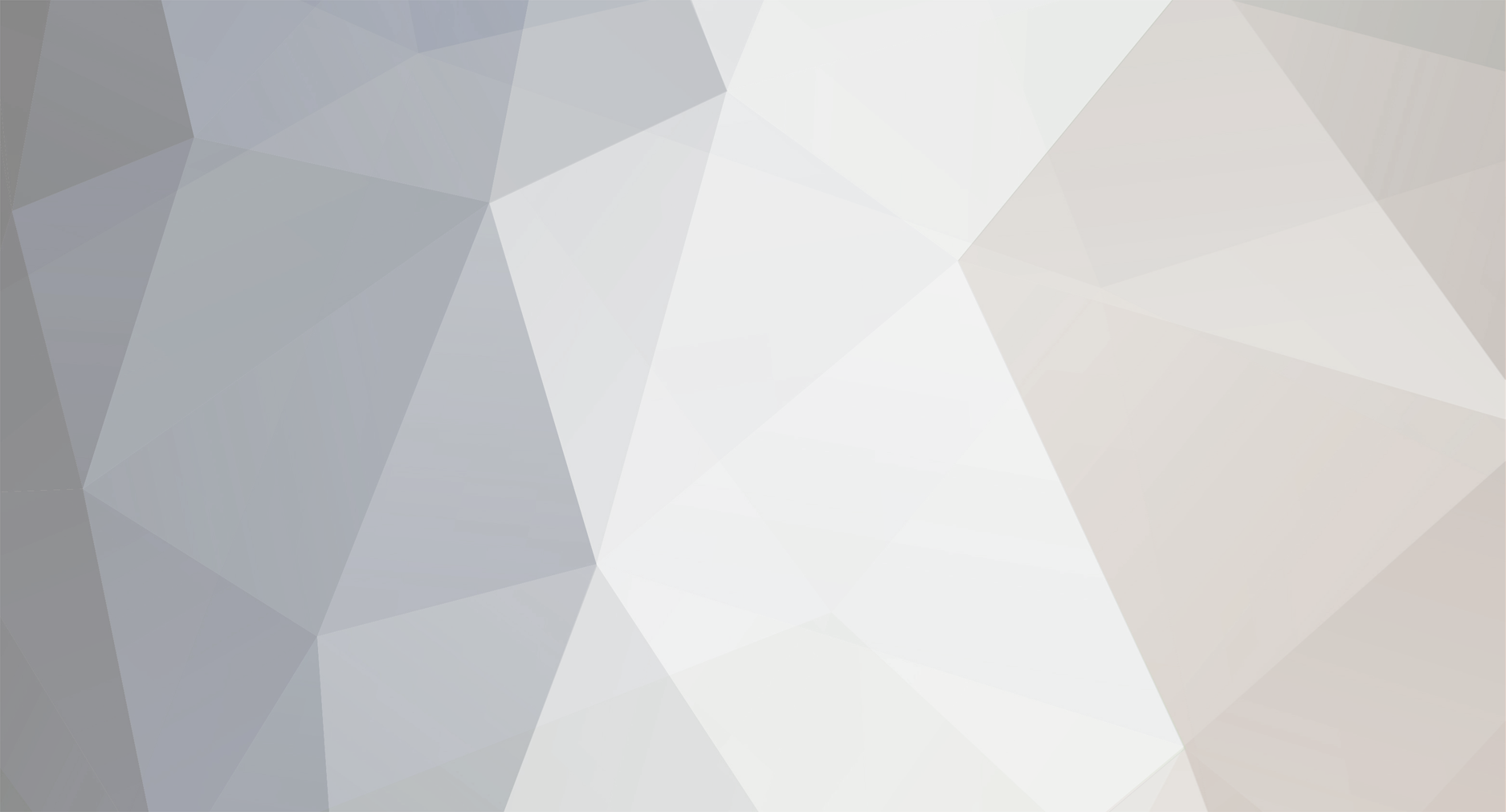
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Instead of storing ID's in constants, maybe you can use OOP to accomplish what you are doing: class PageRepository { private static $QUERY_HOME_PAGE = 'SELECT .. FROM pages WHERE page_id = 1'; private static $QUERY_CONTACT_PAGE = 'SELECT ..'; private $pdo; private $pageFactory; public function __construct(PDO $db, PageFactory $pageFactory) { $this->pdo = $db; $this->pageFactory = $pageFactory; } public function getHomePage() { $stmt = $this->pdo->query($this->QUERY_HOMEPAGE); // return $this->pageFactory->createFromArray($data); } public function getContactPage() { return $this->pageFactory->createFromArray(..); } }
-
Since people are using the native app it may be an opportunity for you to contact the creator of the app and come to a business arrangement, where he pays you X% of the profits. Be reasonable, he did create a native app for your website. If not, you have it taken down through the app store. Though the first option is better. Not really sure what you can do as a defense, assuming the user enters his credentials into the app, which then performs a sub-request on your website and stores the cookie locally and sends them with every request there after making anti-CSRF useless. In essence the app acts as a browser, so you need to figure out how to filter out the app using only the request headers, though I assume it will only be a matter of time before these are spoofed. Also keep in mind that any counter measures you may take may result in further visitor loss which is why I really advise you to take the first option.
