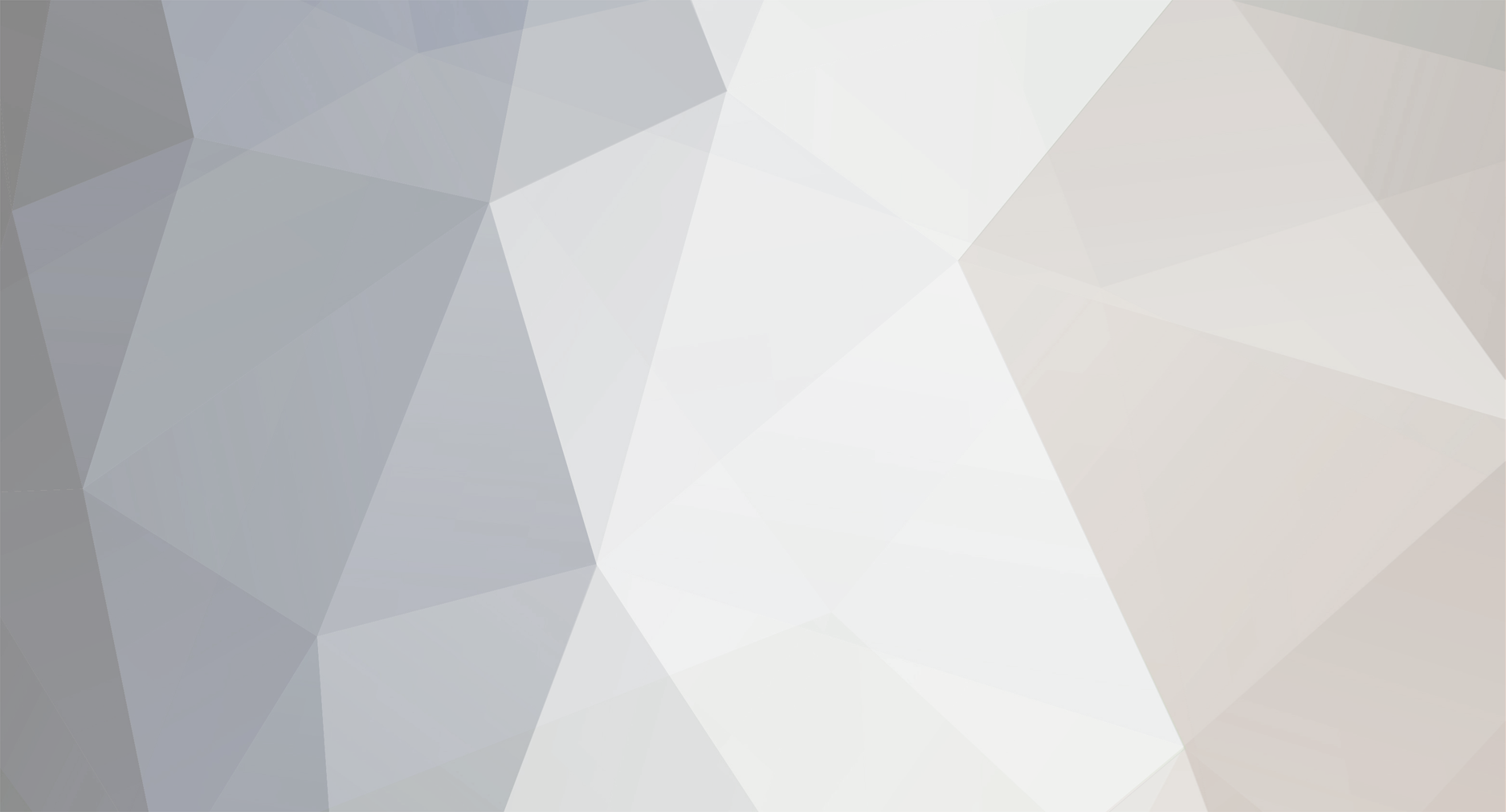
NotionCommotion
Members-
Posts
2,446 -
Joined
-
Last visited
-
Days Won
10
Everything posted by NotionCommotion
-
<?php ini_set('display_errors', 1); error_reporting(E_ALL); echo('sanity check1'); require_once('/path/to/chart.php'); chart($_POST['userinput']); echo('sanity check2'); ?>
-
I am starting to come around and agree that my approach was not correct, and I should pass the object as an argument. That being said, defining a bunch of constants is no better than using a global variable (or some surrogate for a global such as a singleton class), right? Maybe even worse since it is harder to identify all the user defined constants? I suppose I could use get_defined_constants(true)['user'], however. When is it appropriate to use constants throughout an application?
-
Just to confirm, http://yourdomain.com/phil/x.php is the small PHP script you are showing, right? Maybe read/write privileges? What does the following give you? <?php echo ('Current script owner: ' . get_current_user().'</br>'); echo(exec("Rscript /var/www/html/phil/figs/map.R").'</br>'); shell_echo(exec("Rscript /var/www/html/phil/figs/map.R").'</br>'); ?>
-
How to display Print_R array in seperate columns
NotionCommotion replied to jamesreno20's topic in PHP Coding Help
Assuming "ref" in your database corresponds to the checkbox, this line... echo '<td><input type="checkbox" name="check_list[]" value="'. $row[1]. $row[2]. $row[3].$row[4].$row[5].$row[6].$row[7].$row[8].$row[9].'"></td>'; should be... echo '<td><input type="checkbox" name="check_list[]" value="'. $row[0].'"></td>'; That being said, why have the mini-loop to display columns? Typically, you just want the row loop and echo each column independently (at least that is how I do it I also usually use associated or object outputs from the DB so need to use the name). -
body { background-color: black; }
-
Well, yes laziness, but it does make the code more concise. As of yesterday, I have never heard the term "dependency injection, and just learned about the god object now (http://en.wikipedia.org/wiki/God_object). Is tightly coupling always a bad thing? Note that my god object would be only properties, and maybe a method or two to add properties. If I didn't wish to use a third party framework, do you have general recommendations on how to implement a "configurable dependency injection container"?
-
Forget about the part about DB connections, and back to the original question. I have a homegrown MVC architecture, and I have an object with a bunch of properties which are used by the controllers and models. I would rather not pass each given property on a need to use basis, but just pass the whole object. Instead of using a global variable or a singleton object, should I be doing something like... <?php $bigObject=new bigObject(); $bigObject->bla="blabla"; $controller=new someController($bigObject); $controller->someTask(); class controller { protected $bigObject; public function __construct($bigObject) { $this->bigObject=$bigObject; } } class someController extends controller { public function someTask() { $model=new someModel($this->bigObject); $data=$model->getSomeData(); //Deal with view } } class model { protected $bigObject; public function __construct($bigObject) { $this->bigObject=$bigObject; } } class someModel extends model { public function getSomeData() { return array(1,2,3); } } ?>
-
MYSQL Field with PHP variable in it.
NotionCommotion replied to billhobbs's topic in PHP Coding Help
You "could" parse your CSS file through PHP. That being said, you shouldn't as it ultimately leads to a troubleshooting nightmare. One option is just to store a CSS class name in your DB, and have PHP write that class when you are rendering your HTML. You of course would need CSS to assign the proper color to the given class. If you really want any possible color, I would just include a bit of CSS in your HTML file. <style type='text/css'> .background { background-color:<?php echo($backgroundcolor);?>; } </style> -
I realize you are just testing, but this is a big no-no. Read up on SQL injection if you don't know. Easiest solution is to use PDO's prepared statements as Ch0cu3r describes.
-
Hello Chris, Maybe you know all this, and if so, tell me so and I will shut up. The only reason I harp on it is I feel it is the number one important thing beginners need to understand. Not understanding leads to frustration as I have personally experienced. If you already know, disregard, otherwise... There are two things that talk to each other: the client (AKA the IE/FF/Chrome/etc browser) and the server (AKA your PHP server). The client communicates to the server via three ways: GET request. Basically includes the information in name/pairs in the URL, and is typically used when the client just wants to get more stuff. POST request. Definitely not in the URL, but I think a header or something. Typically used when the client wants to change the state of the server (i.e. write to the database). COOKIE request. Used to tell the server information about who the client is. SESSIONS are also used for this, but are just a glorified COOKIE where the real information is derived from the key given in the cookie. The server just pumps stuff to the client. In your case, it will probably be just HTML, however, it could also be JSON, XML, etc. If you don't get this working right, nothing else will work right. Don't worry about you SQL, etc, unless you know this is working. The whole purpose of var_dump($_POST) or print_r($_POST) is just to know that the client is communicating to the server. Have I made myself clear? Get this part working before doing anything else! If your server is attempting to access POST (or GET or COOKIE) data which doesn't exist, go back to square one, and find why it isn't being sent. Hope this helps!