-
Does this Hostgator Technician Know What He's Talking About?
ignace replied to Fluoresce's topic in MySQL Help
Also if your pages always generate the same darn page, then cache it and avoid the DB connection completely. You create a unique cache key for each page and check if it exists, then stop and echo it, or continue and create the cache. Something like this: <?php $cacheKey = 'video_' . intval($_GET['id']); if (file_exists('cache/' . $cacheKey)) { include('cache/' . $cacheKey); exit; } // query DB $html = include('some/view.html'); file_put_contents('cache/' . $cacheKey, $html); echo $html; -
Does this Hostgator Technician Know What He's Talking About?
ignace replied to Fluoresce's topic in MySQL Help
It is possible that Google, Yahoo, Bing and the likes are crawling your website. They are known to open multiple connections to crawl your website faster, but it is possible that by this they are actually hitting the limit. If you have Google Analytics installed on your website, it should be possible to detect who is at your website doing what at the time this occurs. In the case of crawlers you can tell them (atleast the legal ones) to only use 1 connection, not multiple. -
class Horse { public function __construct(DateTime $lastFedAt) { $this->lastFedAt = $lastFedAt; } public function feed() { $this->lastFedAt = new DateTime(); } public function isHungry() { if (date('H') < 6 || date('H') > 22) { return false; // sleeping } return time() - $this->lastFedAt->getTimestamp() > 4 * 3600; // feed every 4 hours } } class HorseFeeder { public function check(Horse $horse) { $horse->isHungry() && $horse->feed(); } public function checkLoop(Horse $horse) { while (true) { $this->check($horse); sleep(600); } }
-
Keeping it Separately with Object Oriented Programming
ignace replied to glassfish's topic in PHP Coding Help
It could look like this: public function uploadAction(Request $request) { $uploadService = $this->get('upload_service'); $form = $uploadService->getForm(); if ($uploadService->handle($form, $request)) { $request->getSession()->getFlashBag()->add('success', 'Image uploaded successfully!'); return $this->redirect($this->generateUrl('foo_bar')); } return array(); }Yes, this is the same script as what you wrote up there. And this is typical for OO programming. It hides ALL the details. The UploadService would do something like this: public function handle(FormInterface $form, Request $request) { $form->handleRequest($request); if (!$form->isValid()) { return false; } foreach ($form->get('upload')->getData() as $uploadedFile) { $file = $uploadedFile->move($this->targetDirectory); $upload = new Upload($uploadedFile->getClientOriginalName(), $file->getMimeType(), $file->getSize()); $this->em->persist($upload); } $this->em->flush(); return true; }This is an example using Symfony framework. -
@Ionut-Bajescu thank you for your very insightful advice. Take a look at composer, it allows you to create packages that you can "require" in new projects. The Symfony framework uses independent bundles that can be distributed and installed through configuration. FOSUserBundle is such an example.
-
Requesting suggestion about my programming future
ignace replied to Digitizer's topic in Miscellaneous
You should have said to him: "So, your classes are empty." The truth is that the polishing only happens at the workplace not in uni. I have had graduates who I had to unlearn things and teach them the proper way of doing it. -
Requesting suggestion about my programming future
ignace replied to Digitizer's topic in Miscellaneous
It depends on what you want to do. If you want to make websites and online applications, PHP is a good choice as it's easy to learn. If you wanna become a mobile developer, you want to learn Java or Swift. If you want to make games you need to learn C++ or Lua. So, it all depends on what you want to become. -
The second class should not extend the first class. Because then the second class can build itself thus making the first class obsolete. Also it wouldn't need the first class either to set errors, it can simply set it on itself to set it on the first class.
-
Template method pattern and process interruption
ignace replied to Rike's topic in Application Design
Post your code. -
When assigning responsibilities you should keep in mind that Crate is the Information Expert: http://en.wikipedia.org/wiki/GRASP_(object-oriented_design)#Information_Expert SRP is about the big picture, class cohesion, not about trimming all classes until they only have 1 method.
-
Why do you require a factory to get basic properties from a Crate object? Shouldn't it be: $crate->getDimensions(); $crate->getWeight();Whatever that $options variable hold should be modelled into your Special Case Crate object.
-
Making a program to automatically install PHP frameworks
ignace replied to CrimpJiggler's topic in Miscellaneous
composer also supports git clone (or if git is not installed on the server, can download's the dist zip): https://getcomposer.org/doc/04-schema.md#repositories -
Static Methods vs. Singleton vs. Dependency/constructor Injection
ignace replied to adam_bray's topic in PHP Coding Help
None of your examples includes a DI. Here's a rewrite of your index.php with DI: $di = include 'includes/di.php'; $di['content']->loadPage($_GET['page']);And your article_index.php $di = include 'includes/di.php'; $di['articles']->getArticles(0, 15);The includes/di.php could look something like: // download from http://pimple.sensiolabs.org/ $container = new Pimple(); $container['config_filepath'] = 'config/config.ini'; $container['config'] = function($c) { return new Config($c['config_filepath']); }; $container['db'] = function($c) { return new Database($c['config']); }; $container['content'] = function($c) { return new Content($c['db']); }; $container['articles'] = function($c) { return new Articles_Model($c['db']); }; return $container;For all your autoloading you may want to consider composer -
CodeIgniter is dead. And security is only as good as the programmer who wrote it. It's like the lock on your front door, it's nice to have it, useless if you never actually lock your door. From your description I am guessing they did not hack your website through software problems, but through FTP (since the JS is gone off the pages, unless you built a removeJsFromViews() function). So your FTP access is likely compromised, you might wanna change your password, or if using shared hosting, another account on the server has been able to access your account. In that case, you'll need the provider to dig through his log files.
-
Doctrine - Configure Entities in more than one directory
ignace replied to LeoFelipe's topic in Other Libraries
That is really hard, your mind may be blown. $dirs = array(__DIR__ . "/src", __DIR__ . "/src2"); $config = Setup::createAnnotationMetadataConfiguration($dirs, $isDevMode); To make it easier to consume: $config = Setup::createAnnotationMetadataConfiguration(array(__DIR__ . "/src", __DIR__ . "/src2"), $isDevMode);Now for the complete meltdown: $config = Setup::createConfiguration(); $chain = new MetadataChain(); foreach (array('xml' => __DIR__ . "/src", 'yml' => __DIR__ . "/src2") as $driverName => $path) { switch ($driverName) { case 'xml': $driver = new SimplifiedXmlMetadataDriver($path); case 'yml': $driver = new SimplifiedYamlMetadataDriver($path); } $chain->addDriver($driver); } $config->setMetadataDriver($chain); ..EDIT: do not copy paste, it won't work, you'll need to do your own research. -
How to struture class when products are different?
ignace replied to dennis-fedco's topic in PHP Coding Help
maxxd has the right idea, but i would keep it simple like this: abstract class Auto { abstract public function getWheelBase(); } class Mustang2013 extends Auto { public function getWheelBase() { return new WheelBase(17, ; } }Other option is to pass specific info to the class: class Auto { private $wheelBase; public function __construct(WheelBase $wheelBase, ..) { .. } } -
How does one become great at php and mysql?
ignace replied to lexijensjacejack's topic in Miscellaneous
You can find some good books in this thread: http://forums.phpfreaks.com/topic/2307-good-programming-and-web-design-books/?p=11836 You might also want to read: http://www.phptherightway.com/ -
Post a username and password interested can use to test your game.
-
How to struture class when products are different?
ignace replied to dennis-fedco's topic in PHP Coding Help
I've seen your questions pass on these forums and they are always very vague and very abstract. So at best you get a very vague and very abstract answer for which the chances of it actually solving your design problems are less than likely. But anyway here goes: How about: public function someMethodFromSomeGhostClass { $this->model->doSomeMethodFromSomeClassInsideGhostClassAndCrossFingers(); } -
You can learn HTML here: https://developer.mozilla.org/en-US/learn/html You can learn CSS here: https://developer.mozilla.org/en-US/learn/css You can learn JavaScript here: https://developer.mozilla.org/en-US/learn/javascript You can learn PHP here: http://www.codecademy.com/tracks/php http://www.phptherightway.com/ (continued) Start off with HTML and CSS, then continue onwards to JavaScript, try out jQuery. Then finally learn PHP.
-
What would be the significance of displaying your ring finger. I think you meant it to be the middle finger, in which case, it would be id = 2. "There are 10 types of people in the world. Those who understand binary and those who don't" Psycho, you are making it too hard on him. Now he has to actually engage his brain. I sense an overuse of Tylenol is coming up. Also he does not realize he actually selected all fingers from his 3rd hand...
-
Why do you keep persisting that he should? 1) It breaks class cohesion. 2) It adds an unnecessary dependency. Team is not a database. There is no relation between Team and the database at all. ---- @OP Your Team class should have setters to set the data from the database onto your object. namespace My\Model\Mapper { class TeamMapper { private $db; public function find($id) { $this->db->query("SELECT team_name, nickname, founded FROM club WHERE team_id=:teamid"); $this->db->bind(':teamid', $id); if ($row = $this->db->single()) { $team = new Team; $team->setId($id); $team->setName($row['name']); $team->setFoundedDate(new \DateTime($row['founded'])); $this->db->query("SELECT result FROM results WHERE team_id=:teamid"); $this->db->bind(':teamid', $id); if ($rows = $this->db->results()) { foreach ($rows as $row) { $team->addScore($row['result']); } } } return $team; } } }