-
Calculating Inbreeding on a 10 Generation Pedigree
NotionCommotion replied to Triple_Deuce's topic in PHP Coding Help
Don't think you need binary trees, just simple math. What are you summing over? Probably could just do in in SQL, however, I suppose if you want, you could iterate over an array.- 40 replies
-
- inbreeding
- pedigree
-
(and 2 more)
Tagged with:
-
Never mind. Looks like I was trying to access $_SESSION[2996699736].
-
I am getting the following error from my error handler (shown below). I don't know if it is related, but I am using register_shutdown_function in a static class. I don't even know where to start troubleshooting. Any recommendations? Thanks <?php function my_error_handler($e_number, $e_message, $e_file, $e_line, $e_vars) { $message = "An error occurred in script '$e_file' on line $e_line: $e_message (error no: $e_number)";//.print_r($e_vars,1); syslog(LOG_INFO,'my_error_handler: '.$message); } ini_set('display_errors', 1); error_reporting(E_ALL); set_error_handler("my_error_handler"); ?>
-
Your form seems to work fine. Try adding <?php echo('<pre>'.print_r($_POST,1).'</pre>'); ?> right below the opening <body> tag, and you will see your post values. Note that I never used the attribute formaction before, however, it seems to override the form to go to that URL (however, it doesn't validate?). Either get rid of this attribute and let your form tag define the location, or name this main file "add-contactme.php"
-
You mean I need to do some work Do you feel this same way regarding a DB connection? Currently, I create a singleton and call it in any script. class db { private static $instance = NULL; private function __construct() {} //Make private private function __clone(){} //Make private public static function db() { if (!self::$instance) { try{self::$instance = new PDO("mysql:host=localhost;dbname=myDatabase;charset=utf8",'myUsername','myPassword',array(PDO::ATTR_EMULATE_PREPARES=>false,PDO::MYSQL_ATTR_USE_BUFFERED_QUERY=>true,PDO::ATTR_ERRMODE=>PDO::ERRMODE_EXCEPTION,PDO::ATTR_DEFAULT_FETCH_MODE=>PDO::FETCH_ASSOC));} catch(PDOException $e){die(library::sql_error($e,null));} } return self::$instance; } }
-
Yea, it was kind of bugging me too...
-
I literally have no clue what that means? Can you explain where I could put this line to make it useful in my code. Per my original post.... At the top of your script, add the following. This will print out all the fields you are sending in the form to the server. When things are not as you expect, I have found NEVER assume that the values in your variables are correct. Always display them on the screen using print_r() or dump_var(). If your SQL isn't working, similarly just echo the query to the page, make sure it looks okay, and manually execute it using MySQL.
-
Hi Gristoi, How would you recommend implementing a singleton? How I show it, or different? Thanks
-
At the top of your script, add the following. This will print out all the fields you are sending in the form to the server. What do you see? If $_POST["phoneno"], etc are not set, you will get this error notice. So, your issue has nothing to do with the script you are showing, but your HTML form. Make sure the inputs have names, and always use print_r or var_dump as a reality check. echo('<pre>'.print_r($_POST,1).'</pre>');
-
It looks basically okay to me. Your submit form HTML is not well formed, and you might want to check that out. Also, no need to "declare" $conversionValue, $conversionFrom, and $conversionTo at the top. You should also consider some basic validation to ensure $conversionType exists (yes, I know you used a select menu so not a huge deal) and that the submitted values are numbers.
-
Sometimes I have various data which I wish to be available to all scripts. Typically, they are constants which are related, and using PHP's constants makes them difficult to group. As such, my solution has been to create a single global variable such as $GLOBALS['myGlobalVariable'], and dump them all in it. Are there better ways to implement this? For instance, using a singleton? Please provide rational why one solution is better than the other. Thank you <?php class mySinglton { private static $instance = NULL; private function __construct() {} private function __clone(){} public static function mySinglton($values=null) { if (!self::$instance) { self::$instance = new stdClass(); foreach ($values as $key=>$value) { self::$instance->$key=$value; } } return self::$instance; } } class someClass { public $a=1,$b=2,$c=3; } $globals=array( 'foo'=>'bar', 'daysOfWeek'=>array('Sunday','Monday','Tuesday','Wednesday','Thursday','Friday','Saturday'), 'someClass'=>new someClass ); $GLOBALS['myGlobalVariable']=$globals; mySinglton::mySinglton($globals); testIt(); function testIt() { echo('<pre>'.print_r($GLOBALS['myGlobalVariable'],1).'</pre>'); echo('<pre>'.print_r($GLOBALS['myGlobalVariable']['foo'],1).'</pre>'); echo('<pre>'.print_r(mySinglton::mySinglton(),1).'</pre>'); echo('<pre>'.print_r(mySinglton::mySinglton()->foo,1).'</pre>'); } ?>
-
Two sessions in a given script
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
It is not a "real" admin, but I agree with you. I agree What is the purpose of the "site" sub domain (does not "public" or "admin" indicated that it is part of a site?) ? Earlier we talked about joe.public.mysite.com. What are the implications of using public.joe.mysite.com instead? I agree about having preview as a subdomain to admin (and will likely use different session cookie names). Note taking the bait! I know that you know that it was just proof of concept -
Two sessions in a given script
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks requinix, session_write_close() seems to be the answer. My webserver is setup using ServerAlias to send all subdomains to the same script. When a session_id is included in the URL, the following script accesses both the cookie based session as well as the session indicated by the session_id in the URL. If you see any flaws in my logic, please advise, otherwise I think I am good to go. <?php //Use a cookie based session, and read and write to it session_start(); $session_id=session_id(); $_SESSION['s1_'.time()]=time(); echo('Main session using session_id '.$session_id.'<pre>'.print_r($_SESSION,1).'</pre>'); if(isset($_GET['session_id'])) { $new_session_id=$_GET['session_id']; session_write_close(); session_id($new_session_id); session_start(); $_SESSION['s2_'.time()]=time(); echo('Second session using session_id '.$new_session_id.'<pre>'.print_r($_SESSION,1).'</pre>'); //Go back to first session. session_write_close(); session_id($session_id); session_start(); } $_SESSION['s1_'.(2*time())]=2*time(); echo('Main session using session_id '.$session_id.'<pre>'.print_r($_SESSION,1).'</pre>'); $domains=explode('.',$_SERVER['HTTP_HOST']); $ext = array_pop($domains); $primary = array_pop($domains); echo('<ul>'); while($domains){ $domain=implode('.',$domains); $link="http://{$domain}.{$primary}.{$ext}{$_SERVER['PHP_SELF']}"; echo("<li><a href='{$link}'>{$link}</a></li>"); array_shift($domains); } $link="http://{$primary}.{$ext}{$_SERVER['PHP_SELF']}"; echo("<li><a href='{$link}'>{$link}</a></li>"); $link="http://subdomain.{$_SERVER['HTTP_HOST']}{$_SERVER['PHP_SELF']}?session_id={$session_id}"; echo("<li><a href='{$link}'>{$link}</a></li></ul>"); ?> -
Two sessions in a given script
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Promise, I will not make a habit of it! My goal... I have three domains: bob.public.mysite.com bob.admin.mysite.com bob.preview.mysite.com bob.admin.mysite.com generates HTML which creates an IFRAME which points to bob.preview.mysite.com, and includes the session_id of bob.admin.mysite.com in the URL. bob.preview.mysite.com needs to confirm that bob is logged on to bob.admin.mysite.com, and if so, presents HTML for bob.public.mysite.com. My hopes were to use the session_id in the URL to confirm that bob is logged on to bob.admin.mysite.com. When attempting to do so, however, I found that the multiple session_starts() were not allowed. After if confirms, it should return to its original cookie based session. Thoughts? -
Two sessions in a given script
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Access a second session. I've tested the following, however, seem cannot implement session_start() twice. <?php //Use a cookie based session, and read and write to it session_start(); echo('<pre>'.print_r($_SESSION,1).'</pre>'); $data=array(); $_SESSION['foo1']='bar1'; $foo1=$_SESSION['foo1']; if(isset($_GET['session_id'])) { //Get some data from a second session (note that I don't think it matters, but I will not be writing to the second session) $session_id=session_id(); session_id($_GET['session_id']); session_start(); $data=isset($_SESSION['data'])?$_SESSION['data']:array(); //Go back to first session. session_id($session_id); session_start(); } //Read and write to the first session $_SESSION['foo2']='bar2'; $foo2=$_SESSION['foo2']; echo('$_SESSION<pre>'.print_r($_SESSION,1).'</pre>'); echo('$data<pre>'.print_r($data,1).'</pre>'); echo("<a href={$_SERVER['PHP_SELF']}>Without session id</a><br>"); echo("<a href={$_SERVER['PHP_SELF']}?session_id=".session_id().">With session id</a>"); die(); ?>